Tizen Native API
|
A list widget is a container whose children are displayed vertically or horizontally, in order, and can be selected.
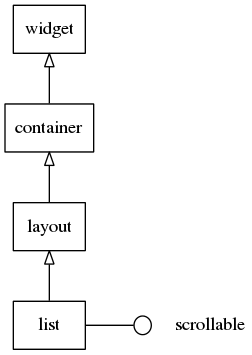
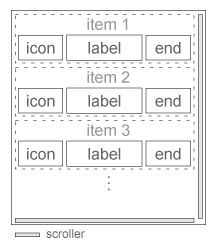
The list can accept only one or multiple item selections. Also it has many modes of items displaying.
A list is a very simple type of list widget. For more robust lists, Genlist should be used.
This widget inherits from the Layout one, so that all the functions acting on it also work for list objects.
This widget emits the following signals, besides the ones sent from Layout :
"activated"
- The user has double-clicked or pressed (enter|return|spacebar) an item. The event_info parameter is the item that is activated."clicked,double"
- The user has double-clicked an item. The event_info parameter is the item that is double-clicked."selected"
- When the user selected an item."unselected"
- When the user unselected an item."longpressed"
- An item in the list is long-pressed."edge,top"
- The list is scrolled till the top edge."edge,bottom"
- The list is scrolled till the bottom edge."edge,left"
- The list is scrolled till the left edge."edge,right"
- The list is scrolled till the right edge."language,changed"
- The program's language is changed."highlighted"
- An item in the list is pressed and highlighted. The event_info parameter is the item that is highlighted."unhighlighted"
- An item in the list is unpressed and unhighlighted. The event_info parameter is the item that is unhighlighted.
Available styles for it are:
"default"
The default content parts of the list items that you can use are:
"start"
- A start position object in the list item."end"
- An end position object in the list item.
The default text parts of the list items that you can use are:
"default"
- The label in the list item.
Supported common elm_object_item
APIs.
- elm_object_item_disabled_set
- elm_object_item_disabled_get
- elm_object_item_part_text_set
- elm_object_item_part_text_get
- elm_object_item_part_content_set
- elm_object_item_part_content_get
- elm_object_item_part_content_unset
This widget implements the elm-scrollable-interface interface, so that all (non-deprecated) functions for the base Scroller widget also work for lists.
Some calls on the list's API are marked as deprecated, as they just wrap the scrollable widgets counterpart functions. Use the ones mentioned for each case of deprecation here. Eventually, the deprecated ones are discarded (next major release).
Functions | |
Evas_Object * | elm_list_add (Evas_Object *parent) |
Adds a new list widget to the given parent Elementary (container) object. | |
void | elm_list_go (Evas_Object *obj) |
Starts the list. | |
void | elm_list_multi_select_set (Evas_Object *obj, Eina_Bool multi) |
Enables or disables multiple items selection on the list object. | |
Eina_Bool | elm_list_multi_select_get (const Evas_Object *obj) |
Gets a value that indicates whether multiple items selection is enabled. | |
void | elm_list_mode_set (Evas_Object *obj, Elm_List_Mode mode) |
Sets which mode to use for the list object. | |
Elm_List_Mode | elm_list_mode_get (const Evas_Object *obj) |
Gets the mode that the list is at. | |
void | elm_list_horizontal_set (Evas_Object *obj, Eina_Bool horizontal) |
Enables or disables horizontal mode on the list object. | |
Eina_Bool | elm_list_horizontal_get (const Evas_Object *obj) |
Gets a value that indicates whether the horizontal mode is enabled. | |
void | elm_list_select_mode_set (Evas_Object *obj, Elm_Object_Select_Mode mode) |
Sets the list select mode. | |
Elm_Object_Select_Mode | elm_list_select_mode_get (const Evas_Object *obj) |
Gets the list select mode. | |
Elm_Object_Item * | elm_list_item_append (Evas_Object *obj, const char *label, Evas_Object *icon, Evas_Object *end, Evas_Smart_Cb func, const void *data) |
Appends a new item to the list object. | |
Elm_Object_Item * | elm_list_item_prepend (Evas_Object *obj, const char *label, Evas_Object *icon, Evas_Object *end, Evas_Smart_Cb func, const void *data) |
Prepends a new item to the list object. | |
Elm_Object_Item * | elm_list_item_insert_before (Evas_Object *obj, Elm_Object_Item *before, const char *label, Evas_Object *icon, Evas_Object *end, Evas_Smart_Cb func, const void *data) |
Inserts a new item into the list object before item before. | |
Elm_Object_Item * | elm_list_item_insert_after (Evas_Object *obj, Elm_Object_Item *after, const char *label, Evas_Object *icon, Evas_Object *end, Evas_Smart_Cb func, const void *data) |
Inserts a new item into the list object after item after. | |
Elm_Object_Item * | elm_list_item_sorted_insert (Evas_Object *obj, const char *label, Evas_Object *icon, Evas_Object *end, Evas_Smart_Cb func, const void *data, Eina_Compare_Cb cmp_func) |
Inserts a new item into the sorted list object. | |
void | elm_list_clear (Evas_Object *obj) |
Removes all list items. | |
const Eina_List * | elm_list_items_get (const Evas_Object *obj) |
Gets a list of all the list items. | |
Elm_Object_Item * | elm_list_selected_item_get (const Evas_Object *obj) |
Gets the selected item. | |
const Eina_List * | elm_list_selected_items_get (const Evas_Object *obj) |
Returns a list of the currently selected list items. | |
void | elm_list_item_selected_set (Elm_Object_Item *it, Eina_Bool selected) |
Sets the selected state of an item. | |
Eina_Bool | elm_list_item_selected_get (const Elm_Object_Item *it) |
Gets whether the it is selected. | |
void | elm_list_item_separator_set (Elm_Object_Item *it, Eina_Bool setting) |
Sets or unsets an item as a separator. | |
Eina_Bool | elm_list_item_separator_get (const Elm_Object_Item *it) |
Gets a value that indicates whether an item is a separator. | |
void | elm_list_item_show (Elm_Object_Item *it) |
Shows it in the list view. | |
void | elm_list_item_bring_in (Elm_Object_Item *it) |
Brings in the given item to the list view. | |
Evas_Object * | elm_list_item_object_get (const Elm_Object_Item *it) |
Gets the base object of the item. | |
Elm_Object_Item * | elm_list_item_prev (const Elm_Object_Item *it) |
Gets the item before it in list. | |
Elm_Object_Item * | elm_list_item_next (const Elm_Object_Item *it) |
Gets the item after it in list. | |
Elm_Object_Item * | elm_list_first_item_get (const Evas_Object *obj) |
Gets the first item in the list. | |
Elm_Object_Item * | elm_list_last_item_get (const Evas_Object *obj) |
Gets the last item in the list. | |
Elm_Object_Item * | elm_list_at_xy_item_get (const Evas_Object *obj, Evas_Coord x, Evas_Coord y, int *posret) |
Gets the item that is at the x, y canvas coordinates. |
Enumeration Type Documentation
enum Elm_List_Mode |
Enumeration that sets the list's resizing behavior, transverse axis scrolling and items cropping. See each mode's description for more details.
- Remarks:
- The default value is ELM_LIST_SCROLL.
- The values here don't work as bitmasks, only one can be chosen at a time.
- See also:
- elm_list_mode_set()
- elm_list_mode_get()
- Enumerator:
ELM_LIST_COMPRESS The list won't set any of its size hints to inform how a possible container should resize it. If it's not created as a "resize object", it might end with zeroed dimensions. The list respects the container's geometry and if any of its items don't fit into its transverse axis, one won't be able to scroll it in that direction
ELM_LIST_SCROLL The default value. This is the same as ELM_LIST_COMPRESS, with the exception that if any of its items don't fit into its transverse axis, one won't be able to scroll it in that direction
ELM_LIST_LIMIT Sets a minimum size hint on the list object, so that containers may respect it (and resize itself to fit the child properly). More specifically, a minimum size hint is set for its transverse axis, so that the largest item in that direction fits well. This is naturally bound by the list object's maximum size hints, set externally
ELM_LIST_EXPAND Besides setting a minimum size on the transverse axis, just like on ELM_LIST_LIMIT, the list sets a minimum size on the longitudinal axis, trying to reserve space for all its children to be visible at a time. This is naturally bound by the list object's maximum size hints, set externally
ELM_LIST_LAST Indicates an error if returned by elm_list_mode_get()
Function Documentation
Evas_Object* elm_list_add | ( | Evas_Object * | parent | ) |
Adds a new list widget to the given parent Elementary (container) object.
This function inserts a new list widget on the canvas.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- A new list widget handle, otherwise
NULL
in case of an error
Elm_Object_Item* elm_list_at_xy_item_get | ( | const Evas_Object * | obj, |
Evas_Coord | x, | ||
Evas_Coord | y, | ||
int * | posret | ||
) |
Gets the item that is at the x, y canvas coordinates.
This returns the item at the given coordinates (which are canvas relative, not object-relative). If an item is at that coordinate, that item handle is returned, and if posret is not NULL
, the integer pointed to is set to a value of -1
, 0
or 1
, depending on whether the coordinate is at the upper portion of that item (-1), in the middle section (0) or at the lower part (1). If NULL
is returned as an item (no item found there), then posret may indicate -1
or 1
based on whether the coordinate is above or below all items in the list respectively.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object [in] x The input x coordinate [in] y The input y coordinate [out] posret The position relative to the returned item
- Returns:
- The item at the coordinates, otherwise
NULL
if no item is present
void elm_list_clear | ( | Evas_Object * | obj | ) |
Removes all list items.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
Elm_Object_Item* elm_list_first_item_get | ( | const Evas_Object * | obj | ) |
Gets the first item in the list.
This returns the first item in the list.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
- The first item, otherwise
NULL
if none are present
void elm_list_go | ( | Evas_Object * | obj | ) |
Starts the list.
- Since :
- 2.3.1
- Remarks:
- Call before running show() on the list object.
- If not called, it won't display the list properly.
li = elm_list_add(win); elm_list_item_append(li, "First", NULL, NULL, NULL, NULL); elm_list_item_append(li, "Second", NULL, NULL, NULL, NULL); elm_list_go(li); evas_object_show(li);
- Parameters:
-
[in] obj The list object
Eina_Bool elm_list_horizontal_get | ( | const Evas_Object * | obj | ) |
Gets a value that indicates whether the horizontal mode is enabled.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
EINA_TRUE
means horizontal mode selection is enabled, otherwiseEINA_FALSE
indicates it's disabled
If obj isNULL
,EINA_FALSE
is returned.
- See also:
- elm_list_horizontal_set()
void elm_list_horizontal_set | ( | Evas_Object * | obj, |
Eina_Bool | horizontal | ||
) |
Enables or disables horizontal mode on the list object.
- Since :
- 2.3.1
- Remarks:
- The vertical mode is set by default.
- Use this when the horizontal mode items are displayed on the list from left to right, instead of from top to bottom. Also, the list scrolls horizontally. Each item presents the left icon on top and the right icon, or end, at the bottom.
- Parameters:
-
[in] obj The list object [in] horizontal If EINA_TRUE
horizontal is enabled, otherwiseEINA_FALSE
to disable it, i.e., to enable the vertical mode
- See also:
- elm_list_horizontal_get()
Elm_Object_Item* elm_list_item_append | ( | Evas_Object * | obj, |
const char * | label, | ||
Evas_Object * | icon, | ||
Evas_Object * | end, | ||
Evas_Smart_Cb | func, | ||
const void * | data | ||
) |
Appends a new item to the list object.
- Since :
- 2.3.1
- Remarks:
- A new item is created and appended to the list, i.e., it is set as the last item.
- Items created with this method can be deleted with elm_object_item_del().
- Associated data can be properly freed when the item is deleted if a callback function is set with elm_object_item_del_cb_set().
-
If a function is passed as an argument, it is called every time this item is selected, i.e., the user clicks over an unselected item. If always select is enabled it calls this function every time the user clicks over an item (already selected or not). If such a function isn't needed, just passing
NULL
as func is enough. The same should be done for data.
- Parameters:
-
[in] obj The list object [in] label The label of the list item [in] icon The icon object to use for the left side of the item
An icon can be any Evas object, but usually it is an icon created with elm_icon_add().[in] end The icon object to use for the right side of the item
An icon can be any Evas object.[in] func The function to call when the item is clicked [in] data The data to associate with the item for related callbacks
- Returns:
- The created item, otherwise
NULL
on failure
void elm_list_item_bring_in | ( | Elm_Object_Item * | it | ) |
Brings in the given item to the list view.
- Since :
- 2.3.1
- Remarks:
- This causes the list to jump to the given item it and show it (by scrolling), if it is not fully visible.
- This may use animation to do so and may take a period of time.
- If animation isn't wanted, elm_list_item_show() can be used.
- Parameters:
-
[in] it The item
Elm_Object_Item* elm_list_item_insert_after | ( | Evas_Object * | obj, |
Elm_Object_Item * | after, | ||
const char * | label, | ||
Evas_Object * | icon, | ||
Evas_Object * | end, | ||
Evas_Smart_Cb | func, | ||
const void * | data | ||
) |
Inserts a new item into the list object after item after.
- Since :
- 2.3.1
- Remarks:
- A new item is created and added to the list. Its position in this list is just after item after.
- Items created with this method can be deleted with elm_object_item_del().
- Associated data can be properly freed when the item is deleted if a callback function is set with elm_object_item_del_cb_set().
-
If a function is passed as an argument, it is called every time this item is selected, i.e., the user clicks over an unselected item. If always select is enabled it calls this function every time the user clicks over an item (already selected or not). If such a function isn't needed, just passing
NULL
as func is enough. The same should be done for data.
- Parameters:
-
[in] obj The list object [in] after The list item to insert after [in] label The label of the list item [in] icon The icon object to use for the left side of the item
An icon can be any Evas object, but usually it is an icon created with elm_icon_add().[in] end The icon object to use for the right side of the item
An icon can be any Evas object.[in] func The function to call when the item is clicked [in] data The data to associate with the item for related callbacks
- Returns:
- The created item, otherwise
NULL
on failure
- See also:
- elm_list_item_append() for a simple code example.
- elm_list_select_mode_set()
- elm_object_item_del()
- elm_object_item_del_cb_set()
- elm_list_clear()
- elm_icon_add()
Elm_Object_Item* elm_list_item_insert_before | ( | Evas_Object * | obj, |
Elm_Object_Item * | before, | ||
const char * | label, | ||
Evas_Object * | icon, | ||
Evas_Object * | end, | ||
Evas_Smart_Cb | func, | ||
const void * | data | ||
) |
Inserts a new item into the list object before item before.
- Since :
- 2.3.1
- Remarks:
- A new item is created and added to the list. Its position in this list is just before item before.
- Items created with this method can be deleted with elm_object_item_del().
- Associated data can be properly freed when the item is deleted if a callback function is set with elm_object_item_del_cb_set().
-
If a function is passed as an argument, it is called every time this item is selected, i.e., the user clicks over an unselected item. If always select is enabled it calls this function every time the user clicks over an item (already selected or not). If such a function isn't needed, just passing
NULL
as func is enough. The same should be done for data.
- Parameters:
-
[in] obj The list object [in] before The list item to insert before [in] label The label of the list item [in] icon The icon object to use for the left side of the item
An icon can be any Evas object, but usually it is an icon created with elm_icon_add().[in] end The icon object to use for the right side of the item
An icon can be any Evas object.[in] func The function to call when the item is clicked [in] data The data to associate with the item for related callbacks
- Returns:
- The created item, otherwise
NULL
on failure
- See also:
- elm_list_item_append() for a simple code example.
- elm_list_select_mode_set()
- elm_object_item_del()
- elm_object_item_del_cb_set()
- elm_list_clear()
- elm_icon_add()
Elm_Object_Item* elm_list_item_next | ( | const Elm_Object_Item * | it | ) |
Gets the item after it in list.
- Since :
- 2.3.1
- Remarks:
- If it is the last item,
NULL
is returned.
- Parameters:
-
[in] it The list item
- Returns:
- The item after it, otherwise
NULL
if none are present or on failure
Evas_Object* elm_list_item_object_get | ( | const Elm_Object_Item * | it | ) |
Gets the base object of the item.
- Since :
- 2.3.1
- Remarks:
- The base object is the
Evas_Object
that represents that item.
- Parameters:
-
[in] it The list item
- Returns:
- The base object associated with it
Elm_Object_Item* elm_list_item_prepend | ( | Evas_Object * | obj, |
const char * | label, | ||
Evas_Object * | icon, | ||
Evas_Object * | end, | ||
Evas_Smart_Cb | func, | ||
const void * | data | ||
) |
Prepends a new item to the list object.
- Since :
- 2.3.1
- Remarks:
- A new item is created and prepended to the list, i.e., it is set as the first item.
- Items created with this method can be deleted with elm_object_item_del().
- Associated data can be properly freed when the item is deleted if a callback function is set with elm_object_item_del_cb_set().
-
If a function is passed as an argument, it is called every time this item is selected, i.e., the user clicks over an unselected item. If always select is enabled it calls this function every time the user clicks over an item (already selected or not). If such a function isn't needed, just passing
NULL
as func is enough. The same should be done for data.
- Parameters:
-
[in] obj The list object [in] label The label of the list item [in] icon The icon object to use for the left side of the item
An icon can be any Evas object, but usually it is an icon created with elm_icon_add().[in] end The icon object to use for the right side of the item
An icon can be any Evas object.[in] func The function to call when the item is clicked [in] data The data to associate with the item for related callbacks
- Returns:
- The created item, otherwise
NULL
on failure
- See also:
- elm_list_item_append() for a simple code example.
- elm_list_select_mode_set()
- elm_object_item_del()
- elm_object_item_del_cb_set()
- elm_list_clear()
- elm_icon_add()
Elm_Object_Item* elm_list_item_prev | ( | const Elm_Object_Item * | it | ) |
Gets the item before it in list.
- Since :
- 2.3.1
- Remarks:
- If it is the first item,
NULL
is returned.
- Parameters:
-
it The list item
- Returns:
- The item before it, otherwise
NULL
if none are present or on failure
Eina_Bool elm_list_item_selected_get | ( | const Elm_Object_Item * | it | ) |
Gets whether the it is selected.
- Since :
- 2.3.1
- Parameters:
-
[in] it The list item
- Returns:
EINA_TRUE
indicates that the item is selected, otherwiseEINA_FALSE
indicates it's not
If obj isNULL
,EINA_FALSE
is returned.
- See also:
- elm_list_selected_item_set()
- elm_list_item_selected_get()
void elm_list_item_selected_set | ( | Elm_Object_Item * | it, |
Eina_Bool | selected | ||
) |
Sets the selected state of an item.
- Since :
- 2.3.1
- Remarks:
- This sets the selected state of the given item it.
EINA_TRUE
for selected,EINA_FALSE
for not selected. - If a new item is selected the previously selected is unselected, unless multiple selection is enabled with elm_list_multi_select_set(). Previously selected item can be obtained with function elm_list_selected_item_get().
- Selected items are highlighted.
- Parameters:
-
[in] it The list item [in] selected The selected state
Eina_Bool elm_list_item_separator_get | ( | const Elm_Object_Item * | it | ) |
Gets a value that indicates whether an item is a separator.
- Since :
- 2.3.1
- Parameters:
-
[in] it The list item
- Returns:
EINA_TRUE
indicates that the item it is a separator, otherwiseEINA_FALSE
indicates it's not
If it isNULL
,EINA_FALSE
is returned.
- See also:
- elm_list_item_separator_set()
void elm_list_item_separator_set | ( | Elm_Object_Item * | it, |
Eina_Bool | setting | ||
) |
Sets or unsets an item as a separator.
- Since :
- 2.3.1
- Remarks:
- Items aren't set as a separator by default.
- If set as a separator it displays the separator theme, so it won't display icons or labels.
- Parameters:
-
[in] it The list item [in] setting If EINA_TRUE
it sets the item it as a separator, otherwiseEINA_FALSE
to unset it, i.e., item is used as a regular item
- See also:
- elm_list_item_separator_get()
void elm_list_item_show | ( | Elm_Object_Item * | it | ) |
Shows it in the list view.
- Since :
- 2.3.1
- Remarks:
- It won't animate the list until an item is visible. If such a behavior is wanted, use elm_list_bring_in() instead.
- Parameters:
-
[in] it The list item to be shown
Elm_Object_Item* elm_list_item_sorted_insert | ( | Evas_Object * | obj, |
const char * | label, | ||
Evas_Object * | icon, | ||
Evas_Object * | end, | ||
Evas_Smart_Cb | func, | ||
const void * | data, | ||
Eina_Compare_Cb | cmp_func | ||
) |
Inserts a new item into the sorted list object.
This function inserts values into a list object assuming it is sorted and the result is going to be sorted.
- Since :
- 2.3.1
- Remarks:
- A new item is created and added to the list. Its position in this list is found by comparing the new item with previously inserted items using function cmp_func.
- Items created with this method can be deleted with elm_object_item_del().
- Associated data can be properly freed when the item is deleted if a callback function is set with elm_object_item_del_cb_set().
-
If a function is passed as an argument, it is called every time this item is selected, i.e., the user clicks over an unselected item. If always select is enabled it calls this function every time the user clicks over an item (already selected or not). If such a function isn't needed, just passing
NULL
as func is enough. The same should be done for data.
- Parameters:
-
[in] obj The list object [in] label The label of the list item [in] icon The icon object to use for the left side of the item
An icon can be any Evas object, but usually it is an icon created with elm_icon_add().[in] end The icon object to use for the right side of the item
An icon can be any Evas object.[in] func The function to call when the item is clicked [in] data The data to associate with the item for related callbacks [in] cmp_func The comparing function to be used to sort list items by Elm_Object_Item item handles
This function receives two items and compares them, returning a non-negative integer if the second item should be placed after the first, or a negative value if it should be placed before.
- Returns:
- The created item, otherwise
NULL
on failure
- See also:
- elm_list_item_append() for a simple code example.
- elm_list_select_mode_set()
- elm_object_item_del()
- elm_object_item_del_cb_set()
- elm_list_clear()
- elm_icon_add()
const Eina_List* elm_list_items_get | ( | const Evas_Object * | obj | ) |
Gets a list of all the list items.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
- An
Eina_List
of the list items, Elm_Object_Item, otherwiseNULL
on failure
Elm_Object_Item* elm_list_last_item_get | ( | const Evas_Object * | obj | ) |
Gets the last item in the list.
This returns the last item in the list.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
- The last item, otherwise
NULL
if none are present
Elm_List_Mode elm_list_mode_get | ( | const Evas_Object * | obj | ) |
Gets the mode that the list is at.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
- One of Elm_List_Mode: ELM_LIST_COMPRESS, ELM_LIST_SCROLL, ELM_LIST_LIMIT, ELM_LIST_EXPAND, or ELM_LIST_LAST on errors
- See also:
- elm_list_mode_set()
void elm_list_mode_set | ( | Evas_Object * | obj, |
Elm_List_Mode | mode | ||
) |
Sets which mode to use for the list object.
This sets the list's resize behavior, transverse axis scroll and items cropping. See each mode's description for more details.
- Since :
- 2.3.1
- Remarks:
- This default value is ELM_LIST_SCROLL.
- Only one mode at a time can be set. If a previous one is set, it is changed by the new mode after this call. Bitmasks won't work here.
- This function's behavior clashes with those of elm_scroller_content_min_limit(), so use either one of them, but not both.
- Parameters:
-
[in] obj The list object [in] mode One of Elm_List_Mode: ELM_LIST_COMPRESS, ELM_LIST_SCROLL, ELM_LIST_LIMIT, or ELM_LIST_EXPAND.
- See also:
- elm_list_mode_get()
Eina_Bool elm_list_multi_select_get | ( | const Evas_Object * | obj | ) |
Gets a value that indicates whether multiple items selection is enabled.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
EINA_TRUE
indicates that multiple items selection is enabled, otherwiseEINA_FALSE
indicates it's disabled
If obj isNULL
,EINA_FALSE
is returned.
- See also:
- elm_list_multi_select_set()
void elm_list_multi_select_set | ( | Evas_Object * | obj, |
Eina_Bool | multi | ||
) |
Enables or disables multiple items selection on the list object.
- Since :
- 2.3.1
- Remarks:
- It is disabled by default. If disabled, the user can select a single item from the list each time. Selected items are highlighted on the list. If enabled, many items can be selected.
- If a selected item is selected again, it is unselected.
- Parameters:
-
[in] obj The list object [in] multi If EINA_TRUE
multi selection is enabled, otherwiseEINA_FALSE
to disable it
- See also:
- elm_list_multi_select_get()
Elm_Object_Select_Mode elm_list_select_mode_get | ( | const Evas_Object * | obj | ) |
Gets the list select mode.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The list object
- Returns:
- The select mode (If the getting mode is fails, it returns
ELM_OBJECT_SELECT_MODE_MAX
)
- See also:
- elm_list_select_mode_set()
void elm_list_select_mode_set | ( | Evas_Object * | obj, |
Elm_Object_Select_Mode | mode | ||
) |
Sets the list select mode.
- Since :
- 2.3.1
- Remarks:
- elm_list_select_mode_set() changes the item select mode in the list widget.
- ELM_OBJECT_SELECT_MODE_DEFAULT : Items only call their selection func and callback when first becoming selected. Any further clicks do nothing, unless you set the always select mode.
- ELM_OBJECT_SELECT_MODE_ALWAYS : This means that, even if selected, every click calls the selected callbacks.
- ELM_OBJECT_SELECT_MODE_NONE : This turns off the ability to select items entirely and they neither appear selected nor call selected callback functions.
- Parameters:
-
[in] obj The list object [in] mode The select mode
- See also:
- elm_list_select_mode_get()
Elm_Object_Item* elm_list_selected_item_get | ( | const Evas_Object * | obj | ) |
Gets the selected item.
- Since :
- 2.3.1
- Remarks:
- The selected item can be unselected with function elm_list_item_selected_set().
- The selected item is always highlighted on the list.
- Parameters:
-
[in] obj The list object
- Returns:
- The selected list item
- See also:
- elm_list_selected_items_get()
const Eina_List* elm_list_selected_items_get | ( | const Evas_Object * | obj | ) |
Returns a list of the currently selected list items.
- Since :
- 2.3.1
- Remarks:
- Multiple items can be selected if multi select is enabled. It can be done with elm_list_multi_select_set().
- Parameters:
-
[in] obj The list object
- Returns:
- An
Eina_List
of list items, Elm_Object_Item, otherwiseNULL
on failure