Tizen Native API
|
A container widget to arrange other widgets in a table where items can span multiple columns or rows - even overlap (and then be raised or lowered accordingly to adjust stacking if they do overlap).
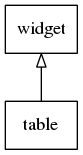
The row and column count is not fixed. The table widget adjusts itself when subobjects are added to it dynamically.
The most common way to use a table is:
table = elm_table_add(win); evas_object_show(table); elm_table_padding_set(table, space_between_columns, space_between_rows); elm_table_pack(table, table_content_object, x_coord, y_coord, colspan, rowspan); elm_table_pack(table, table_content_object, next_x_coord, next_y_coord, colspan, rowspan); elm_table_pack(table, table_content_object, other_x_coord, other_y_coord, colspan, rowspan);
Functions | |
Evas_Object * | elm_table_add (Evas_Object *parent) |
Adds a new table to the parent. | |
void | elm_table_homogeneous_set (Evas_Object *obj, Eina_Bool homogeneous) |
Sets the homogeneous layout in the table. | |
Eina_Bool | elm_table_homogeneous_get (const Evas_Object *obj) |
Gets the current table homogeneous mode. | |
void | elm_table_padding_set (Evas_Object *obj, Evas_Coord horizontal, Evas_Coord vertical) |
Sets padding between the cells. | |
void | elm_table_padding_get (const Evas_Object *obj, Evas_Coord *horizontal, Evas_Coord *vertical) |
Gets the padding between cells. | |
void | elm_table_pack (Evas_Object *obj, Evas_Object *subobj, int col, int row, int colspan, int rowspan) |
Adds a subobject on the table with the coordinates passed. | |
void | elm_table_unpack (Evas_Object *obj, Evas_Object *subobj) |
Removes the child from the table. | |
void | elm_table_clear (Evas_Object *obj, Eina_Bool clear) |
Removes all child objects from a table object. | |
void | elm_table_pack_set (Evas_Object *subobj, int x, int y, int w, int h) |
Sets the packing location of an existing child of the table. | |
void | elm_table_pack_get (Evas_Object *subobj, int *x, int *y, int *w, int *h) |
Gets the packing location of an existing child of the table. |
Function Documentation
Evas_Object* elm_table_add | ( | Evas_Object * | parent | ) |
Adds a new table to the parent.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object, otherwise
NULL
if it cannot be created
void elm_table_clear | ( | Evas_Object * | obj, |
Eina_Bool | clear | ||
) |
Removes all child objects from a table object.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The table object [in] clear If true
, the children are deleted, otherwisefalse
to just remove them from the table
Eina_Bool elm_table_homogeneous_get | ( | const Evas_Object * | obj | ) |
Gets the current table homogeneous mode.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The table object
- Returns:
- The boolean that indicates if the layout is homogeneous in the table (
EINA_TRUE
= homogeneous,EINA_FALSE
= no homogeneous)
void elm_table_homogeneous_set | ( | Evas_Object * | obj, |
Eina_Bool | homogeneous | ||
) |
Sets the homogeneous layout in the table.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] homogeneous The boolean value to set if the layout is homogeneous in the table ( EINA_TRUE
= homogeneous,EINA_FALSE
= no homogeneous)
void elm_table_pack | ( | Evas_Object * | obj, |
Evas_Object * | subobj, | ||
int | col, | ||
int | row, | ||
int | colspan, | ||
int | rowspan | ||
) |
Adds a subobject on the table with the coordinates passed.
- Since :
- 2.3.1
- Remarks:
- All positioning inside the table is relative to rows and columns, so a value of
0
for x and y, means the top left cell of the table, and a value of1
for w and h means subobj only takes that1
cell. - Note that columns and rows only guarantee 16bit unsigned values at best. That means that col + colspan AND row + rowspan must fit inside 16bit unsigned values cleanly. You have been warned once that values exceed 15bit storage, and attempting to use values that are not able to fit in 16bits result in failure.
- Parameters:
-
[in] obj The table object [in] subobj The subobject to be added to the table [in] col The column number [in] row The row number [in] colspan The column span [in] rowspan The row span
void elm_table_pack_get | ( | Evas_Object * | subobj, |
int * | x, | ||
int * | y, | ||
int * | w, | ||
int * | h | ||
) |
Gets the packing location of an existing child of the table.
- Since :
- 2.3.1
- Parameters:
-
[in] subobj The subobject to be modified in the table [out] x The row number [out] y The column number [out] w The row span [out] h The column span
- See also:
- elm_table_pack_set()
void elm_table_pack_set | ( | Evas_Object * | subobj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Sets the packing location of an existing child of the table.
- Since :
- 2.3.1
- Remarks:
- Modifies the position of an object already in the table.
-
All positioning inside the table is relative to rows and columns, so a value of
0
for x and y, means the top left cell of the table, and a value of1
for w and h means subobj only takes that1
cell.
- Parameters:
-
[in] subobj The subobject to be modified in the table [in] x The row number [in] y The column number [in] w The row span [in] h The column span
void elm_table_padding_get | ( | const Evas_Object * | obj, |
Evas_Coord * | horizontal, | ||
Evas_Coord * | vertical | ||
) |
Gets the padding between cells.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [out] horizontal The horizontal padding [out] vertical The vertical padding
void elm_table_padding_set | ( | Evas_Object * | obj, |
Evas_Coord | horizontal, | ||
Evas_Coord | vertical | ||
) |
Sets padding between the cells.
- Since :
- 2.3.1
- Remarks:
- Default value is
0
.
- Parameters:
-
[in] obj The layout object [in] horizontal The horizontal padding [in] vertical The vertical padding
void elm_table_unpack | ( | Evas_Object * | obj, |
Evas_Object * | subobj | ||
) |
Removes the child from the table.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The table object [in] subobj The subobject