Tizen Native API
|
This group provides functions for size hints.
Objects may carry hints, so that another object that acts as a manager (see Smart Object Functions) may know how to properly position and resize its subordinate objects. The Size Hints provide a common interface that is recommended as the protocol for such information.
For example, box objects use alignment hints to align its lines or columns inside its container, padding hints to set the padding between each individual child, and so on.
Functions | |
void | evas_object_size_hint_min_get (const Evas_Object *obj, Evas_Coord *w, Evas_Coord *h) |
Gets the hints for an object's minimum size. | |
void | evas_object_size_hint_min_set (Evas_Object *obj, Evas_Coord w, Evas_Coord h) |
Sets the hints for an object's minimum size. | |
void | evas_object_size_hint_max_get (const Evas_Object *obj, Evas_Coord *w, Evas_Coord *h) |
Gets the hints for an object's maximum size. | |
void | evas_object_size_hint_max_set (Evas_Object *obj, Evas_Coord w, Evas_Coord h) |
Sets the hints for an object's maximum size. | |
Evas_Display_Mode | evas_object_size_hint_display_mode_get (const Evas_Object *obj) |
Gets the hints for an object's display mode. | |
void | evas_object_size_hint_display_mode_set (Evas_Object *obj, Evas_Display_Mode dispmode) |
Sets the hints for an object's display mode. | |
void | evas_object_size_hint_aspect_get (const Evas_Object *obj, Evas_Aspect_Control *aspect, Evas_Coord *w, Evas_Coord *h) |
Gets the hints for an object's aspect ratio. | |
void | evas_object_size_hint_aspect_set (Evas_Object *obj, Evas_Aspect_Control aspect, Evas_Coord w, Evas_Coord h) |
Sets the hints for an object's aspect ratio. | |
void | evas_object_size_hint_align_get (const Evas_Object *obj, double *x, double *y) |
Gets the hints for the object's alignment. | |
void | evas_object_size_hint_align_set (Evas_Object *obj, double x, double y) |
Sets the hints for an object's alignment. | |
void | evas_object_size_hint_weight_get (const Evas_Object *obj, double *x, double *y) |
Gets the hints for an object's weight. | |
void | evas_object_size_hint_weight_set (Evas_Object *obj, double x, double y) |
Sets the hints for an object's weight. | |
void | evas_object_size_hint_padding_get (const Evas_Object *obj, Evas_Coord *l, Evas_Coord *r, Evas_Coord *t, Evas_Coord *b) |
Gets the hints for an object's padding space. | |
void | evas_object_size_hint_padding_set (Evas_Object *obj, Evas_Coord l, Evas_Coord r, Evas_Coord t, Evas_Coord b) |
Sets the hints for an object's padding space. | |
Defines | |
#define | evas_object_size_hint_fill_set evas_object_size_hint_align_set |
Convenience macro to make it easier to understand that align is also used for fill properties (as fill is mutually exclusive to align) | |
#define | evas_object_size_hint_fill_get evas_object_size_hint_align_get |
Convenience macro to make it easier to understand that align is also used for fill properties (as fill is mutually exclusive to align) | |
#define | evas_object_size_hint_expand_set evas_object_size_hint_weight_set |
Convenience macro to make it easier to understand that weight is also used for expand properties. | |
#define | evas_object_size_hint_expand_get evas_object_size_hint_weight_get |
Convenience macro to make it easier to understand that weight is also used for expand properties. |
Define Documentation
Convenience macro to make it easier to understand that weight is also used for expand properties.
- Since :
- 2.3.1
Convenience macro to make it easier to understand that weight is also used for expand properties.
- Since :
- 2.3.1
Convenience macro to make it easier to understand that align is also used for fill properties (as fill is mutually exclusive to align)
- Since :
- 2.3.1
Convenience macro to make it easier to understand that align is also used for fill properties (as fill is mutually exclusive to align)
- Since :
- 2.3.1
Function Documentation
void evas_object_size_hint_align_get | ( | const Evas_Object * | obj, |
double * | x, | ||
double * | y | ||
) |
Gets the hints for the object's alignment.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Use
NULL
pointers on the hint components that you are not interested in: they are ignored by the function. -
If
obj
is invalid, then the hint components are set with0.5
- Parameters:
-
[in] obj The given Evas object to query hints from [out] x The pointer to a double in which to store the horizontal alignment hint [out] y The pointer to a double in which to store the vertical alignment hint
- See also:
- evas_object_size_hint_align_set() for more information
void evas_object_size_hint_align_set | ( | Evas_Object * | obj, |
double | x, | ||
double | y | ||
) |
Sets the hints for an object's alignment.
- Since :
- 2.3.1
- Remarks:
- These are hints on how to align an object inside the boundaries of a container/manager. Accepted values are in the
0.0
to1.0
range, with the special value EVAS_HINT_FILL used to specify "justify" or "fill" by some users. In this case, maximum size hints should be enforced with higher priority, if they are set. Also, any padding hint set on objects should add up to the alignment space on the final scene composition. - See documentation of possible users: in Evas, they are the box and table smart objects.
-
For the horizontal component,
0.0
means to the left,1.0
means to the right. Analogously, for the vertical component,0.0
to the top,1.0
means to the bottom. - See the following figure:
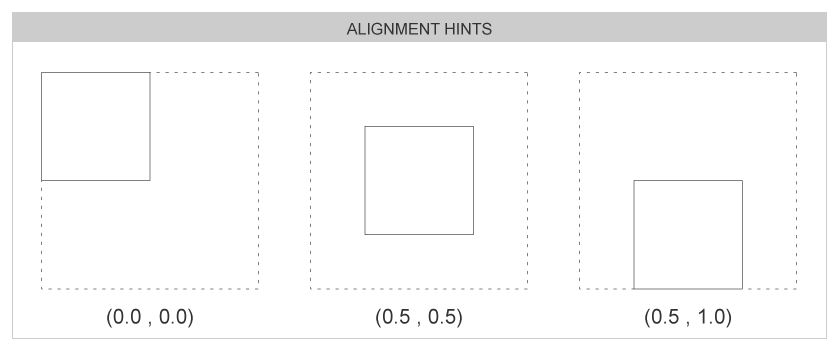
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
The default alignment hint values are
0.5
, for both axis.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] x The horizontal alignment hint as double value ranging from 0.0
to1.0
or with the special value EVAS_HINT_FILL[in] y The vertical alignment hint as double value ranging from 0.0
to1.0
or with the special value EVAS_HINT_FILL
void evas_object_size_hint_aspect_get | ( | const Evas_Object * | obj, |
Evas_Aspect_Control * | aspect, | ||
Evas_Coord * | w, | ||
Evas_Coord * | h | ||
) |
Gets the hints for an object's aspect ratio.
- Since :
- 2.3.1
- Remarks:
- The different aspect ratio policies are documented in the Evas_Aspect_Control type. A container respecting these size hints would resize its children accordingly to those policies.
-
For any policy, if any of the given aspect ratio terms are
0
, the object's container should ignore the aspect and scale obj to occupy the whole available area. If they are both positive integers, that proportion is respected, under each scaling policy. - These images illustrate some of the Evas_Aspect_Control policies:
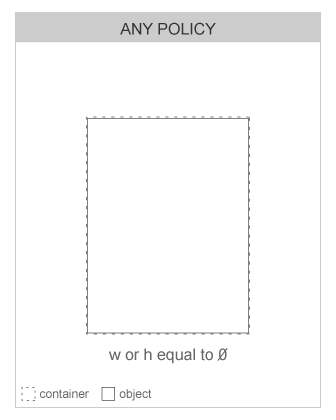
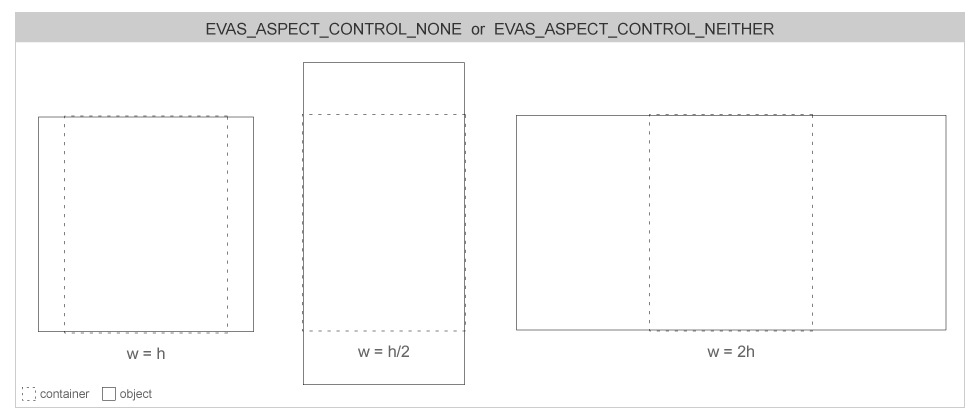
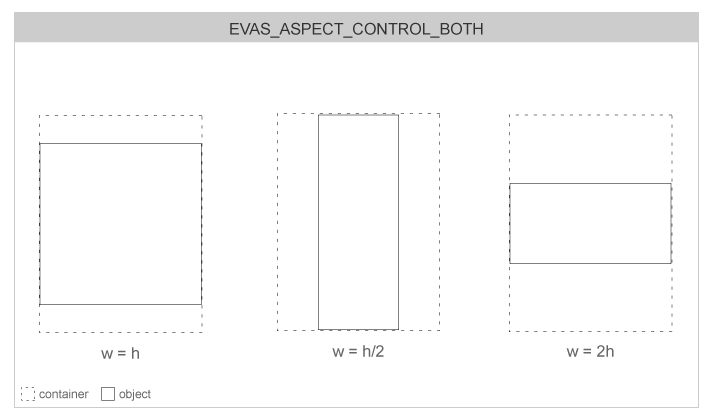
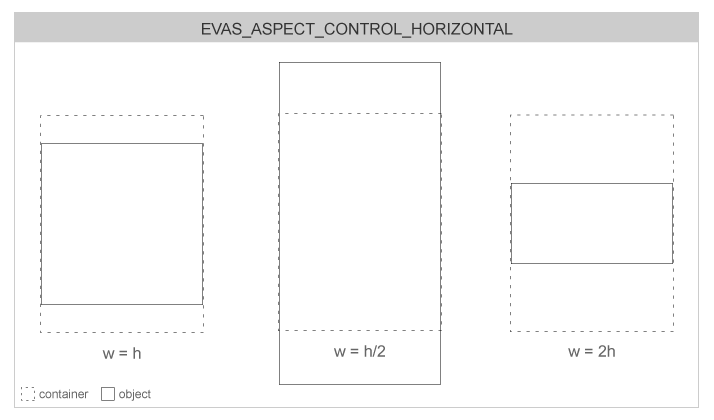
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Use
NULL
pointers on the hint components that you are not interested in: they are ignored by the function.
- Parameters:
-
[in] obj The given Evas object to query hints from [out] aspect The policy or type of aspect ratio applied to obj that is returned [out] w The pointer to an integer in which to store the aspect's width ratio term [out] h The pointer to an integer in which to store the aspect's height ratio term
- See also:
- evas_object_size_hint_aspect_set()
void evas_object_size_hint_aspect_set | ( | Evas_Object * | obj, |
Evas_Aspect_Control | aspect, | ||
Evas_Coord | w, | ||
Evas_Coord | h | ||
) |
Sets the hints for an object's aspect ratio.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
If any of the given aspect ratio terms are
0
, the object's container ignores the aspect and scale obj to occupy the whole available area, for any given policy.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] aspect The policy or type of aspect ratio to apply to obj [in] w The integer to use as aspect width ratio term [in] h The integer to use as aspect height ratio term
- See also:
- evas_object_size_hint_aspect_get() for more information.
Evas_Display_Mode evas_object_size_hint_display_mode_get | ( | const Evas_Object * | obj | ) |
Gets the hints for an object's display mode.
- Since :
- 2.3.1
- Remarks:
- These are hints on the display mode obj. This is not a size enforcement in any way. It is just a hint that can be used whenever appropriate. This mode can be used with object's display mode like compress or expand.
- Parameters:
-
[in] obj The given Evas object to query hints from
- Returns:
- The display mode hints
void evas_object_size_hint_display_mode_set | ( | Evas_Object * | obj, |
Evas_Display_Mode | dispmode | ||
) |
Sets the hints for an object's display mode.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that can be used whenever appropriate.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] dispmode The display mode hint
void evas_object_size_hint_max_get | ( | const Evas_Object * | obj, |
Evas_Coord * | w, | ||
Evas_Coord * | h | ||
) |
Gets the hints for an object's maximum size.
- Since :
- 2.3.1
- Remarks:
- These are hints on the maximum sizes obj should have. This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Use
NULL
pointers on the hint components that you are not interested in: they are ignored by the function.
- Parameters:
-
[in] obj The given Evas object to query hints from [out] w The pointer to an integer in which to store the maximum width [out] h The pointer to an integer in which to store the maximum height
- See also:
- evas_object_size_hint_max_set()
void evas_object_size_hint_max_set | ( | Evas_Object * | obj, |
Evas_Coord | w, | ||
Evas_Coord | h | ||
) |
Sets the hints for an object's maximum size.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Values like
-1
are treated as unset hint components, when queried by managers.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] w The integer to use as the maximum width hint [in] h The integer to use as the maximum height hint
- See also:
- evas_object_size_hint_max_get()
void evas_object_size_hint_min_get | ( | const Evas_Object * | obj, |
Evas_Coord * | w, | ||
Evas_Coord * | h | ||
) |
Gets the hints for an object's minimum size.
- Since :
- 2.3.1
- Remarks:
- These are hints on the minimim sizes obj should have. This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Use
NULL
pointers on the hint components that you are not interested in: they are ignored by the function.
- Parameters:
-
[in] obj The given Evas object to query hints from [out] w The pointer to an integer in which to store the minimum width [out] h The pointer to an integer in which to store the minimum height
- See also:
- evas_object_size_hint_min_set() for an example
void evas_object_size_hint_min_set | ( | Evas_Object * | obj, |
Evas_Coord | w, | ||
Evas_Coord | h | ||
) |
Sets the hints for an object's minimum size.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Values like
0
are treated as unset hint components, when queried by managers.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] w The integer to use as the minimum width hint [in] h The integer to use as the minimum height hint
- See also:
- evas_object_size_hint_min_get()
void evas_object_size_hint_padding_get | ( | const Evas_Object * | obj, |
Evas_Coord * | l, | ||
Evas_Coord * | r, | ||
Evas_Coord * | t, | ||
Evas_Coord * | b | ||
) |
Gets the hints for an object's padding space.
- Since :
- 2.3.1
- Remarks:
- Padding is extra space that an object takes on each of its delimiting rectangle sides, in canvas units. This space is rendered transparent, naturally, as in the following figure:
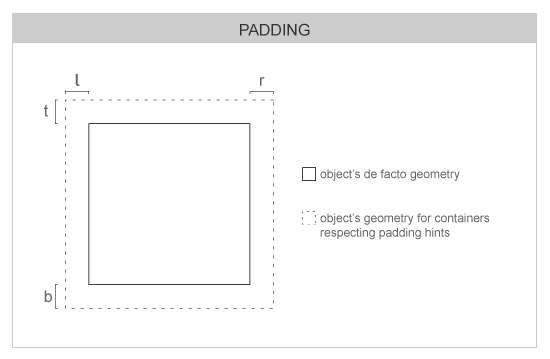
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Use
NULL
pointers on the hint components that you are not interested in: they are ignored by the function.
- Parameters:
-
[in] obj The given Evas object to query hints from [out] l The pointer to an integer in which to store left padding [out] r The pointer to an integer in which to store right padding [out] t The pointer to an integer in which to store top padding [out] b The pointer to an integer in which to store bottom padding
- See also:
- evas_object_size_hint_padding_set()
void evas_object_size_hint_padding_set | ( | Evas_Object * | obj, |
Evas_Coord | l, | ||
Evas_Coord | r, | ||
Evas_Coord | t, | ||
Evas_Coord | b | ||
) |
Sets the hints for an object's padding space.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] l The integer to specify left padding [in] r The integer to specify right padding [in] t The integer to specify top padding [in] b The integer to specify bottom padding
- See also:
- evas_object_size_hint_padding_get() for more information
void evas_object_size_hint_weight_get | ( | const Evas_Object * | obj, |
double * | x, | ||
double * | y | ||
) |
Gets the hints for an object's weight.
- Since :
- 2.3.1
- Remarks:
- Accepted values are
0
or positive values. Some users might use this hint as a boolean, but some might consider it as a proportion. See documentation of possible users, which in Evas are the box and table smart objects. - This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
-
Use
NULL
pointers on the hint components that you are not interested in: they are ignored by the function. -
If
obj
is invalid, then the hint components are set with0.0
- Parameters:
-
[in] obj The given Evas object to query hints from [out] x The pointer to a double in which to store the horizontal weight [out] y The pointer to a double in which to store the vertical weight
- See also:
- evas_object_size_hint_weight_set() for an example
void evas_object_size_hint_weight_set | ( | Evas_Object * | obj, |
double | x, | ||
double | y | ||
) |
Sets the hints for an object's weight.
- Since :
- 2.3.1
- Remarks:
- This is not a size enforcement in any way. It is just a hint that should be used whenever appropriate.
- This is a hint on how a container object should resize a given child within its area. Containers may adhere to the simpler logic of just expanding the child object's dimensions to fit its own (see the EVAS_HINT_EXPAND helper weight macro) or the complete one of taking each child's weight hint as real weights to how much of its size to allocate for them in each axis. A container is supposed to, after normalizing the weights of its children (with weight hints), distribute the space it has to layout them by those factors -- most weighted children get larger in this process than the least ones.
-
Default weight hint values are
0.0
, for both axis.
- Parameters:
-
[in] obj The given Evas object to query hints from [in] x The non-negative double value to use as horizontal weight hint [in] y The non-negative double value to use as vertical weight hint
- See also:
- evas_object_size_hint_weight_get() for more information