Tizen Native API
|
This is a container widget that takes a standard Edje design file and wraps it very thinly in a widget.
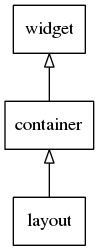
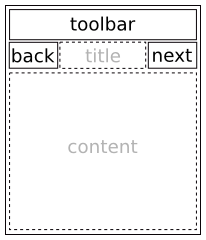
A Layout is a direct realization of elm-layout-class.
An Edje design (theme) file has a very wide range of possibilities to describe the behavior of elements added to the Layout. Check out the Edje documentation and the EDC reference to get more information about what can be done with Edje.
Just like List, Box, and other container widgets, any object added to the Layout becomes its child, meaning that it is deleted if the Layout is deleted, moved if the Layout is moved, and so on.
The Layout widget can contain as many Contents, Boxes, or Tables as described in its theme file. For instance, objects can be added to different Tables by specifying the respective Table part names. The same is valid for Contents and Boxes.
The objects added as the child of the Layout behave as described in the part description where they were added. There are 3 possible types of parts where a child can be added:
Content (SWALLOW part)
Only one object can be added to the SWALLOW
part (but you still can have many SWALLOW
parts and one object on each of them). Use the elm_object_content_set/get/unset functions to set, retrieve, and unset objects as content of the SWALLOW
. After being set to this part, the object size, position, visibility, clipping, and other description properties are totally controlled by the description of the given part (inside the Edje theme file).
One can use evas_object_size_hint_*
functions on the child to have some kind of control over its behavior, but the resulting behavior still depends heavily on the SWALLOW
part description.
The Edje theme also can change the part description, based on signals or scripts running inside the theme. This change can also be animated. All of this affects the child object set as the content accordingly. The object size changes if the part size is changed, it animates move if the part is moving, and so on.
The following picture demonstrates a Layout widget with a child object added to its SWALLOW:
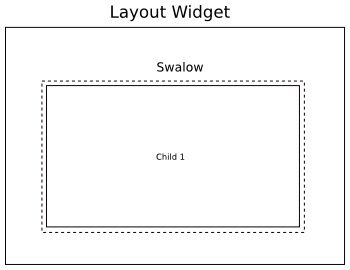
Box (BOX part)
An Edje BOX
part is very similar to the Elementary Box widget. It allows one to add objects to the box and have them distributed along its area, accordingly as per the specified layout property (now by layout we mean the chosen layouting design of the Box, not the Layout widget itself).
A similar effect for having a box with its position, size, and other things controlled by the Layout theme would be to create an Elementary Box widget and add it as Content in the SWALLOW
part.
The main difference of using the Layout Box is that its behavior, the box properties like layouting format, padding, align, etc. is all controlled by the theme. This means, for example, that a signal could be sent to the Layout theme (with elm_object_signal_emit()) and the theme handles the signal by changing the box padding, or alignment, or both. Using the Elementary Box widget is not necessarily harder or easier, it just depends on the circumstances and requirements.
The Layout Box can be used through the elm_layout_box_*
set of functions.
The following picture demonstrates a Layout widget with many child objects added to its BOX
part:
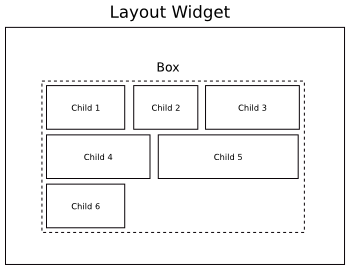
Table (TABLE part)
Just like the Box (BOX part), the Layout Table is very similar to the Elementary Table widget. It allows one to add objects to the Table specifying the row and column where the object should be added, and any column or row span if necessary.
Again, we could have this design by adding a Table widget to the SWALLOW
part using elm_object_part_content_set(). The same difference happens here when choosing to use the Layout Table (a TABLE
part) instead of the Table plus SWALLOW
part. It's just a matter of convenience.
The Layout Table can be used through the elm_layout_table_*
set of functions.
The following picture demonstrates a Layout widget with many child objects added to its TABLE
part:
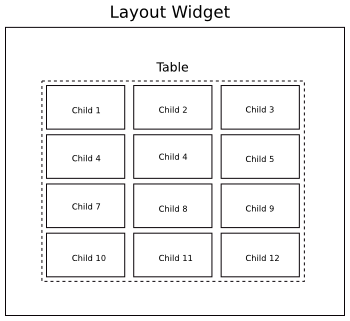
Predefined Layouts
Another interesting thing about the Layout widget is that it offers some predefined themes that come with the default Elementary theme. These themes can be set by elm_layout_theme_set(), and can provide some basic functionality depending on the theme used.
Most of them already send some signals, some already provide a toolbar or back and next buttons.
These are available, predefined theme layouts. All of them have class = layout
, group = application
, and style = one of the following options:
toolbar-content
- Application with a toolbar and main content area.toolbar-content-back
- Application with a toolbar and main content area having a back button and title area.toolbar-content-back-next
- Application with a toolbar and main content area having back and next buttons and a title area.content-back
- Application with a main content area having a back button and title area.content-back-next
- Application with a main content area having back and next buttons and a title area.toolbar-vbox
- Application with a toolbar and main content area as a vertical box.toolbar-table
- Application with a toolbar and main content area as a table.
Supported common elm_object APIs.
- elm_object_signal_emit
- elm_object_signal_callback_add
- elm_object_signal_callback_del
- elm_object_part_text_set
- elm_object_part_text_get
- elm_object_part_content_set
- elm_object_part_content_get
- elm_object_part_content_unset
Emitted signals
This widget emits the following signals:
- "theme,changed" - The theme is changed.
- "language,changed" - The program's language is changed.
Functions | |
Evas_Object * | elm_layout_add (Evas_Object *parent) |
Adds a new layout to the parent. | |
Eina_Bool | elm_layout_file_set (Evas_Object *obj, const char *file, const char *group) |
Sets the file that is used as a layout. | |
int | elm_layout_freeze (Evas_Object *obj) |
Freezes the Elementary layout object. | |
int | elm_layout_thaw (Evas_Object *obj) |
Thaws the Elementary object. | |
Eina_Bool | elm_layout_theme_set (Evas_Object *obj, const char *clas, const char *group, const char *style) |
Sets the edje group from the elementary theme that is used as a layout. | |
void | elm_layout_signal_emit (Evas_Object *obj, const char *emission, const char *source) |
Sends a (Edje) signal to a given layout widget's underlying Edje object. | |
void | elm_layout_signal_callback_add (Evas_Object *obj, const char *emission, const char *source, Edje_Signal_Cb func, void *data) |
Adds a callback for a (Edje) signal emitted by a layout widget's underlying Edje object. | |
void * | elm_layout_signal_callback_del (Evas_Object *obj, const char *emission, const char *source, Edje_Signal_Cb func) |
Removes a signal-triggered callback from a given layout widget. | |
Eina_Bool | elm_layout_box_append (Evas_Object *obj, const char *part, Evas_Object *child) |
Appends a child to the layout box part. | |
Eina_Bool | elm_layout_box_prepend (Evas_Object *obj, const char *part, Evas_Object *child) |
Prepends a child to the layout box part. | |
Eina_Bool | elm_layout_box_insert_before (Evas_Object *obj, const char *part, Evas_Object *child, const Evas_Object *reference) |
Inserts a child to the layout box part before a reference object. | |
Eina_Bool | elm_layout_box_insert_at (Evas_Object *obj, const char *part, Evas_Object *child, unsigned int pos) |
Inserts a child to the layout box part at a given position. | |
Evas_Object * | elm_layout_box_remove (Evas_Object *obj, const char *part, Evas_Object *child) |
Removes a child of the given part box. | |
Eina_Bool | elm_layout_box_remove_all (Evas_Object *obj, const char *part, Eina_Bool clear) |
Removes all children of the given part box. | |
Eina_Bool | elm_layout_table_pack (Evas_Object *obj, const char *part, Evas_Object *child_obj, unsigned short col, unsigned short row, unsigned short colspan, unsigned short rowspan) |
Inserts a child to the layout table part. | |
Evas_Object * | elm_layout_table_unpack (Evas_Object *obj, const char *part, Evas_Object *child_obj) |
Unpacks (remove) a child of the given part table. | |
Eina_Bool | elm_layout_table_clear (Evas_Object *obj, const char *part, Eina_Bool clear) |
Removes all the child objects of the given part table. | |
Evas_Object * | elm_layout_edje_get (const Evas_Object *obj) |
Gets the edje layout. | |
const char * | elm_layout_data_get (const Evas_Object *obj, const char *key) |
Gets the edje data from the given layout. | |
void | elm_layout_sizing_eval (Evas_Object *obj) |
Re-evaluates Eval sizing. | |
Eina_Bool | elm_layout_part_cursor_set (Evas_Object *obj, const char *part_name, const char *cursor) |
Sets a specific cursor for an edje part. | |
const char * | elm_layout_part_cursor_get (const Evas_Object *obj, const char *part_name) |
Gets the cursor to be shown when the mouse is over an edje part. | |
Eina_Bool | elm_layout_part_cursor_unset (Evas_Object *obj, const char *part_name) |
Unsets a cursor previously set with elm_layout_part_cursor_set(). | |
Eina_Bool | elm_layout_part_cursor_style_set (Evas_Object *obj, const char *part_name, const char *style) |
Sets a specific cursor style for an edje part. | |
const char * | elm_layout_part_cursor_style_get (const Evas_Object *obj, const char *part_name) |
Gets a specific cursor style for an edje part. | |
Eina_Bool | elm_layout_part_cursor_engine_only_set (Evas_Object *obj, const char *part_name, Eina_Bool engine_only) |
Sets whether the cursor set should be searched on the theme or should use what is provided by the engine, only. | |
Eina_Bool | elm_layout_edje_object_can_access_set (Evas_Object *obj, Eina_Bool can_access) |
Sets accessibility to all texblock(text) parts in the layout object. | |
Eina_Bool | elm_layout_edje_object_can_access_get (Evas_Object *obj) |
Gets the accessibility state of the texblock(text) parts in the layout object. | |
Eina_Bool | elm_layout_part_cursor_engine_only_get (const Evas_Object *obj, const char *part_name) |
Gets a specific cursor engine_only for an edje part. | |
Eina_Bool | elm_layout_content_set (Evas_Object *obj, const char *swallow, Evas_Object *content) |
Sets the layout content. | |
Evas_Object * | elm_layout_content_get (const Evas_Object *obj, const char *swallow) |
Gets the child object in the given content part. | |
Evas_Object * | elm_layout_content_unset (Evas_Object *obj, const char *swallow) |
Unsets the layout content. | |
Eina_Bool | elm_layout_text_set (Evas_Object *obj, const char *part, const char *text) |
Sets the text of the given part. | |
const char * | elm_layout_text_get (const Evas_Object *obj, const char *part) |
Gets the text set in the given part. | |
Defines | |
#define | elm_layout_icon_set(_ly, _obj) |
Convenience macro to set the icon object in a layout that follows the Elementary naming convention for its parts. | |
#define | elm_layout_icon_get(_ly) elm_object_part_content_get((_ly), "elm.swallow.icon") |
Convenience macro to get the icon object from a layout that follows the Elementary naming convention for its parts. | |
#define | elm_layout_end_set(_ly, _obj) |
Convenience macro to set the end object in a layout that follows the Elementary naming convention for its parts. | |
#define | elm_layout_end_get(_ly) elm_object_part_content_get((_ly), "elm.swallow.end") |
Convenience macro to get the end object in a layout that follows the Elementary naming convention for its parts. |
Define Documentation
#define elm_layout_end_get | ( | _ly | ) | elm_object_part_content_get((_ly), "elm.swallow.end") |
Convenience macro to get the end object in a layout that follows the Elementary naming convention for its parts.
- Since :
- 2.3.1
#define elm_layout_end_set | ( | _ly, | |
_obj | |||
) |
do { \ const char *sig; \ elm_object_part_content_set((_ly), "elm.swallow.end", (_obj)); \ if ((_obj)) sig = "elm,state,end,visible"; \ else sig = "elm,state,end,hidden"; \ elm_object_signal_emit((_ly), sig, "elm"); \ } while (0)
Convenience macro to set the end object in a layout that follows the Elementary naming convention for its parts.
- Since :
- 2.3.1
#define elm_layout_icon_get | ( | _ly | ) | elm_object_part_content_get((_ly), "elm.swallow.icon") |
Convenience macro to get the icon object from a layout that follows the Elementary naming convention for its parts.
- Since :
- 2.3.1
#define elm_layout_icon_set | ( | _ly, | |
_obj | |||
) |
do { \ const char *sig; \ elm_object_part_content_set((_ly), "elm.swallow.icon", (_obj)); \ if ((_obj)) sig = "elm,state,icon,visible"; \ else sig = "elm,state,icon,hidden"; \ elm_object_signal_emit((_ly), sig, "elm"); \ } while (0)
Convenience macro to set the icon object in a layout that follows the Elementary naming convention for its parts.
- Since :
- 2.3.1
Function Documentation
Evas_Object* elm_layout_add | ( | Evas_Object * | parent | ) |
Adds a new layout to the parent.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object, otherwise
NULL
if it cannot be created
Eina_Bool elm_layout_box_append | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child | ||
) |
Appends a child to the layout box part.
- Since :
- 2.3.1
- Remarks:
- Once the object is appended, it becomes a child of the layout. Its lifetime is bound to the layout, whenever the layout dies the child is deleted automatically. One should use elm_layout_box_remove() to make this layout forget about the object.
- Parameters:
-
[in] obj The layout object [in] part The box part to which the object is appended [in] child The child object to append to the box
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Eina_Bool elm_layout_box_insert_at | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child, | ||
unsigned int | pos | ||
) |
Inserts a child to the layout box part at a given position.
- Since :
- 2.3.1
- Remarks:
- Once the object is inserted, it becomes the child of the layout. Its lifetime is bound to the layout, whenever the layout dies the child is deleted automatically. One should use elm_layout_box_remove() to make this layout forget about the object.
- Parameters:
-
[in] obj The layout object [in] part The box part to insert [in] child The child object to insert into the box [in] pos The numeric position >=0 to insert the child
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Eina_Bool elm_layout_box_insert_before | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child, | ||
const Evas_Object * | reference | ||
) |
Inserts a child to the layout box part before a reference object.
- Since :
- 2.3.1
- Remarks:
- Once the object is inserted, it becomes the child of the layout. Its lifetime is bound to the layout, whenever the layout dies the child is deleted automatically. One should use elm_layout_box_remove() to make this layout forget about the object.
- Parameters:
-
[in] obj The layout object [in] part The box part to insert [in] child The child object to insert into the box [in] reference Another reference object to insert before in the box
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Eina_Bool elm_layout_box_prepend | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child | ||
) |
Prepends a child to the layout box part.
- Since :
- 2.3.1
- Remarks:
- Once the object is prepended, it becomes the child of the layout. Its lifetime is bound to the layout, whenever the layout dies the child is deleted automatically. One should use elm_layout_box_remove() to make this layout forget about the object.
- Parameters:
-
[in] obj The layout object [in] part The box part to prepend [in] child The child object to prepend to the box
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Evas_Object* elm_layout_box_remove | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child | ||
) |
Removes a child of the given part box.
- Since :
- 2.3.1
- Remarks:
- The object is removed from the box part and its lifetime is not handled by the layout anymore. This is equivalent to elm_object_part_content_unset() for the box.
- Parameters:
-
[in] obj The layout object [in] part The box part name from which to remove the child [in] child The object to remove from the box
- Returns:
- The object that is being used, otherwise
NULL
if not found
Eina_Bool elm_layout_box_remove_all | ( | Evas_Object * | obj, |
const char * | part, | ||
Eina_Bool | clear | ||
) |
Removes all children of the given part box.
- Since :
- 2.3.1
- Remarks:
- The objects are removed from the box part and their lifetime is not handled by the layout anymore. This is equivalent to elm_layout_box_remove() for all box children.
- Parameters:
-
[in] obj The layout object [in] part The box part name from which to remove the child [in] clear If EINA_TRUE
all objects are deleted as well, otherwiseEINA_FALSE
if they are just removed and are dangling on the canvas
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Evas_Object* elm_layout_content_get | ( | const Evas_Object * | obj, |
const char * | swallow | ||
) |
Gets the child object in the given content part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] swallow The SWALLOW part to get its content
- Returns:
- The swallowed object, otherwise
NULL
if none are present or an error occurs
Eina_Bool elm_layout_content_set | ( | Evas_Object * | obj, |
const char * | swallow, | ||
Evas_Object * | content | ||
) |
Sets the layout content.
- Since :
- 2.3.1
- Remarks:
- Once the content object is set, a previously set one is deleted. If you want to keep that old content object, use the elm_object_part_content_unset() function.
-
In an Edje theme, the part used as a content container is called
SWALLOW
. This is why the parameter name is called swallow, but it is expected to be a part name just like the second parameter of elm_layout_box_append().
- Parameters:
-
[in] obj The layout object [in] swallow The swallow part name in the edje file [in] content The child that is added in this layout object
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Evas_Object* elm_layout_content_unset | ( | Evas_Object * | obj, |
const char * | swallow | ||
) |
Unsets the layout content.
- Since :
- 2.3.1
- Remarks:
- Unparent and return the content object which is set for this part.
- Parameters:
-
[in] obj The layout object [in] swallow The swallow part name in the edje file
- Returns:
- The content that is being used
const char* elm_layout_data_get | ( | const Evas_Object * | obj, |
const char * | key | ||
) |
Gets the edje data from the given layout.
This function fetches the data specified inside the edje theme of this layout. This function returns NULL
if data is not found.
- Since :
- 2.3.1
- Remarks:
- In EDC this comes from a data block within the group block that obj is loaded from. E.g.
collections { group { name: "a_group"; data { item: "key1" "value1"; item: "key2" "value2"; } } }
- Parameters:
-
[in] obj The layout object [in] key The data key
- Returns:
- The edje data string
Evas_Object* elm_layout_edje_get | ( | const Evas_Object * | obj | ) |
Gets the edje layout.
- Since :
- 2.3.1
- Remarks:
- This returns the edje object. It is not expected to be used to then swallow objects via edje_object_part_swallow() for example. Use elm_object_part_content_set() instead so child object handling and sizing is done properly.
- This function should only be used if you really need to call some low level Edje function on this edje object. All the common stuff (setting text, emitting signals, hooking callbacks to signals, etc.) can be done with proper elementary functions.
- Parameters:
-
[in] obj The layout object
- Returns:
- An Evas_Object with the edje layout settings loaded using function elm_layout_file_set
Gets the accessibility state of the texblock(text) parts in the layout object.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object
- Returns:
EINA_TRUE
, if all textblock(text) parts in the layout can be accessible, otherwiseEINA_FALSE
if those cannot be accessible
If obj is not a proper layout object,EINA_FALSE
is returned.
- See also:
- elm_layout_edje_object_access_set()
Eina_Bool elm_layout_edje_object_can_access_set | ( | Evas_Object * | obj, |
Eina_Bool | can_access | ||
) |
Sets accessibility to all texblock(text) parts in the layout object.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] can_access It makes all textblock(text) parts in the layout obj possible to have accessibility
EINA_TRUE
means textblock(text) parts can be accessible.
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
If obj is not a proper layout object,EINA_FALSE
is returned.
Eina_Bool elm_layout_file_set | ( | Evas_Object * | obj, |
const char * | file, | ||
const char * | group | ||
) |
Sets the file that is used as a layout.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] file The path to the file (edj) that is used as a layout [in] group The group that the layout belongs to in the edje file
- Returns:
- (
1
= success,0
= error)
int elm_layout_freeze | ( | Evas_Object * | obj | ) |
Freezes the Elementary layout object.
This function puts all changes on hold. Successive freezes are nested, requiring an equal number of thaws.
- Since :
- 2.3.1
- Parameters:
-
[in] obj A handle to an Elementary layout object
- Returns:
- The frozen state, otherwise
0
on error
- See also:
- elm_layout_thaw()
Eina_Bool elm_layout_part_cursor_engine_only_get | ( | const Evas_Object * | obj, |
const char * | part_name | ||
) |
Gets a specific cursor engine_only for an edje part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group
- Returns:
- Whenever the cursor is just provided by the engine or also from the theme
Eina_Bool elm_layout_part_cursor_engine_only_set | ( | Evas_Object * | obj, |
const char * | part_name, | ||
Eina_Bool | engine_only | ||
) |
Sets whether the cursor set should be searched on the theme or should use what is provided by the engine, only.
- Since :
- 2.3.1
- Remarks:
- Before you set its look on the theme you should define a cursor with elm_layout_part_cursor_set(). By default it only looks for cursors provided by the engine.
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group [in] engine_only If cursors should be just provided by the engine ( EINA_TRUE
), otherwise should also search on the widget's theme as well (EINA_FALSE
)
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure, that may be a part not existing or it may not had a cursor set
const char* elm_layout_part_cursor_get | ( | const Evas_Object * | obj, |
const char * | part_name | ||
) |
Gets the cursor to be shown when the mouse is over an edje part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group
- Returns:
- The cursor name
Eina_Bool elm_layout_part_cursor_set | ( | Evas_Object * | obj, |
const char * | part_name, | ||
const char * | cursor | ||
) |
Sets a specific cursor for an edje part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group [in] cursor The cursor name to use, see Elementary_Cursor.h
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure, that may be a part not existing or it may have "mouse_events: 0"
const char* elm_layout_part_cursor_style_get | ( | const Evas_Object * | obj, |
const char * | part_name | ||
) |
Gets a specific cursor style for an edje part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group
- Returns:
- The theme style in use, defaults to "default"
If the object does not have a cursor set, thenNULL
is returned
Eina_Bool elm_layout_part_cursor_style_set | ( | Evas_Object * | obj, |
const char * | part_name, | ||
const char * | style | ||
) |
Sets a specific cursor style for an edje part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group [in] style The theme style to use (default, transparent, ...)
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure, that may be a part not existing or it may not have a cursor set.
Eina_Bool elm_layout_part_cursor_unset | ( | Evas_Object * | obj, |
const char * | part_name | ||
) |
Unsets a cursor previously set with elm_layout_part_cursor_set().
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part_name The part from the loaded edje group, that had a cursor set with elm_layout_part_cursor_set()
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
void elm_layout_signal_callback_add | ( | Evas_Object * | obj, |
const char * | emission, | ||
const char * | source, | ||
Edje_Signal_Cb | func, | ||
void * | data | ||
) |
Adds a callback for a (Edje) signal emitted by a layout widget's underlying Edje object.
This function connects a callback function to a signal emitted by the underlying Edje object of obj. Globs are accepted in either the emission or source strings (see edje_object_signal_callback_add()).
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object handle [in] emission The signal's name string [in] source The signal's source string [in] func The callback function to be executed when the signal is emitted [in] data A pointer to data to pass to the callback function
void* elm_layout_signal_callback_del | ( | Evas_Object * | obj, |
const char * | emission, | ||
const char * | source, | ||
Edje_Signal_Cb | func | ||
) |
Removes a signal-triggered callback from a given layout widget.
This function removes the last callback attached to a signal emitted by the undelying Edje object of obj, with parameters emission, source, and func matching exactly those passed to a previous call to elm_object_signal_callback_add(). The data pointer that is passed to this call is returned.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object handle [in] emission The signal's name string [in] source The signal's source string [in] func The callback function being executed when the signal is emitted
- Returns:
- The data pointer of the signal callback (passed on elm_layout_signal_callback_add()), otherwise
NULL
on errors
void elm_layout_signal_emit | ( | Evas_Object * | obj, |
const char * | emission, | ||
const char * | source | ||
) |
Sends a (Edje) signal to a given layout widget's underlying Edje object.
This function sends a signal to the underlying Edje object of obj. An Edje program on that Edje object's definition can respond to a signal by specifying matching 'signal' and 'source' fields.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object handle [in] emission The signal's name string [in] source The signal's source string
void elm_layout_sizing_eval | ( | Evas_Object * | obj | ) |
Re-evaluates Eval sizing.
- Since :
- 2.3.1
- Remarks:
- Manually forces a sizing re-evaluation. This is useful when the minimum size required by the edje theme of this layout has changed. The change on the minimum size required by the edje theme is not immediately reported to the elementary layout, so one needs to call this function in order to tell the widget (layout) that it needs to re-evaluate its own size.
- The minimum size of the theme is calculated based on the minimum size of the parts, the size of the elements inside containers like box and table, etc. All of this can change due to state changes, and that's when this function should be called.
- Also note that a standard signal of "size,eval" "elm" emitted from the edje object causes this to happen too.
- Parameters:
-
[in] obj The layout object
Eina_Bool elm_layout_table_clear | ( | Evas_Object * | obj, |
const char * | part, | ||
Eina_Bool | clear | ||
) |
Removes all the child objects of the given part table.
- Since :
- 2.3.1
- Remarks:
- The objects are removed from the table part and their lifetime is not handled by the layout anymore. This is equivalent to elm_layout_table_unpack() for all table children.
- Parameters:
-
[in] obj The layout object [in] part The table part name from which to remove the child [in] clear If EINA_TRUE
all objects are deleted as well, otherwiseEINA_FALSE
if they are just removed and are dangling on the canvas
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Eina_Bool elm_layout_table_pack | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child_obj, | ||
unsigned short | col, | ||
unsigned short | row, | ||
unsigned short | colspan, | ||
unsigned short | rowspan | ||
) |
Inserts a child to the layout table part.
- Since :
- 2.3.1
- Remarks:
- Once the object is inserted, it becomes the child of the table. Its lifetime is bound to the layout, and whenever the layout dies the child is deleted automatically. One should use elm_layout_table_remove() to make this layout forget about the object.
-
If colspan or rowspan are bigger than
1
, that object occupies more space than a single cell. For instance, the following code:elm_layout_table_pack(layout, "table_part", child, 0, 1, 3, 1);
- This would result in an object being added like the following picture:
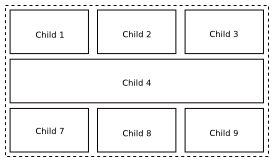
- Parameters:
-
[in] obj The layout object [in] part The box part to pack the child [in] child_obj The child object to pack into the table [in] col The column to which the child should be added (>= 0) [in] row The row to which the child should be added (>= 0) [in] colspan The number of columns that should be used to store this object (>= 1) [in] rowspan The number of rows that should be used to store this object (>= 1)
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
Evas_Object* elm_layout_table_unpack | ( | Evas_Object * | obj, |
const char * | part, | ||
Evas_Object * | child_obj | ||
) |
Unpacks (remove) a child of the given part table.
- Since :
- 2.3.1
- Remarks:
- The object is unpacked from the table part and its lifetime is not handled by the layout anymore. This is equivalent to elm_object_part_content_unset() for the table.
- Parameters:
-
[in] obj The layout object [in] part The table part name from which to remove the child [in] child_obj The object to remove from the table
- Returns:
- The object that is being used, otherwise
NULL
if no object is found
const char* elm_layout_text_get | ( | const Evas_Object * | obj, |
const char * | part | ||
) |
Gets the text set in the given part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part The TEXT part to retrieve the text from
- Returns:
- The text set in part
Eina_Bool elm_layout_text_set | ( | Evas_Object * | obj, |
const char * | part, | ||
const char * | text | ||
) |
Sets the text of the given part.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The layout object [in] part The TEXT part where to set the text [in] text The text to set
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
on failure
int elm_layout_thaw | ( | Evas_Object * | obj | ) |
Thaws the Elementary object.
This function thaws the given Edje object and the Elementary sizing calc.
- Since :
- 2.3.1
- Remarks:
- If sucessive freezes are done, an equal number of thaws are required.
- Parameters:
-
[in] obj A handle to an Elementary layout object
- Returns:
- The frozen state, otherwise
0
if the object is not frozen or on error
- See also:
- elm_layout_freeze()
Eina_Bool elm_layout_theme_set | ( | Evas_Object * | obj, |
const char * | clas, | ||
const char * | group, | ||
const char * | style | ||
) |
Sets the edje group from the elementary theme that is used as a layout.
- Since :
- 2.3.1
- Remarks:
- Note that style is the new style of obj too, as in an elm_object_style_set() call.
- Parameters:
-
[in] obj The layout object [in] clas The class of the group [in] group The group [in] style The style to use
- Returns:
- (
1
= success,0
= error)