Tizen Native API
7.0
|
This group of functions is applied to an Ecore_Con_Server object. It doesn't mean that they should be used in the server application, but on the server object. In fact, most of them should be used in the client application, when retrieving information or sending data.
Setting up a server is very simple: you just need to start it with ecore_con_server_add() and setup some callbacks to the events ECORE_CON_EVENT_CLIENT_ADD, ECORE_CON_EVENT_CLIENT_DEL and ECORE_CON_EVENT_CLIENT_DATA, that will be called when a client is communicating with the server:
if (!(svr = ecore_con_server_add(ECORE_CON_REMOTE_TCP, "127.0.0.1", 8080, NULL))) exit(1); ecore_event_handler_add(ECORE_CON_EVENT_CLIENT_ADD, _add_cb, NULL); ecore_event_handler_add(ECORE_CON_EVENT_CLIENT_DEL, _del_cb, NULL); ecore_event_handler_add(ECORE_CON_EVENT_CLIENT_DATA, _data_cb, NULL); ecore_main_loop_begin();
The function ecore_con_server_connect() can be used to write a client that connects to a server. The resulting code will be very similar to the server code:
if (!(svr = ecore_con_server_connect(ECORE_CON_REMOTE_TCP, "127.0.0.1", 8080, NULL))) exit(1); ecore_event_handler_add(ECORE_CON_EVENT_SERVER_ADD, _add_cb, NULL); ecore_event_handler_add(ECORE_CON_EVENT_SERVER_DEL, _del_cb, NULL); ecore_event_handler_add(ECORE_CON_EVENT_SERVER_DATA, _data_cb, NULL); ecore_main_loop_begin();
After these two pieces of code are executed, respectively, in the server and client code, the server will be up and running and the client will try to connect to it. The connection, with its subsequent messages being sent from server to client and client to server, can be represented in the following sequence diagram:
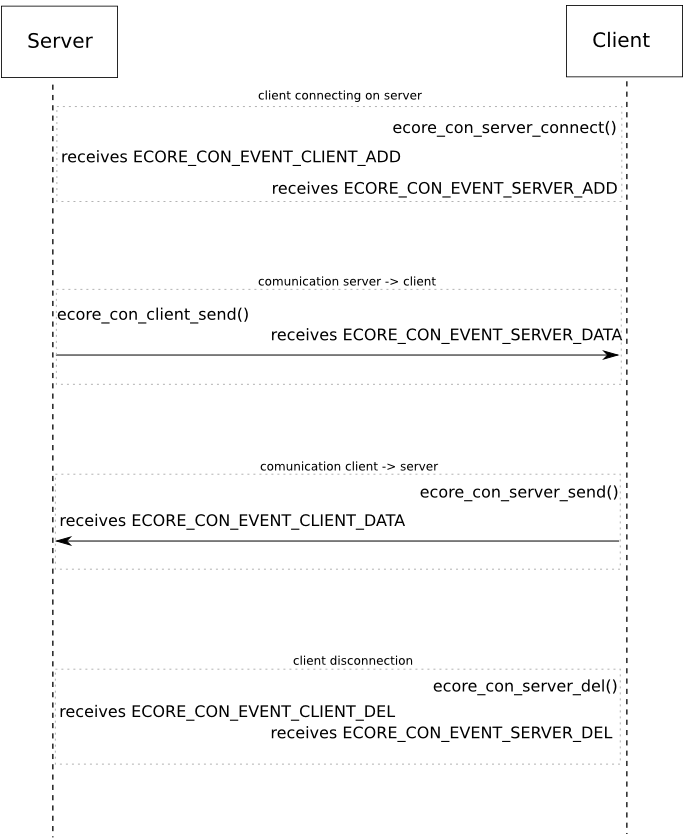
Please notice the important difference between these two codes: the first is used for writing a server, while the second should be used for writing a client.
A reference for the client
functions can be found at Ecore Connection Client Functions.
Examples of usage for this API can be found here:
Functions | |
Ecore_Con_Server * | ecore_con_server_add (Ecore_Con_Type type, const char *name, int port, const void *data) |
Creates a server to listen for connections. | |
Ecore_Con_Server * | ecore_con_server_connect (Ecore_Con_Type type, const char *name, int port, const void *data) |
Creates a connection to the specified server and return an associated object. | |
void * | ecore_con_server_del (Ecore_Con_Server *svr) |
Closes the connection and free the given server. | |
const char * | ecore_con_server_name_get (const Ecore_Con_Server *svr) |
Retrieves the name of server. | |
void * | ecore_con_server_data_get (Ecore_Con_Server *svr) |
Retrieves the data associated with the given server. | |
void * | ecore_con_server_data_set (Ecore_Con_Server *svr, void *data) |
Sets the data associated with the given server. | |
Eina_Bool | ecore_con_server_connected_get (const Ecore_Con_Server *svr) |
Retrieves whether the given server is currently connected. | |
int | ecore_con_server_port_get (const Ecore_Con_Server *svr) |
Retrieves the server port in use. | |
double | ecore_con_server_uptime_get (const Ecore_Con_Server *svr) |
Checks how long a server has been connected. | |
int | ecore_con_server_send (Ecore_Con_Server *svr, const void *data, int size) |
Sends the given data to the given server. | |
void | ecore_con_server_client_limit_set (Ecore_Con_Server *svr, int client_limit, char reject_excess_clients) |
Sets a limit on the number of clients that can be handled concurrently by the given server, and a policy on what to do if excess clients try to connect. | |
const Eina_List * | ecore_con_server_clients_get (const Ecore_Con_Server *svr) |
Retrieves the current list of clients. | |
const char * | ecore_con_server_ip_get (const Ecore_Con_Server *svr) |
Gets the IP address of a server that has been connected to. | |
void | ecore_con_server_flush (Ecore_Con_Server *svr) |
Flushes all pending data to the given server. | |
void | ecore_con_server_timeout_set (Ecore_Con_Server *svr, double timeout) |
Sets the default time after which an inactive client will be disconnected. | |
double | ecore_con_server_timeout_get (const Ecore_Con_Server *svr) |
Gets the default time after which an inactive client will be disconnected. | |
int | ecore_con_server_fd_get (const Ecore_Con_Server *svr) |
Gets the fd that the server is connected to. | |
int | ecore_con_client_fd_get (const Ecore_Con_Client *cl) |
Gets the fd that the client is connected to. | |
Typedefs | |
typedef struct _Ecore_Con_Server | Ecore_Con_Server |
Typedef Documentation
Used to provide legacy API/ABI compatibility with non-Eo applications.
Function Documentation
int ecore_con_client_fd_get | ( | const Ecore_Con_Client * | cl | ) |
Gets the fd that the client is connected to.
- Parameters:
-
cl The client object
- Returns:
- The fd, or
-1
on failure
This function returns the fd which is used by the underlying client connection. It should not be tampered with unless you REALLY know what you are doing.
- Since (EFL) :
- 1.1
- Since :
- 3.0
Ecore_Con_Server* ecore_con_server_add | ( | Ecore_Con_Type | type, |
const char * | name, | ||
int | port, | ||
const void * | data | ||
) |
Creates a server to listen for connections.
- Parameters:
-
type The connection type. name Name to associate with the socket. It is used when generating the socket name of a Unix socket, or for determining what host to listen on for TCP sockets. NULL
will not be accepted.port Number to identify socket. When a Unix socket is used, it becomes part of the socket name. When a TCP socket is used, it is used as the TCP port. data Data to associate with the created Ecore_Con_Server object.
- Returns:
- A new Ecore_Con_Server.
The socket on which the server listens depends on the connection type:
- If type is
ECORE_CON_LOCAL_USER
, the server will listen on the Unix socket. The path to the socket is taken from XDG_RUNTIME_DIR, if that is not set, then from HOME, even if this is not set, then from TMPDIR. If none is set, then path would be /tmp. From this path socket would be created as "[path]/.ecore/[name]/[port]". If port is negative, then "[path]/.ecore/[name]". - If type is
ECORE_CON_LOCAL_SYSTEM
, the server will listen on Unix socket "/tmp/.ecore_service|[name]|[port]". If port is negative, then "/tmp/.ecore_service|[name]". - If type is
ECORE_CON_LOCAL_ABSTRACT
, then port number is not considered while creating the socket. - If type is
ECORE_CON_REMOTE_TCP
, the server will listen on TCP portport
.
More information about the type
can be found at _Ecore_Con_Type.
The data
parameter can be fetched later using ecore_con_server_data_get() or changed with ecore_con_server_data_set().
- See also:
- ecore_con_local_path_new()
- Note:
- This API is deprecated and new code should use #EFL_NET_SERVER_SIMPLE_CLASS. See
- efl_net_server_simple_example::c
- Since :
- 3.0
- Examples:
- ecore_con_server_simple_example.c.
void ecore_con_server_client_limit_set | ( | Ecore_Con_Server * | svr, |
int | client_limit, | ||
char | reject_excess_clients | ||
) |
Sets a limit on the number of clients that can be handled concurrently by the given server, and a policy on what to do if excess clients try to connect.
- Parameters:
-
svr The given server. client_limit The maximum number of clients to handle concurrently. -1 means unlimited (default). 0 effectively disables the server. reject_excess_clients Set to 1 to automatically disconnect excess clients as soon as they connect if you are already handling client_limit clients. Set to 0 (default) to just hold off on the "accept()" system call until the number of active clients drops. This causes the kernel to queue up to 4096 connections (or your kernel's limit, whichever is lower).
Beware that if you set this once ecore is already running, you may already have pending CLIENT_ADD events in your event queue. Those clients have already connected and will not be affected by this call. Only clients subsequently trying to connect will be affected.
- Since :
- 3.0
- Examples:
- ecore_con_server_simple_example.c.
const Eina_List* ecore_con_server_clients_get | ( | const Ecore_Con_Server * | svr | ) |
Retrieves the current list of clients.
Each node in the returned list points to an Efl_Network_Client. This list cannot be modified or freed. It can also change if new clients are connected or disconnected, and will become invalid when the server is deleted/freed.
- Parameters:
-
svr The given server.
- Returns:
- The list of clients on this server.
- Since :
- 3.0
- Examples:
- ecore_con_server_simple_example.c.
Ecore_Con_Server* ecore_con_server_connect | ( | Ecore_Con_Type | type, |
const char * | name, | ||
int | port, | ||
const void * | data | ||
) |
Creates a connection to the specified server and return an associated object.
- Parameters:
-
type The connection type. name Name used when determining what socket to connect to. It is used to generate the socket name when the socket is a Unix socket. It is used as the hostname when connecting with a TCP socket. port Number to identify the socket to connect to. Used when generating the socket name for a Unix socket, or as the TCP port when connecting to a TCP socket. data Data to associate with the created Ecore_Con_Server object.
- Returns:
- A new Ecore_Con_Server.
The socket to which the connection is made depends on the connection type:
- If type is
ECORE_CON_LOCAL_USER
, the server will connect to the Unix socket. The path to the socket is taken from XDG_RUNTIME_DIR, if that is not set, then from HOME, even if this is not set, then from TMPDIR. If none is set, then path would be /tmp. From this path the function would connect to socket at "[path]/.ecore/[name]/[port]". If port is negative, then to socket at "[path]/.ecore/[name]". - If type is
ECORE_CON_LOCAL_SYSTEM
, the server will connect to Unix socket at "/tmp/.ecore_service|[name]|[port]". If port is negative, then to Unix socket at "/tmp/.ecore_service|[name]". - If type is
ECORE_CON_LOCAL_ABSTRACT
, then port number is not considered while connecting to socket. - If type is
ECORE_CON_REMOTE_TCP
, the server will listen on TCP portport
.
More information about the type
can be found at _Ecore_Con_Type.
This function won't block. It will either succeed, or fail due to invalid parameters, failed memory allocation, etc., returning NULL
on that case.
However, even if this call returns a valid Ecore_Con_Server, the connection will only be successfully completed if an event of type ECORE_CON_EVENT_SERVER_ADD is received. If it fails to complete, an ECORE_CON_EVENT_SERVER_DEL will be received.
The created object gets deleted automatically if the connection to the server is lost.
The data
parameter can be fetched later using ecore_con_server_data_get() or changed with ecore_con_server_data_set().
- See also:
- ecore_con_local_path_new()
- Note:
- This API is deprecated and new code should use #EFL_NET_DIALER_SIMPLE_CLASS. See
- efl_net_dialer_simple_example::c
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
Eina_Bool ecore_con_server_connected_get | ( | const Ecore_Con_Server * | svr | ) |
Retrieves whether the given server is currently connected.
- Parameters:
-
svr The given server.
- Returns:
EINA_TRUE
if the server is connected,EINA_FALSE
otherwise.
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
void* ecore_con_server_data_get | ( | Ecore_Con_Server * | svr | ) |
Retrieves the data associated with the given server.
- Parameters:
-
svr The given server.
- Returns:
- The associated data.
- See also:
- ecore_con_server_data_set()
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
void* ecore_con_server_data_set | ( | Ecore_Con_Server * | svr, |
void * | data | ||
) |
Sets the data associated with the given server.
- Parameters:
-
svr The given server. data The data to associate with svr
.
- Returns:
- The previously associated data, if any.
- See also:
- ecore_con_server_data_get()
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
void* ecore_con_server_del | ( | Ecore_Con_Server * | svr | ) |
Closes the connection and free the given server.
- Parameters:
-
svr The given server.
- Returns:
- Data associated with the server when it was created.
All the clients connected to this server will be disconnected.
- See also:
- ecore_con_server_add, ecore_con_server_connect
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
int ecore_con_server_fd_get | ( | const Ecore_Con_Server * | svr | ) |
Gets the fd that the server is connected to.
- Parameters:
-
svr The server object
- Returns:
- The fd, or
-1
on failure
This function returns the fd which is used by the underlying server connection. It should not be tampered with unless you REALLY know what you are doing.
- Note:
- This function is only valid for servers created with ecore_con_server_connect().
- Warning:
- Seriously. Don't use this unless you know what you are doing.
- Since (EFL) :
- 1.1
- Since :
- 3.0
void ecore_con_server_flush | ( | Ecore_Con_Server * | svr | ) |
Flushes all pending data to the given server.
- Parameters:
-
svr The given server.
This function will block until all data is sent to the server.
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
const char* ecore_con_server_ip_get | ( | const Ecore_Con_Server * | svr | ) |
Gets the IP address of a server that has been connected to.
- Parameters:
-
svr The given server.
- Returns:
- A pointer to an internal string that contains the IP address of the connected server in the form "XXX.YYY.ZZZ.AAA" IP notation. This string should not be modified or trusted to stay valid after deletion for the
svr
object. If no IP is knownNULL
is returned.
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
const char* ecore_con_server_name_get | ( | const Ecore_Con_Server * | svr | ) |
Retrieves the name of server.
The name returned is the name used to connect on this server.
- Parameters:
-
svr The given server.
- Returns:
- The name of the server.
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
int ecore_con_server_port_get | ( | const Ecore_Con_Server * | svr | ) |
Retrieves the server port in use.
- Parameters:
-
svr The given server.
- Returns:
- The server port in use.
The port where the server is listening for connections.
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
int ecore_con_server_send | ( | Ecore_Con_Server * | svr, |
const void * | data, | ||
int | size | ||
) |
Sends the given data to the given server.
- Parameters:
-
svr The given server. data The given data. size Length of the data, in bytes, to send.
- Returns:
- The number of bytes sent.
0
will be returned if there is an error.
This function will send the given data to the server as soon as the program is back to the main loop. Thus, this function returns immediately (non-blocking). If the data needs to be sent now, call ecore_con_server_flush() after this one.
- Since :
- 3.0
- Examples:
- ecore_con_client_simple_example.c.
double ecore_con_server_timeout_get | ( | const Ecore_Con_Server * | svr | ) |
Gets the default time after which an inactive client will be disconnected.
- Parameters:
-
svr The server object.
- Returns:
- The timeout, in seconds, to disconnect after.
This function is used to get the idle timeout for clients. A value of < 1 means the idle timeout is disabled.
- Since :
- 3.0
void ecore_con_server_timeout_set | ( | Ecore_Con_Server * | svr, |
double | timeout | ||
) |
Sets the default time after which an inactive client will be disconnected.
- Parameters:
-
svr The server object. timeout The timeout, in seconds, to disconnect after.
This function is used by the server to set the default idle timeout on clients. If the any of the clients becomes idle for a time higher than this value, it will be disconnected. A value of < 1 disables the idle timeout.
This timeout is not affected by the one set by ecore_con_client_timeout_set(). A client will be disconnected whenever the client or the server timeout is reached. That means, the lower timeout value will be used for that client if ecore_con_client_timeout_set() is used on it.
- Since :
- 3.0
- Examples:
- ecore_con_server_simple_example.c.
double ecore_con_server_uptime_get | ( | const Ecore_Con_Server * | svr | ) |
Checks how long a server has been connected.
- Parameters:
-
svr The server to check
- Returns:
- The total time, in seconds, that the server has been connected/running.
This function is used to find out the time that has been elapsed since ecore_con_server_add() succeeded.
- Since :
- 3.0
- Examples:
- ecore_con_server_simple_example.c.