Tizen Native API
|
A box arranges objects in a linear fashion, governed by a layout function that defines the details of this arrangement.
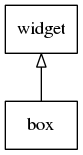
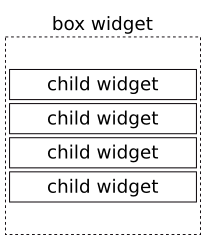
By default, the box uses an internal function to set the layout to a single row, either vertical or horizontal. This layout is affected by a number of parameters, such as the homogeneous flag set by elm_box_homogeneous_set(), the values given by elm_box_padding_set() and elm_box_align_set(), and the hints set to each object in the box.
For this default layout, it's possible to change the orientation with elm_box_horizontal_set(). The box starts in the vertical orientation, placing its elements ordered from top to bottom. When horizontal is set, the order goes from left to right. If the box is set to be homogeneous, every object in it is assigned the same space, which is that of the largest object. Padding can be used to set some spacing between the cell given to each object. The alignment of the box, set with elm_box_align_set(), determines how the bounding box of all the elements is placed within the space given to the box widget itself.
The size hints of each object also affect how they are placed and sized within the box. evas_object_size_hint_min_set() gives the minimum size that the object can have, and the box uses it as the basis for all latter calculations. Elementary widgets set their own minimum size as needed, so there's rarely any need to use it manually.
evas_object_size_hint_weight_set(), when not in the homogeneous mode, is used to tell whether the object is allocated the minimum size it needs or if the space given to it should be expanded. It's important to realize that expanding the size given to the object is not the same thing as resizing the object. It could very well end being a small widget floating in a much larger empty space. If not set, the weight for objects normally is 0.0
for both axis, meaning the widget is not expanded. To take as much space as possible, set the weight to EVAS_HINT_EXPAND (defined to 1.0
) for the desired axis to expand.
Besides how much space is allocated for each object, it's possible to control how the widget is placed within that space using evas_object_size_hint_align_set(). By default, this value is 0.5
for both axes, meaning the object is centered, but any value from 0.0
(left or top, for the x
and y
axis, respectively) to 1.0
(right or bottom) can be used. The special value EVAS_HINT_FILL, which is -1.0
, means the object is resized to fill the entire space it is allocated.
In addition, customized functions to define the layout can be set, which allow the application developer to organize the objects within the box in a number of ways.
The special elm_box_layout_transition() function can be used to switch from one layout to another, animating the motion of the children of the box.
- Remarks:
- Objects should not be added to box objects using _add() calls.
Functions | |
Evas_Object * | elm_box_add (Evas_Object *parent) |
Adds a new box to the parent. | |
void | elm_box_horizontal_set (Evas_Object *obj, Eina_Bool horizontal) |
Sets the horizontal orientation. | |
Eina_Bool | elm_box_horizontal_get (const Evas_Object *obj) |
Gets the horizontal orientation. | |
void | elm_box_homogeneous_set (Evas_Object *obj, Eina_Bool homogeneous) |
Sets the box to arrange its children homogeneously. | |
Eina_Bool | elm_box_homogeneous_get (const Evas_Object *obj) |
Gets whether the box is using the homogeneous mode. | |
void | elm_box_pack_start (Evas_Object *obj, Evas_Object *subobj) |
Adds an object to the beginning of the pack list. | |
void | elm_box_pack_end (Evas_Object *obj, Evas_Object *subobj) |
Adds an object at the end of the pack list. | |
void | elm_box_pack_before (Evas_Object *obj, Evas_Object *subobj, Evas_Object *before) |
Adds an object to the box before the indicated object. | |
void | elm_box_pack_after (Evas_Object *obj, Evas_Object *subobj, Evas_Object *after) |
Adds an object to the box after the indicated object. | |
void | elm_box_clear (Evas_Object *obj) |
Clears the box of all its children. | |
void | elm_box_unpack (Evas_Object *obj, Evas_Object *subobj) |
Unpacks a box item. | |
void | elm_box_unpack_all (Evas_Object *obj) |
Removes all the items from the box, without deleting them. | |
Eina_List * | elm_box_children_get (const Evas_Object *obj) |
Retrieves a list of the objects packed into the box. | |
void | elm_box_padding_set (Evas_Object *obj, Evas_Coord horizontal, Evas_Coord vertical) |
Sets the space (padding) between the box's elements. | |
void | elm_box_padding_get (const Evas_Object *obj, Evas_Coord *horizontal, Evas_Coord *vertical) |
Gets the space (padding) between the box's elements. | |
void | elm_box_align_set (Evas_Object *obj, double horizontal, double vertical) |
Sets the alignment of the whole bounding box of contents. | |
void | elm_box_align_get (const Evas_Object *obj, double *horizontal, double *vertical) |
Gets the alignment of the whole bounding box of contents. | |
void | elm_box_recalculate (Evas_Object *obj) |
Forces the box to recalculate its children packing. | |
void | elm_box_layout_set (Evas_Object *obj, Evas_Object_Box_Layout cb, const void *data, Ecore_Cb free_data) |
Sets the layout defining function to be used by the box. | |
void | elm_box_layout_transition (Evas_Object *obj, Evas_Object_Box_Data *priv, void *data) |
Special layout function that animates the transition from one layout to another. | |
Elm_Box_Transition * | elm_box_transition_new (const double duration, Evas_Object_Box_Layout start_layout, void *start_layout_data, Ecore_Cb start_layout_free_data, Evas_Object_Box_Layout end_layout, void *end_layout_data, Ecore_Cb end_layout_free_data, Ecore_Cb transition_end_cb, void *transition_end_data) |
Creates a new Elm_Box_Transition to animate the switch of the layouts. | |
void | elm_box_transition_free (void *data) |
Frees an Elm_Box_Transition instance created with elm_box_transition_new(). | |
Typedefs | |
typedef struct _Elm_Box_Transition | Elm_Box_Transition |
The structure type which is an opaque handler containing the parameters to perform an animated transition of the layout that the box uses. |
Typedef Documentation
The structure type which is an opaque handler containing the parameters to perform an animated transition of the layout that the box uses.
Function Documentation
Evas_Object* elm_box_add | ( | Evas_Object * | parent | ) |
Adds a new box to the parent.
- Since :
- 2.3.1
- Remarks:
- By default, the box is in the vertical mode and is non-homogeneous.
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object, otherwise
NULL
if it cannot be created
void elm_box_align_get | ( | const Evas_Object * | obj, |
double * | horizontal, | ||
double * | vertical | ||
) |
Gets the alignment of the whole bounding box of contents.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object [out] horizontal The horizontal alignment of elements [out] vertical The vertical alignment of elements
- See also:
- elm_box_align_set()
void elm_box_align_set | ( | Evas_Object * | obj, |
double | horizontal, | ||
double | vertical | ||
) |
Sets the alignment of the whole bounding box of contents.
This sets how the bounding box containing all the elements of the box, after their sizes and position has been calculated, is aligned within the space given for the whole box widget.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object [in] horizontal The horizontal alignment of elements [in] vertical The vertical alignment of elements
Eina_List* elm_box_children_get | ( | const Evas_Object * | obj | ) |
Retrieves a list of the objects packed into the box.
- Since :
- 2.3.1
- Remarks:
- This returns a new
Eina_List
with a pointer toEvas_Object
in its nodes. The order of the list corresponds to the packing order that the box uses. - You must free this list with eina_list_free() once you are done with it.
- Parameters:
-
[in] obj The box object
- Returns:
- The children objects list
void elm_box_clear | ( | Evas_Object * | obj | ) |
Clears the box of all its children.
This removes all the elements contained by the box, deleting the respective objects.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object
- See also:
- elm_box_unpack()
- elm_box_unpack_all()
Eina_Bool elm_box_homogeneous_get | ( | const Evas_Object * | obj | ) |
Gets whether the box is using the homogeneous mode.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object
- Returns:
EINA_TRUE
if it's homogeneous, otherwiseEINA_FALSE
void elm_box_homogeneous_set | ( | Evas_Object * | obj, |
Eina_Bool | homogeneous | ||
) |
Sets the box to arrange its children homogeneously.
- Since :
- 2.3.1
- Remarks:
- If enabled, homogeneous layout makes all items of the same size, according to the size of the largest of its children.
- This flag is ignored if a custom layout function is set.
- Parameters:
-
[in] obj The box object [in] homogeneous The homogeneous flag
Eina_Bool elm_box_horizontal_get | ( | const Evas_Object * | obj | ) |
Gets the horizontal orientation.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object
- Returns:
EINA_TRUE
if the box is set to the horizontal mode, otherwiseEINA_FALSE
void elm_box_horizontal_set | ( | Evas_Object * | obj, |
Eina_Bool | horizontal | ||
) |
Sets the horizontal orientation.
- Since :
- 2.3.1
- Remarks:
- By default, the box object arranges their contents vertically from top to bottom.
-
By calling this function with horizontal as
EINA_TRUE
, the box becomes horizontal, arranging contents from left to right. - This flag is ignored if a custom layout function is set.
- Parameters:
-
[in] obj The box object [in] horizontal The horizontal flag ( EINA_TRUE
= horizontal,EINA_FALSE
= vertical)
void elm_box_layout_set | ( | Evas_Object * | obj, |
Evas_Object_Box_Layout | cb, | ||
const void * | data, | ||
Ecore_Cb | free_data | ||
) |
Sets the layout defining function to be used by the box.
- Since :
- 2.3.1
- Remarks:
- Whenever anything changes that requires the box in obj to recalculate the size and position of its elements, the function cb is called to determine what the layout of the children is.
-
Once a custom function is set, everything about the children layout is defined by it. The flags set by elm_box_horizontal_set() and elm_box_homogeneous_set() no longer have any meaning, and the values given by elm_box_padding_set() and elm_box_align_set() are up to the layout function to decide whether they are used and how. These last two are found in the priv parameter, of type
Evas_Object_Box_Data
, passed to cb. TheEvas_Object
that the function receives is not the Elementary widget, but the internal Evas Box it uses, so none of the functions described here can be used on it. -
Any of the layout functions in
Evas
can be used here, as well as the special elm_box_layout_transition(). - The final data argument received by cb is the same data passed here, and the free_data function is called to free it whenever the box is destroyed or another layout function is set.
-
Setting cb to
NULL
reverts back to the default layout function.
- Parameters:
-
[in] obj The box object [in] cb The callback function used for the layout [in] data The data that is passed to the layout function [in] free_data The function called to free data
- See also:
- elm_box_layout_transition()
void elm_box_layout_transition | ( | Evas_Object * | obj, |
Evas_Object_Box_Data * | priv, | ||
void * | data | ||
) |
Special layout function that animates the transition from one layout to another.
- Since :
- 2.3.1
- Remarks:
- Normally, when switching the layout function for a box, this reflects immediately on screen on the next render, but it's also possible to do this through an animated transition.
- This is done by creating an Elm_Box_Transition and setting the box layout to this function.
For example:
Elm_Box_Transition *t = elm_box_transition_new(1.0, evas_object_box_layout_vertical, // start NULL, // data for initial layout NULL, // free function for initial data evas_object_box_layout_horizontal, // end NULL, // data for final layout NULL, // free function for final data anim_end, // will be called when animation ends NULL); // data for anim_end function\ elm_box_layout_set(box, elm_box_layout_transition, t, elm_box_transition_free);
- Remarks:
- This function can only be used with elm_box_layout_set(). Calling it directly does not have the expected results.
- Parameters:
-
[in] obj The box object [in] priv The smart data of the obj
[in] data The data pointer passed
void elm_box_pack_after | ( | Evas_Object * | obj, |
Evas_Object * | subobj, | ||
Evas_Object * | after | ||
) |
Adds an object to the box after the indicated object.
This adds subobj to the box indicated after the object indicated with after. If after is not already in the box, the results are undefined. After means either to the right of the indicated object or below it depending on the orientation.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object [in] subobj The object to add to the box [in] after The object after which to add it
void elm_box_pack_before | ( | Evas_Object * | obj, |
Evas_Object * | subobj, | ||
Evas_Object * | before | ||
) |
Adds an object to the box before the indicated object.
This adds subobj to the box indicated before the object indicated with before. If before is not already in the box, the results are undefined. Before means either to the left of the indicated object or above it depending on the orientation.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object [in] subobj The object to add to the box [in] before The object before which to add it
void elm_box_pack_end | ( | Evas_Object * | obj, |
Evas_Object * | subobj | ||
) |
Adds an object at the end of the pack list.
- Since :
- 2.3.1
- Remarks:
- This packs subobj into the box obj, placing it last in the list of children objects. The actual position that the object gets on screen depends on the layout used. If no custom layout is set, it is at the bottom or right, depending on whether the box is vertical or horizontal, respectively.
- Parameters:
-
obj The box object subobj The object to add to the box
void elm_box_pack_start | ( | Evas_Object * | obj, |
Evas_Object * | subobj | ||
) |
Adds an object to the beginning of the pack list.
- Since :
- 2.3.1
- Remarks:
- This packs subobj into the box obj, placing it first in the list of children objects. The actual position that the object gets on screen depends on the layout used. If no custom layout is set, it is at the top or left, depending on whether the box is vertical or horizontal, respectively.
- Parameters:
-
[in] obj The box object [in] subobj The object to add to the box
void elm_box_padding_get | ( | const Evas_Object * | obj, |
Evas_Coord * | horizontal, | ||
Evas_Coord * | vertical | ||
) |
Gets the space (padding) between the box's elements.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object [out] horizontal The horizontal space between elements [out] vertical The vertical space between elements
- See also:
- elm_box_padding_set()
void elm_box_padding_set | ( | Evas_Object * | obj, |
Evas_Coord | horizontal, | ||
Evas_Coord | vertical | ||
) |
Sets the space (padding) between the box's elements.
- Since :
- 2.3.1
- Remarks:
- This gives the extra space in pixels that is added between a box child and its neighbors after its containing cell has been calculated. This padding is set for all elements in the box, besides any possible padding that individual elements may have through their size hints.
- Parameters:
-
[in] obj The box object [in] horizontal The horizontal space between elements [in] vertical The vertical space between elements
void elm_box_recalculate | ( | Evas_Object * | obj | ) |
Forces the box to recalculate its children packing.
- Since :
- 2.3.1
- Remarks:
- If any child is added or removed, the box does not calculate the values immediately rather it leaves it to the next main loop iteration. While this is great as it would save lots of recalculation, whenever you need to get the position of a recently added item you must force recalculation before doing so.
- Parameters:
-
[in] obj The box object
void elm_box_transition_free | ( | void * | data | ) |
Frees an Elm_Box_Transition instance created with elm_box_transition_new().
- Since :
- 2.3.1
- Remarks:
- This function is mostly useful as the free_data parameter in elm_box_layout_set() with elm_box_layout_transition().
- Parameters:
-
[in] data The Elm_Box_Transition instance to be freed
Elm_Box_Transition* elm_box_transition_new | ( | const double | duration, |
Evas_Object_Box_Layout | start_layout, | ||
void * | start_layout_data, | ||
Ecore_Cb | start_layout_free_data, | ||
Evas_Object_Box_Layout | end_layout, | ||
void * | end_layout_data, | ||
Ecore_Cb | end_layout_free_data, | ||
Ecore_Cb | transition_end_cb, | ||
void * | transition_end_data | ||
) |
Creates a new Elm_Box_Transition to animate the switch of the layouts.
- Since :
- 2.3.1
- Remarks:
- If you want to animate the change from one layout to another, you need to set the layout function of the box to elm_box_layout_transition(), passing user data to it as an instance of Elm_Box_Transition with the necessary information to perform this animation. The free function to set the layout is elm_box_transition_free().
- The parameters to create an Elm_Box_Transition sum up to how long it is, in seconds, a layout function to describe the initial point, another for the final position of the children and one function to be called when the whole animation ends. This last function is useful to set the definitive layout for the box, usually it is same as the end layout for the animation, but could be used to start another transition.
- Parameters:
-
[in] duration The duration of the transition in seconds [in] start_layout The layout function that is used to start the animation [in] start_layout_data The data to be passed to the start_layout function [in] start_layout_free_data The function to free start_layout_data [in] end_layout The layout function that is used to end the animation [in] end_layout_data The data parameter passed to end_layout end_layout_free_data The data to be passed to the end_layout function end_layout_free_data The function to free end_layout_data [in] transition_end_cb The callback function called when the animation ends [in] transition_end_data The data to be passed to transition_end_cb
- Returns:
- An instance of Elm_Box_Transition
void elm_box_unpack | ( | Evas_Object * | obj, |
Evas_Object * | subobj | ||
) |
Unpacks a box item.
This removes the object given by subobj from the box obj without deleting it.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object [in] subobj The object to unpack
- See also:
- elm_box_unpack_all()
- elm_box_clear()
void elm_box_unpack_all | ( | Evas_Object * | obj | ) |
Removes all the items from the box, without deleting them.
This clears the box from all its children, but doesn't delete the respective objects. If no other references of the box children exist, the objects is never deleted, and thus the application leaks the memory. Make sure when using this function that you hold a reference to all the objects in the box obj.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The box object
- See also:
- elm_box_clear()
- elm_box_unpack()