Tizen Native API
|
A spinner is a widget which allows the user to increase or decrease numeric values using arrow buttons, or edit values directly by clicking over it and typing the new value.
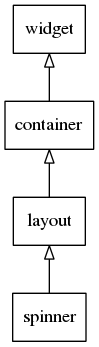
By default, the spinner does not wrap and has a label of "%.0f" (just showing the integer value of double).
A spinner has a label that is formatted with floating point values and thus accepts a printf-style format string, like “%1.2f units”.
It also allows specific values to be replaced by pre-defined labels.
This widget inherits from the Layout one, so that all the functions acting on it also work for spinner objects.
This widget emits the following signals, besides the ones sent from Layout :
"changed"
- Whenever the spinner value is changed."delay,changed"
- A short time after the value is changed by the user. This is called only when the user stops dragging for a very short period or when they release their finger/mouse, so it avoids the possible expensive reactions to the value change."language,changed"
- The program's language is changed.
Available styles for it:
"default"
;"vertical"
: Up/down buttons on the right side and text aligned to the left.
Supported common elm_object APIs.
- elm_object_signal_emit
- elm_object_signal_callback_add
- elm_object_signal_callback_del
- elm_object_disabled_set
- elm_object_disabled_get
Functions | |
Evas_Object * | elm_spinner_add (Evas_Object *parent) |
Adds a new spinner widget to the given parent Elementary (container) object. | |
void | elm_spinner_label_format_set (Evas_Object *obj, const char *fmt) |
Sets the format string of the displayed label. | |
const char * | elm_spinner_label_format_get (const Evas_Object *obj) |
Gets the label format of the spinner. | |
void | elm_spinner_min_max_set (Evas_Object *obj, double min, double max) |
Sets the minimum and maximum values for the spinner. | |
void | elm_spinner_min_max_get (const Evas_Object *obj, double *min, double *max) |
Gets the minimum and maximum values of the spinner. | |
void | elm_spinner_step_set (Evas_Object *obj, double step) |
Sets the step used to increment or decrement the spinner value. | |
double | elm_spinner_step_get (const Evas_Object *obj) |
Gets the step used to increment or decrement the spinner value. | |
void | elm_spinner_value_set (Evas_Object *obj, double val) |
Sets the value that the spinner displays. | |
double | elm_spinner_value_get (const Evas_Object *obj) |
Gets the value displayed by the spinner. | |
void | elm_spinner_wrap_set (Evas_Object *obj, Eina_Bool wrap) |
Sets whether the spinner should wrap when it reaches its minimum or maximum value. | |
Eina_Bool | elm_spinner_wrap_get (const Evas_Object *obj) |
Gets whether the spinner should wrap when it reaches its minimum or maximum value. | |
void | elm_spinner_editable_set (Evas_Object *obj, Eina_Bool editable) |
Sets whether the spinner can be directly edited by the user. | |
Eina_Bool | elm_spinner_editable_get (const Evas_Object *obj) |
Gets whether the spinner can be directly edited by the user. | |
void | elm_spinner_special_value_add (Evas_Object *obj, double value, const char *label) |
Sets a special string to display in place of the numerical value. | |
void | elm_spinner_special_value_del (Evas_Object *obj, double value) |
Deletes the special string displayed in place of the numerical value. | |
const char * | elm_spinner_special_value_get (Evas_Object *obj, double value) |
Gets the special string displayed in place of the numerical value. | |
void | elm_spinner_interval_set (Evas_Object *obj, double interval) |
Sets the interval on the time updates for a user's mouse button hold on the spinner widgets' arrows. | |
double | elm_spinner_interval_get (const Evas_Object *obj) |
Gets the interval on the time updates for a user's mouse button hold on the spinner widgets' arrows. | |
void | elm_spinner_base_set (Evas_Object *obj, double base) |
Sets the base for rounding. | |
double | elm_spinner_base_get (const Evas_Object *obj) |
Gets the base for rounding. | |
void | elm_spinner_round_set (Evas_Object *obj, int rnd) |
Sets the round value for rounding. | |
int | elm_spinner_round_get (const Evas_Object *obj) |
Gets the round value for rounding. |
Function Documentation
Evas_Object* elm_spinner_add | ( | Evas_Object * | parent | ) |
Adds a new spinner widget to the given parent Elementary (container) object.
This function inserts a new spinner widget on the canvas.
- Since :
- 2.3.1
- Parameters:
-
[in] parent The parent object
- Returns:
- A new spinner widget handle, otherwise
NULL
in case of an error
double elm_spinner_base_get | ( | const Evas_Object * | obj | ) |
Gets the base for rounding.
- Since :
- 2.3.1
- Remarks:
- This returns the base for rounding
- Parameters:
-
[in] obj The spinner object
- Returns:
- The base rounding value
void elm_spinner_base_set | ( | Evas_Object * | obj, |
double | base | ||
) |
Sets the base for rounding.
- Since :
- 2.3.1
- Remarks:
- Rounding works as follows:
rounded_val = base + (double)(((value - base) / round) * round)
Where rounded_val, value, and base are doubles, and round is an integer.
This means that things are rounded to increments (or decrements) of "round" starting from the value base. The default base for rounding is 0
.
Example: round = 3, base = 2 Values: 3, 6, 9, 12, 15, ...
Example: round = 2, base = 5.5 Values: 5.5, 7.5, 9.5, 11.5, ...
- Parameters:
-
[in] obj The spinner object [in] base The base value
Eina_Bool elm_spinner_editable_get | ( | const Evas_Object * | obj | ) |
Gets whether the spinner can be directly edited by the user.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
EINA_TRUE
indicates that the edition is enabled, otherwiseEINA_FALSE
indicates that it's disabled
If obj isNULL
,EINA_FALSE
is returned.
- See also:
- elm_spinner_editable_set()
void elm_spinner_editable_set | ( | Evas_Object * | obj, |
Eina_Bool | editable | ||
) |
Sets whether the spinner can be directly edited by the user.
- Since :
- 2.3.1
- Remarks:
- Spinner objects can have an edition disabled, in which case they can be changed only by arrows. Useful for contexts where you don't want your users to interact by writing the value. Specially when using special values, the user can see real values instead of special labels on the edition.
- It's enabled by default.
- Parameters:
-
[in] obj The spinner object [in] editable If EINA_TRUE
allow users to edit it, otherwiseEINA_FALSE
to not allow users to edit it directly
- See also:
- elm_spinner_editable_get()
double elm_spinner_interval_get | ( | const Evas_Object * | obj | ) |
Gets the interval on the time updates for a user's mouse button hold on the spinner widgets' arrows.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
- The interval value, in seconds, set on it
- See also:
- elm_spinner_interval_set()
void elm_spinner_interval_set | ( | Evas_Object * | obj, |
double | interval | ||
) |
Sets the interval on the time updates for a user's mouse button hold on the spinner widgets' arrows.
- Since :
- 2.3.1
- Remarks:
- This interval value is decreased while the user holds the mouse pointer either by incrementing or decrementing the spinner's value.
- This helps the user to get to a given value, which is distant from the current one, in an easier/faster way, as it updates the value in that set interval time on mouse button holds.
-
The default starting interval value for automatic changes is
0.85
seconds.
- Parameters:
-
[in] obj The spinner object [in] interval The interval value in seconds
- See also:
- elm_spinner_interval_get()
const char* elm_spinner_label_format_get | ( | const Evas_Object * | obj | ) |
Gets the label format of the spinner.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
- The text label format string in UTF-8
- See also:
- elm_spinner_label_format_set()
void elm_spinner_label_format_set | ( | Evas_Object * | obj, |
const char * | fmt | ||
) |
Sets the format string of the displayed label.
- Since :
- 2.3.1
- Remarks:
- If
NULL
, this sets the format to "%.0f". If not, it sets the format string for the label text. For label text a floating point value is provided, so the label text can display up to1
floating point value. Note that this is optional. - Use a format string such as "%1.2f meters" for example, and it displays values like: "3.14 meters" for a value equal to 3.14159.
- Default is "%0.f".
- Parameters:
-
[in] obj The spinner object [in] fmt The format string for the label display
- See also:
- elm_spinner_label_format_get()
void elm_spinner_min_max_get | ( | const Evas_Object * | obj, |
double * | min, | ||
double * | max | ||
) |
Gets the minimum and maximum values of the spinner.
- Since :
- 2.3.1
- Remarks:
- If only one value is needed, the other pointer can be passed as
NULL
.
- Parameters:
-
[in] obj The spinner object [out] min The pointer to store the minimum value [out] max The pointer to store the maximum value
- See also:
- elm_spinner_min_max_set()
void elm_spinner_min_max_set | ( | Evas_Object * | obj, |
double | min, | ||
double | max | ||
) |
Sets the minimum and maximum values for the spinner.
This defines the allowed range of values to be selected by the user.
- Since :
- 2.3.1
- Remarks:
- If the actual value is less than min, it is updated to min. If it is bigger then max, it is updated to max. Actual value can be obtained with elm_spinner_value_get().
-
By default, min is equal to
0
, and max is equal to100
. - Maximum must be greater than minimum.
- Parameters:
-
[in] obj The spinner object [in] min The minimum value [in] max The maximum value
- See also:
- elm_spinner_min_max_get()
int elm_spinner_round_get | ( | const Evas_Object * | obj | ) |
Gets the round value for rounding.
This returns the round value for rounding.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
- The rounding value
void elm_spinner_round_set | ( | Evas_Object * | obj, |
int | rnd | ||
) |
Sets the round value for rounding.
This sets the rounding value used for the value rounding in the spinner.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object [in] rnd The rounding value
void elm_spinner_special_value_add | ( | Evas_Object * | obj, |
double | value, | ||
const char * | label | ||
) |
Sets a special string to display in place of the numerical value.
- Since :
- 2.3.1
- Remarks:
- It's useful for cases when a user should select an item that is better indicated by a label than a value. For example, weekdays or months.
E.g.:
sp = elm_spinner_add(win); elm_spinner_min_max_set(sp, 1, 3); elm_spinner_special_value_add(sp, 1, "January"); elm_spinner_special_value_add(sp, 2, "February"); elm_spinner_special_value_add(sp, 3, "March"); evas_object_show(sp);
- Remarks:
- If another label was previously set to value, it is replaced by the new label.
- Parameters:
-
[in] obj The spinner object [in] value The value to be replaced [in] label The label to be used
void elm_spinner_special_value_del | ( | Evas_Object * | obj, |
double | value | ||
) |
Deletes the special string displayed in place of the numerical value.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Remarks:
- It removes a previously added special value. After this, the spinner displays the value itself instead of a label.
- Parameters:
-
[in] obj The spinner object [in] value The replaced value
- See also:
- elm_spinner_special_value_add()
const char* elm_spinner_special_value_get | ( | Evas_Object * | obj, |
double | value | ||
) |
Gets the special string displayed in place of the numerical value.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object [in] value The replaced value
- Returns:
- The used label
- See also:
- elm_spinner_special_value_add()
double elm_spinner_step_get | ( | const Evas_Object * | obj | ) |
Gets the step used to increment or decrement the spinner value.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
- The step value
- See also:
- elm_spinner_step_get()
void elm_spinner_step_set | ( | Evas_Object * | obj, |
double | step | ||
) |
Sets the step used to increment or decrement the spinner value.
- Since :
- 2.3.1
- Remarks:
- This value is incremented or decremented to the displayed value. It is incremented while the user keeps the right or top arrow pressed, and is decremented while the user keeps the left or bottom arrow pressed.
- The interval to increment / decrement can be set using elm_spinner_interval_set().
-
By default the step value is equal to
1
.
- Parameters:
-
[in] obj The spinner object [in] step The step value
- See also:
- elm_spinner_step_get()
double elm_spinner_value_get | ( | const Evas_Object * | obj | ) |
Gets the value displayed by the spinner.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
- The value displayed
- See also:
- elm_spinner_value_set()
void elm_spinner_value_set | ( | Evas_Object * | obj, |
double | val | ||
) |
Sets the value that the spinner displays.
- Since :
- 2.3.1
- Remarks:
- The value is presented on the label following the format specified by elm_spinner_format_set().
- The value must to be between the min and max values. These values are set by elm_spinner_min_max_set().
- Parameters:
-
[in] obj The spinner object [in] val The value to be displayed
- See also:
- elm_spinner_value_get()
- elm_spinner_format_set()
- elm_spinner_min_max_set()
Eina_Bool elm_spinner_wrap_get | ( | const Evas_Object * | obj | ) |
Gets whether the spinner should wrap when it reaches its minimum or maximum value.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The spinner object
- Returns:
EINA_TRUE
indicates that wrap is enabled, otherwiseEINA_FALSE
indicates that it's disabled
If obj isNULL
,EINA_FALSE
is returned.
- See also:
- elm_spinner_wrap_set()
void elm_spinner_wrap_set | ( | Evas_Object * | obj, |
Eina_Bool | wrap | ||
) |
Sets whether the spinner should wrap when it reaches its minimum or maximum value.
- Since :
- 2.3.1
- Remarks:
- It is disabled by default. If disabled when the user tries to increment the value, but the displayed value plus the step value is bigger than the maximum value, the new value is the maximum value. The same happens when the user tries to decrement it, but the value less than step is less than the minimum value. In this case, the new displayed value is the minimum value.
- If wrap is enabled when the user tries to increment the value, but the displayed value plus the step value is bigger than the maximum value, the new value is the minimum value. When the the user tries to decrement it, but the value less step is less than the minimum value, the new displayed value is the maximum value.
E.g.:
- min value = 10
- max value = 50
- step value = 20
- displayed value = 20
When the user decrements the value (using left or bottom arrow), it displays 50
.
- Parameters:
-
[in] obj The spinner object [in] wrap If EINA_TRUE
wrap is enabled, otherwiseEINA_FALSE
to disable it
- See also:
- elm_spinner_wrap_get()