Tizen Native API
|
Image widget allows one to load and display an image file on it, be it from a disk file or from a memory region.
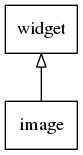
Exceptionally, one may also load an Edje group as the contents of the image. In this case, though, most of the functions of the image API act as a no-op.
An Elementary image object is a direct realization of elm-image-class.
One can tune various properties of the image, like:
- pre-scaling,
- smooth scaling,
- orientation,
- aspect ratio during resizes, etc.
An image object may also be made a valid source and destination for drag and drop actions, through the elm_image_editable_set() call.
Signals that you can add callbacks for are:
"drop"
- This is called when a user has dropped an image typed object onto the object in question, the event info argument is the path to that image file."clicked"
- This is called when a user has clicked the image.
Functions | |
Evas_Object * | elm_image_add (Evas_Object *parent) |
Adds a new image to the parent. | |
Eina_Bool | elm_image_memfile_set (Evas_Object *obj, const void *img, size_t size, const char *format, const char *key) |
Sets a location in the memory to be used as an image object's source bitmap. | |
Eina_Bool | elm_image_file_set (Evas_Object *obj, const char *file, const char *group) |
Sets the file that is used as the image's source. | |
void | elm_image_file_get (const Evas_Object *obj, const char **file, const char **group) |
Gets the file that is used as an image. | |
void | elm_image_smooth_set (Evas_Object *obj, Eina_Bool smooth) |
Sets the smooth effect for an image. | |
Eina_Bool | elm_image_smooth_get (const Evas_Object *obj) |
Gets the smooth effect for an image. | |
void | elm_image_object_size_get (const Evas_Object *obj, int *w, int *h) |
Gets the current size of the image. | |
void | elm_image_no_scale_set (Evas_Object *obj, Eina_Bool no_scale) |
Disables scaling of this object. | |
Eina_Bool | elm_image_no_scale_get (const Evas_Object *obj) |
Gets whether scaling is disabled on the object. | |
void | elm_image_resizable_set (Evas_Object *obj, Eina_Bool size_up, Eina_Bool size_down) |
Sets whether the object is (up/down) resizeable. | |
void | elm_image_resizable_get (const Evas_Object *obj, Eina_Bool *size_up, Eina_Bool *size_down) |
Gets whether the object is (up/down) resizable. | |
void | elm_image_fill_outside_set (Evas_Object *obj, Eina_Bool fill_outside) |
Sets whether the image fills the entire object area, when keeping the aspect ratio. | |
Eina_Bool | elm_image_fill_outside_get (const Evas_Object *obj) |
Gets whether the object is filled outside. | |
void | elm_image_preload_disabled_set (Evas_Object *obj, Eina_Bool disabled) |
Enables or disables preloading of the image. | |
void | elm_image_prescale_set (Evas_Object *obj, int size) |
Sets the prescale size for the image. | |
int | elm_image_prescale_get (const Evas_Object *obj) |
Gets the prescale size for the image. | |
void | elm_image_orient_set (Evas_Object *obj, Elm_Image_Orient orient) |
Sets the image orientation. | |
Elm_Image_Orient | elm_image_orient_get (const Evas_Object *obj) |
Gets the image orientation. | |
void | elm_image_editable_set (Evas_Object *obj, Eina_Bool set) |
Makes the image 'editable'. | |
Eina_Bool | elm_image_editable_get (const Evas_Object *obj) |
Checks whether the image is 'editable'. | |
Evas_Object * | elm_image_object_get (const Evas_Object *obj) |
Gets the inlined image object of the image widget. | |
void | elm_image_aspect_fixed_set (Evas_Object *obj, Eina_Bool fixed) |
Set whether the original aspect ratio of the image should be kept on resize. | |
Eina_Bool | elm_image_aspect_fixed_get (const Evas_Object *obj) |
Gets whether the object retains the original aspect ratio. | |
Eina_Bool | elm_image_animated_available_get (const Evas_Object *obj) |
Gets whether an image object supports animation. | |
void | elm_image_animated_set (Evas_Object *obj, Eina_Bool animated) |
Sets whether an image object (which supports animation) is to animate itself. | |
Eina_Bool | elm_image_animated_get (const Evas_Object *obj) |
Gets whether an image object has animation enabled. | |
void | elm_image_animated_play_set (Evas_Object *obj, Eina_Bool play) |
Starts or stops an image object's animation. | |
Eina_Bool | elm_image_animated_play_get (const Evas_Object *obj) |
Gets whether an image object is under animation. |
Enumeration Type Documentation
enum Elm_Image_Orient |
Enumeration that defines the possible orientation options for elm_image_orient_set().
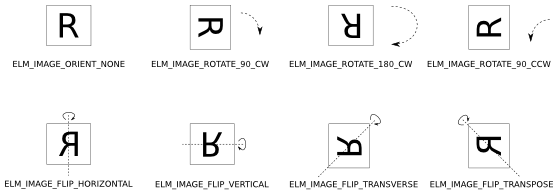
- Enumerator:
Function Documentation
Evas_Object* elm_image_add | ( | Evas_Object * | parent | ) |
Adds a new image to the parent.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object, otherwise
NULL
if it cannot be created
- See also:
- elm_image_file_set()
Eina_Bool elm_image_animated_available_get | ( | const Evas_Object * | obj | ) |
Gets whether an image object supports animation.
This function returns if this Elementary image object's internal image can be animated. Currently Evas only supports GIF animation. If the return value is EINA_FALSE
, other elm_image_animated_xxx
API calls won't work.
- Since :
- 2.3.1
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if the image supports animation, otherwiseEINA_FALSE
- See also:
- elm_image_animated_set()
Eina_Bool elm_image_animated_get | ( | const Evas_Object * | obj | ) |
Gets whether an image object has animation enabled.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if the image has animation enabled, otherwiseEINA_FALSE
- See also:
- elm_image_animated_set()
Eina_Bool elm_image_animated_play_get | ( | const Evas_Object * | obj | ) |
Gets whether an image object is under animation.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if the image is being animated, otherwiseEINA_FALSE
- See also:
- elm_image_animated_play_get()
void elm_image_animated_play_set | ( | Evas_Object * | obj, |
Eina_Bool | play | ||
) |
Starts or stops an image object's animation.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- To actually start playing any image object's animation, if it supports it, one must do something like:
if (elm_image_animated_available_get(img)) { elm_image_animated_set(img, EINA_TRUE); elm_image_animated_play_set(img, EINA_TRUE); }
- Remarks:
- elm_image_animated_set() enables animation on the image, but does not start it yet. This is the function one uses to start and stop animations on image objects.
- Parameters:
-
[in] obj The image object [in] play If EINA_TRUE
animation is started, otherwiseEINA_FALSE
Default isEINA_FALSE
.
void elm_image_animated_set | ( | Evas_Object * | obj, |
Eina_Bool | animated | ||
) |
Sets whether an image object (which supports animation) is to animate itself.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- An image object, even if it supports animation, is displayed by default without animation. Call this function with animated set to
EINA_TRUE
to enable its animation. To start or stop the animation, use elm_image_animated_play_set().
- Parameters:
-
[in] obj The image object [in] animated If EINA_TRUE
the object is to animate itself, otherwiseEINA_FALSE
Default isEINA_FALSE
.
Eina_Bool elm_image_aspect_fixed_get | ( | const Evas_Object * | obj | ) |
Gets whether the object retains the original aspect ratio.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if the object keeps the original aspect, otherwiseEINA_FALSE
void elm_image_aspect_fixed_set | ( | Evas_Object * | obj, |
Eina_Bool | fixed | ||
) |
Set whether the original aspect ratio of the image should be kept on resize.
- Since :
- 2.3.1
- Remarks:
- The original aspect ratio (width / height) of the image is usually distorted to match the object's size. Enabling this option retains this original aspect, and the way that the image is fit into the object's area depends on the option set by elm_image_fill_outside_set().
- Parameters:
-
[in] obj The image object [in] fixed If EINA_TRUE
the image should retain the aspect, otherwiseEINA_FALSE
Eina_Bool elm_image_editable_get | ( | const Evas_Object * | obj | ) |
Checks whether the image is 'editable'.
- Since :
- 2.3.1
- Remarks:
- A return value of
EINA_TRUE
means the image is a valid drag target for drag and drop, and can be cut or pasted.
- Parameters:
-
[in] obj The image object
- Returns:
- The boolean value to turn on or turn off editability
void elm_image_editable_set | ( | Evas_Object * | obj, |
Eina_Bool | set | ||
) |
Makes the image 'editable'.
- Since :
- 2.3.1
- Remarks:
- This means the image is a valid drag target for drag and drop, and can be cut or pasted.
- Parameters:
-
[in] obj The image object [in] set The boolean value to turn on or turn off editability
Default isEINA_FALSE
.
void elm_image_file_get | ( | const Evas_Object * | obj, |
const char ** | file, | ||
const char ** | group | ||
) |
Gets the file that is used as an image.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [out] file The path to the file [out] group The group that the image belongs to, in edje file
- See also:
- elm_image_file_set()
Eina_Bool elm_image_file_set | ( | Evas_Object * | obj, |
const char * | file, | ||
const char * | group | ||
) |
Sets the file that is used as the image's source.
This function triggers the Edje file case based on the extension of the file string (expect ".edj"
, for this case). If one wants to force this type of file independent of the extension, elm_image_file_edje_set() must be used, instead.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [in] file The path to the file that is used as an image source [in] group The group that the image belongs to, in case it's an EET (including Edje case) file
- Returns:
- (
EINA_TRUE
= success,EINA_FALSE
= error)
- See also:
- elm_image_file_get()
Eina_Bool elm_image_fill_outside_get | ( | const Evas_Object * | obj | ) |
Gets whether the object is filled outside.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if the object is filled outside, otherwiseEINA_FALSE
- See also:
- elm_image_fill_outside_set()
void elm_image_fill_outside_set | ( | Evas_Object * | obj, |
Eina_Bool | fill_outside | ||
) |
Sets whether the image fills the entire object area, when keeping the aspect ratio.
- Since :
- 2.3.1
- Remarks:
- When the image should keep its aspect ratio even if resized to another aspect ratio, there are two possibilities to resize it: keep the entire image inside the limits of the height and width of the object (fill_outside is
EINA_FALSE
) or let the extra width or height go outside the object, and the image fills the entire object (fill_outside isEINA_TRUE
). -
This option has no effect if elm_image_aspect_fixed_set() is set to
EINA_FALSE
.
- Parameters:
-
[in] obj The image object [in] fill_outside If EINA_TRUE
the object is filled outside, otherwiseEINA_FALSE
Default isEINA_FALSE
.
Eina_Bool elm_image_memfile_set | ( | Evas_Object * | obj, |
const void * | img, | ||
size_t | size, | ||
const char * | format, | ||
const char * | key | ||
) |
Sets a location in the memory to be used as an image object's source bitmap.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- This function is handy when the contents of an image file are mapped into the memory, for example.
-
The format string should be something like
"png"
,"jpg"
,"tga"
,"tiff"
,"bmp"
etc, when provided (NULL
, on the contrary). This improves the loader performance as it tries the "correct" loader first, before trying a range of other possible loaders until one succeeds.
- Parameters:
-
[in] obj The image object [in] img The binary data that is used as an image source [in] size The size of the binary data blob img [in] format The (Optional) expected format of img bytes [in] key The optional indexing key of img to be passed to the image loader (eg. if img is a memory-mapped EET file)
- Returns:
- (
EINA_TRUE
= success,EINA_FALSE
= error)
Eina_Bool elm_image_no_scale_get | ( | const Evas_Object * | obj | ) |
Gets whether scaling is disabled on the object.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if scaling is disabled, otherwiseEINA_FALSE
- See also:
- elm_image_no_scale_set()
void elm_image_no_scale_set | ( | Evas_Object * | obj, |
Eina_Bool | no_scale | ||
) |
Disables scaling of this object.
This function disables scaling of elm_image widget through the function elm_object_scale_set(). However, this does not affect the widget size/resize in any way. For that effect, take a look at elm_image_resizable_set().
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [in] no_scale If EINA_TRUE
the object is not scalable, otherwiseEINA_FALSE
Default isEINA_FALSE
.
Evas_Object* elm_image_object_get | ( | const Evas_Object * | obj | ) |
Gets the inlined image object of the image widget.
This function allows one to get the underlying Evas_Object
of type Image from this elementary widget. It can be useful to do things like get the pixel data, save the image to a file, etc.
- Since :
- 2.3.1
- Remarks:
- Be careful to not manipulate it, as it is under the control of elementary.
- Parameters:
-
[in] obj The image object to get the inlined image from
- Returns:
- The inlined image object, otherwise
NULL
if none exist
void elm_image_object_size_get | ( | const Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Gets the current size of the image.
- Since :
- 2.3.1
- Remarks:
- This is the real size of the image, not the size of the object.
- Parameters:
-
[in] obj The image object [out] w The pointer to the store width, otherwise NULL
[out] h The pointer to the store height, otherwise NULL
Elm_Image_Orient elm_image_orient_get | ( | const Evas_Object * | obj | ) |
Gets the image orientation.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
- The image orientation Elm_Image_Orient
- See also:
- elm_image_orient_set()
- Elm_Image_Orient
void elm_image_orient_set | ( | Evas_Object * | obj, |
Elm_Image_Orient | orient | ||
) |
Sets the image orientation.
This function allows to rotate or flip the given image.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [in] orient The image orientation Elm_Image_Orient
Default is ELM_IMAGE_ORIENT_NONE.
- See also:
- elm_image_orient_get()
- Elm_Image_Orient
void elm_image_preload_disabled_set | ( | Evas_Object * | obj, |
Eina_Bool | disabled | ||
) |
Enables or disables preloading of the image.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [in] disabled If EINA_TRUE
preloading is disabled, otherwiseEINA_FALSE
int elm_image_prescale_get | ( | const Evas_Object * | obj | ) |
Gets the prescale size for the image.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
- The prescale size
- See also:
- elm_image_prescale_set()
void elm_image_prescale_set | ( | Evas_Object * | obj, |
int | size | ||
) |
Sets the prescale size for the image.
This function sets a new size for pixmap representation of the given image. It allows the image to be loaded in advance in the specified size, reducing the memory usage and load time when loading a big image with load size set to a smaller size.
- Since :
- 2.3.1
- Remarks:
- It's equivalent to the elm_bg_load_size_set() function for bg.
- This is just a hint, the real size of the pixmap may differ depending on the type of image being loaded, being bigger than requested.
- Parameters:
-
[in] obj The image object [in] size The prescale size
This value is used for both width and height.
void elm_image_resizable_get | ( | const Evas_Object * | obj, |
Eina_Bool * | size_up, | ||
Eina_Bool * | size_down | ||
) |
Gets whether the object is (up/down) resizable.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [out] size_up The boolean value to set if the object is resizable up [out] size_down The boolean to set if the object is resizable down
- See also:
- elm_image_resizable_set()
void elm_image_resizable_set | ( | Evas_Object * | obj, |
Eina_Bool | size_up, | ||
Eina_Bool | size_down | ||
) |
Sets whether the object is (up/down) resizeable.
This function limits the image resize ability. If size_up is set to EINA_FALSE
, the object can't have its height or width resized to a value higher than the original image size. Same is valid for size_down.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object [in] size_up The boolean value to set if the object is resizeable up
Default isEINA_TRUE
.[in] size_down The boolean value to set if the object is resizeable down
Default isEINA_TRUE
.
- See also:
- elm_image_resizable_get()
Eina_Bool elm_image_smooth_get | ( | const Evas_Object * | obj | ) |
Gets the smooth effect for an image.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The image object
- Returns:
EINA_TRUE
if smooth scaling is enabled, otherwiseEINA_FALSE
- See also:
- elm_image_smooth_get()
void elm_image_smooth_set | ( | Evas_Object * | obj, |
Eina_Bool | smooth | ||
) |
Sets the smooth effect for an image.
This sets the scaling algorithm to be used when scaling the image. Smooth scaling provides a better resulting image, but is slower.
- Since :
- 2.3.1
- Remarks:
- The smooth scaling should be disabled when making animations that change the image size, since it is faster. Animations that don't require resizing of the image can keep the smooth scaling enabled (even if the image is already scaled, since the scaled image is cached).
- Parameters:
-
[in] obj The image object [in] smooth If EINA_TRUE
smooth scaling should be used, otherwiseEINA_FALSE
Default isEINA_TRUE
.
- See also:
- elm_image_smooth_get()