Tizen Native API
|
Photocam is a widget meant specifically for displaying high-resolution digital camera photos, giving speedy feedback (fast * load), zooming and panning as well as fitting logic, all with low memory footprint.
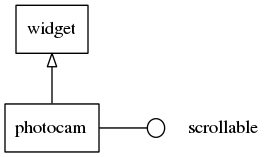
It is entirely focused on jpeg images, and takes advantage of the properties of the jpeg format (via Evas loader features in the jpeg loader).
Signals that you can add callbacks for are:
"clicked"
- This is called when a user has clicked the photo without dragging around."press"
- This is called when a user has pressed down on the photo."longpressed"
- This is called when a user has pressed down on the photo for a long time without dragging around."clicked,double"
- This is called when a user has double-clicked the photo."load"
- Photo load begins."loaded"
- This is called when the image file load is completed for the first view (low resolution blurry version)."load,detail"
- Detailed photo data load begins."loaded,detail"
- This is called when the image file load is completed with the detailed image data (full resolution needed)."zoom,start"
- Zoom animation started."zoom,stop"
- Zoom animation stopped."zoom,change"
- Zoom changed when using the auto zoom mode."scroll"
- The content has been scrolled (moved)."scroll,anim,start"
- Scrolling animation has started."scroll,anim,stop"
- Scrolling animation has stopped."scroll,drag,start"
- Dragging the contents around has started."scroll,drag,stop"
- Dragging the contents around has stopped.
This widget implements the elm-scrollable-interface interface, so that all (non-deprecated) functions for the base Scroller widget also work for photocam objects.
Some calls on the photocam's API are marked as deprecated, as they just wrap the scrollable widget's counterpart functions. Use the ones mentioned for each case of deprecation here. Eventually, the deprecated ones are discarded (next major release).
Functions | |
Evas_Object * | elm_photocam_add (Evas_Object *parent) |
Adds a new Photocam object. | |
Evas_Load_Error | elm_photocam_file_set (Evas_Object *obj, const char *file) |
Sets the photo file to be shown. | |
const char * | elm_photocam_file_get (const Evas_Object *obj) |
Gets the path of the current image file. | |
void | elm_photocam_zoom_set (Evas_Object *obj, double zoom) |
Sets the zoom level of the photo. | |
double | elm_photocam_zoom_get (const Evas_Object *obj) |
Gets the zoom level of the photo. | |
void | elm_photocam_zoom_mode_set (Evas_Object *obj, Elm_Photocam_Zoom_Mode mode) |
Sets the zoom mode. | |
Elm_Photocam_Zoom_Mode | elm_photocam_zoom_mode_get (const Evas_Object *obj) |
Gets the zoom mode. | |
void | elm_photocam_image_size_get (const Evas_Object *obj, int *w, int *h) |
Gets the current image pixel's width and height. | |
void | elm_photocam_image_region_get (const Evas_Object *obj, int *x, int *y, int *w, int *h) |
Gets the region of the image that is currently shown. | |
void | elm_photocam_image_region_show (Evas_Object *obj, int x, int y, int w, int h) |
Sets the viewed region of the image. | |
void | elm_photocam_image_region_bring_in (Evas_Object *obj, int x, int y, int w, int h) |
Brings in the viewed portion of the image. | |
void | elm_photocam_paused_set (Evas_Object *obj, Eina_Bool paused) |
Sets the paused state for the photocam. | |
Eina_Bool | elm_photocam_paused_get (const Evas_Object *obj) |
Gets the paused state for the photocam. | |
Evas_Object * | elm_photocam_internal_image_get (const Evas_Object *obj) |
Gets the internal low-resolution image used for the photocam. | |
void | elm_photocam_gesture_enabled_set (Evas_Object *obj, Eina_Bool gesture) |
Sets the gesture state for the photocam. | |
Eina_Bool | elm_photocam_gesture_enabled_get (const Evas_Object *obj) |
Gets the gesture state for the photocam. |
Enumeration Type Documentation
Enumeration that defines the types of zoom available.
- Enumerator:
Function Documentation
Evas_Object* elm_photocam_add | ( | Evas_Object * | parent | ) |
Adds a new Photocam object.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object, otherwise
NULL
if it cannot be created
const char* elm_photocam_file_get | ( | const Evas_Object * | obj | ) |
Gets the path of the current image file.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object
- Returns:
- The path
- See also:
- elm_photocam_file_set()
Evas_Load_Error elm_photocam_file_set | ( | Evas_Object * | obj, |
const char * | file | ||
) |
Sets the photo file to be shown.
This sets (and shows) the specified file (with a relative or absolute path) and returns a load error (same error that evas_object_image_load_error_get() returns). The image changes and adjusts its size at this point and begins a background load process for this photo that at some time in the future is displayed at the full quality needed.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [in] file The photo file
- Returns:
- The return error (see EVAS_LOAD_ERROR_NONE, EVAS_LOAD_ERROR_GENERIC)
Eina_Bool elm_photocam_gesture_enabled_get | ( | const Evas_Object * | obj | ) |
Gets the gesture state for the photocam.
This gets the current gesture state for the photocam object.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object
- Returns:
- The current gesture state
- See also:
- elm_photocam_gesture_enabled_set()
void elm_photocam_gesture_enabled_set | ( | Evas_Object * | obj, |
Eina_Bool | gesture | ||
) |
Sets the gesture state for the photocam.
This sets the gesture state to on(EINA_TRUE
) or off (EINA_FALSE
) for the photocam. The default is off. This starts multi touch zooming.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [in] gesture The gesture state to set
void elm_photocam_image_region_bring_in | ( | Evas_Object * | obj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Brings in the viewed portion of the image.
- Since :
- 2.3.1
- Remarks:
- This shows the region of the image using animation.
- Parameters:
-
[in] obj The photocam object [in] x The X-coordinate of the region in the image's original pixels [in] y The Y-coordinate of the region in the image's original pixels [in] w The width of the region in the image's original pixels [in] h The height of the region in the image's original pixels
void elm_photocam_image_region_get | ( | const Evas_Object * | obj, |
int * | x, | ||
int * | y, | ||
int * | w, | ||
int * | h | ||
) |
Gets the region of the image that is currently shown.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [out] x A pointer to the X-coordinate of the region [out] y A pointer to the Y-coordinate of the region [out] w A pointer to the width [out] h A pointer to the height
void elm_photocam_image_region_show | ( | Evas_Object * | obj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Sets the viewed region of the image.
- Since :
- 2.3.1
- Remarks:
- This shows the region of the image without using animation.
- Parameters:
-
[in] obj The photocam object [in] x The X-coordinate of the region in the image's original pixels [in] y The Y-coordinate of the region in the image's original pixels [in] w The width of the region in the image's original pixels [in] h The height of the region in the image's original pixels
void elm_photocam_image_size_get | ( | const Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Gets the current image pixel's width and height.
This gets the current photo pixel's width and height (for the original). The size is returned in the integers w and h that are pointed to.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [out] w A pointer to the width [out] h A pointer to the height
Evas_Object* elm_photocam_internal_image_get | ( | const Evas_Object * | obj | ) |
Gets the internal low-resolution image used for the photocam.
This gets the internal image object inside the photocam. Do not modify it. It is for inspection only, and hooking callbacks to, nothing else. It may be deleted at any time.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object
- Returns:
- The internal image object handle, otherwise
NULL
if none exist
Eina_Bool elm_photocam_paused_get | ( | const Evas_Object * | obj | ) |
Gets the paused state for the photocam.
This gets the current paused state for the photocam object.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object
- Returns:
- The current paused state
- See also:
- elm_photocam_paused_set()
void elm_photocam_paused_set | ( | Evas_Object * | obj, |
Eina_Bool | paused | ||
) |
Sets the paused state for the photocam.
This sets the paused state to on(EINA_TRUE
) or off (EINA_FALSE
) for the photocam. The default is off. This stops zooming using animation on zoom level changes and changes instantly. This stops any existing animations that are running.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [in] paused The pause state to set
double elm_photocam_zoom_get | ( | const Evas_Object * | obj | ) |
Gets the zoom level of the photo.
This returns the current zoom level of the photocam object. Note that if you set the fill mode to a value other than ELM_PHOTOCAM_ZOOM_MODE_MANUAL
(which is the default), the zoom level may be changed at any time by the photocam object itself to account for the photo size and the photocam viewport size.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object
- Returns:
- The current zoom level
Elm_Photocam_Zoom_Mode elm_photocam_zoom_mode_get | ( | const Evas_Object * | obj | ) |
Gets the zoom mode.
This gets the current zoom mode of the photocam object.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object
- Returns:
- The current zoom mode
- See also:
- elm_photocam_zoom_mode_set()
void elm_photocam_zoom_mode_set | ( | Evas_Object * | obj, |
Elm_Photocam_Zoom_Mode | mode | ||
) |
Sets the zoom mode.
This sets the zoom mode to manual or one of the several automatic levels. Manual (ELM_PHOTOCAM_ZOOM_MODE_MANUAL
) means that zoom is set manually by elm_photocam_zoom_set() and stays at that level until changed by code or until the zoom mode is changed. This is the default mode. The Automatic modes allow the photocam object to automatically adjust the zoom mode based on its properties. ELM_PHOTOCAM_ZOOM_MODE_AUTO_FIT
adjusts the zoom so that the photo fits EXACTLY inside the scroll frame with no pixels outside this region. ELM_PHOTOCAM_ZOOM_MODE_AUTO_FILL
is similar but ensures that no pixels within the frame are left unfilled.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [in] mode The desired mode
void elm_photocam_zoom_set | ( | Evas_Object * | obj, |
double | zoom | ||
) |
Sets the zoom level of the photo.
This sets the zoom level. 1
is 1:1 pixel for pixel. 2
is 2:1 (that is 2x2 photo pixels displays as 1 on-screen pixel). 4:1 is 4x4 photo pixels as 1 screen pixel, and so on. The zoom parameter must be greater than 0
. It is suggested to stick to powers of 2
. (1, 2, 4, 8, 16, 32, etc.).
- Since :
- 2.3.1
- Parameters:
-
[in] obj The photocam object [in] zoom The zoom level to set