Tizen Native API
7.0
|
Evas allows different transformations to be applied to all kinds of objects. These are applied by means of UV mapping.
With UV mapping, one maps points in the source object to a 3D space positioning at target. This allows rotation, perspective, scale and lots of other effects, depending on the map that is used.
Each map point may carry a multiplier color. If properly calculated, these can do shading effects on the object, producing 3D effects.
As usual, Evas provides both the raw and easy to use methods. The raw methods allow developers to create their maps somewhere else, possibly loading them from some file format. The easy to use methods calculate the points given some high-level parameters such as rotation angle, ambient light, and so on.
- Note:
- applying mapping will reduce performance, so use with care. The impact on performance depends on engine in use. Software is quite optimized, but not as fast as OpenGL.
Map points
Rotation
A map consists of a set of points, currently only four are supported. Each of these points contains a set of canvas coordinates x
and y
that can be used to alter the geometry of the mapped object, and a z
coordinate that indicates the depth of that point. This last coordinate does not normally affect the map, but it's used by several of the utility functions to calculate the right position of the point given other parameters.
The coordinates for each point are set with evas_map_point_coord_set(). The following image shows a map set to match the geometry of an existing object.
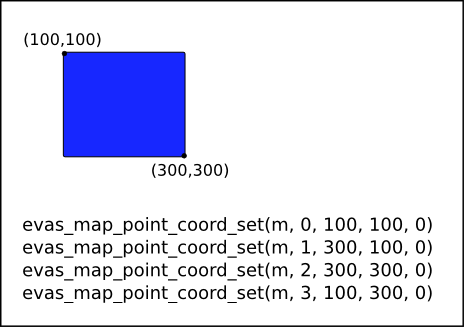
This is a common practice, so there are a few functions that help make it easier.
evas_map_util_points_populate_from_geometry() sets the coordinates of each point in the given map to match the rectangle defined by the function parameters.
evas_map_util_points_populate_from_object() and evas_map_util_points_populate_from_object_full() both take an object and set the map points to match its geometry. The difference between the two is that the first function sets the z
value of all points to 0, while the latter receives the value to set in said coordinate as a parameter.
The following lines of code all produce the same result as in the image above.
evas_map_util_points_populate_from_geometry(m, 100, 100, 200, 200, 0); // Assuming o is our original object evas_object_move(o, 100, 100); evas_object_resize(o, 200, 200); evas_map_util_points_populate_from_object(m, o); evas_map_util_points_populate_from_object_full(m, o, 0);
Several effects can be applied to an object by simply setting each point of the map to the right coordinates. For example, a simulated perspective could be achieve as follows.
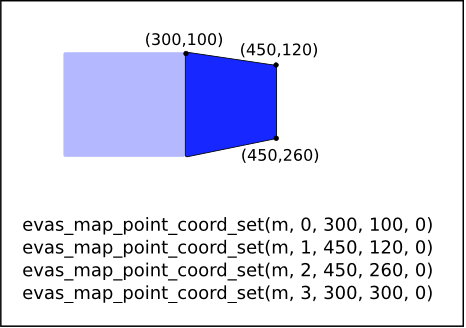
As said before, the z
coordinate is unused here so when setting points by hand, its value is of no importance.
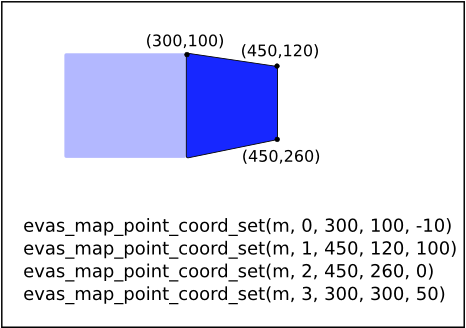
In all three cases above, setting the map to be used by the object is the same.
evas_object_map_set(o, m); evas_object_map_enable_set(o, EINA_TRUE);
Doing things this way, however, is a lot of work that can be avoided by using the provided utility functions, as described in the next section.
Utility functions
Utility functions take an already set up map and alter it to produce a specific effect. For example, to rotate an object around its own center you would need to take the rotation angle, the coordinates of each corner of the object and do all the math to get the new set of coordinates that need to be set in the map.
Or you can use this code:
evas_object_geometry_get(o, &x, &y, &w, &h); m = evas_map_new(4); evas_map_util_points_populate_from_object(m, o); evas_map_util_rotate(m, 45, x + (w / 2), y + (h / 2)); evas_object_map_set(o, m); evas_object_map_enable_set(o, EINA_TRUE); evas_map_free(m);
Which will rotate the object around its center point in a 45 degree angle in the clockwise direction, taking it from this
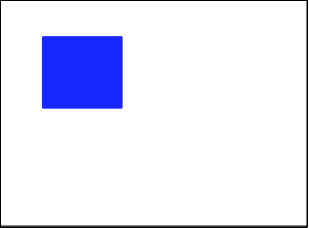
to this
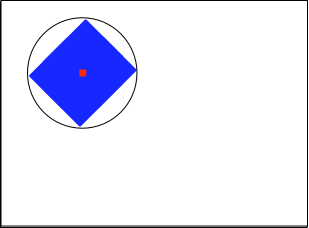
Objects may be rotated around any other point just by setting the last two paramaters of the evas_map_util_rotate() function to the right values. A circle of roughly the diameter of the object overlaid on each image shows where the center of rotation is set for each example.
For example, this code
evas_object_geometry_get(o, &x, &y, &w, &h); m = evas_map_new(4); evas_map_util_points_populate_from_object(m, o); evas_map_util_rotate(m, 45, x + w - 20, y + h - 20); evas_object_map_set(o, m); evas_object_map_enable_set(o, EINA_TRUE); evas_map_free(m);
produces something like
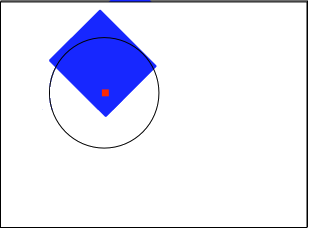
And the following
evas_output_size_get(evas, &w, &h); m = evas_map_new(4); evas_map_util_points_populate_from_object(m, o); evas_map_util_rotate(m, 45, w, h); evas_object_map_set(o, m); evas_object_map_enable_set(o, EINA_TRUE); evas_map_free(m);
rotates the object around the center of the window
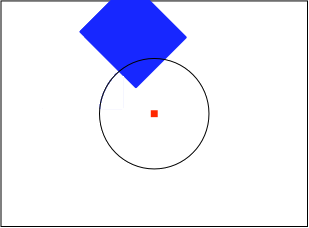
3D Maps
Maps can also be used to achieve the effect of 3-dimensionality. When doing this, the z
coordinate of each point counts, with higher values meaning the point is further into the screen, and smaller values (negative, usually) meaning the point is closer towards the user.
Thinking in 3D also introduces the concept of back-face of an object. An object is said to be facing the user when all its points are placed in a clockwise fashion. The next image shows this, with each point showing the with which is identified within the map.
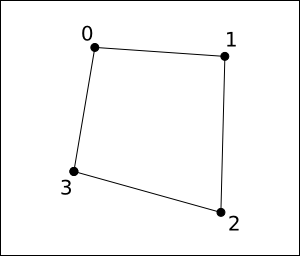
Rotating this map around the Y
axis would leave the order of the points in a counter-clockwise fashion, as seen in the following image.
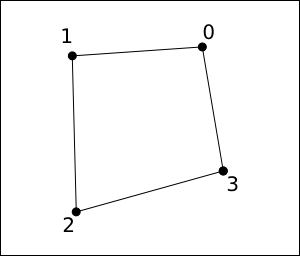
This way we can say that we are looking at the back face of the object. This will have stronger implications later when we talk about lighting.
To know if a map is facing towards the user or not it's enough to use the evas_map_util_clockwise_get() function, but this is normally done after all the other operations are applied on the map.
3D rotation and perspective
Much like evas_map_util_rotate(), there's the function evas_map_util_3d_rotate() that transforms the map to apply a 3D rotation to an object. As in its 2D counterpart, the rotation can be applied around any point in the canvas, this time with a z
coordinate too. The rotation can also be around any of the 3 axis.
Starting from this simple setup
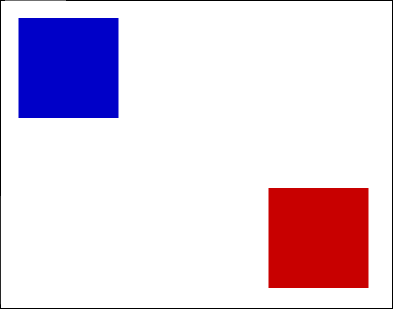
and setting maps so that the blue square to rotate on all axis around a sphere that uses the object as its center, and the red square to rotate around the Y
axis, we get the following. A simple overlay over the image shows the original geometry of each object and the axis around which they are being rotated, with the Z
one not appearing due to being orthogonal to the screen.
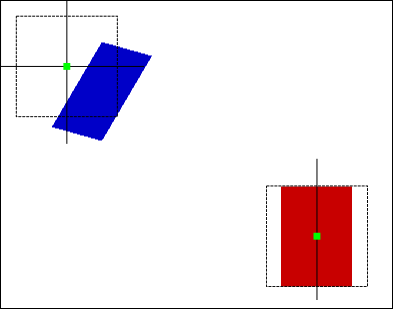
which doesn't look very real. This can be helped by adding perspective to the transformation, which can be simply done by calling evas_map_util_3d_perspective() on the map after its position has been set. The result in this case, making the vanishing point the center of each object:
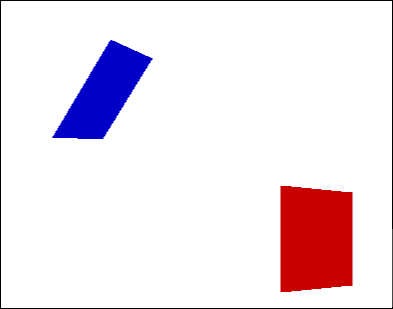
Color and lighting
Each point in a map can be set to a color, which will be multiplied with the objects own color and linearly interpolated in between adjacent points. This is done with evas_map_point_color_set() for each point of the map, or evas_map_util_points_color_set() to set every point to the same color.
When using 3D effects, colors can be used to improve the looks of them by simulating a light source. The evas_map_util_3d_lighting() function makes this task easier by taking the coordinates of the light source and its color, along with the color of the ambient light. Evas then sets the color of each point based on the distance to the light source, the angle with which the object is facing the light and the ambient light. Here, the orientation of each point as explained before, becomes more important. If the map is defined counter-clockwise, the object will be facing away from the user and thus become obscured, since no light would be reflecting from it.
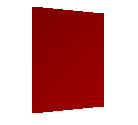
- Note:
- Object facing the light source
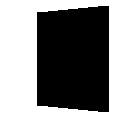
- Note:
- Same object facing away from the user
mapping
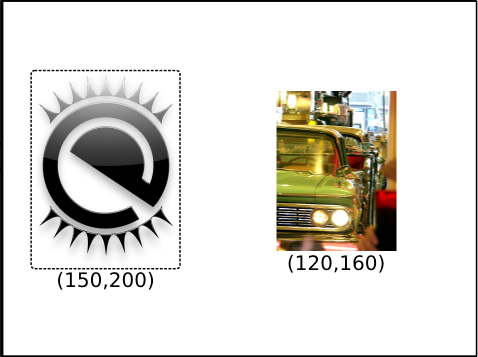
Images need some special handling when mapped. Evas can easily take care of objects and do almost anything with them, but it's completely oblivious to the content of images, so each point in the map needs to be told to what pixel in the source image it belongs. Failing to do may sometimes result in the expected behavior, or it may look like a partial work.
The next image illustrates one possibility of a map being set to an image object, without setting the right UV mapping for each point. The objects themselves are mapped properly to their new geometry, but the image content may not be displayed correctly within the mapped object.
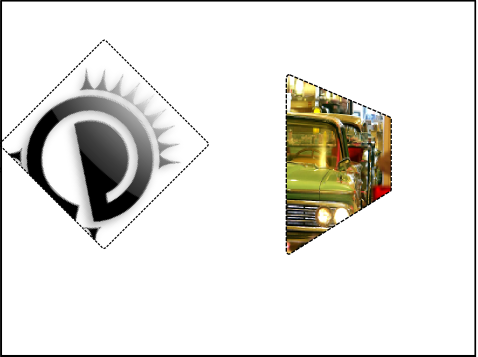
Once Evas knows how to handle the source image within the map, it will transform it as needed. This is done with evas_map_point_image_uv_set(), which tells the map to which pixel in image it maps.
To match our example images to the maps above all we need is the size of each image, which can always be found with evas_object_image_size_get().
evas_map_point_image_uv_set(m, 0, 0, 0); evas_map_point_image_uv_set(m, 1, 150, 0); evas_map_point_image_uv_set(m, 2, 150, 200); evas_map_point_image_uv_set(m, 3, 0, 200); evas_object_map_set(o, m); evas_object_map_enable_set(o, EINA_TRUE); evas_map_point_image_uv_set(m, 0, 0, 0); evas_map_point_image_uv_set(m, 1, 120, 0); evas_map_point_image_uv_set(m, 2, 120, 160); evas_map_point_image_uv_set(m, 3, 0, 160); evas_object_map_set(o2, m); evas_object_map_enable_set(o2, EINA_TRUE);
To get
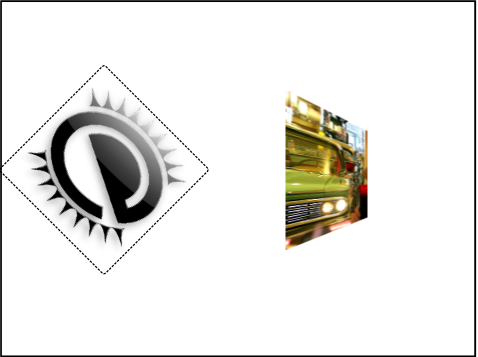
Maps can also be set to use part of an image only, or even map them inverted, and combined with evas_object_image_source_set() it can be used to achieve more interesting results.
evas_object_image_size_get(evas_object_image_source_get(o), &w, &h); evas_map_point_image_uv_set(m, 0, 0, h); evas_map_point_image_uv_set(m, 1, w, h); evas_map_point_image_uv_set(m, 2, w, h / 3); evas_map_point_image_uv_set(m, 3, 0, h / 3); evas_object_map_set(o, m); evas_object_map_enable_set(o, EINA_TRUE);
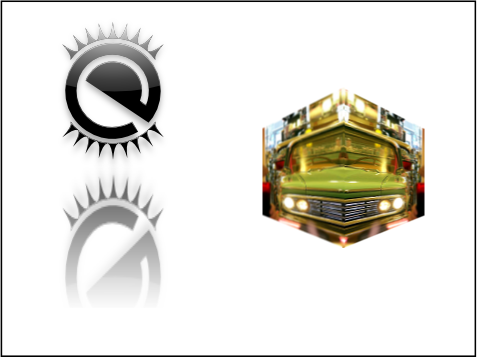
Examples:
Functions | |
void | evas_map_util_points_populate_from_object_full (Evas_Map *m, const Evas_Object *obj, Evas_Coord z) |
void | evas_map_util_points_populate_from_object (Evas_Map *m, const Evas_Object *obj) |
void | evas_map_util_points_populate_from_geometry (Evas_Map *m, Evas_Coord x, Evas_Coord y, Evas_Coord w, Evas_Coord h, Evas_Coord z) |
void | evas_map_util_points_color_set (Evas_Map *m, int r, int g, int b, int a) |
void | evas_map_util_rotate (Evas_Map *m, double degrees, Evas_Coord cx, Evas_Coord cy) |
void | evas_map_util_zoom (Evas_Map *m, double zoomx, double zoomy, Evas_Coord cx, Evas_Coord cy) |
void | evas_map_util_3d_rotate (Evas_Map *m, double dx, double dy, double dz, Evas_Coord cx, Evas_Coord cy, Evas_Coord cz) |
void | evas_map_util_quat_rotate (Evas_Map *m, double qx, double qy, double qz, double qw, double cx, double cy, double cz) |
void | evas_map_util_3d_lighting (Evas_Map *m, Evas_Coord lx, Evas_Coord ly, Evas_Coord lz, int lr, int lg, int lb, int ar, int ag, int ab) |
void | evas_map_util_3d_perspective (Evas_Map *m, Evas_Coord px, Evas_Coord py, Evas_Coord z0, Evas_Coord foc) |
Eina_Bool | evas_map_util_clockwise_get (Evas_Map *m) |
Evas_Map * | evas_map_new (int count) |
void | evas_map_smooth_set (Evas_Map *m, Eina_Bool enabled) |
Eina_Bool | evas_map_smooth_get (const Evas_Map *m) |
void | evas_map_alpha_set (Evas_Map *m, Eina_Bool enabled) |
Eina_Bool | evas_map_alpha_get (const Evas_Map *m) |
void | evas_map_util_object_move_sync_set (Evas_Map *m, Eina_Bool enabled) |
Eina_Bool | evas_map_util_object_move_sync_get (const Evas_Map *m) |
Evas_Map * | evas_map_dup (const Evas_Map *m) |
void | evas_map_free (Evas_Map *m) |
int | evas_map_count_get (const Evas_Map *m) |
Eina_Bool | evas_map_coords_get (const Evas_Map *m, double x, double y, double *mx, double *my, int grab) |
void | evas_map_point_coord_set (Evas_Map *m, int idx, Evas_Coord x, Evas_Coord y, Evas_Coord z) |
void | evas_map_point_coord_get (const Evas_Map *m, int idx, Evas_Coord *x, Evas_Coord *y, Evas_Coord *z) |
void | evas_map_point_precise_coord_set (Evas_Map *m, int idx, double x, double y, double z) |
void | evas_map_point_precise_coord_get (const Evas_Map *m, int idx, double *x, double *y, double *z) |
void | evas_map_point_image_uv_set (Evas_Map *m, int idx, double u, double v) |
void | evas_map_point_image_uv_get (const Evas_Map *m, int idx, double *u, double *v) |
void | evas_map_point_color_set (Evas_Map *m, int idx, int r, int g, int b, int a) |
void | evas_map_point_color_get (const Evas_Map *m, int idx, int *r, int *g, int *b, int *a) |
Function Documentation
Eina_Bool evas_map_alpha_get | ( | const Evas_Map * | m | ) |
Get the alpha flag for map rendering
This gets the alpha flag for map rendering.
- Parameters:
-
m map to get the alpha from. Must not be NULL.
- Returns:
- EINA_FALSE if map is NULL EINA_TRUE otherwise.
- Since :
- 2.3
void evas_map_alpha_set | ( | Evas_Map * | m, |
Eina_Bool | enabled | ||
) |
Set the alpha flag for map rendering
This sets alpha flag for map rendering. If the object is a type that has its own alpha settings, then this will take precedence. Only image objects have this currently. Setting this off stops alpha blending of the map area, and is useful if you know the object and/or all sub-objects is 100% solid.
- Parameters:
-
m map to modify. Must not be NULL. enabled enable or disable alpha map rendering
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
Eina_Bool evas_map_coords_get | ( | const Evas_Map * | m, |
double | x, | ||
double | y, | ||
double * | mx, | ||
double * | my, | ||
int | grab | ||
) |
Apply a map transformation on given coordinate
- Parameters:
-
m map to use to transform x and y x point x source coordinate y point y source coordinate mx point x after transformation by m my point y after transformation by m grab
- Returns:
- EINA_TRUE on success interpolation, EINA_FALSE otherwise
- Since (EFL) :
- 1.20
int evas_map_count_get | ( | const Evas_Map * | m | ) |
Get a maps size.
Returns the number of points in a map. Should be at least 4.
- Parameters:
-
m map to get size.
- Returns:
- -1 on error, points otherwise.
- Since :
- 2.3
Evas_Map* evas_map_dup | ( | const Evas_Map * | m | ) |
Copy a previously allocated map.
This makes a duplicate of the m
object and returns it.
- Parameters:
-
m map to copy. Must not be NULL.
- Returns:
- newly allocated map with the same count and contents as
m
.
- Since :
- 2.3
void evas_map_free | ( | Evas_Map * | m | ) |
Free a previously allocated map.
This frees a given map m
and all memory associated with it. You must NOT free a map returned by evas_object_map_get() as this is internal.
- Parameters:
-
m map to free.
- Since :
- 2.3
- Examples:
- evas-map-utils.c, and evas-smart-object.c.
Evas_Map* evas_map_new | ( | int | count | ) |
Create map of transformation points to be later used with an Evas object.
This creates a set of points (currently only 4 is supported. no other number for count
will work). That is empty and ready to be modified with evas_map calls.
- Parameters:
-
count number of points in the map.
- Returns:
- a newly allocated map or
NULL
on errors.
- See also:
- evas_map_free()
- evas_map_dup()
- evas_map_point_coord_set()
- evas_map_point_image_uv_set()
- evas_map_util_points_populate_from_object_full()
- evas_map_util_points_populate_from_object()
- evas_object_map_set()
- Since :
- 2.3
- Examples:
- evas-map-utils.c, and evas-smart-object.c.
void evas_map_point_color_get | ( | const Evas_Map * | m, |
int | idx, | ||
int * | r, | ||
int * | g, | ||
int * | b, | ||
int * | a | ||
) |
Get the color set on a vertex in the map
This gets the color set by evas_map_point_color_set() on the given vertex of the map.
- Parameters:
-
m map to get the color of the vertex from. idx index of point get. Must be smaller than map size. r pointer to red return g pointer to green return b pointer to blue return a pointer to alpha return
- Since :
- 2.3
void evas_map_point_color_set | ( | Evas_Map * | m, |
int | idx, | ||
int | r, | ||
int | g, | ||
int | b, | ||
int | a | ||
) |
Set the color of a vertex in the map
This sets the color of the vertex in the map. Colors will be linearly interpolated between vertex points through the map. Color will multiply the "texture" pixels (like GL_MODULATE in OpenGL). The default color of a vertex in a map is white solid (255, 255, 255, 255) which means it will have no affect on modifying the texture pixels.
- Parameters:
-
m map to change the color of. idx index of point to change. Must be smaller than map size. r red (0 - 255) g green (0 - 255) b blue (0 - 255) a alpha (0 - 255)
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_point_coord_get | ( | const Evas_Map * | m, |
int | idx, | ||
Evas_Coord * | x, | ||
Evas_Coord * | y, | ||
Evas_Coord * | z | ||
) |
Get the map point's coordinate.
This returns the coordinates of the given point in the map.
- Parameters:
-
m map to query point. idx index of point to query. Must be smaller than map size. x where to return the X coordinate. y where to return the Y coordinate. z where to return the Z coordinate.
- Since :
- 2.3
void evas_map_point_coord_set | ( | Evas_Map * | m, |
int | idx, | ||
Evas_Coord | x, | ||
Evas_Coord | y, | ||
Evas_Coord | z | ||
) |
Change the map point's coordinate.
This sets the fixed point's coordinate in the map. Note that points describe the outline of a quadrangle and are ordered either clockwise or counter-clockwise. It is suggested to keep your quadrangles concave and non-complex, though these polygon modes may work, they may not render a desired set of output. The quadrangle will use points 0 and 1 , 1 and 2, 2 and 3, and 3 and 0 to describe the edges of the quadrangle.
The X and Y and Z coordinates are in canvas units. Z is optional and may or may not be honored in drawing. Z is a hint and does not affect the X and Y rendered coordinates. It may be used for calculating fills with perspective correct rendering.
Remember all coordinates are canvas global ones like with move and resize in evas.
- Parameters:
-
m map to change point. Must not be NULL
.idx index of point to change. Must be smaller than map size. x Point X Coordinate y Point Y Coordinate z Point Z Coordinate hint (pre-perspective transform)
- See also:
- evas_map_util_rotate()
- evas_map_util_zoom()
- evas_map_util_points_populate_from_object_full()
- evas_map_util_points_populate_from_object()
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_point_image_uv_get | ( | const Evas_Map * | m, |
int | idx, | ||
double * | u, | ||
double * | v | ||
) |
Get the map point's U and V texture source points
This returns the texture points set by evas_map_point_image_uv_set().
- Parameters:
-
m map to query point. idx index of point to query. Must be smaller than map size. u where to write the X coordinate within the image/texture source v where to write the Y coordinate within the image/texture source
- Since :
- 2.3
void evas_map_point_image_uv_set | ( | Evas_Map * | m, |
int | idx, | ||
double | u, | ||
double | v | ||
) |
Change the map point's U and V texture source point
This sets the U and V coordinates for the point. This determines which coordinate in the source image is mapped to the given point, much like OpenGL and textures. Notes that these points do select the pixel, but are double floating point values to allow for accuracy and sub-pixel selection.
- Parameters:
-
m map to change the point of. idx index of point to change. Must be smaller than map size. u the X coordinate within the image/texture source v the Y coordinate within the image/texture source
- See also:
- evas_map_point_coord_set()
- evas_object_map_set()
- evas_map_util_points_populate_from_object_full()
- evas_map_util_points_populate_from_object()
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_point_precise_coord_get | ( | const Evas_Map * | m, |
int | idx, | ||
double * | x, | ||
double * | y, | ||
double * | z | ||
) |
- Since :
- 5.5
void evas_map_point_precise_coord_set | ( | Evas_Map * | m, |
int | idx, | ||
double | x, | ||
double | y, | ||
double | z | ||
) |
- Since :
- 5.5
Eina_Bool evas_map_smooth_get | ( | const Evas_Map * | m | ) |
Get the smoothing for map rendering
This gets smoothing for map rendering.
- Parameters:
-
m map to get the smooth from. Must not be NULL.
- Returns:
EINA_TRUE
if the smooth is enabled,EINA_FALSE
otherwise.
- Since :
- 2.3
void evas_map_smooth_set | ( | Evas_Map * | m, |
Eina_Bool | enabled | ||
) |
Set the smoothing for map rendering
This sets smoothing for map rendering. If the object is a type that has its own smoothing settings, then both the smooth settings for this object and the map must be turned off. By default smooth maps are enabled.
- Parameters:
-
m map to modify. Must not be NULL. enabled enable or disable smooth map rendering
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_util_3d_lighting | ( | Evas_Map * | m, |
Evas_Coord | lx, | ||
Evas_Coord | ly, | ||
Evas_Coord | lz, | ||
int | lr, | ||
int | lg, | ||
int | lb, | ||
int | ar, | ||
int | ag, | ||
int | ab | ||
) |
Perform lighting calculations on the given Map
This is used to apply lighting calculations (from a single light source) to a given map. The R, G and B values of each vertex will be modified to reflect the lighting based on the light point coordinates, the light color and the ambient color, and at what angle the map is facing the light source. A surface should have its points be declared in a clockwise fashion if the face is "facing" towards you (as opposed to away from you) as faces have a "logical" side for lighting.
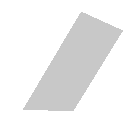
- Note:
- Grey object, no lighting used
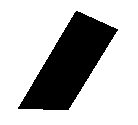
- Note:
- Lights out! Every color set to 0
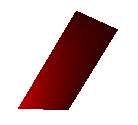
- Note:
- Ambient light to full black, red light coming from close at the bottom-left vertex
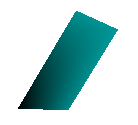
- Note:
- Same light as before, but not the light is set to 0 and ambient light is cyan
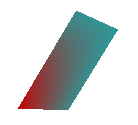
- Note:
- Both lights are on
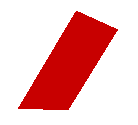
- Note:
- Both lights again, but this time both are the same color.
- Parameters:
-
m map to change. lx X coordinate in space of light point ly Y coordinate in space of light point lz Z coordinate in space of light point lr light red value (0 - 255) lg light green value (0 - 255) lb light blue value (0 - 255) ar ambient color red value (0 - 255) ag ambient color green value (0 - 255) ab ambient color blue value (0 - 255)
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_util_3d_perspective | ( | Evas_Map * | m, |
Evas_Coord | px, | ||
Evas_Coord | py, | ||
Evas_Coord | z0, | ||
Evas_Coord | foc | ||
) |
Apply a perspective transform to the map
This applies a given perspective (3D) to the map coordinates. X, Y and Z values are used. The px and py points specify the "infinite distance" point in the 3D conversion (where all lines converge to like when artists draw 3D by hand). The z0
value specifies the z value at which there is a 1:1 mapping between spatial coordinates and screen coordinates. Any points on this z value will not have their X and Y values modified in the transform. Those further away (Z value higher) will shrink into the distance, and those less than this value will expand and become bigger. The foc
value determines the "focal length" of the camera. This is in reality the distance between the camera lens plane itself (at or closer than this rendering results are undefined) and the "z0" z value. This allows for some "depth" control and foc
must be greater than 0.
- Parameters:
-
m map to change. px The perspective distance X coordinate py The perspective distance Y coordinate z0 The "0" z plane value foc The focal distance
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_util_3d_rotate | ( | Evas_Map * | m, |
double | dx, | ||
double | dy, | ||
double | dz, | ||
Evas_Coord | cx, | ||
Evas_Coord | cy, | ||
Evas_Coord | cz | ||
) |
Rotate the map around 3 axes in 3D
This will rotate not just around the "Z" axis as in evas_map_util_rotate() (which is a convenience call for those only wanting 2D). This will rotate around the X, Y and Z axes. The Z axis points "into" the screen with low values at the screen and higher values further away. The X axis runs from left to right on the screen and the Y axis from top to bottom. Like with evas_map_util_rotate() you provide a center point to rotate around (in 3D).
- Parameters:
-
m map to change. dx amount of degrees from 0.0 to 360.0 to rotate around X axis. dy amount of degrees from 0.0 to 360.0 to rotate around Y axis. dz amount of degrees from 0.0 to 360.0 to rotate around Z axis. cx rotation's center horizontal position. cy rotation's center vertical position. cz rotation's center vertical position.
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
Eina_Bool evas_map_util_clockwise_get | ( | Evas_Map * | m | ) |
Get the clockwise state of a map
This determines if the output points (X and Y. Z is not used) are clockwise or counter-clockwise. This can be used for "back-face culling". This is where you hide objects that "face away" from you. In this case objects that are not clockwise.
- Parameters:
-
m map to query.
- Returns:
- 1 if clockwise, 0 otherwise
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
Eina_Bool evas_map_util_object_move_sync_get | ( | const Evas_Map * | m | ) |
Get the flag of the object move synchronization for map rendering
This gets the flag of the object move synchronization for map rendering.
- Parameters:
-
m map to get the flag of the object move synchronization from. Must not be NULL.
- Returns:
- EINA_FALSE if map is NULL EINA_TRUE otherwise.
- Since (EFL) :
- 1.13
- Since :
- 3.0
void evas_map_util_object_move_sync_set | ( | Evas_Map * | m, |
Eina_Bool | enabled | ||
) |
Set the flag of the object move synchronization for map rendering
This sets the flag of the object move synchronization for map rendering. If the flag is set as enabled, the map will be moved as the object of the map is moved. By default, the flag of the object move synchronization is not enabled.
- Parameters:
-
m map to modify. Must not be NULL. enabled enable or disable the object move synchronization for map rendering.
- Since (EFL) :
- 1.13
- Since :
- 3.0
void evas_map_util_points_color_set | ( | Evas_Map * | m, |
int | r, | ||
int | g, | ||
int | b, | ||
int | a | ||
) |
Set color of all points to given color.
This call is useful to reuse maps after they had 3d lighting or any other colorization applied before.
- Parameters:
-
m map to change the color of. r red (0 - 255) g green (0 - 255) b blue (0 - 255) a alpha (0 - 255)
- See also:
- evas_map_point_color_set()
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_util_points_populate_from_geometry | ( | Evas_Map * | m, |
Evas_Coord | x, | ||
Evas_Coord | y, | ||
Evas_Coord | w, | ||
Evas_Coord | h, | ||
Evas_Coord | z | ||
) |
Populate source and destination map points to match given geometry.
Similar to evas_map_util_points_populate_from_object_full(), this call takes raw values instead of querying object's unmapped geometry. The given width will be used to calculate destination points (evas_map_point_coord_set()) and set the image uv (evas_map_point_image_uv_set()).
- Parameters:
-
m map to change all 4 points (must be of size 4). x Point X Coordinate y Point Y Coordinate w width to use to calculate second and third points. h height to use to calculate third and fourth points. z Point Z Coordinate hint (pre-perspective transform). This value will be used for all four points.
- See also:
- evas_map_util_points_populate_from_object()
- evas_map_point_coord_set()
- evas_map_point_image_uv_set()
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_util_points_populate_from_object | ( | Evas_Map * | m, |
const Evas_Object * | obj | ||
) |
Populate source and destination map points to match exactly object.
Usually one initialize map of an object to match it's original position and size, then transform these with evas_map_util_* functions, such as evas_map_util_rotate() or evas_map_util_3d_rotate(). The original set is done by this function, avoiding code duplication all around.
Z Point coordinate is assumed as 0 (zero).
- Parameters:
-
m map to change all 4 points (must be of size 4). obj object to use unmapped geometry to populate map coordinates.
- See also:
- evas_map_util_points_populate_from_object_full()
- evas_map_util_points_populate_from_geometry()
- evas_map_point_coord_set()
- evas_map_point_image_uv_set()
- Since :
- 2.3
- Examples:
- evas-map-utils.c, and evas-smart-object.c.
void evas_map_util_points_populate_from_object_full | ( | Evas_Map * | m, |
const Evas_Object * | obj, | ||
Evas_Coord | z | ||
) |
Populate source and destination map points to match exactly object.
Usually one initialize map of an object to match it's original position and size, then transform these with evas_map_util_* functions, such as evas_map_util_rotate() or evas_map_util_3d_rotate(). The original set is done by this function, avoiding code duplication all around.
- Parameters:
-
m map to change all 4 points (must be of size 4). obj object to use unmapped geometry to populate map coordinates. z Point Z Coordinate hint (pre-perspective transform). This value will be used for all four points.
- See also:
- evas_map_util_points_populate_from_object()
- evas_map_point_coord_set()
- evas_map_point_image_uv_set()
- Since :
- 2.3
- Examples:
- evas-map-utils.c.
void evas_map_util_quat_rotate | ( | Evas_Map * | m, |
double | qx, | ||
double | qy, | ||
double | qz, | ||
double | qw, | ||
double | cx, | ||
double | cy, | ||
double | cz | ||
) |
Rotate the map in 3D using a unit quaternion.
This will rotate in 3D using a unit quaternion. Like with evas_map_util_3d_rotate() you provide a center point to rotate around (in 3D).
- Parameters:
-
m map to change. qx the x component of the imaginary part of the quaternion. qy the y component of the imaginary part of the quaternion. qz the z component of the imaginary part of the quaternion. qw the w component of the real part of the quaternion. cx rotation's center x. cy rotation's center y. cz rotation's center z.
- Warning:
- Rotations can be done using a unit quaternion. Thus, this function expects a unit quaternion (i.e. qx² + qy² + qz² + qw² == 1). If this is not the case the behavior is undefined.
- Since (EFL) :
- 1.8
- Since :
- 2.3
void evas_map_util_rotate | ( | Evas_Map * | m, |
double | degrees, | ||
Evas_Coord | cx, | ||
Evas_Coord | cy | ||
) |
Change the map to apply the given rotation.
This rotates the indicated map's coordinates around the center coordinate given by cx
and cy
as the rotation center. The points will have their X and Y coordinates rotated clockwise by degrees
degrees (360.0 is a full rotation). Negative values for degrees will rotate counter-clockwise by that amount. All coordinates are canvas global coordinates.
- Parameters:
-
m map to change. degrees amount of degrees from 0.0 to 360.0 to rotate. cx rotation's center horizontal position. cy rotation's center vertical position.
- Since :
- 2.3
- Examples:
- evas-map-utils.c, and evas-smart-object.c.
void evas_map_util_zoom | ( | Evas_Map * | m, |
double | zoomx, | ||
double | zoomy, | ||
Evas_Coord | cx, | ||
Evas_Coord | cy | ||
) |
Change the map to apply the given zooming.
Like evas_map_util_rotate(), this zooms the points of the map from a center point. That center is defined by cx
and cy
. The zoomx
and zoomy
parameters specify how much to zoom in the X and Y direction respectively. A value of 1.0 means "don't zoom". 2.0 means "double the size". 0.5 is "half the size" etc. All coordinates are canvas global coordinates.
- Parameters:
-
m map to change. zoomx horizontal zoom to use. zoomy vertical zoom to use. cx zooming center horizontal position. cy zooming center vertical position.
- Since :
- 2.3