Tizen Native API
7.0
|
Widget application API.
Required Header
#include <widget_app.h> #include <widget_app_efl.h>
Overview
The Widget Application API provides functions for handling Tizen widget application state changes or system events. Tizen widget application can be shown in the home screen. This API supports making multiple widget instances per an application. This API provides interfaces for the following categories:
- Starting or exiting the main event loop.
- Registering callbacks for application state change events.
- Registering callbacks for basic system events.
- Registering callbacks for instance state change events.
Registering Callbacks for Application State Change Events
As for Tizen widget application states, it is very simple and somewhat similar to Tizen service application states.
Callback | Description |
---|---|
widget_app_create_cb() | Hook to take necessary actions before the main event loop starts. Your UI generation code should be placed here so that you do not miss any events from your application UI. Please make sure that you make a class handle and return it. It will be used when the event for creating widget instance is received. You can initialize shared resources for widget instances in this callback function as well. |
widget_app_terminate_cb() | Hook to take necessary actions when your application is terminating. Your application should release all resources, especially any allocations and shared resources must be freed here so that other running applications can fully use these shared resources. |
Please refer to the following state diagram to see the possible transitions and callbacks that are called while transition.
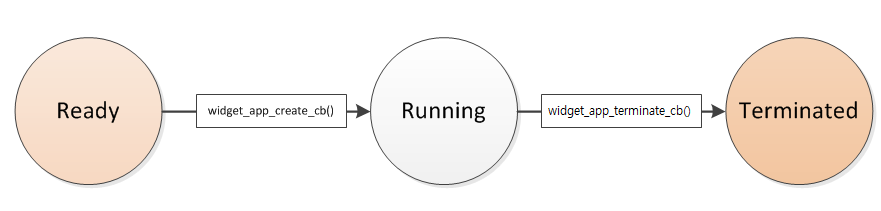
Registering Callbacks for System Events
Tizen widget applications can receive system events with widget_app_add_event_handler() API. The type of system events that can be received are same as Tizen UI applications except for APP_EVENT_DEVICE_ORIENTATION_CHANGED. See Application. The event for APP_EVENT_DEVICE_ORIENTATION_CHANGED is not supported in this module.
Registering callbacks for instance state change events
As for Tizen widget instance states, it is somewhat similar to Tizen application states.
Callback | Description |
---|---|
widget_instance_create_cb() | Called after widget instance is created. In this callback, you can initialize resources for this instance. If parameter 'content' is not NULL, you should restore the previous status. |
widget_instance_destroy_cb() | Called before widget instance is destroyed. In this callback, you can finalize resources for this instance. |
widget_instance_pause_cb() | Called when the widget is invisible. The paused instance may be destroyed by framework |
widget_instance_resume_cb() | Called when the widget is visible. The callback function is called when the widget is visible. |
widget_instance_resize_cb() | Called before the widget size is changed. The callback function is called before the widget size is changed. |
widget_instance_update_cb() | Called when the event for updating widget is received. The callback function is called when the event for updating widget is received. |
Please refer to the following state diagram to see the possible transitions and callbacks that are called while transition.
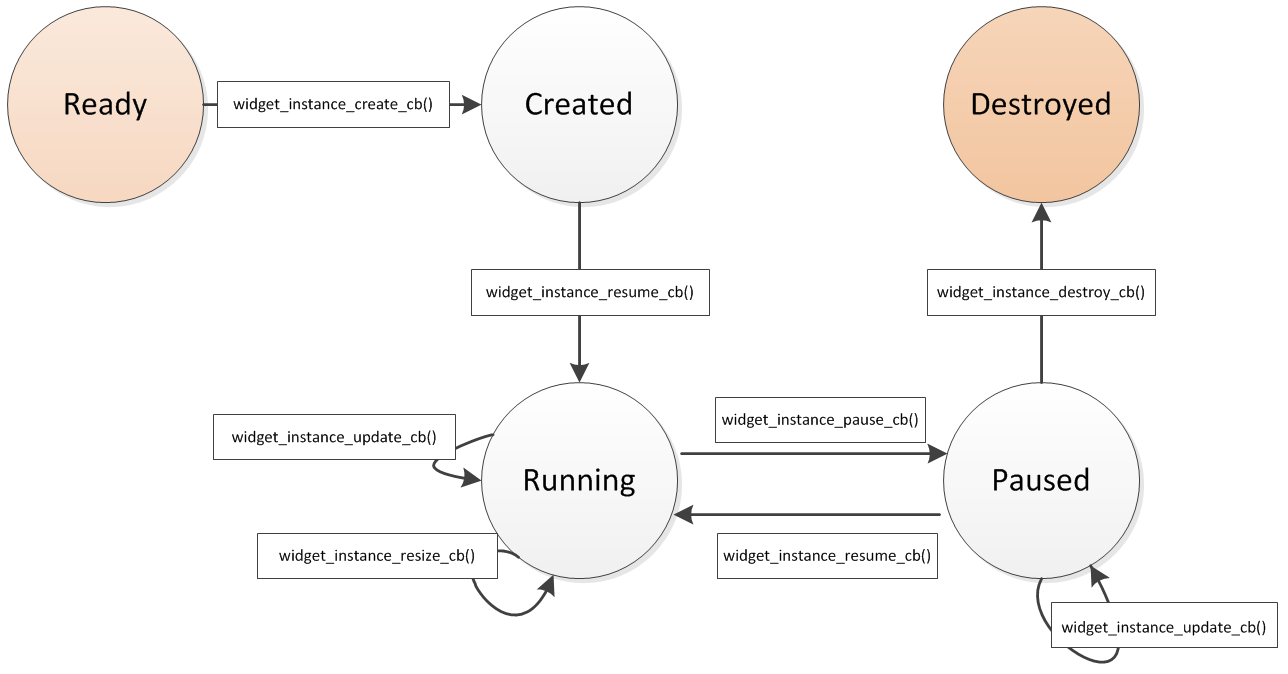
Related Features
This API is related with the following feature:
Functions | |
int | widget_app_main (int argc, char **argv, widget_app_lifecycle_callback_s *callback, void *user_data) |
Runs the main loop of the application until widget_app_exit() is called. | |
int | widget_app_exit (void) |
Exits the main loop of the application. | |
int | widget_app_terminate_context (widget_context_h context) |
Finishes context for the widget instance. | |
int | widget_app_foreach_context (widget_context_cb callback, void *data) |
Retrieves all widget contexts in this application. | |
int | widget_app_add_event_handler (app_event_handler_h *event_handler, app_event_type_e event_type, app_event_cb callback, void *user_data) |
Adds the system event handler. | |
int | widget_app_remove_event_handler (app_event_handler_h event_handler) |
Removes registered event handler. | |
const char * | widget_app_get_id (widget_context_h context) |
Gets a widget instance id. | |
widget_class_h | widget_app_class_create (widget_instance_lifecycle_callback_s callback, void *user_data) |
Makes a class for widget instances. | |
int | widget_app_context_set_tag (widget_context_h context, void *tag) |
Sets a tag in the context. | |
int | widget_app_context_get_tag (widget_context_h context, void **tag) |
Gets the tag in the context. | |
int | widget_app_context_set_content_info (widget_context_h context, bundle *content_info) |
Sets the content info to the widget. | |
int | widget_app_context_set_title (widget_context_h context, const char *title) |
Sends the title to the widget. | |
widget_class_h | widget_app_class_add (widget_class_h widget_class, const char *class_id, widget_instance_lifecycle_callback_s callback, void *user_data) |
Adds an additional widget class for multi-class of widget instantiation. | |
int | widget_app_get_elm_win (widget_context_h context, Evas_Object **win) |
Gets an Evas object for the widget. | |
Typedefs | |
typedef enum widget_app_destroy_type | widget_app_destroy_type_e |
Enumeration for destroy type of widget instance. | |
typedef struct _widget_class * | widget_class_h |
The widget class handle. | |
typedef struct _widget_context * | widget_context_h |
The widget context handle. | |
typedef int(* | widget_instance_create_cb )(widget_context_h context, bundle *content, int w, int h, void *user_data) |
Called when the widget instance starts. | |
typedef int(* | widget_instance_destroy_cb )(widget_context_h context, widget_app_destroy_type_e reason, bundle *content, void *user_data) |
Called before the widget instance is destroyed. | |
typedef int(* | widget_instance_pause_cb )(widget_context_h context, void *user_data) |
Called when the widget is invisible. | |
typedef int(* | widget_instance_resume_cb )(widget_context_h context, void *user_data) |
Called when the widget is visible. | |
typedef int(* | widget_instance_resize_cb )(widget_context_h context, int w, int h, void *user_data) |
Called before the widget size is changed. | |
typedef int(* | widget_instance_update_cb )(widget_context_h context, bundle *content, int force, void *user_data) |
Called when the event for updating widget is received. | |
typedef widget_class_h(* | widget_app_create_cb )(void *user_data) |
Called when the application starts. | |
typedef void(* | widget_app_terminate_cb )(void *user_data) |
Called when the application's main loop exits. | |
typedef bool(* | widget_context_cb )(widget_context_h context, void *user_data) |
Called for each widget context. |
Typedef Documentation
typedef widget_class_h(* widget_app_create_cb)(void *user_data) |
Called when the application starts.
The callback function is called before the main loop of the application starts. In this callback, you can initialize resources which can be shared among widget instances. This function should return the handle for widget class so that it will be used for making instances of widget.
- Since :
- 2.3.1
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Returns:
- The object of widget class
- See also:
- widget_app_main()
static widget_class_h __widget_app_created(void *user_data) { widget_instance_lifecycle_callback_s callback = { .... }; return widget_app_class_create(callback); }
typedef enum widget_app_destroy_type widget_app_destroy_type_e |
Enumeration for destroy type of widget instance.
- Since :
- 2.3.1
typedef void(* widget_app_terminate_cb)(void *user_data) |
Called when the application's main loop exits.
This callback function is called once after the main loop of the application exits. You should release the application's resources in this function.
- Since :
- 2.3.1
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- See also:
- widget_app_main()
typedef struct _widget_class* widget_class_h |
The widget class handle.
- Since :
- 2.3.1
typedef bool(* widget_context_cb)(widget_context_h context, void *user_data) |
Called for each widget context.
This function will be called in the function of widget_app_foreach_context() repeatedly.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance [in] user_data The data for caller
- Returns:
true
to continue with the next iteration of the loop, otherwisefalse
to break out of the loop
- See also:
- widget_app_foreach_context()
typedef struct _widget_context* widget_context_h |
The widget context handle.
- Since :
- 2.3.1
typedef int(* widget_instance_create_cb)(widget_context_h context, bundle *content, int w, int h, void *user_data) |
Called when the widget instance starts.
The callback function is called after widget instance is created. In this callback, you can initialize resources for this instance.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context of widget instance [in] content The data set for the previous status [in] w The pixel value for widget width [in] h The pixel value for widget height [in] user_data The user data passed from widget_app_class_create() function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
typedef int(* widget_instance_destroy_cb)(widget_context_h context, widget_app_destroy_type_e reason, bundle *content, void *user_data) |
Called before the widget instance is destroyed.
The callback function is called before widget instance is destroyed. In this callback, you can finalize resources for this instance. If reason is not WIDGET_APP_DESTROY_TYPE_TEMPORARY, it should store the current status by using incoming bundle.
- Since :
- 2.3.1
- Remarks:
- Note that the parameter 'content' is used to save the status of the widget instance. As a input parameter, content contains the saved status of the widget instance. You can fill the content parameter with the current status in this callback, then the framework will save the content by receiving it as a output parameter. Consequently, you should not use widget_app_context_set_content_info() function in this callback. The content will be overwritten after this callback returns with the 'content' parameter.
- Parameters:
-
[in] context The context of widget instance [in] reason The reason for destruction [in,out] content The data set to save [in] user_data The user data passed from widget_app_class_create() function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
typedef int(* widget_instance_pause_cb)(widget_context_h context, void *user_data) |
Called when the widget is invisible.
The callback function is called when the widget is invisible. The paused instance may be destroyed by framework.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context of widget instance [in] user_data The user data passed from widget_app_class_create() function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
typedef int(* widget_instance_resize_cb)(widget_context_h context, int w, int h, void *user_data) |
Called before the widget size is changed.
The callback function is called before the widget size is changed.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context of widget instance [in] w The pixel value for widget width [in] h The pixel value for widget height [in] user_data The user data passed from widget_app_class_create() function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
typedef int(* widget_instance_resume_cb)(widget_context_h context, void *user_data) |
Called when the widget is visible.
The callback function is called when the widget is visible.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context of widget instance [in] user_data The user data passed from widget_app_class_create() function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
typedef int(* widget_instance_update_cb)(widget_context_h context, bundle *content, int force, void *user_data) |
Called when the event for updating widget is received.
The callback function is called when the event for updating widget is received.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context of widget instance [in] content The data set for updating this widget. It will be provided by requester. Requester can use widget_service_trigger_update() [in] force Although the widget is paused, if it is TRUE, the widget can be updated [in] user_data The user data passed from widget_app_class_create() function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- See also:
- widget_service_trigger_update()
Enumeration Type Documentation
Function Documentation
int widget_app_add_event_handler | ( | app_event_handler_h * | event_handler, |
app_event_type_e | event_type, | ||
app_event_cb | callback, | ||
void * | user_data | ||
) |
Adds the system event handler.
- Since :
- 2.3.1
- Parameters:
-
[out] event_handler The event handler [in] event_type The system event type. APP_EVENT_DEVICE_ORIENTATION_CHANGED is not supported [in] callback The callback function [in] user_data The user data to be passed to the callback function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_OUT_OF_MEMORY Out of memory WIDGET_ERROR_FAULT Unrecoverable error
widget_class_h widget_app_class_add | ( | widget_class_h | widget_class, |
const char * | class_id, | ||
widget_instance_lifecycle_callback_s | callback, | ||
void * | user_data | ||
) |
Adds an additional widget class for multi-class of widget instantiation.
- Since :
- 3.0
- Remarks:
- The specific error code can be obtained using the get_last_result() method. Error codes are described in Exception section.
- Parameters:
-
[in] widget_class The result of widget_app_class_create() [in] class_id The class id of provider [in] callback The set of lifecycle callbacks [in] user_data The user data to be passed to the callback functions
- Returns:
- The new widget class object, NULL on error
- Exceptions:
-
WIDGET_ERROR_NONE Successfully added WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_OUT_OF_MEMORY Out of memory
- See also:
- get_last_result()
widget_class_h widget_app_class_create | ( | widget_instance_lifecycle_callback_s | callback, |
void * | user_data | ||
) |
Makes a class for widget instances.
- Since :
- 2.3.1
- Remarks:
- The specific error code can be obtained using the get_last_result() method. Error codes are described in Exception section.
- Parameters:
-
[in] callback The set of lifecycle callbacks [in] user_data The user data to be passed to the callback functions
- Returns:
- The new widget class object, NULL on error
- Exceptions:
-
WIDGET_ERROR_NONE Successfully added WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_OUT_OF_MEMORY Out of memory
- See also:
- get_last_result()
int widget_app_context_get_tag | ( | widget_context_h | context, |
void ** | tag | ||
) |
Gets the tag in the context.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance [out] tag The value to get
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Unrecoverable error
int widget_app_context_set_content_info | ( | widget_context_h | context, |
bundle * | content_info | ||
) |
Sets the content info to the widget.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance [in] content_info The data set to save
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successfully sent WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid argument WIDGET_ERROR_OUT_OF_MEMORY Out of memory WIDGET_ERROR_FAULT Unrecoverable error
int widget_app_context_set_tag | ( | widget_context_h | context, |
void * | tag | ||
) |
Sets a tag in the context.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance [in] tag The value to save
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Unrecoverable error
int widget_app_context_set_title | ( | widget_context_h | context, |
const char * | title | ||
) |
Sends the title to the widget.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance [in] title When an accessibility mode is turned on, this string will be read
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successfully sent WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid argument WIDGET_ERROR_OUT_OF_MEMORY Out of memory WIDGET_ERROR_FAULT Unrecoverable error
int widget_app_exit | ( | void | ) |
Exits the main loop of the application.
The main loop of the application stops and widget_app_terminate_cb() is invoked.
- Since :
- 2.3.1
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_FAULT Unrecoverable error
int widget_app_foreach_context | ( | widget_context_cb | callback, |
void * | data | ||
) |
Retrieves all widget contexts in this application.
- Since :
- 2.3.1
- Parameters:
-
[in] callback The iteration callback function [in] data The data for the callback function
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_CANCELED The iteration is canceled WIDGET_ERROR_FAULT Unrecoverable error
- See also:
- widget_context_cb()
int widget_app_get_elm_win | ( | widget_context_h | context, |
Evas_Object ** | win | ||
) |
Gets an Evas object for the widget.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance [out] win evas object for window
- Returns:
- 0 on success, otherwise a negative error value
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Failed to make evas object
const char* widget_app_get_id | ( | widget_context_h | context | ) |
Gets a widget instance id.
- Since :
- 2.3.1
- Remarks:
- The specific error code can be obtained using the get_last_result() method. Error codes are described in Exception section.
- You must not free returned widget instance id
-
The returned widget instance id is volatile. If the device reboots or the widget's process restarts, it will be changed.
So, you should not assume this value is a persistent one. -
widget_service_trigger_update(), widget_service_change_period(), widget_service_get_content_of_widget_instance()
can be called with returned instance id.
- Parameters:
-
[in] context The context for widget instance
- Returns:
- widget instance id on success, otherwise NULL
- Exceptions:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Unrecoverable error
int widget_app_main | ( | int | argc, |
char ** | argv, | ||
widget_app_lifecycle_callback_s * | callback, | ||
void * | user_data | ||
) |
Runs the main loop of the application until widget_app_exit() is called.
- Since :
- 2.3.1
- Parameters:
-
[in] argc The argument count [in] argv The argument vector [in] callback The set of callback functions to handle application events [in] user_data The user data to be passed to the callback functions
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Unrecoverable error
- See also:
- widget_app_exit()
int widget_app_remove_event_handler | ( | app_event_handler_h | event_handler | ) |
Removes registered event handler.
- Since :
- 2.3.1
- Parameters:
-
[in] event_handler The event handler
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Unrecoverable error
- See also:
- widget_app_add_event_handler()
int widget_app_terminate_context | ( | widget_context_h | context | ) |
Finishes context for the widget instance.
- Since :
- 2.3.1
- Parameters:
-
[in] context The context for widget instance
- Returns:
- WIDGET_ERROR_NONE on success, otherwise an error code (see WIDGET_ERROR_XXX) on failure
- Return values:
-
WIDGET_ERROR_NONE Successful WIDGET_ERROR_NOT_SUPPORTED Not supported WIDGET_ERROR_INVALID_PARAMETER Invalid parameter WIDGET_ERROR_FAULT Unrecoverable error