Tizen Native API
7.0
|
In this example we add three buttons to a relative_container.
An interesting thing is children have chain-relationship like group behavior horizontally.
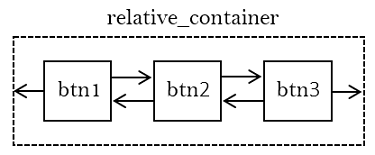
In chain-relationship, relative_position
parameter in function elm_relative_container_relation_xxx_set
is ignored. the edge is positioned at the end of target edge. 0.5
and 0.3
won't affect the position of the edge.
elm_relative_container_relation_left_set(layout, bt2, bt1, 0.5); elm_relative_container_relation_right_set(layout, bt2, bt3, 0.3);
Another interesting point is the align of chain group is affected only by the align of leftmost/topmost child. hint_align
of bt1
will work:
evas_object_size_hint_align_set(bt1, 1.0, 0.5);
evas_object_size_hint_align_set(bt2, 0.0, 0.5); evas_object_size_hint_align_set(bt3, 0.5, 0.5);
Here you can see the full source:
// gcc -o relative_container_example_02 relative_container_example_02.c `pkg-config --cflags --libs elementary` #include <Elementary.h> EAPI_MAIN int elm_main(int argc, char **argv) { Evas_Object *win, *layout, *bt1, *bt2, *bt3; win = elm_win_util_standard_add("elm-relative-container", "Elm.RelativeContainer"); elm_win_autodel_set(win, EINA_TRUE); elm_policy_set(ELM_POLICY_QUIT, ELM_POLICY_QUIT_LAST_WINDOW_CLOSED); evas_object_resize(win, 500, 500); evas_object_show(win); layout = elm_relative_container_add(win); evas_object_size_hint_weight_set(layout, EVAS_HINT_EXPAND, EVAS_HINT_EXPAND); evas_object_show(layout); elm_win_resize_object_add(win, layout); bt1 = elm_button_add(layout); bt2 = elm_button_add(layout); bt3 = elm_button_add(layout); elm_object_text_set(bt1, "bt1"); evas_object_size_hint_min_set(bt1, 100, 100); evas_object_size_hint_align_set(bt1, 1.0, 0.5); evas_object_show(bt1); elm_relative_container_relation_right_set(layout, bt1, bt2, 0.0); elm_object_text_set(bt2, "bt2"); evas_object_size_hint_min_set(bt2, 100, 100); evas_object_size_hint_align_set(bt2, 0.0, 0.5); evas_object_show(bt2); elm_relative_container_relation_left_set(layout, bt2, bt1, 0.5); elm_relative_container_relation_right_set(layout, bt2, bt3, 0.3); elm_object_text_set(bt3, "bt3"); evas_object_size_hint_min_set(bt3, 100, 100); evas_object_size_hint_align_set(bt3, 0.5, 0.5); evas_object_show(bt3); elm_relative_container_relation_left_set(layout, bt3, bt2, 1.0); elm_run(); return 0; } ELM_MAIN()