Tizen Native API
|
The slider adds a draggable “slider” widget for selecting the value of something within a range.
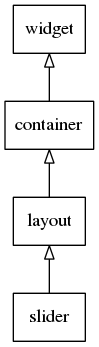
A slider can be horizontal or vertical. It can contain an Icon and can have a primary label as well as a units label (that is formatted with floating point values and thus accepts a printf-style format string, like “%1.2f units”. There is also an indicator string that may be somewhere else (like on the slider itself) that also accepts a format string like units. Label, Icon Unit, and Indicator strings/objects are optional.
A slider may be inverted, which means values invert, with high values being on the left or top and low values on the right or bottom (as opposed to normally being low on the left or top and high at the bottom and right).
The slider should have its minimum and maximum values set by the application with elm_slider_min_max_set() and values should also be set by the application before it is used with elm_slider_value_set(). The span of the slider is its length (horizontally or vertically). This is scaled by the object or applications scaling factor. At any point, the code can query the slider for its value using elm_slider_value_get().
This widget inherits from the Layout one, so that all the functions acting on it also work for slider objects.
This widget emits the following signals, besides the ones sent from Layout :
"changed"
- Whenever the slider value is changed by the user."slider,drag,start"
- Dragging the slider indicator has started."slider,drag,stop"
- Dragging the slider indicator has stopped."delay,changed"
- A short time after the value is changed by the user. This is called only when the user stops dragging for a very short period or when they release their finger/mouse, so it avoids the possible expensive reactions to the value change.
Available styles for it:
"default"
The default content parts of the slider widget that you can use are:
- "icon" - An icon of the slider.
- "end" - An end part content of the slider.
The default text parts of the slider widget that you can use are:
- "default" - Label of the slider.
Supported common elm_object APIs.
- elm_object_disabled_set
- elm_object_disabled_get
- elm_object_part_text_set
- elm_object_part_text_get
- elm_object_part_content_set
- elm_object_part_content_get
- elm_object_part_content_unset
Functions | |
Evas_Object * | elm_slider_add (Evas_Object *parent) |
Adds a new slider widget to the given parent Elementary (container) object. | |
void | elm_slider_span_size_set (Evas_Object *obj, Evas_Coord size) |
Sets the (exact) length of the bar region of a given slider widget. | |
Evas_Coord | elm_slider_span_size_get (const Evas_Object *obj) |
Gets the length set for the bar region of a given slider widget. | |
void | elm_slider_unit_format_set (Evas_Object *obj, const char *format) |
Sets the format string for the unit label. | |
const char * | elm_slider_unit_format_get (const Evas_Object *obj) |
Gets the unit label format of the slider. | |
void | elm_slider_indicator_format_set (Evas_Object *obj, const char *indicator) |
Sets the format string for the indicator label. | |
const char * | elm_slider_indicator_format_get (const Evas_Object *obj) |
Gets the indicator label format of the slider. | |
void | elm_slider_indicator_format_function_set (Evas_Object *obj, char *(*func)(double val), void(*free_func)(char *str)) |
Sets the format function pointer for the indicator label. | |
void | elm_slider_units_format_function_set (Evas_Object *obj, char *(*func)(double val), void(*free_func)(char *str)) |
Sets the format function pointer for the units label. | |
void | elm_slider_horizontal_set (Evas_Object *obj, Eina_Bool horizontal) |
Sets the orientation of a given slider widget. | |
Eina_Bool | elm_slider_horizontal_get (const Evas_Object *obj) |
Retrieves the orientation of a given slider widget. | |
void | elm_slider_min_max_set (Evas_Object *obj, double min, double max) |
Sets the minimum and maximum values for the slider. | |
void | elm_slider_min_max_get (const Evas_Object *obj, double *min, double *max) |
Gets the minimum and maximum values of the slider. | |
void | elm_slider_value_set (Evas_Object *obj, double val) |
Sets the value that the slider displays. | |
double | elm_slider_value_get (const Evas_Object *obj) |
Gets the value displayed by the spinner. | |
void | elm_slider_inverted_set (Evas_Object *obj, Eina_Bool inverted) |
Inverts a given slider widget's displaying values order. | |
Eina_Bool | elm_slider_inverted_get (const Evas_Object *obj) |
Gets whether a given slider widget's displaying values are inverted. | |
void | elm_slider_indicator_show_set (Evas_Object *obj, Eina_Bool show) |
Sets whether to enlarge the slider indicator (augmented knob). | |
Eina_Bool | elm_slider_indicator_show_get (const Evas_Object *obj) |
Gets whether a given slider widget is an enlarging indicator. | |
void | elm_slider_step_set (Evas_Object *obj, double step) |
Sets the step by which the slider indicator moves. | |
double | elm_slider_step_get (const Evas_Object *obj) |
Gets the step by which the slider indicator moves. |
Function Documentation
Evas_Object* elm_slider_add | ( | Evas_Object * | parent | ) |
Adds a new slider widget to the given parent Elementary (container) object.
This function inserts a new slider widget on the canvas.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- A new slider widget handle, otherwise
NULL
in case of an error
Eina_Bool elm_slider_horizontal_get | ( | const Evas_Object * | obj | ) |
Retrieves the orientation of a given slider widget.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The slider object
- Returns:
EINA_TRUE
, if obj is set to horizontal, otherwiseEINA_FALSE
if it's vertical (and on errors)
- See also:
- elm_slider_horizontal_set()
void elm_slider_horizontal_set | ( | Evas_Object * | obj, |
Eina_Bool | horizontal | ||
) |
Sets the orientation of a given slider widget.
- Since :
- 2.3.1
- Remarks:
- Use this function to change how your slider is to be disposed: vertically or horizontally.
- By default, it's displayed horizontally.
- Parameters:
-
[in] obj The slider object [in] horizontal If EINA_TRUE
, it makes obj horizontal, otherwiseEINA_FALSE
to make it vertical
- See also:
- elm_slider_horizontal_get()
void elm_slider_indicator_format_function_set | ( | Evas_Object * | obj, |
char *(*)(double val) | func, | ||
void(*)(char *str) | free_func | ||
) |
Sets the format function pointer for the indicator label.
This sets the callback function to format the indicator string.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The slider object [in] func The indicator format function [in] free_func The freeing function for the format string
- See also:
- elm_slider_indicator_format_set() for more info on how this works.
const char* elm_slider_indicator_format_get | ( | const Evas_Object * | obj | ) |
Gets the indicator label format of the slider.
- Since :
- 2.3.1
- Remarks:
- The slider may display its value somewhere other than the unit label, for example, above the slider knob that is dragged around. This function gets the format string used for this.
- Parameters:
-
[in] obj The slider object
- Returns:
- The indicator label format string in UTF-8
- See also:
- elm_slider_indicator_format_set() for more information on how this works.
void elm_slider_indicator_format_set | ( | Evas_Object * | obj, |
const char * | indicator | ||
) |
Sets the format string for the indicator label.
- Since :
- 2.3.1
- Remarks:
- The slider may display its value somewhere other than the unit label, for example, above the slider knob that is dragged around. This function sets the format string used for this.
-
If
NULL
, the indicator label won't be visible. If not, it sets the format string for the label text. For the label text floating point value is provided, so the label text can display up to1
floating point value. Note that this is optional. - Use a format string such as "%1.2f meters" for example, and it displays values like: "3.14 meters" for a value equal to 3.14159.
- By default, the indicator label is disabled.
- Parameters:
-
[in] obj The slider object [in] indicator The format string for the indicator display
- See also:
- elm_slider_indicator_format_get()
Eina_Bool elm_slider_indicator_show_get | ( | const Evas_Object * | obj | ) |
Gets whether a given slider widget is an enlarging indicator.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The slider object
- Returns:
EINA_TRUE
, if obj is an enlarging indicator, otherwiseEINA_FALSE
(and on errors)
- See also:
- elm_slider_indicator_show_set()
void elm_slider_indicator_show_set | ( | Evas_Object * | obj, |
Eina_Bool | show | ||
) |
Sets whether to enlarge the slider indicator (augmented knob).
- Since :
- 2.3.1
- Remarks:
- By default, the indicator is bigger when dragged by the user.
- It won't display values set with elm_slider_indicator_format_set() if you disable the indicator.
- Parameters:
-
[in] obj The slider object [in] show If EINA_TRUE
the knob is enlarged, otherwiseEINA_FALSE
always lets the knob of the default size
Eina_Bool elm_slider_inverted_get | ( | const Evas_Object * | obj | ) |
Gets whether a given slider widget's displaying values are inverted.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The slider object
- Returns:
EINA_TRUE
, if obj has inverted values, otherwiseEINA_FALSE
(and on errors)
- See also:
- elm_slider_inverted_set()
void elm_slider_inverted_set | ( | Evas_Object * | obj, |
Eina_Bool | inverted | ||
) |
Inverts a given slider widget's displaying values order.
- Since :
- 2.3.1
- Remarks:
- A slider may be inverted, in which case it gets its values inverted, with high values being on the left or top and low values on the right or bottom, as opposed to normally have the low values on the former and high values on the latter, respectively, for the horizontal and vertical modes.
- Parameters:
-
[in] obj The slider object [in] inverted If EINA_TRUE
obj is inverted, otherwiseEINA_FALSE
to bring it back to the default, non-inverted value
- See also:
- elm_slider_inverted_get()
void elm_slider_min_max_get | ( | const Evas_Object * | obj, |
double * | min, | ||
double * | max | ||
) |
Gets the minimum and maximum values of the slider.
- Since :
- 2.3.1
- Remarks:
- If only one value is needed, the other pointer can be passed as
NULL
.
- Parameters:
-
[in] obj The slider object [out] min The pointer to store the minimum value [out] max The pointer to store the maximum value
- See also:
- elm_slider_min_max_set()
void elm_slider_min_max_set | ( | Evas_Object * | obj, |
double | min, | ||
double | max | ||
) |
Sets the minimum and maximum values for the slider.
- Since :
- 2.3.1
- Remarks:
- This defines the allowed range of values to be selected by the user.
- If the actual value is less than min, it is updated to min. If it is bigger then max, it is updated to max. Actual value can be obtained with elm_slider_value_get().
-
By default, min is equal to
0.0
, and max is equal to1.0
. - Maximum must be greater than minimum, otherwise the behavior is undefined.
- Parameters:
-
[in] obj The slider object [in] min The minimum value [in] max The maximum value
- See also:
- elm_slider_min_max_get()
Evas_Coord elm_slider_span_size_get | ( | const Evas_Object * | obj | ) |
Gets the length set for the bar region of a given slider widget.
- Since :
- 2.3.1
- Remarks:
- If that size is not set previously, with elm_slider_span_size_set(), this call returns
0
.
- Parameters:
-
[in] obj The slider object
- Returns:
- The length of the slider's bar region
void elm_slider_span_size_set | ( | Evas_Object * | obj, |
Evas_Coord | size | ||
) |
Sets the (exact) length of the bar region of a given slider widget.
This sets the minimum width (when in the horizontal mode) or height (when in the vertical mode) of the actual bar area of the slider obj. This in turn affects the object's minimum size. Use this when you're not setting other size hints expanding on the given direction (like weight and alignment hints), and you would like it to have a specific size.
- Since :
- 2.3.1
- Remarks:
- Icon, end, label, indicator, and unit text around obj require their own space, which makes obj require more than size, actually.
- Parameters:
-
[in] obj The slider object [in] size The length of the slider's bar region
- See also:
- elm_slider_span_size_get()
double elm_slider_step_get | ( | const Evas_Object * | obj | ) |
Gets the step by which the slider indicator moves.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The slider object
- Returns:
- The step value
- See also:
- elm_slider_step_set()
void elm_slider_step_set | ( | Evas_Object * | obj, |
double | step | ||
) |
Sets the step by which the slider indicator moves.
- Since :
- 2.3.1
- Remarks:
- This value is used when the draggable object is moved automatically i.e., in case of a key event when up/down/left/right key is pressed or in case accessibility is set and the flick event is used to inc/dec slider values. By default, the step value is equal to
0.05
.
- Parameters:
-
[in] obj The slider object [in] step The step value
- See also:
- elm_slider_step_get()
const char* elm_slider_unit_format_get | ( | const Evas_Object * | obj | ) |
Gets the unit label format of the slider.
- Since :
- 2.3.1
- Remarks:
- Unit label is displayed all the time, if set, after the slider's bar. In the horizontal mode, on the right and in the vertical mode, at the bottom.
- Parameters:
-
[in] obj The slider object
- Returns:
- The unit label format string in UTF-8
- See also:
- elm_slider_unit_format_set() for more information on how this works.
void elm_slider_unit_format_set | ( | Evas_Object * | obj, |
const char * | format | ||
) |
Sets the format string for the unit label.
- Since :
- 2.3.1
- Remarks:
- Unit label is displayed all the time, if set, after the slider's bar. In the horizontal mode, on the right and in the vertical mode, at the bottom.
-
If
NULL
, the unit label won't be visible. If not, it sets the format string for the label text. For the label text a floating point value is provided, so the label text can display up to1
floating point value. Note that this is optional. - Use a format string such as "%1.2f meters" for example, and it displays values like: "3.14 meters" for a value equal to 3.14159.
- By default, unit label is disabled.
- Parameters:
-
[in] obj The slider object [in] format The format string for the unit display
- See also:
- elm_slider_indicator_format_get()
void elm_slider_units_format_function_set | ( | Evas_Object * | obj, |
char *(*)(double val) | func, | ||
void(*)(char *str) | free_func | ||
) |
Sets the format function pointer for the units label.
This sets the callback function to format the units string.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The slider object [in] func The units format function [in] free_func The freeing function for the format string
- See also:
- elm_slider_unit_format_set() for more info on how this works.
double elm_slider_value_get | ( | const Evas_Object * | obj | ) |
Gets the value displayed by the spinner.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
- The value displayed
- See also:
- elm_spinner_value_set()
void elm_slider_value_set | ( | Evas_Object * | obj, |
double | val | ||
) |
Sets the value that the slider displays.
- Since :
- 2.3.1
- Remarks:
- The value is presented on the unit label following the format specified with elm_slider_unit_format_set() and on the indicator with elm_slider_indicator_format_set().
- The value must be between the min and max values. These values are set by elm_slider_min_max_set().
- Parameters:
-
[in] obj The slider object [in] val The value to be displayed