Tizen Native API
|
A Scroller can contain a scrollable object.
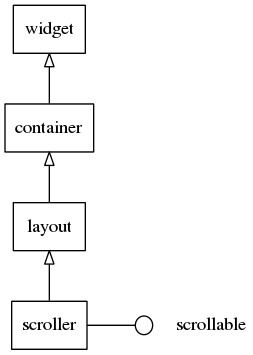
A scroller holds (and clips) a single object and "scrolls it around". This means that it allows the user to use a scroll bar (or a finger) to drag the viewable region around, moving through a much larger object that is contained in the scroller. The scroller is always having a small minimum size by default as it is not limited by the contents of the scroller.
This widget inherits from the Layout one, so that all the functions acting on it also work for scroller objects.
This widget emits the following signals, besides the ones sent from
"edge,left"
- The left edge of the content has been reached."edge,right"
- The right edge of the content has been reached."edge,top"
- The top edge of the content has been reached."edge,bottom"
- The bottom edge of the content has been reached."scroll"
- The content has been scrolled (moved)."scroll,anim,start"
- Scrolling animation has started."scroll,anim,stop"
- Scrolling animation has stopped."scroll,drag,start"
- Dragging the contents around has started."scroll,drag,stop"
- Dragging the contents around has stopped.
This widget implements the elm-scrollable-interface interface. Its (non-deprecated) API functions, except for elm_scroller_add(), which gives basic scroller objects, are meant to be a basis for all other scrollable widgets (i.e. widgets implementing elm-scrollable-interface). So, they work on both pristine scroller widgets and on other "specialized" scrollable widgets.
The "scroll,anim,*"
and "scroll,drag,*"
signals are only emitted by user intervention.
When Elementary is under its default profile and theme (meant for touch interfaces), scroll bars are not draggable, their function is merely to indicate how much has been scrolled.
When Elementary is under its desktop/standard profile and theme, the thumb scroll (a.k.a. finger scroll) won't work.
Default content parts of the scroller widget that you can use are:
"default"
- Content of the scroller.
Functions | |
Evas_Object * | elm_scroller_add (Evas_Object *parent) |
Adds a new scroller to the parent. | |
void | elm_scroller_content_min_limit (Evas_Object *obj, Eina_Bool w, Eina_Bool h) |
Makes the scroller's minimum size limited to the minimum size of the content. | |
void | elm_scroller_region_show (Evas_Object *obj, Evas_Coord x, Evas_Coord y, Evas_Coord w, Evas_Coord h) |
Shows a specific virtual region within the scroller content object. | |
void | elm_scroller_policy_set (Evas_Object *obj, Elm_Scroller_Policy policy_h, Elm_Scroller_Policy policy_v) |
Sets the scrollbar visibility policy. | |
void | elm_scroller_policy_get (const Evas_Object *obj, Elm_Scroller_Policy *policy_h, Elm_Scroller_Policy *policy_v) |
Gets the scrollbar visibility policy. | |
void | elm_scroller_single_direction_set (Evas_Object *obj, Elm_Scroller_Single_Direction single_dir) |
Sets the type of a single direction scroll. | |
Elm_Scroller_Single_Direction | elm_scroller_single_direction_get (const Evas_Object *obj) |
Gets the type of a single direction scroll. | |
void | elm_scroller_region_get (const Evas_Object *obj, Evas_Coord *x, Evas_Coord *y, Evas_Coord *w, Evas_Coord *h) |
Gets the currently visible content region. | |
void | elm_scroller_child_size_get (const Evas_Object *obj, Evas_Coord *w, Evas_Coord *h) |
Gets the size of the content object. | |
void | elm_scroller_bounce_set (Evas_Object *obj, Eina_Bool h_bounce, Eina_Bool v_bounce) |
Sets the bounce behavior. | |
void | elm_scroller_bounce_get (const Evas_Object *obj, Eina_Bool *h_bounce, Eina_Bool *v_bounce) |
Gets the bounce behaviour. | |
void | elm_scroller_page_relative_set (Evas_Object *obj, double h_pagerel, double v_pagerel) |
Sets the scroll page size relative to the viewport size. | |
void | elm_scroller_page_relative_get (const Evas_Object *obj, double *h_pagerel, double *v_pagerel) |
Gets a given scroller widget's scrolling page size, relative to its viewport size. | |
void | elm_scroller_page_size_set (Evas_Object *obj, Evas_Coord h_pagesize, Evas_Coord v_pagesize) |
Sets the scroll page size. | |
void | elm_scroller_page_size_get (const Evas_Object *obj, Evas_Coord *h_pagesize, Evas_Coord *v_pagesize) |
Retrieves a scroller widget's current page size. | |
void | elm_scroller_page_scroll_limit_set (Evas_Object *obj, int page_limit_h, int page_limit_v) |
Sets the maximum limit of the movable page at flicking. | |
void | elm_scroller_page_scroll_limit_get (Evas_Object *obj, int *page_limit_h, int *page_limit_v) |
Gets the maximum limit of the movable page at flicking. | |
void | elm_scroller_current_page_get (const Evas_Object *obj, int *h_pagenumber, int *v_pagenumber) |
Gets the scroll's current page number. | |
void | elm_scroller_last_page_get (const Evas_Object *obj, int *h_pagenumber, int *v_pagenumber) |
Gets the scroll's last page number. | |
void | elm_scroller_page_show (Evas_Object *obj, int h_pagenumber, int v_pagenumber) |
Shows a specific virtual region within the scroller content object by the page number. | |
void | elm_scroller_page_bring_in (Evas_Object *obj, int h_pagenumber, int v_pagenumber) |
Shows a specific virtual region within the scroller content object by the page number. | |
void | elm_scroller_region_bring_in (Evas_Object *obj, Evas_Coord x, Evas_Coord y, Evas_Coord w, Evas_Coord h) |
Shows a specific virtual region within the scroller content object. | |
void | elm_scroller_propagate_events_set (Evas_Object *obj, Eina_Bool propagation) |
Sets event propagation on a scroller. | |
Eina_Bool | elm_scroller_propagate_events_get (const Evas_Object *obj) |
Gets event propagation for a scroller. | |
void | elm_scroller_gravity_set (Evas_Object *obj, double x, double y) |
Sets the scrolling gravity on a scroller. | |
void | elm_scroller_gravity_get (const Evas_Object *obj, double *x, double *y) |
Gets scrolling gravity values for a scroller. | |
void | elm_scroller_loop_set (Evas_Object *obj, Eina_Bool loop_h, Eina_Bool loop_v) |
Sets the infinite loop for a scroller. | |
void | elm_scroller_movement_block_set (Evas_Object *obj, Elm_Scroller_Movement_Block block) |
Sets blocking of scrolling (per axis) on a given scroller. | |
Elm_Scroller_Movement_Block | elm_scroller_movement_block_get (const Evas_Object *obj) |
Gets a scroller's scroll blocking state. |
Enumeration Type Documentation
Enumeration that defines types that block the scroll movement in one or more directions.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- See also:
- elm_scroller_movement_block()
enum Elm_Scroller_Policy |
Enumeration that defines types that control when scrollbars should appear.
- Since :
- 2.3.1
- See also:
- elm_scroller_policy_set()
Enumeration that defines types that control how the content is scrolled.
- Since :
- 2.3.1
- See also:
- elm_scroller_single_direction_set()
Function Documentation
Evas_Object* elm_scroller_add | ( | Evas_Object * | parent | ) |
Adds a new scroller to the parent.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object, otherwise
NULL
if it cannot be created
void elm_scroller_bounce_get | ( | const Evas_Object * | obj, |
Eina_Bool * | h_bounce, | ||
Eina_Bool * | v_bounce | ||
) |
Gets the bounce behaviour.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [out] h_bounce If EINA_TRUE
horizontal bouncing is enabled otherwiseEINA_FALSE
to disable it[out] v_bounce If EINA_TRUE
vertical bouncing is enabled otherwiseEINA_FALSE
to disable it
- See also:
- elm_scroller_bounce_set()
void elm_scroller_bounce_set | ( | Evas_Object * | obj, |
Eina_Bool | h_bounce, | ||
Eina_Bool | v_bounce | ||
) |
Sets the bounce behavior.
- Since :
- 2.3.1
- Remarks:
- When scrolling, the scroller may "bounce" when reaching an edge of the content object. This is a visual way to indicate that the end has been reached. This is enabled by default for both axis. This API is set if it is enabled for the given axis with the boolean parameters for each axis.
- Parameters:
-
[in] obj The scroller object [in] h_bounce If EINA_TRUE
horizontal bouncing is enabled, otherwiseEINA_FALSE
to disable it[in] v_bounce If EINA_TRUE
vertical bouncing is enabled otherwiseEINA_FALSE
to disable it
void elm_scroller_child_size_get | ( | const Evas_Object * | obj, |
Evas_Coord * | w, | ||
Evas_Coord * | h | ||
) |
Gets the size of the content object.
This gets the size of the content object of the scroller.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [out] w The width of the content object [out] h The height of the content object
void elm_scroller_content_min_limit | ( | Evas_Object * | obj, |
Eina_Bool | w, | ||
Eina_Bool | h | ||
) |
Makes the scroller's minimum size limited to the minimum size of the content.
- Since :
- 2.3.1
- Remarks:
- By default the scroller is as small as its design allows, irrespective of its content. This makes the scroller's minimum size the right size horizontally and/or vertically to perfectly fit its content in that direction.
- Parameters:
-
[in] obj The scroller object [in] w The boolean value that enables limiting minimum size horizontally [in] h The boolean value that enables limiting minimum size vertically
void elm_scroller_current_page_get | ( | const Evas_Object * | obj, |
int * | h_pagenumber, | ||
int * | v_pagenumber | ||
) |
Gets the scroll's current page number.
- Since :
- 2.3.1
- Remarks:
- The page number starts from
0
.0
is the first page. Current page means the page which meets the top-left corner of the viewport. If there are two or more pages in the viewport, it returns the number of the pages that meet the top-left corner of the viewport.
- Parameters:
-
[in] obj The scroller object [out] h_pagenumber The horizontal page number [out] v_pagenumber The vertical page number
void elm_scroller_gravity_get | ( | const Evas_Object * | obj, |
double * | x, | ||
double * | y | ||
) |
Gets scrolling gravity values for a scroller.
- Since :
- 2.3.1
- Remarks:
- This gets gravity values for a scroller.
- Parameters:
-
[in] obj The scroller object [out] x The scrolling horizontal gravity [out] y The scrolling vertical gravity
- See also:
- elm_scroller_gravity_set()
void elm_scroller_gravity_set | ( | Evas_Object * | obj, |
double | x, | ||
double | y | ||
) |
Sets the scrolling gravity on a scroller.
- Since :
- 2.3.1
- Remarks:
- The gravity, defines how the scroller adjusts its view when the size of the scroller content increases.
- The scroller adjusts the view to glue itself as follows:
x=0.0, for showing the left most region of the content. x=1.0, for showing the right most region of the content. y=0.0, for showing the bottom most region of the content. y=1.0, for showing the top most region of the content.
- Remarks:
- Default value for x and y is
0.0
.
- Parameters:
-
[in] obj The scroller object [in] x The scrolling horizontal gravity [in] y The scrolling vertical gravity
void elm_scroller_last_page_get | ( | const Evas_Object * | obj, |
int * | h_pagenumber, | ||
int * | v_pagenumber | ||
) |
Gets the scroll's last page number.
This returns the last page number among the pages.
- Since :
- 2.3.1
- Remarks:
- The page number starts from
0
.0
is the first page.
- Parameters:
-
[in] obj The scroller object [out] h_pagenumber The horizontal page number [out] v_pagenumber The vertical page number
void elm_scroller_loop_set | ( | Evas_Object * | obj, |
Eina_Bool | loop_h, | ||
Eina_Bool | loop_v | ||
) |
Sets the infinite loop for a scroller.
- Remarks:
- Tizen only feature (but will be patched into upstream)
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Remarks:
- This sets the infinite loop for a scroller.
- Parameters:
-
[in] obj The scroller object [in] loop_h The scrolling horizontal loop [in] loop_v The scrolling vertical loop
Gets a scroller's scroll blocking state.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object
- Returns:
- The blocking state
- See also:
- elm_scroller_movement_block_set()
void elm_scroller_movement_block_set | ( | Evas_Object * | obj, |
Elm_Scroller_Movement_Block | block | ||
) |
Sets blocking of scrolling (per axis) on a given scroller.
This function blocks the scrolling movement (by input of a user) in a given direction. One can disable movements in the X axis, the Y axis, or both. The default value is ELM_SCROLLER_MOVEMENT_NO_BLOCK
, where movements are allowed in both directions.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Remarks:
- What makes this function different from elm_object_scroll_freeze_push(), elm_object_scroll_hold_push(), and elm_object_scroll_lock_x_set() (or elm_object_scroll_lock_y_set()) is that it doesn't propagate its effects to any parent or child widget of obj. Only the target scrollable widget is locked with regards to scrolling.
- Parameters:
-
[in] obj The scroller object [in] block The axis to block
void elm_scroller_page_bring_in | ( | Evas_Object * | obj, |
int | h_pagenumber, | ||
int | v_pagenumber | ||
) |
Shows a specific virtual region within the scroller content object by the page number.
- Since :
- 2.3.1
- Remarks:
- (0, 0) of the indicated page is located at the top-left corner of the viewport. This slides to the page with animation.
Example of usage:
sc = elm_scroller_add(win); elm_object_content_set(sc, content); elm_scroller_page_relative_set(sc, 1, 0); elm_scroller_last_page_get(sc, &h_page, &v_page); elm_scroller_page_bring_in(sc, h_page, v_page);
- Parameters:
-
[in] obj The scroller object [in] h_pagenumber The horizontal page number [in] v_pagenumber The vertical page number
- See also:
- elm_scroller_page_show()
void elm_scroller_page_relative_get | ( | const Evas_Object * | obj, |
double * | h_pagerel, | ||
double * | v_pagerel | ||
) |
Gets a given scroller widget's scrolling page size, relative to its viewport size.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [out] h_pagerel The pointer to a variable in which to store the horizontal page (relative) size [out] v_pagerel The pointer to a variable in which to store the vertical page (relative) size
- See also:
- elm_scroller_page_relative_set()
void elm_scroller_page_relative_set | ( | Evas_Object * | obj, |
double | h_pagerel, | ||
double | v_pagerel | ||
) |
Sets the scroll page size relative to the viewport size.
- Since :
- 2.3.1
- Remarks:
- The scroller is capable of limiting scrolling by the user to "pages". That is to jump by and only show a "whole page" at a time as if the continuous area of the scroller content is split into page sized pieces. This sets the size of a page relative to the viewport of the scroller.
1.0
is "1 viewport" which is the size (horizontally or vertically).0.0
turns it off in that axis. This is mutually exclusive with the page size (see elm_scroller_page_size_set() for more information). Likewise0.5
is "half a viewport". Usable values are normally between0.0
and1.0
including1.0
. If you only want1
axis to be page "limited", use0.0
for the other axis.
- Parameters:
-
[in] obj The scroller object [in] h_pagerel The horizontal page relative size [in] v_pagerel The vertical page relative size
void elm_scroller_page_scroll_limit_get | ( | Evas_Object * | obj, |
int * | page_limit_h, | ||
int * | page_limit_v | ||
) |
Gets the maximum limit of the movable page at flicking.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [out] page_limit_h The maximum limit of the movable horizontal page [out] page_limit_v The maximum limit of the movable vertical page
- See also:
- elm_scroller_page_scroll_limit_set()
void elm_scroller_page_scroll_limit_set | ( | Evas_Object * | obj, |
int | page_limit_h, | ||
int | page_limit_v | ||
) |
Sets the maximum limit of the movable page at flicking.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Remarks:
- The value of the maximum movable page should be more than
1
.
- Parameters:
-
[in] obj The scroller object [in] page_limit_h The maximum limit of the movable horizontal page [in] page_limit_v The maximum limit of the movable vertical page
- See also:
- elm_scroller_page_scroll_limit_get()
void elm_scroller_page_show | ( | Evas_Object * | obj, |
int | h_pagenumber, | ||
int | v_pagenumber | ||
) |
Shows a specific virtual region within the scroller content object by the page number.
- Since :
- 2.3.1
- Remarks:
- (0, 0) of the indicated page is located at the top-left corner of the viewport. This jumps to the page directly without animation.
Example of usage:
sc = elm_scroller_add(win); elm_object_content_set(sc, content); elm_scroller_page_relative_set(sc, 1, 0); elm_scroller_current_page_get(sc, &h_page, &v_page); elm_scroller_page_show(sc, h_page + 1, v_page);
- Parameters:
-
[in] obj The scroller object [in] h_pagenumber The horizontal page number [in] v_pagenumber The vertical page number
- See also:
- elm_scroller_page_bring_in()
void elm_scroller_page_size_get | ( | const Evas_Object * | obj, |
Evas_Coord * | h_pagesize, | ||
Evas_Coord * | v_pagesize | ||
) |
Retrieves a scroller widget's current page size.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [out] h_pagesize The location to store its horizontal page size [out] v_pagesize The location to store its vertical page size
- See also:
- elm_scroller_page_size_set() for more details
- elm_scroller_page_relative_set()
void elm_scroller_page_size_set | ( | Evas_Object * | obj, |
Evas_Coord | h_pagesize, | ||
Evas_Coord | v_pagesize | ||
) |
Sets the scroll page size.
This sets the page size to an absolute fixed value, with 0
turning it off for that axis.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [in] h_pagesize The horizontal page size [in] v_pagesize The vertical page size
void elm_scroller_policy_get | ( | const Evas_Object * | obj, |
Elm_Scroller_Policy * | policy_h, | ||
Elm_Scroller_Policy * | policy_v | ||
) |
Gets the scrollbar visibility policy.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [out] policy_h The horizontal scrollbar policy [out] policy_v The vertical scrollbar policy
- See also:
- elm_scroller_policy_set()
void elm_scroller_policy_set | ( | Evas_Object * | obj, |
Elm_Scroller_Policy | policy_h, | ||
Elm_Scroller_Policy | policy_v | ||
) |
Sets the scrollbar visibility policy.
This sets the scrollbar visibility policy for the given scroller. ELM_SCROLLER_POLICY_AUTO
means the scrollbar is made visible if it is needed, and otherwise kept hidden. ELM_SCROLLER_POLICY_ON
turns it on all the time, and ELM_SCROLLER_POLICY_OFF
always keeps it off. This applies respectively for the horizontal and vertical scrollbars.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [in] policy_h The horizontal scrollbar policy [in] policy_v The vertical scrollbar policy
Eina_Bool elm_scroller_propagate_events_get | ( | const Evas_Object * | obj | ) |
Gets event propagation for a scroller.
This gets the event propagation for a scroller.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object
- Returns:
- The propagation state
- See also:
- elm_scroller_propagate_events_set()
void elm_scroller_propagate_events_set | ( | Evas_Object * | obj, |
Eina_Bool | propagation | ||
) |
Sets event propagation on a scroller.
This enables or disables event propagation from the scroller content to the scroller and its parent. By default event propagation is enabled.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [in] propagation The boolean value that indicates whether propagation is enabled
void elm_scroller_region_bring_in | ( | Evas_Object * | obj, |
Evas_Coord | x, | ||
Evas_Coord | y, | ||
Evas_Coord | w, | ||
Evas_Coord | h | ||
) |
Shows a specific virtual region within the scroller content object.
- Since :
- 2.3.1
- Remarks:
- This ensures that all (or part, if it does not fit) of the designated region in the virtual content object ((0, 0) starting at the top-left of the virtual content object) is shown within the scroller. Unlike elm_scroller_region_show(), this allows the scroller to "smoothly slide" to this location (if configuration in general calls for transitions). It may not jump immediately to the new location and may take a while and show other content along the way.
- Parameters:
-
[in] obj The scroller object [in] x The x coordinate of the region [in] y The y coordinate of the region [in] w The width of the region [in] h The height of the region
- See also:
- elm_scroller_region_show()
void elm_scroller_region_get | ( | const Evas_Object * | obj, |
Evas_Coord * | x, | ||
Evas_Coord * | y, | ||
Evas_Coord * | w, | ||
Evas_Coord * | h | ||
) |
Gets the currently visible content region.
This gets the current region in the content object that is visible through the scroller. The region co-ordinates are returned in the values that x, y, w, and h point to.
- Since :
- 2.3.1
- Remarks:
- All the coordinates are relative to the content.
- Parameters:
-
[in] obj The scroller object [out] x The x coordinate of the region [out] y The y coordinate of the region [out] w The width of the region [out] h The height of the region
- See also:
- elm_scroller_region_show()
void elm_scroller_region_show | ( | Evas_Object * | obj, |
Evas_Coord | x, | ||
Evas_Coord | y, | ||
Evas_Coord | w, | ||
Evas_Coord | h | ||
) |
Shows a specific virtual region within the scroller content object.
- Since :
- 2.3.1
- Remarks:
- This ensures that all (or part if it does not fit) of the designated region in the virtual content object ((0, 0) starting at the top-left of the virtual content object) is shown within the scroller.
- Parameters:
-
[in] obj The scroller object [in] x The x coordinate of the region [in] y The y coordinate of the region [in] w The width of the region [in] h The height of the region
Gets the type of a single direction scroll.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object
- Returns:
- The type of single direction
- See also:
- elm_scroller_single_direction_get()
void elm_scroller_single_direction_set | ( | Evas_Object * | obj, |
Elm_Scroller_Single_Direction | single_dir | ||
) |
Sets the type of a single direction scroll.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The scroller object [in] single_dir The type of single direction
- See also:
- elm_scroller_single_direction_get()