Tizen Native API
7.0
|
The Voice control API provides functions for registering command and getting notification when registered command is recognized.
Required Header
#include <voice_control.h>
Overview
A main function of Voice Control API register command and gets notification for recognition result. Applications can add their own commands and be provided result when their command is recognized by user voice input. To use of Voice Control, use the following steps:
1. Initialize
2. Register callback functions for notifications
3. Connect to voice control service asynchronously. The state should be changed to Ready
4. Make command list as the following step and Step 4 is called repeatedly for each command which an application wants
4-1. Create command list handle
4-2. Create command handle
4-3. Set command and type for command handle
4-4. Add command handle to command list
5. Set command list for recognition
6. Set an invocation name for an application
7. Get recognition results
8. Request the dialogue
9. If an application wants to finish voice control,
9-1. Destroy command and command list handle
9-2. Deinitialize
An application can obtain command handle from command list, and also get information from handle. The Voice Control API also notifies you (by callback mechanism) when the states of client and service are changed, command is recognized, current language is changed or error occurred. An application should register callback functions: vc_state_changed_cb(), vc_service_state_changed_cb(), vc_result_cb(), vc_current_language_changed_cb(), vc_error_cb().
State Diagram
The following diagram shows the life cycle and the states of the Voice Control.
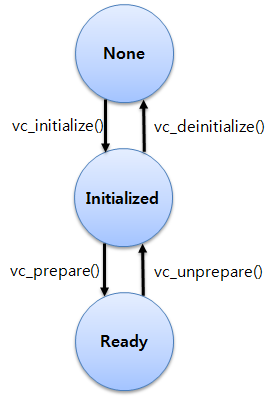
The following diagram shows the states of Voice Control service.
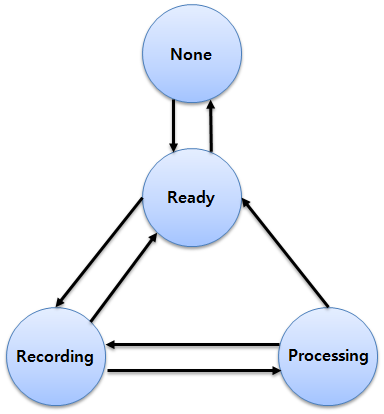
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
vc_initialize() | None | Initialized | SYNC |
vc_deinitialize() | Initialized | None | SYNC |
vc_prepare() | Initialized | Ready | ASYNC |
vc_unprepare() | Ready | Initialized | SYNC |
State Dependent Function Calls
The following table shows state-dependent function calls. It is forbidden to call functions listed below in wrong states. Violation of this rule may result in an unpredictable behavior.
Related Features
This API is related with the following features:
- http://tizen.org/feature/microphone
- http://tizen.org/feature/speech.control
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Copyright (c) 2011-2016 Samsung Electronics Co., Ltd All Rights Reserved
Licensed under the Apache License, Version 2.0 (the License); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an AS IS BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
Functions | |
int | vc_initialize (void) |
Initializes voice control. | |
int | vc_deinitialize (void) |
Deinitializes voice control. | |
int | vc_prepare (void) |
Connects the voice control service. | |
int | vc_unprepare (void) |
Disconnects the voice control service. | |
int | vc_foreach_supported_languages (vc_supported_language_cb callback, void *user_data) |
Retrieves all supported languages using callback function. | |
int | vc_get_current_language (char **language) |
Gets current language. | |
int | vc_get_state (vc_state_e *state) |
Gets current state of voice control client. | |
int | vc_get_service_state (vc_service_state_e *state) |
Gets current state of voice control service. | |
int | vc_get_system_command_list (vc_cmd_list_h *vc_sys_cmd_list) |
Gets the system command list. | |
int | vc_set_invocation_name (const char *name) |
Sets the invocation name. | |
int | vc_set_server_dialog (const char *app_id, const char *credential) |
Requests to set app id which is to want to ask the server dialogue. | |
int | vc_unset_server_dialog (const char *app_id) |
Requests to unset app id which is to not want to ask the server dialogue. | |
int | vc_request_dialog (const char *disp_text, const char *utt_text, bool auto_start) |
Requests to start the dialogue. | |
int | vc_set_command_list (vc_cmd_list_h vc_cmd_list, int type) |
Sets command list. | |
int | vc_unset_command_list (int type) |
Unsets command list. | |
int | vc_get_result (vc_result_cb callback, void *user_data) |
Gets the recognition result. | |
int | vc_set_result_cb (vc_result_cb callback, void *user_data) |
Sets a callback function for getting recognition result. | |
int | vc_unset_result_cb (void) |
Unsets the callback function. | |
int | vc_set_service_state_changed_cb (vc_service_state_changed_cb callback, void *user_data) |
Sets a callback function to be called when service state is changed. | |
int | vc_unset_service_state_changed_cb (void) |
Unsets the callback function. | |
int | vc_set_state_changed_cb (vc_state_changed_cb callback, void *user_data) |
Sets a callback function to be called when state is changed. | |
int | vc_unset_state_changed_cb (void) |
Unsets the callback function. | |
int | vc_set_current_language_changed_cb (vc_current_language_changed_cb callback, void *user_data) |
Sets a callback function to be called when current language is changed. | |
int | vc_unset_current_language_changed_cb (void) |
Unsets the callback function. | |
int | vc_set_error_cb (vc_error_cb callback, void *user_data) |
Sets a callback function to be called when an error occurred. | |
int | vc_unset_error_cb (void) |
Unsets the callback function. | |
int | vc_tts_request (const char *text, const char *language, bool to_vc_manager, int *utt_id) |
Requests to send TTS streaming data, asynchronously. | |
int | vc_tts_cancel (int utt_id) |
Requests to cancel TTS streaming data. | |
int | vc_tts_get_synthesized_audio_details (int *rate, vc_audio_channel_e *channel, vc_audio_type_e *audio_type) |
Gets the TTS audio details. | |
int | vc_tts_set_streaming_cb (vc_tts_streaming_cb callback, void *user_data) |
Sets the TTS streaming callback function. | |
int | vc_tts_unset_streaming_cb (void) |
Unsets the TTS streaming callback function. | |
int | vc_tts_set_utterance_status_cb (vc_tts_utterance_status_cb callback, void *user_data) |
Sets the TTS utterance status callback function. | |
int | vc_tts_unset_utterance_status_cb (void) |
Unsets the TTS utterance status callback function. | |
Typedefs | |
typedef void(* | vc_result_cb )(vc_result_event_e event, vc_cmd_list_h vc_cmd_list, const char *result, void *user_data) |
Called when client gets the recognition result. | |
typedef void(* | vc_current_language_changed_cb )(const char *previous, const char *current, void *user_data) |
Called when default language is changed. | |
typedef bool(* | vc_supported_language_cb )(const char *language, void *user_data) |
Called to retrieve supported language. | |
typedef void(* | vc_state_changed_cb )(vc_state_e previous, vc_state_e current, void *user_data) |
Called when the state of voice control client is changed. | |
typedef void(* | vc_service_state_changed_cb )(vc_service_state_e previous, vc_service_state_e current, void *user_data) |
Called when the state of voice control service is changed. | |
typedef void(* | vc_error_cb )(vc_error_e reason, void *user_data) |
Called when error occurred. | |
typedef void(* | vc_tts_streaming_cb )(vc_tts_event_e event, char *buffer, int len, int utt_id, void *user_data) |
Called when the client receives TTS streaming data from the VC engine service. | |
typedef void(* | vc_tts_utterance_status_cb )(int utt_id, vc_tts_utterance_status_e status, void *user_data) |
Called when the client receives the TTS utterance status. | |
Defines | |
#define | VC_COMMAND_TYPE_FOREGROUND 1 |
Definition for foreground command type. | |
#define | VC_COMMAND_TYPE_BACKGROUND 2 |
Definition for background command type. | |
#define | VC_DIALOG_END 0 |
Definition for ended dialog. | |
#define | VC_DIALOG_CONTINUE 1 |
Definition for continued dialog. |
Define Documentation
#define VC_COMMAND_TYPE_BACKGROUND 2 |
Definition for background command type.
- Since :
- 2.4
#define VC_COMMAND_TYPE_FOREGROUND 1 |
Definition for foreground command type.
- Since :
- 2.4
#define VC_DIALOG_CONTINUE 1 |
Definition for continued dialog.
- Since :
- 3.0
#define VC_DIALOG_END 0 |
Definition for ended dialog.
- Since :
- 3.0
Typedef Documentation
typedef void(* vc_current_language_changed_cb)(const char *previous, const char *current, void *user_data) |
Called when default language is changed.
- Since :
- 2.4
- Parameters:
-
[in] previous Previous language [in] current Current language [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback to detect changing mode.
- See also:
- vc_set_current_language_changed_cb()
typedef void(* vc_error_cb)(vc_error_e reason, void *user_data) |
Called when error occurred.
- Since :
- 2.4
- Parameters:
-
[in] reason The error type (e.g. VC_ERROR_OUT_OF_MEMORY, VC_ERROR_TIMED_OUT) [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback to detect error.
- See also:
- vc_set_error_cb()
typedef void(* vc_result_cb)(vc_result_event_e event, vc_cmd_list_h vc_cmd_list, const char *result, void *user_data) |
Called when client gets the recognition result.
- Since :
- 2.4
- Remarks:
- If the duplicated commands are recognized, the event(e.g. VC_RESULT_EVENT_REJECTED) of command may be rejected for selecting command as priority. If you set similar or same commands or the recognized results are multi-results, vc_cmd_list has the multi commands.
- Parameters:
-
[in] event The result event (e.g. VC_RESULT_EVENT_RESULT_SUCCESS, VC_RESULT_EVENT_REJECTED) [in] vc_cmd_list The recognized command list [in] result The spoken text [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers callback function.
- See also:
- vc_set_result_cb()
typedef void(* vc_service_state_changed_cb)(vc_service_state_e previous, vc_service_state_e current, void *user_data) |
Called when the state of voice control service is changed.
- Since :
- 2.4
- Parameters:
-
[in] previous A previous state [in] current A current state [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback to detect changing service state.
- See also:
- vc_set_service_state_changed_cb()
typedef void(* vc_state_changed_cb)(vc_state_e previous, vc_state_e current, void *user_data) |
Called when the state of voice control client is changed.
- Since :
- 2.4
- Parameters:
-
[in] previous A previous state [in] current A current state [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback to detect changing state.
- See also:
- vc_set_state_changed_cb()
typedef bool(* vc_supported_language_cb)(const char *language, void *user_data) |
Called to retrieve supported language.
- Since :
- 2.4
- Parameters:
-
[in] language A language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code. For example, "ko_KR" for Korean, "en_US" for American English [in] user_data The user data passed from the foreach function
- Returns:
true
to continue with the next iteration of the loop,false
to break out of the loop
- Precondition:
- The function will invoke this callback.
typedef void(* vc_tts_streaming_cb)(vc_tts_event_e event, char *buffer, int len, int utt_id, void *user_data) |
Called when the client receives TTS streaming data from the VC engine service.
- Since :
- 5.5
- Remarks:
- The buffer must be released with free() by you when you no longer need it.
- Parameters:
-
[in] event The TTS event [in] buffer The TTS streaming data [in] len The length of the TTS streaming data [in] utt_id The utterance id [in] user_data The user data passed from the callback registration function
- Precondition:
- The application registers the callback function using vc_tts_set_streaming_cb().
- See also:
- vc_tts_set_streaming_cb()
typedef void(* vc_tts_utterance_status_cb)(int utt_id, vc_tts_utterance_status_e status, void *user_data) |
Called when the client receives the TTS utterance status.
- Since :
- 5.5
- Parameters:
-
[in] utt_id The utterance id [in] status The new TTS utterance status (e.g. VC_TTS_UTTERANCE_STARTED, VC_TTS_UTTERANCE_COMPLETED, and so on) [in] user_data The user data passed from the callback registration function
- Precondition:
- The application registers the callback function using vc_tts_set_utterance_status_cb().
- See also:
- vc_tts_set_utterance_status_cb()
Enumeration Type Documentation
enum vc_audio_channel_e |
enum vc_audio_type_e |
enum vc_error_e |
Enumeration for error codes.
- Since :
- 2.4
- Enumerator:
enum vc_feedback_event_e |
enum vc_result_event_e |
enum vc_service_state_e |
enum vc_state_e |
enum vc_tts_event_e |
Function Documentation
int vc_deinitialize | ( | void | ) |
Deinitializes voice control.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state VC_ERROR_OPERATION_FAILED Operation failure
- See also:
- vc_initialize()
int vc_foreach_supported_languages | ( | vc_supported_language_cb | callback, |
void * | user_data | ||
) |
Retrieves all supported languages using callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_OPERATION_FAILED Operation failure VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED or VC_STATE_READY.
- Postcondition:
- This function invokes vc_supported_language_cb() repeatedly for getting languages.
int vc_get_current_language | ( | char ** | language | ) |
Gets current language.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, language must be released with free() by you when you no longer need it.
- Parameters:
-
[out] language A language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code. For example, "ko_KR" for Korean, "en_US" for American English
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_OUT_OF_MEMORY Out of memory VC_ERROR_OPERATION_FAILED Operation failure VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED or VC_STATE_READY.
- See also:
- vc_foreach_supported_languages()
int vc_get_result | ( | vc_result_cb | callback, |
void * | user_data | ||
) |
Gets the recognition result.
- Since :
- 3.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to get recognition result [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
- See also:
- vc_result_cb()
int vc_get_service_state | ( | vc_service_state_e * | state | ) |
Gets current state of voice control service.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[out] state The current state
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
int vc_get_state | ( | vc_state_e * | state | ) |
Gets current state of voice control client.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[out] state The current state
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter
int vc_get_system_command_list | ( | vc_cmd_list_h * | vc_sys_cmd_list | ) |
Gets the system command list.
- Since :
- 3.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- In the system command list, there are system commands predefined by product manufacturers. Those commands have the highest priority. Therefore, the user can not set any commands same with the system commands. The vc_sys_cmd_list must be released using vc_cmd_list_destroy() when it is no longer required.
- Parameters:
-
[out] vc_sys_cmd_list System command list handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The service state should be VC_SERVICE_STATE_READY.
- See also:
- vc_cmd_list_destroy()
int vc_initialize | ( | void | ) |
Initializes voice control.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, vc must be released with vc_deinitialize().
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_OUT_OF_MEMORY Out of memory VC_ERROR_OPERATION_FAILED Operation failure
- Postcondition:
- If this function is called, the state will be VC_STATE_INITIALIZED.
- See also:
- vc_deinitialize()
int vc_prepare | ( | void | ) |
Connects the voice control service.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state VC_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- Postcondition:
- If this function is called, the state will be VC_STATE_READY.
- See also:
- vc_unprepare()
int vc_request_dialog | ( | const char * | disp_text, |
const char * | utt_text, | ||
bool | auto_start | ||
) |
Requests to start the dialogue.
Using this function, the developer can request starting the dialogue to the framework. When the developer requests the dialogue, two types of texts, disp_text and utt_text, can be sent by this function. disp_text is a text for displaying, and utt_text is that for uttering. For example, if disp_text is "October 10th" and utt_text is "Today is October 10th.", "October 10th" will be displayed on the screen and "Today is October 10th." will be spoken. Also, the developer can set whether the dialogue starts automatically or not, using auto_start. If the developer sets auto_start as true
, the framework will start to record next speech and continue the dialogue.
- Since :
- 3.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If auto_start is
true
, the recognition will start again. In this case, it can be restarted up to 4 times. disp_text and utt_text allowNULL
. However, it is not allowed to set both disp_text and utt_text asNULL
.
- Parameters:
-
[in] disp_text Text to be displayed on the screen [in] utt_text Text to be spoken [in] auto_start A variable for setting whether the dialog session will be restarted automatically or not
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The service state should be VC_SERVICE_STATE_READY.
int vc_set_command_list | ( | vc_cmd_list_h | vc_cmd_list, |
int | type | ||
) |
Sets command list.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- The command type is valid for VC_COMMAND_TYPE_FOREGROUND or VC_COMMAND_TYPE_BACKGROUND. The matched commands of command list should be set and they should include type and command text at least.
- Parameters:
-
[in] vc_cmd_list Command list handle [in] type Command type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
- See also:
- vc_unset_command_list()
int vc_set_current_language_changed_cb | ( | vc_current_language_changed_cb | callback, |
void * | user_data | ||
) |
Sets a callback function to be called when current language is changed.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_set_error_cb | ( | vc_error_cb | callback, |
void * | user_data | ||
) |
Sets a callback function to be called when an error occurred.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_error_cb()
- vc_unset_error_cb()
int vc_set_invocation_name | ( | const char * | name | ) |
Sets the invocation name.
Invocation name is used to activate background commands. The invocation name can be the same as the application name or any other phrase. For example, an application "Tizen Sample" has a background command, "Play music", and the invocation name of the application is set to "Tizen Sample". In order to activate the background command, users can say "Tizen Sample, Play music". The invocation name is dependent on the current language. For example, if the current language is "en_US"(English), the invocation name is also "en_US". If the current language is "ja_JP"(Japanese) and the invocation name is "en_US", the invocation name will not be recognized. This function should be called before vc_set_command_list().
- Since :
- 3.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If name is
NULL
, the invocation name will be unset.
- Parameters:
-
[in] name Invocation name that an application wants to be invoked by
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
- See also:
- vc_set_command_list()
int vc_set_result_cb | ( | vc_result_cb | callback, |
void * | user_data | ||
) |
Sets a callback function for getting recognition result.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_result_cb()
- vc_unset_result_cb()
int vc_set_server_dialog | ( | const char * | app_id, |
const char * | credential | ||
) |
Requests to set app id which is to want to ask the server dialogue.
Using this function, the developer can request registering the application on vc framework. If developer requests to register app_id with credential which is valid, the application will be set on vc framework. and then, when the developer requests the dialogue using vc_request_dialog(), dialog from specific engine server will be played by vc framework.
- Since :
- 5.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If app_id is
NULL
, the API tries to get app ID using app manager framework. However, getting app ID may be failed.
- Parameters:
-
[in] app_id App id which is to want to ask server dialog. [in] credential Credential key.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The service state should be VC_SERVICE_STATE_READY.
- See also:
- vc_unset_server_dialog()
int vc_set_service_state_changed_cb | ( | vc_service_state_changed_cb | callback, |
void * | user_data | ||
) |
Sets a callback function to be called when service state is changed.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_set_state_changed_cb | ( | vc_state_changed_cb | callback, |
void * | user_data | ||
) |
Sets a callback function to be called when state is changed.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] callback Callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_tts_cancel | ( | int | utt_id | ) |
Requests to cancel TTS streaming data.
- Warning:
- This is not for use by third-party applications.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Parameters:
-
[in] utt_id The utterance id
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
- See also:
- vc_tts_request()
int vc_tts_get_synthesized_audio_details | ( | int * | rate, |
vc_audio_channel_e * | channel, | ||
vc_audio_type_e * | audio_type | ||
) |
Gets the TTS audio details.
- Warning:
- This is not for use by third-party applications.
Using this function, the developer can get details of synthesized audio data which is requested by vc_tts_request() function.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Parameters:
-
[out] rate The audio sampling rate [out] channel The audio channel [out] audio_type The audio type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
int vc_tts_request | ( | const char * | text, |
const char * | language, | ||
bool | to_vc_manager, | ||
int * | utt_id | ||
) |
Requests to send TTS streaming data, asynchronously.
- Warning:
- This is not for use by third-party applications.
Using this function, the developer can request text to speech to the framework. When the developer requests the TTS with language, VC engine will send PCM data which is synthesized using VC engine's own persona. If to_vc_manager is true, the synthesized PCM data will be delivered to the VC manager, otherwise it will be delivered to the VC client For example, if text is "Alarm is set as 7 PM" and to_vc_manager is true, the PCM data corresponding "Alarm is set as 7 PM" will be delivered to VC manager client, and then it will be spoken in VC manager. If to_vc_manager is false, you will receive PCM data through the vc_tts_streaming_cb() callback function if it was set using vc_tts_set_streaming_cb(). This function is executed asynchronously, so if there is an error while synthesizing, vc_error_cb() will be called.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Parameters:
-
[in] text The text to be requested for TTS [in] language The language is specified as an ISO 3166 alpha-2 two-letter country code followed by ISO 639-1 for the two-letter language code. For example, "ko_KR" for Korean, "en_US" for American English [in] to_vc_manager The value for selection between VC client and VC manager
Iftrue
, the synthesized PCM data will be delivered to the VC manager, otherwise it will be delivered to the VC client[out] utt_id The utterance id
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state VC_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be VC_STATE_READY.
- See also:
- vc_tts_cancel()
int vc_tts_set_streaming_cb | ( | vc_tts_streaming_cb | callback, |
void * | user_data | ||
) |
Sets the TTS streaming callback function.
- Warning:
- This is not for use by third-party applications.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Parameters:
-
[in] callback The callback function [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_tts_set_utterance_status_cb | ( | vc_tts_utterance_status_cb | callback, |
void * | user_data | ||
) |
Sets the TTS utterance status callback function.
- Warning:
- This is not for use by third-party applications.
Using this function, the developer can set the utterance status callback to be called when the VC manager client starts or stops playing TTS PCM data which was requested to be synthesized with the vc_tts_request() function. This function is called when to_vc_manager in the vc_tts_request() function call is true
.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Parameters:
-
[in] callback The callback function [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state VC_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_tts_unset_streaming_cb | ( | void | ) |
Unsets the TTS streaming callback function.
- Warning:
- This is not for use by third-party applications.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_tts_unset_utterance_status_cb | ( | void | ) |
Unsets the TTS utterance status callback function.
- Warning:
- This is not for use by third-party applications.
- Since :
- 5.5
- Privilege Level:
- partner
- Privilege:
- http://tizen.org/privilege/voicecontrol.tts
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
int vc_unprepare | ( | void | ) |
Disconnects the voice control service.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
- Postcondition:
- If this function is called, the state will be VC_STATE_INITIALIZED.
- See also:
- vc_prepare()
int vc_unset_command_list | ( | int | type | ) |
Unsets command list.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] type Command type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_READY.
- See also:
- vc_set_command_list()
int vc_unset_current_language_changed_cb | ( | void | ) |
Unsets the callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_set_current_language_changed_cb()
int vc_unset_error_cb | ( | void | ) |
Unsets the callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_set_error_cb()
int vc_unset_result_cb | ( | void | ) |
Unsets the callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_set_result_cb()
int vc_unset_server_dialog | ( | const char * | app_id | ) |
Requests to unset app id which is to not want to ask the server dialogue.
Using this function, the developer can disable function to ask dialog based on server.
- Since :
- 5.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If app_id is
NULL
, the API tries to get app ID using app manager framework. However, getting app ID may be failed.
- Parameters:
-
[in] app_id App id which is to not want to ask server dialog.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_PARAMETER Invalid parameter VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The service state should be VC_SERVICE_STATE_READY.
- See also:
- vc_set_server_dialog()
int vc_unset_service_state_changed_cb | ( | void | ) |
Unsets the callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_set_service_state_changed_cb()
int vc_unset_state_changed_cb | ( | void | ) |
Unsets the callback function.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
VC_ERROR_NONE Successful VC_ERROR_NOT_SUPPORTED Not supported VC_ERROR_PERMISSION_DENIED Permission denied VC_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be VC_STATE_INITIALIZED.
- See also:
- vc_set_state_changed_cb()