Tizen Native API
7.0
|
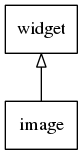
An Elementary image object is a direct realization of elm-image-class, and it allows one to load and display an image file on it, be it from a disk file or from a memory region. Exceptionally, one may also load an Edje group as the contents of the image. In this case, though, most of the functions of the image API will act as a no-op.
One can tune various properties of the image, like:
- pre-scaling,
- smooth scaling,
- orientation,
- aspect ratio during resizes, etc.
An image object may also be made valid source and destination for drag and drop actions, through the elm_image_editable_set() call.
If the image source size is bigger than maximum texture size of the GPU (or also of the software rendering code too), evas can't render it because of such a limitation. If evas just magically always downscales on load if it's too big, then the user has a new bug: "the image is blurry". Potentially any image can cause issue. What if the image is too big to allocate memory for it? A 30000x30000 image will need just a bit under 4GB of RAM to store it. Texture size limitations are something every game developer has to deal with game engines, OpenGL, D3D etc. You can get the maximum image size evas can possibly handle by the calling evas_image_max_size_get() function. If the image size is bigger than this, you can try using load options to pre-scale down on load to lower quality. So use the elm_image_prescale_set() function to scale the image down. Another option is to use the Photocam widget. Photocam solves this issue by loading the prices as needed asynchronously in tiles and automatically using pre-scaling as well So use Photocam if you expect to load very large images.
Signals that you can add callbacks for are:
"drop"
- This is called when a user has dropped an image typed object onto the object in question -- the event info argument is the path to that image file"clicked"
- This is called when a user has clicked the image"download,start"
- This is called when the remote image file download has started."download,progress"
- This is continuously called before the remote image file download has done. The event info data is of type Elm_Image_Progress."download,done"
- This is called when the download has completed."download,error"-
This is called when the download has failed."load,open"
- Triggered when the file has been opened, if async open is enabled (image size is known). (since 1.19)"load,ready"
- Triggered when the image file is ready for display, if preload is enabled. (since 1.19)"load,error"
- Triggered if an async I/O or decoding error occurred, if async open or preload is enabled (since 1.19)"load,cancel"
- Triggered whenever async I/O was cancelled. (since 1.19)
An example of usage for this API follows:
Functions | |
Evas_Object * | elm_image_add (Evas_Object *parent) |
Eina_Bool | elm_image_file_set (Evas_Object *obj, const char *file, const char *group) |
void | elm_image_file_get (const Eo *obj, const char **file, const char **group) |
void | elm_image_prescale_set (Evas_Object *obj, int size) |
int | elm_image_prescale_get (const Evas_Object *obj) |
Eina_Bool | elm_image_mmap_set (Evas_Object *obj, const Eina_File *file, const char *group) |
void | elm_image_smooth_set (Evas_Object *obj, Eina_Bool smooth) |
Control the smooth effect for an image. | |
Eina_Bool | elm_image_smooth_get (const Evas_Object *obj) |
Get the smooth effect for an image. | |
void | elm_image_animated_play_set (Evas_Object *obj, Eina_Bool play) |
Eina_Bool | elm_image_animated_play_get (const Evas_Object *obj) |
void | elm_image_animated_set (Evas_Object *obj, Eina_Bool anim) |
Eina_Bool | elm_image_animated_get (const Evas_Object *obj) |
Eina_Bool | elm_image_animated_available_get (const Evas_Object *obj) |
void | elm_image_editable_set (Evas_Object *obj, Eina_Bool set) |
Contrtol if the image is 'editable'. | |
Eina_Bool | elm_image_editable_get (const Evas_Object *obj) |
Contrtol if the image is 'editable'. | |
Eina_Bool | elm_image_memfile_set (Evas_Object *obj, const void *img, size_t size, const char *format, const char *key) |
Set a location in memory to be used as an image object's source bitmap. | |
void | elm_image_fill_outside_set (Evas_Object *obj, Eina_Bool fill_outside) |
Control if the image fills the entire object area, when keeping the aspect ratio. | |
Eina_Bool | elm_image_fill_outside_get (const Evas_Object *obj) |
Control if the image fills the entire object area, when keeping the aspect ratio. | |
void | elm_image_preload_disabled_set (Evas_Object *obj, Eina_Bool disabled) |
Enable or disable preloading of the image. | |
void | elm_image_orient_set (Evas_Object *obj, Elm_Image_Orient orient) |
Contrtol the image orientation. | |
Elm_Image_Orient | elm_image_orient_get (const Evas_Object *obj) |
Contrtol the image orientation. | |
Evas_Object * | elm_image_object_get (const Evas_Object *obj) |
Get the inlined image object of the image widget. | |
void | elm_image_object_size_get (const Evas_Object *obj, int *w, int *h) |
Get the current size of the image. | |
void | elm_image_resizable_set (Evas_Object *obj, Eina_Bool up, Eina_Bool down) |
Control if the object is (up/down) resizable. | |
void | elm_image_resizable_get (const Evas_Object *obj, Eina_Bool *up, Eina_Bool *down) |
Control if the object is (up/down) resizable. | |
void | elm_image_no_scale_set (Evas_Object *obj, Eina_Bool no_scale) |
Control scaling behaviour of this object. | |
Eina_Bool | elm_image_no_scale_get (const Evas_Object *obj) |
Control scaling behaviour of this object. | |
void | elm_image_aspect_fixed_set (Evas_Object *obj, Eina_Bool fixed) |
Control whether the internal image's aspect ratio is fixed to the original image's aspect ratio. | |
Eina_Bool | elm_image_aspect_fixed_get (const Evas_Object *obj) |
Get whether the internal image's aspect ratio is fixed to the original image's. | |
void | elm_image_async_open_set (Evas_Object *obj, Eina_Bool async) |
Enable asynchronous file I/O for elm_image_file_set. | |
Typedefs | |
typedef struct _Elm_Image_Progress | Elm_Image_Progress |
typedef struct _Elm_Image_Error | Elm_Image_Error |
typedef enum _Elm_Image_Orient_Type | Elm_Image_Orient |
Typedef Documentation
typedef struct _Elm_Image_Error Elm_Image_Error |
Structure associated with smart callback 'download,progress'.
- Since (EFL) :
- 1.8
typedef enum _Elm_Image_Orient_Type Elm_Image_Orient |
Using Evas_Image_Orient enums.
- Since (EFL) :
- 1.14
typedef struct _Elm_Image_Progress Elm_Image_Progress |
Structure associated with smart callback 'download,progress'.
- Since (EFL) :
- 1.8
Enumeration Type Documentation
Using Evas_Image_Orient enums.
- Since (EFL) :
- 1.14
- Enumerator:
Function Documentation
Evas_Object* elm_image_add | ( | Evas_Object * | parent | ) |
Add a new image to the parent.
- Parameters:
-
parent The parent object
- Returns:
- The new object or NULL if it cannot be created
- See also:
- elm_image_file_set()
- Since :
- 2.3
- Examples:
- image_example_01.c.
Eina_Bool elm_image_animated_available_get | ( | const Evas_Object * | obj | ) |
Get whether an image object supports animation or not.
- Returns:
EINA_TRUE
if the image supports animation,EINA_FALSE
otherwise.
This function returns if this Elementary image object's internal image can be animated. Currently Evas only supports GIF animation. If the return value is EINA_FALSE, other elm_image_animated_xxx
API calls won't work.
- See also:
- elm_image_animated_set()
- Since (EFL) :
- 1.7
- Since :
- 2.3
Eina_Bool elm_image_animated_get | ( | const Evas_Object * | obj | ) |
Get whether an image object has animation enabled or not.
- Parameters:
-
obj The image object
- Returns:
EINA_TRUE
if the image has animation enabled,EINA_FALSE
otherwise.
- See also:
- elm_image_animated_set()
- Since (EFL) :
- 1.7
- Since :
- 2.3
Eina_Bool elm_image_animated_play_get | ( | const Evas_Object * | obj | ) |
Get whether an image object is under animation or not.
- Parameters:
-
obj The image object
- Returns:
EINA_TRUE
, if the image is being animated,EINA_FALSE
otherwise.
- See also:
- elm_image_animated_play_get()
- Since (EFL) :
- 1.7
- Since :
- 2.3
void elm_image_animated_play_set | ( | Evas_Object * | obj, |
Eina_Bool | play | ||
) |
Start or stop an image object's animation.
To actually start playing any image object's animation, if it supports it, one must do something like:
if (elm_image_animated_available_get(img)) { elm_image_animated_set(img, EINA_TRUE); elm_image_animated_play_set(img, EINA_TRUE); }
elm_image_animated_set() will enable animation on the image, but not start it yet. This is the function one uses to start and stop animations on image objects.
- Since (EFL) :
- 1.7
- Parameters:
-
obj The image object [in] play EINA_TRUE
to start the animation,EINA_FALSE
otherwise. Default isEINA_FALSE
.
- Since :
- 2.3
void elm_image_animated_set | ( | Evas_Object * | obj, |
Eina_Bool | anim | ||
) |
Set whether an image object (which supports animation) is to animate itself or not.
An image object, even if it supports animation, will be displayed by default without animation. Call this function with animated set to EINA_TRUE
to enable its animation. To start or stop the animation, actually, use elm_image_animated_play_set().
- Since (EFL) :
- 1.7
- Parameters:
-
obj The image object [in] anim EINA_TRUE
if the object is to animate itself,EINA_FALSE
otherwise. Default isEINA_FALSE
.
- Since :
- 2.3
Eina_Bool elm_image_aspect_fixed_get | ( | const Evas_Object * | obj | ) |
Get whether the internal image's aspect ratio is fixed to the original image's.
- Returns:
- @ true if the aspect ratio is fixed
- Since :
- 2.3
void elm_image_aspect_fixed_set | ( | Evas_Object * | obj, |
Eina_Bool | fixed | ||
) |
Control whether the internal image's aspect ratio is fixed to the original image's aspect ratio.
- Parameters:
-
[in] fixed @ true if the aspect ratio is fixed
- Since :
- 2.3
- Examples:
- image_example_01.c.
void elm_image_async_open_set | ( | Evas_Object * | obj, |
Eina_Bool | async | ||
) |
Enable asynchronous file I/O for elm_image_file_set.
- Parameters:
-
obj The image object [in] async @ true will make elm_image_file_set() an asynchronous operation
If true
, this will make elm_image_file_set() an asynchronous operation. Use of this function is not recommended and the standard EO-based asynchronous I/O API should be preferred instead.
- Since (EFL) :
- 1.19
Eina_Bool elm_image_editable_get | ( | const Evas_Object * | obj | ) |
Contrtol if the image is 'editable'.
This means the image is a valid drag target for drag and drop, and can be cut or pasted too.
- Returns:
- Turn on or off editability. Default is
false
.
- Since :
- 2.3
void elm_image_editable_set | ( | Evas_Object * | obj, |
Eina_Bool | set | ||
) |
Contrtol if the image is 'editable'.
This means the image is a valid drag target for drag and drop, and can be cut or pasted too.
- Parameters:
-
[in] set Turn on or off editability. Default is false
.
- Since :
- 2.3
- Examples:
- image_example_01.c.
void elm_image_file_get | ( | const Eo * | obj, |
const char ** | file, | ||
const char ** | group | ||
) |
Get the file that will be used as image.
- See also:
- elm_image_file_set()
- Parameters:
-
[out] file The path to file that will be used as image source [out] group The group that the image belongs to, in case it's an EET (including Edje case) file. This can be used as a key inside evas image cache if this is a normal image file not eet file.
- Since :
- 2.3
- Examples:
- icon_example_01.c.
Eina_Bool elm_image_file_set | ( | Evas_Object * | obj, |
const char * | file, | ||
const char * | group | ||
) |
Set the file that will be used as the image's source.
- Parameters:
-
obj The image object file The path to file that will be used as image source group The group that the image belongs to, in case it's an EET (including Edje case) file. This can be used as a key inside evas image cache if this is a normal image file not eet file.
- Returns:
- (
EINA_TRUE
= success,EINA_FALSE
= error)
- See also:
- elm_image_file_get()
- Note:
- This function will trigger the Edje file case based on the extension of the file string (expects
".edj"
, for this case). -
If you use animated gif image and create multiple image objects with one gif image file, you should set the
group
differently for each object. Or image objects will share one evas image cache entry and you will get unwanted frames.
- Since :
- 2.3
Eina_Bool elm_image_fill_outside_get | ( | const Evas_Object * | obj | ) |
Control if the image fills the entire object area, when keeping the aspect ratio.
When the image should keep its aspect ratio even if resized to another aspect ratio, there are two possibilities to resize it: keep the entire image inside the limits of height and width of the object ($fill_outside is false
) or let the extra width or height go outside of the object, and the image will fill the entire object ($fill_outside is true
).
- Note:
- This option will have no effect if elm_image_aspect_fixed_get is set to
false
.
- Returns:
true
if the object is filled outside,false
otherwise. Default isfalse
.
- Since :
- 2.3
void elm_image_fill_outside_set | ( | Evas_Object * | obj, |
Eina_Bool | fill_outside | ||
) |
Control if the image fills the entire object area, when keeping the aspect ratio.
When the image should keep its aspect ratio even if resized to another aspect ratio, there are two possibilities to resize it: keep the entire image inside the limits of height and width of the object ($fill_outside is false
) or let the extra width or height go outside of the object, and the image will fill the entire object ($fill_outside is true
).
- Note:
- This option will have no effect if elm_image_aspect_fixed_get is set to
false
.
- Parameters:
-
[in] fill_outside true
if the object is filled outside,false
otherwise. Default isfalse
.
- Since :
- 2.3
- Examples:
- icon_example_01.c, and image_example_01.c.
Eina_Bool elm_image_memfile_set | ( | Evas_Object * | obj, |
const void * | img, | ||
size_t | size, | ||
const char * | format, | ||
const char * | key | ||
) |
Set a location in memory to be used as an image object's source bitmap.
This function is handy when the contents of an image file are mapped in memory, for example.
The format
string should be something like $"png", $"jpg", $"tga", $"tiff", $"bmp" etc, when provided ($NULL, on the contrary). This improves the loader performance as it tries the "correct" loader first, before trying a range of other possible loaders until one succeeds.
- Parameters:
-
[in] img The binary data that will be used as image source [in] size The size of binary data blob img
[in] format (Optional) expected format of img
bytes[in] key Optional indexing key of img
to be passed to the image loader (eg. ifimg
is a memory-mapped EET file)
- Returns:
true
= success,false
= error
- Since (EFL) :
- 1.7
- Since :
- 2.3
Eina_Bool elm_image_mmap_set | ( | Evas_Object * | obj, |
const Eina_File * | file, | ||
const char * | group | ||
) |
Set the file that will be used as the image's source.
- Parameters:
-
obj The image object file The handler to an Eina_File that will be used as image source group The group that the image belongs to, in case it's an EET (including Edje case) file. This can be used as a key inside evas image cache if this is a normal image file not eet file.
- Returns:
- (
EINA_TRUE
= success,EINA_FALSE
= error)
- See also:
- elm_image_file_set()
- Note:
- This function will trigger the Edje file case based on the extension of the file string use to create the Eina_File (expects
".edj"
, for this case). -
If you use animated gif image and create multiple image objects with one gif image file, you should set the
group
differently for each object. Or image objects will share one evas image cache entry and you will get unwanted frames.
- Since :
- 3.0
Eina_Bool elm_image_no_scale_get | ( | const Evas_Object * | obj | ) |
Control scaling behaviour of this object.
This function disables scaling of the elm_image widget through the function elm_object_scale_set(). However, this does not affect the widget size/resize in any way. For that effect, take a look at elm_image_resizable_get and efl_gfx_entity_scale_get
- Returns:
true
if the object is not scalable,false
otherwise. Default isfalse
.
- Since :
- 2.3
void elm_image_no_scale_set | ( | Evas_Object * | obj, |
Eina_Bool | no_scale | ||
) |
Control scaling behaviour of this object.
This function disables scaling of the elm_image widget through the function elm_object_scale_set(). However, this does not affect the widget size/resize in any way. For that effect, take a look at elm_image_resizable_get and efl_gfx_entity_scale_get
- Parameters:
-
[in] no_scale true
if the object is not scalable,false
otherwise. Default isfalse
.
- Since :
- 2.3
- Examples:
- icon_example_01.c, image_example_01.c, and win_example.c.
Evas_Object* elm_image_object_get | ( | const Evas_Object * | obj | ) |
Get the inlined image object of the image widget.
This function allows one to get the underlying Evas_Object
of type Image from this elementary widget. It can be useful to do things like get the pixel data, save the image to a file, etc.
- Note:
- Be careful to not manipulate it, as it is under control of elementary.
- Warning:
- It doesn't guarantee the inlined object must be a type of Evas_Object_Image. It would be one of
Evas_Object_Image
orEdje_Object
depending on image file type.
- Returns:
- The inlined image object, or NULL if none exists
- Since :
- 2.3
void elm_image_object_size_get | ( | const Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Get the current size of the image.
This is the real size of the image, not the size of the object.
- Parameters:
-
[out] w Pointer to store width, or NULL. [out] h Pointer to store height, or NULL.
- Since :
- 2.3
Elm_Image_Orient elm_image_orient_get | ( | const Evas_Object * | obj | ) |
Contrtol the image orientation.
This function allows to rotate or flip the given image.
- Returns:
- The image orientation Elm.Image.Orient Default is ELM_IMAGE_ORIENT_NONE.
- Since :
- 2.3
void elm_image_orient_set | ( | Evas_Object * | obj, |
Elm_Image_Orient | orient | ||
) |
Contrtol the image orientation.
This function allows to rotate or flip the given image.
- Parameters:
-
[in] orient The image orientation Elm.Image.Orient Default is ELM_IMAGE_ORIENT_NONE.
- Since :
- 2.3
- Examples:
- image_example_01.c.
void elm_image_preload_disabled_set | ( | Evas_Object * | obj, |
Eina_Bool | disabled | ||
) |
Enable or disable preloading of the image.
- Parameters:
-
[in] disabled If true, preloading will be disabled
- Since :
- 2.3
int elm_image_prescale_get | ( | const Evas_Object * | obj | ) |
Get the prescale size for the image
- Parameters:
-
obj The image object
- Returns:
- The prescale size
- See also:
- elm_image_prescale_set()
- Since :
- 2.3
void elm_image_prescale_set | ( | Evas_Object * | obj, |
int | size | ||
) |
Set the prescale size for the image
- Parameters:
-
obj The image object size The prescale size. This value is used for both width and height.
This function sets a new size for pixmap representation of the given image. It allows the image to be loaded already in the specified size, reducing the memory usage and load time when loading a big image with load size set to a smaller size.
It's equivalent to the elm_bg_load_size_set() function for bg.
- Note:
- this is just a hint, the real size of the pixmap may differ depending on the type of image being loaded, being bigger than requested.
- Since :
- 2.3
void elm_image_resizable_get | ( | const Evas_Object * | obj, |
Eina_Bool * | up, | ||
Eina_Bool * | down | ||
) |
Control if the object is (up/down) resizable.
This function limits the image resize ability. If size_up
is set to false
, the object can't have its height or width resized to a value higher than the original image size. Same is valid for size_down
.
- Parameters:
-
[out] up A bool to set if the object is resizable up. Default is true
.[out] down A bool to set if the object is resizable down. Default is true
.
- Since :
- 2.3
void elm_image_resizable_set | ( | Evas_Object * | obj, |
Eina_Bool | up, | ||
Eina_Bool | down | ||
) |
Control if the object is (up/down) resizable.
This function limits the image resize ability. If size_up
is set to false
, the object can't have its height or width resized to a value higher than the original image size. Same is valid for size_down
.
- Parameters:
-
[in] up A bool to set if the object is resizable up. Default is true
.[in] down A bool to set if the object is resizable down. Default is true
.
- Since :
- 2.3
Eina_Bool elm_image_smooth_get | ( | const Evas_Object * | obj | ) |
Get the smooth effect for an image.
Get the scaling algorithm to be used when scaling the image. Smooth scaling provides a better resulting image, but is slower.
The smooth scaling should be disabled when making animations that change the image size, since it will be faster. Animations that don't require resizing of the image can keep the smooth scaling enabled (even if the image is already scaled, since the scaled image will be cached).
- Returns:
true
if smooth scaling should be used,false
otherwise. Default istrue
.
- Since :
- 2.3
void elm_image_smooth_set | ( | Evas_Object * | obj, |
Eina_Bool | smooth | ||
) |
Control the smooth effect for an image.
Set the scaling algorithm to be used when scaling the image. Smooth scaling provides a better resulting image, but is slower.
The smooth scaling should be disabled when making animations that change the image size, since it will be faster. Animations that don't require resizing of the image can keep the smooth scaling enabled (even if the image is already scaled, since the scaled image will be cached).
- Parameters:
-
[in] smooth true
if smooth scaling should be used,false
otherwise. Default istrue
.
- Since :
- 2.3
- Examples:
- icon_example_01.c, and image_example_01.c.