Tizen Native API
|
The window class of Elementary contains functions to manipulate Windows.
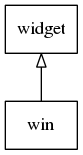
The Evas engine that is used to render the window contents is specified in the system or user elementary config files (whichever is found last), and can be overridden by using the ELM_ENGINE environment variable for testing. Engines that may be supported (depending on Evas and Ecore-Evas compilation setup and modules that are actually installed at runtime) are (listed in order of best supported and most likely to be complete and work to lowest quality).
- "x11", "x", "software-x11", "software_x11" (Software rendering in X11)
- "gl", "opengl", "opengl-x11", "opengl_x11" (OpenGL or OpenGL-ES2 rendering in X11)
- "shot:..." (Virtual screenshot renderer - renders to output file and exits)
- "fb", "software-fb", "software_fb" (Linux framebuffer direct software rendering)
- "sdl", "software-sdl", "software_sdl" (SDL software rendering to SDL buffer)
- "gl-sdl", "gl_sdl", "opengl-sdl", "opengl_sdl" (OpenGL or OpenGL-ES2 rendering using SDL as the buffer)
- "gdi", "software-gdi", "software_gdi" (Windows WIN32 rendering via GDI with software)
- "dfb", "directfb" (Rendering to a DirectFB window)
- "x11-8", "x8", "software-8-x11", "software_8_x11" (Rendering in grayscale using a dedicated 8bit software engine in X11)
- "x11-16", "x16", "software-16-x11", "software_16_x11" (Rendering in X11 using a 16bit software engine)
- "wince-gdi", "software-16-wince-gdi", "software_16_wince_gdi" (Windows CE rendering via GDI with a 16bit software renderer)
- "sdl-16", "software-16-sdl", "software_16_sdl" (Rendering to SDL buffer with a 16bit software renderer)
- "ews" (rendering to EWS - Ecore + Evas Single Process Windowing System)
- "gl-cocoa", "gl_cocoa", "opengl-cocoa", "opengl_cocoa" (OpenGL rendering in Cocoa)
- "psl1ght" (PS3 rendering using PSL1GHT)
All engines use a simple string to select the engine to render, EXCEPT the "shot" engine. This actually encodes the output of the virtual screenshot and the time for which to delay the engine string. The engine string is encoded in the following way:
"shot:[delay=XX][:][repeat=DDD][:][file=XX]"
Where options are separated by a ":" char, if more than one option is given with delay, if provided being the first option and file the last (order is important). The delay specifies how long to wait after the window is displayed before doing the virtual "in memory" rendering and then saving the output to the file specified by the file option (and then exiting). If no delay is given, the default value is 0.5
seconds. If no file is given the default output file is "out.png". The repeat option is for continuous capturing of screenshots. The repeat range is from 1
to 999
and the filename is fixed to "out001.png" Some examples of using the shot engine are:
ELM_ENGINE="shot:delay=1.0:repeat=5:file=elm_test.png" elementary_test ELM_ENGINE="shot:delay=1.0:file=elm_test.png" elementary_test ELM_ENGINE="shot:file=elm_test2.png" elementary_test ELM_ENGINE="shot:delay=2.0" elementary_test ELM_ENGINE="shot:" elementary_test
Signals for which you can add callbacks are:
- "delete,request": The user requested to close the window. See elm_win_autodel_set().
- "focus,in": The window got focus.
- "focus,out": The window lost focus.
- "moved": The window that holds the canvas is moved.
- "withdrawn": The window is still being managed normally but is removed from the view.
- "iconified": The window is minimized (perhaps into an icon or a taskbar).
- "normal": The window is in a normal state (not withdrawn or iconified).
- "stick": The window has become sticky (shows on all desktops).
- "unstick": The window has stopped being sticky.
- "fullscreen": The window has become fullscreen.
- "unfullscreen": The window has stopped being fullscreen.
- "maximized": The window has been maximized.
- "unmaximized": The window has stopped being maximized.
- "wm,rotation,changed": The rotation of the window has been changed by the Windows Manager.
- "ioerr": A low-level I/O error has occurred in the display system.
Functions | |
Evas_Object * | elm_win_add (Evas_Object *parent, const char *name, Elm_Win_Type type) |
Adds a window object. If this is the first window created, pass NULL as parent. | |
Elm_Win_Type | elm_win_type_get (Evas_Object *obj) |
Gets the type of a window. | |
Evas_Object * | elm_win_util_standard_add (const char *name, const char *title) |
Adds a window object with a standard setup. | |
void | elm_win_resize_object_add (Evas_Object *obj, Evas_Object *subobj) |
Adds subobj as a resize object of the window obj. | |
void | elm_win_resize_object_del (Evas_Object *obj, Evas_Object *subobj) |
Deletes subobj as a resize object of the window obj. | |
void | elm_win_title_set (Evas_Object *obj, const char *title) |
Sets the title of the window. | |
const char * | elm_win_title_get (const Evas_Object *obj) |
Gets the title of the window. | |
void | elm_win_icon_name_set (Evas_Object *obj, const char *icon_name) |
Sets the icon name of the window. | |
const char * | elm_win_icon_name_get (const Evas_Object *obj) |
Gets the icon name of the window. | |
void | elm_win_role_set (Evas_Object *obj, const char *role) |
Sets the role of the window. | |
const char * | elm_win_role_get (const Evas_Object *obj) |
Gets the role of the window. | |
void | elm_win_icon_object_set (Evas_Object *obj, Evas_Object *icon) |
Sets a window object icon. | |
const Evas_Object * | elm_win_icon_object_get (const Evas_Object *obj) |
Gets the icon object used for the window. | |
void | elm_win_autodel_set (Evas_Object *obj, Eina_Bool autodel) |
Sets the window autodel state. | |
Eina_Bool | elm_win_autodel_get (const Evas_Object *obj) |
Gets the window autodel state. | |
void | elm_win_activate (Evas_Object *obj) |
Activates the window object. | |
void | elm_win_lower (Evas_Object *obj) |
Lowers the window object. | |
void | elm_win_raise (Evas_Object *obj) |
Raises the window object. | |
void | elm_win_center (Evas_Object *obj, Eina_Bool h, Eina_Bool v) |
Centers a window on its screen. | |
void | elm_win_borderless_set (Evas_Object *obj, Eina_Bool borderless) |
Sets the borderless state of a window. | |
Eina_Bool | elm_win_borderless_get (const Evas_Object *obj) |
Gets the borderless state of a window. | |
void | elm_win_shaped_set (Evas_Object *obj, Eina_Bool shaped) |
Sets the shaped state of a window. | |
Eina_Bool | elm_win_shaped_get (const Evas_Object *obj) |
Gets the shaped state of a window. | |
void | elm_win_alpha_set (Evas_Object *obj, Eina_Bool alpha) |
Sets the alpha channel state of a window. | |
Eina_Bool | elm_win_alpha_get (const Evas_Object *obj) |
Gets the alpha channel state of a window. | |
void | elm_win_override_set (Evas_Object *obj, Eina_Bool override) |
Sets the override state of a window. | |
Eina_Bool | elm_win_override_get (const Evas_Object *obj) |
Gets the override state of a window. | |
void | elm_win_fullscreen_set (Evas_Object *obj, Eina_Bool fullscreen) |
Sets the fullscreen state of a window. | |
Eina_Bool | elm_win_fullscreen_get (const Evas_Object *obj) |
Geta the fullscreen state of a window. | |
void | elm_win_maximized_set (Evas_Object *obj, Eina_Bool maximized) |
Sets the maximized state of a window. | |
Eina_Bool | elm_win_maximized_get (const Evas_Object *obj) |
Gets the maximized state of a window. | |
void | elm_win_iconified_set (Evas_Object *obj, Eina_Bool iconified) |
Sets the iconified state of a window. | |
Eina_Bool | elm_win_iconified_get (const Evas_Object *obj) |
Gets the iconified state of a window. | |
void | elm_win_withdrawn_set (Evas_Object *obj, Eina_Bool withdrawn) |
Sets the withdrawn state of a window. | |
Eina_Bool | elm_win_withdrawn_get (const Evas_Object *obj) |
Gets the withdrawn state of a window. | |
void | elm_win_profiles_set (Evas_Object *obj, const char **profiles, unsigned int num_profiles) |
Sets the profile list of a window. | |
const char * | elm_win_profile_get (const Evas_Object *obj) |
Gets the profile of a window. | |
void | elm_win_urgent_set (Evas_Object *obj, Eina_Bool urgent) |
Sets the urgent state of a window. | |
Eina_Bool | elm_win_urgent_get (const Evas_Object *obj) |
Gets the urgent state of a window. | |
void | elm_win_demand_attention_set (Evas_Object *obj, Eina_Bool demand_attention) |
Sets the demand_attention state of a window. | |
Eina_Bool | elm_win_demand_attention_get (const Evas_Object *obj) |
Gets the demand_attention state of a window. | |
void | elm_win_modal_set (Evas_Object *obj, Eina_Bool modal) |
Sets the modal state of a window. | |
Eina_Bool | elm_win_modal_get (const Evas_Object *obj) |
Gets the modal state of a window. | |
void | elm_win_aspect_set (Evas_Object *obj, double aspect) |
Sets the aspect ratio of a window. | |
double | elm_win_aspect_get (const Evas_Object *obj) |
Gets the aspect ratio of a window. | |
void | elm_win_size_base_set (Evas_Object *obj, int w, int h) |
Sets the base window size used with stepping calculation. | |
void | elm_win_size_base_get (Evas_Object *obj, int *w, int *h) |
Gets the base size of a window. | |
void | elm_win_size_step_set (Evas_Object *obj, int w, int h) |
Sets the window stepping used with sizing calculation. | |
void | elm_win_size_step_get (Evas_Object *obj, int *w, int *h) |
Gets the stepping of a window. | |
void | elm_win_layer_set (Evas_Object *obj, int layer) |
Sets the layer of the window. | |
int | elm_win_layer_get (const Evas_Object *obj) |
Gets the layer of the window. | |
void | elm_win_norender_push (Evas_Object *obj) |
Pushes (incriments) the norender counter on the window. | |
void | elm_win_norender_pop (Evas_Object *obj) |
Pops (decrements) the norender counter on the window. | |
int | elm_win_norender_get (Evas_Object *obj) |
Returns the number of times norender has been pushed on the window. | |
void | elm_win_render (Evas_Object *obj) |
Manually asks evas to render the window immediately. | |
void | elm_win_rotation_set (Evas_Object *obj, int rotation) |
Sets the rotation of the window. | |
void | elm_win_rotation_with_resize_set (Evas_Object *obj, int rotation) |
Rotates the window and resizes it. | |
int | elm_win_rotation_get (const Evas_Object *obj) |
Gets the rotation of the window. | |
void | elm_win_sticky_set (Evas_Object *obj, Eina_Bool sticky) |
Sets the sticky state of the window. | |
Eina_Bool | elm_win_sticky_get (const Evas_Object *obj) |
Gets the sticky state of the window. | |
void | elm_win_conformant_set (Evas_Object *obj, Eina_Bool conformant) |
Sets whether this window is an illume conformant window. | |
Eina_Bool | elm_win_conformant_get (const Evas_Object *obj) |
Gets whether this window is an illume conformant window. | |
void | elm_win_quickpanel_set (Evas_Object *obj, Eina_Bool quickpanel) |
Sets a window to be an illume quickpanel window. | |
Eina_Bool | elm_win_quickpanel_get (const Evas_Object *obj) |
Gets whether this window is a quickpanel. | |
void | elm_win_quickpanel_priority_major_set (Evas_Object *obj, int priority) |
Sets the major priority of a quickpanel window. | |
int | elm_win_quickpanel_priority_major_get (const Evas_Object *obj) |
Gets the major priority of a quickpanel window. | |
void | elm_win_quickpanel_priority_minor_set (Evas_Object *obj, int priority) |
Sets the minor priority of a quickpanel window. | |
int | elm_win_quickpanel_priority_minor_get (const Evas_Object *obj) |
Gets the minor priority of a quickpanel window. | |
void | elm_win_quickpanel_zone_set (Evas_Object *obj, int zone) |
Sets the zone in which this quickpanel should appear. | |
int | elm_win_quickpanel_zone_get (const Evas_Object *obj) |
Gets the zone in which this should appear. | |
void | elm_win_prop_focus_skip_set (Evas_Object *obj, Eina_Bool skip) |
Sets the window to be skipped by keyboard focus. | |
void | elm_win_illume_command_send (Evas_Object *obj, Elm_Illume_Command command, void *params) |
Sends a command to the windowing environment. | |
Evas_Object * | elm_win_inlined_image_object_get (Evas_Object *obj) |
Gets the inlined image object handle. | |
Eina_Bool | elm_win_focus_get (const Evas_Object *obj) |
Determines whether a window has focus. | |
void | elm_win_screen_constrain_set (Evas_Object *obj, Eina_Bool constrain) |
Limits the maximum width and height of a window to the width and height of its screen. | |
Eina_Bool | elm_win_screen_constrain_get (Evas_Object *obj) |
Retrieves the constraints on the maximum width and height of a window relative to the width and height of its screen. | |
void | elm_win_screen_size_get (const Evas_Object *obj, int *x, int *y, int *w, int *h) |
Gets the screen geometry details for the screen that a window is on. | |
void | elm_win_screen_dpi_get (const Evas_Object *obj, int *xdpi, int *ydpi) |
Gets the screen dpi for the screen that a window is on. | |
void | elm_win_focus_highlight_enabled_set (Evas_Object *obj, Eina_Bool enabled) |
Sets the enabled status for the focus highlight in a window. | |
Eina_Bool | elm_win_focus_highlight_enabled_get (const Evas_Object *obj) |
Gets the enabled value of the focus highlight for this window. | |
void | elm_win_focus_highlight_style_set (Evas_Object *obj, const char *style) |
Sets the style for the focus highlight on this window. | |
const char * | elm_win_focus_highlight_style_get (const Evas_Object *obj) |
Gets the style set for the focus highlight object. | |
void | elm_win_keyboard_mode_set (Evas_Object *obj, Elm_Win_Keyboard_Mode mode) |
Sets the keyboard mode of the window. | |
Elm_Win_Keyboard_Mode | elm_win_keyboard_mode_get (const Evas_Object *obj) |
Gets the keyboard mode of the window. | |
void | elm_win_keyboard_win_set (Evas_Object *obj, Eina_Bool is_keyboard) |
Sets whether the window is a keyboard. | |
Eina_Bool | elm_win_keyboard_win_get (const Evas_Object *obj) |
Gets whether the window is a keyboard. | |
void | elm_win_indicator_mode_set (Evas_Object *obj, Elm_Win_Indicator_Mode mode) |
Sets the indicator mode of the window. | |
Elm_Win_Indicator_Mode | elm_win_indicator_mode_get (const Evas_Object *obj) |
Gets the indicator mode of the window. | |
void | elm_win_indicator_opacity_set (Evas_Object *obj, Elm_Win_Indicator_Opacity_Mode mode) |
Sets the indicator opacity mode of the window. | |
Elm_Win_Indicator_Opacity_Mode | elm_win_indicator_opacity_get (const Evas_Object *obj) |
Gets the indicator opacity mode of the window. | |
void | elm_win_screen_position_get (const Evas_Object *obj, int *x, int *y) |
Get the screen position of a window. | |
Eina_Bool | elm_win_socket_listen (Evas_Object *obj, const char *svcname, int svcnum, Eina_Bool svcsys) |
Creates a socket to provide the service for a Plug widget. | |
Eina_Bool | elm_win_trap_set (const Elm_Win_Trap *trap) |
Sets the trap to be used for internal Ecore_Evas management. | |
void | elm_win_floating_mode_set (Evas_Object *obj, Eina_Bool floating) |
Sets the floating mode of a window. | |
Eina_Bool | elm_win_floating_mode_get (const Evas_Object *obj) |
Gets the floating mode of a window. | |
Eina_Bool | elm_win_wm_rotation_supported_get (const Evas_Object *obj) |
Queries whether Windows Manager supports window rotation. | |
void | elm_win_wm_rotation_preferred_rotation_set (Evas_Object *obj, const int rotation) |
Set the preferred rotation value. | |
int | elm_win_wm_rotation_preferred_rotation_get (const Evas_Object *obj) |
Gets the preferred rotation value. | |
void | elm_win_wm_rotation_available_rotations_set (Evas_Object *obj, const int *rotations, unsigned int count) |
Sets the array of available window rotations. | |
Eina_Bool | elm_win_wm_rotation_available_rotations_get (const Evas_Object *obj, int **rotations, unsigned int *count) |
Gets the array of available window rotations. | |
void | elm_win_wm_rotation_manual_rotation_done_set (Evas_Object *obj, Eina_Bool set) |
Sets the manual rotation done mode of the window. | |
Eina_Bool | elm_win_wm_rotation_manual_rotation_done_get (const Evas_Object *obj) |
Gets the manual rotation done mode of the window. | |
void | elm_win_wm_rotation_manual_rotation_done (Evas_Object *obj) |
Sets the rotation finish manually. | |
Typedefs | |
typedef struct _Elm_Win_Trap | Elm_Win_Trap |
Trap can be set with elm_win_trap_set() and intercepts the calls to internal ecore_evas with the same name and parameters. If there is a trap and it returns EINA_TRUE then the call is allowed, otherwise it is ignored. |
Typedef Documentation
Trap can be set with elm_win_trap_set() and intercepts the calls to internal ecore_evas with the same name and parameters. If there is a trap and it returns EINA_TRUE
then the call is allowed, otherwise it is ignored.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
Enumeration Type Documentation
enum Elm_Illume_Command |
Enumeration that defines the available commands that can be sent to the Illume manager.
- Since :
- 2.3.1
- Remarks:
- When running under an Illume session, a window may send commands to the Illume manager to perform different actions.
Enumeration that defines the different indicator states.
- Since :
- 2.3.1
- Remarks:
- In some environments, like phones, you may have an indicator that shows the battery status, reception, time etc. This is the indicator.
-
Sometimes you don't want it because you provide the same functionality inside your app, so this requests that the indicator be hidden in such a circumstance if you use
ELM_ILLUME_INDICATOR_HIDE
. The default is to have the indicator shown.
Enumeration that defines the opacity modes of an indicator that can be shown.
- Since :
- 2.3.1
- Enumerator:
Enumeration that defines the type modes of an indicator that can be shown.
- Since :
- 2.3.1
- Remarks:
- If the indicator can support several types, you can use this enum value to deal with different types of indicators.
Enumeration that defines the different layouts that can be requested for the virtual keyboard.
- Since :
- 2.3.1
- Remarks:
- When the application window is being managed by Illume, it may request any of the following layouts for the virtual keyboard.
- Enumerator:
enum Elm_Win_Type |
Enumeration that defines the types of windows that can be created.
- Since :
- 2.3.1
- Remarks:
- These are hints set on the window so that a running Windows Manager knows how the window should be handled and/or what kind of decorations it should have.
- Currently, only the X11 backed engines use them.
- Enumerator:
ELM_WIN_UNKNOWN Unknown window type
ELM_WIN_BASIC A normal window. It indicates a normal, top-level window. Almost every window is created with this type
ELM_WIN_DIALOG_BASIC Used for simple dialog windows
ELM_WIN_DESKTOP Used for special desktop windows, like a background window holding desktop icons
ELM_WIN_DOCK The window is used as a dock or panel. Usually would be kept on top of any other window by the Windows Manager
ELM_WIN_TOOLBAR The window is used to hold a floating toolbar, or something similar
ELM_WIN_MENU This is similar to ELM_WIN_TOOLBAR
ELM_WIN_UTILITY A persistent utility window, like a toolbox or palette
ELM_WIN_SPLASH A splash window for an application that is starting up
ELM_WIN_DROPDOWN_MENU The window is a dropdown menu, when an entry in a menubar is clicked. Typically used with elm_win_override_set(). This hint exists for completion only, as the EFL way of implementing a menu would not normally use a separate window for its contents
ELM_WIN_POPUP_MENU Like ELM_WIN_DROPDOWN_MENU, but used for the menu triggered by right-clicking an object
ELM_WIN_TOOLTIP The window is a tooltip. A short piece of explanatory text that typically appears after the mouse cursor hovers over an object for a while. Typically used with elm_win_override_set() and also not very commonly used in EFL
ELM_WIN_NOTIFICATION A notification window, like a warning about battery life or a new email that is received
ELM_WIN_COMBO A window holding the contents of a combo box. Not usually used in EFL
ELM_WIN_DND Used to indicate that the window is a representation of an object being dragged across different windows, or even applications. Typically used with elm_win_override_set()
ELM_WIN_INLINED_IMAGE The window is rendered onto an image buffer. No actual window is created for this type, instead the window and all of its contents are rendered to an image buffer. This allows to have a children window inside a parent one just like any other object would be, and do other things like applying
Evas_Map
effects to it. This is the only type of window that requires the parent parameter of elm_win_add() to be a validEvas_Object
ELM_WIN_SOCKET_IMAGE The window is rendered onto an image buffer and can be shown another process's plug image object. No actual window is created for this type, instead the window and all of its contents are rendered to an image buffer and can be shown another process's plug image object
Function Documentation
void elm_win_activate | ( | Evas_Object * | obj | ) |
Activates the window object.
This function sends a request to the Windows Manager to activate the window pointed by obj. If honored by the WM, the window receives the keyboard focus.
- Since :
- 2.3.1
- Remarks:
- This is just a request that a Window Manager may ignore, so calling this function does not ensure in any way that the window is going to be the active one after it.
- Parameters:
-
[in] obj The window object
Evas_Object* elm_win_add | ( | Evas_Object * | parent, |
const char * | name, | ||
Elm_Win_Type | type | ||
) |
Adds a window object. If this is the first window created, pass NULL
as parent.
- Since :
- 2.3.1
- Remarks:
- The parent parameter can be
NULL
for every window type exceptELM_WIN_INLINED_IMAGE
, which needs a parent to retrieve the canvas on which the image object is created.
- Parameters:
-
[in] parent The parent object to add the window to, otherwise NULL
[in] name The name of the window [in] type The window type, one from Elm_Win_Type
- Returns:
- The created object, otherwise
NULL
on failure
Eina_Bool elm_win_alpha_get | ( | const Evas_Object * | obj | ) |
Gets the alpha channel state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window has an alpha channel, otherwisefalse
void elm_win_alpha_set | ( | Evas_Object * | obj, |
Eina_Bool | alpha | ||
) |
Sets the alpha channel state of a window.
- Since :
- 2.3.1
- Remarks:
- If alpha is
EINA_TRUE
, the alpha channel of the canvas is enabled possibly making parts of the window completely or partially transparent. This is also subject to the underlying system supporting it, like for example, running under a compositing manager. If no compositing is available, enabling this option results in using shaped windows with elm_win_shaped_set().
- Parameters:
-
[in] obj The window object [in] alpha If true
the window has an alpha channel, otherwisefalse
- See also:
- elm_win_alpha_set()
double elm_win_aspect_get | ( | const Evas_Object * | obj | ) |
Gets the aspect ratio of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The set aspect ratio (
0
by default)
void elm_win_aspect_set | ( | Evas_Object * | obj, |
double | aspect | ||
) |
Sets the aspect ratio of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] aspect If 0
the window has no aspect limits, otherwise it is width divided by height
Eina_Bool elm_win_autodel_get | ( | const Evas_Object * | obj | ) |
Gets the window autodel state.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window automatically deletes itself when closed, otherwisefalse
- See also:
- elm_win_autodel_set()
void elm_win_autodel_set | ( | Evas_Object * | obj, |
Eina_Bool | autodel | ||
) |
Sets the window autodel state.
- Since :
- 2.3.1
- Remarks:
- When closing the window in any way outside of the program control, like pressing the X button in the titlebar or using a command from the Windows Manager, a
"delete,request"
signal is emitted to indicate that this event has occurred and the developer can take an action, which may or may not include destroying the window object. -
When the autodel parameter is set, the window is automatically destroyed when this event occurs, after the signal is emitted. If autodel is
EINA_FALSE
, then the window is not destroyed and it is up to the program to decide when to do so if required.
- Parameters:
-
[in] obj The window object [in] autodel If true
the window automatically deletes itself when closed, otherwisefalse
Eina_Bool elm_win_borderless_get | ( | const Evas_Object * | obj | ) |
Gets the borderless state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is borderless, otherwisefalse
void elm_win_borderless_set | ( | Evas_Object * | obj, |
Eina_Bool | borderless | ||
) |
Sets the borderless state of a window.
This function requests the Windows Manager to not draw any decoration around the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] borderless If true
the window is borderless, otherwisefalse
void elm_win_center | ( | Evas_Object * | obj, |
Eina_Bool | h, | ||
Eina_Bool | v | ||
) |
Centers a window on its screen.
This function centers a window obj horizontally and/or vertically based on the values of h and v.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] h If true
it centers horizontally, otherwisefalse
if it does not change the horizontal location[in] v If true
it centers vertically, otherwisefalse
if it does not change the vertical location
Eina_Bool elm_win_conformant_get | ( | const Evas_Object * | obj | ) |
Gets whether this window is an illume conformant window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The boolean value that indicates whether this window is illume conformant
void elm_win_conformant_set | ( | Evas_Object * | obj, |
Eina_Bool | conformant | ||
) |
Sets whether this window is an illume conformant window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] conformant The conformant flag ( 1
= conformant,0
= non-conformant)
Eina_Bool elm_win_demand_attention_get | ( | const Evas_Object * | obj | ) |
Gets the demand_attention state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is in the demand_attention state, otherwisefalse
void elm_win_demand_attention_set | ( | Evas_Object * | obj, |
Eina_Bool | demand_attention | ||
) |
Sets the demand_attention state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] demand_attention If true
the window is in the demand_attention state, otherwisefalse
Eina_Bool elm_win_floating_mode_get | ( | const Evas_Object * | obj | ) |
Gets the floating mode of a window.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is in the floating mode, otherwisefalse
- See also:
- elm_win_floating_mode_set()
void elm_win_floating_mode_set | ( | Evas_Object * | obj, |
Eina_Bool | floating | ||
) |
Sets the floating mode of a window.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] floating If true
the window is in the floating mode, otherwisefalse
- See also:
- elm_win_floating_mode_get()
Eina_Bool elm_win_focus_get | ( | const Evas_Object * | obj | ) |
Determines whether a window has focus.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window to query
- Returns:
EINA_TRUE
if the window exists and has focus, otherwiseEINA_FALSE
Eina_Bool elm_win_focus_highlight_enabled_get | ( | const Evas_Object * | obj | ) |
Gets the enabled value of the focus highlight for this window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window in which to check if the focus highlight is enabled
- Returns:
EINA_TRUE
if enabled, otherwiseEINA_FALSE
void elm_win_focus_highlight_enabled_set | ( | Evas_Object * | obj, |
Eina_Bool | enabled | ||
) |
Sets the enabled status for the focus highlight in a window.
This function enables or disables the focus highlight only for the given window, regardless of the global setting for it.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window for which to enable highlight [in] enabled The enabled value for the highlight
const char* elm_win_focus_highlight_style_get | ( | const Evas_Object * | obj | ) |
Gets the style set for the focus highlight object.
This gets the style set for this windows highlight object, otherwise NULL
if no style is set.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window from which to retrieve the highlight style
- Returns:
- The style set, otherwise
NULL
if no style is set
The default value is used in that case.
void elm_win_focus_highlight_style_set | ( | Evas_Object * | obj, |
const char * | style | ||
) |
Sets the style for the focus highlight on this window.
This sets the style to use for theming the highlight of focused objects on the given window. If style is NULL
, the default value is used.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window for which to set the style [in] style The style to set
Eina_Bool elm_win_fullscreen_get | ( | const Evas_Object * | obj | ) |
Geta the fullscreen state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is fullscreen, otherwisefalse
void elm_win_fullscreen_set | ( | Evas_Object * | obj, |
Eina_Bool | fullscreen | ||
) |
Sets the fullscreen state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object fullscreen If true
the window is fullscreen, otherwisefalse
const char* elm_win_icon_name_get | ( | const Evas_Object * | obj | ) |
Gets the icon name of the window.
- Since :
- 2.3.1
- Remarks:
- The returned string is an internal one and should not be freed or modified. It should also be rendered invalid if a new icon name is set or if the window is destroyed.
- Parameters:
-
[in] obj The window object
- Returns:
- The icon name
void elm_win_icon_name_set | ( | Evas_Object * | obj, |
const char * | icon_name | ||
) |
Sets the icon name of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] icon_name The icon name to set
const Evas_Object* elm_win_icon_object_get | ( | const Evas_Object * | obj | ) |
Gets the icon object used for the window.
- Since :
- 2.3.1
- Remarks:
- The object returned is the one marked by elm_win_icon_object_set() as the object to use for the window icon.
- Parameters:
-
[in] obj The window object
- Returns:
- The icon object to set
void elm_win_icon_object_set | ( | Evas_Object * | obj, |
Evas_Object * | icon | ||
) |
Sets a window object icon.
This sets an image to be used as the icon for the given window, in the Windows Manager decoration part. The exact pixel dimensions of the object (not object size) is used, and the image pixels are used as-is when this function is called. If the image object has been updated, then call this function again to source the image pixels and put them on the window's icon. Note that only Evas image objects are allowed.
- Since :
- 2.3.1
- Remarks:
- Example of usage:
icon = evas_object_image_add(evas_object_evas_get(elm_window)); evas_object_image_file_set(icon, "/path/to/the/icon", NULL); elm_win_icon_object_set(elm_window, icon); evas_object_show(icon);
- Parameters:
-
[in] obj The window object used to get an icon [in] icon The Evas image object used as an icon
Eina_Bool elm_win_iconified_get | ( | const Evas_Object * | obj | ) |
Gets the iconified state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is iconified, otherwisefalse
void elm_win_iconified_set | ( | Evas_Object * | obj, |
Eina_Bool | iconified | ||
) |
Sets the iconified state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] iconified If true
the window is iconified, otherwisefalse
void elm_win_illume_command_send | ( | Evas_Object * | obj, |
Elm_Illume_Command | command, | ||
void * | params | ||
) |
Sends a command to the windowing environment.
- Since :
- 2.3.1
- Remarks:
- This is intended to work in touchscreen or small screen device environments where there is a more simplistic window management policy in place. This uses the window object indicated to select which part of the environment to control (the part that this window lives in), and provides a command and an optional parameter structure (use
NULL
for this if not needed).
- Parameters:
-
[in] obj The window object that lives in the environment to control [in] command The command to send [in] params The optional parameters for the command
Elm_Win_Indicator_Mode elm_win_indicator_mode_get | ( | const Evas_Object * | obj | ) |
Gets the indicator mode of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The mode, one from Elm_Win_Indicator_Mode
void elm_win_indicator_mode_set | ( | Evas_Object * | obj, |
Elm_Win_Indicator_Mode | mode | ||
) |
Sets the indicator mode of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] mode The mode to set, one from Elm_Win_Indicator_Mode
Gets the indicator opacity mode of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The mode, one from Elm_Win_Indicator_Opacity_Mode
void elm_win_indicator_opacity_set | ( | Evas_Object * | obj, |
Elm_Win_Indicator_Opacity_Mode | mode | ||
) |
Sets the indicator opacity mode of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] mode The mode to set, one from Elm_Win_Indicator_Opacity_Mode
Gets the inlined image object handle.
- Since :
- 2.3.1
- Remarks:
- When you create a window with elm_win_add() of type
ELM_WIN_INLINED_IMAGE
, then the window is in fact an evas image object inlined in the parent canvas. You can get this object (be careful to not manipulate it as it is under the control of elementary), and use it to do things like get pixel data, save the image to a file, etc.
- Parameters:
-
[in] obj The window object from which to get the inlined image
- Returns:
- The inlined image object, otherwise
NULL
if none exist
Elm_Win_Keyboard_Mode elm_win_keyboard_mode_get | ( | const Evas_Object * | obj | ) |
Gets the keyboard mode of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The mode, one from Elm_Win_Keyboard_Mode
void elm_win_keyboard_mode_set | ( | Evas_Object * | obj, |
Elm_Win_Keyboard_Mode | mode | ||
) |
Sets the keyboard mode of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] mode The mode to set, one from Elm_Win_Keyboard_Mode
Eina_Bool elm_win_keyboard_win_get | ( | const Evas_Object * | obj | ) |
Gets whether the window is a keyboard.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is a virtual keyboard, otherwisefalse
void elm_win_keyboard_win_set | ( | Evas_Object * | obj, |
Eina_Bool | is_keyboard | ||
) |
Sets whether the window is a keyboard.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] is_keyboard If true
the window is a virtual keyboard, otherwisefalse
int elm_win_layer_get | ( | const Evas_Object * | obj | ) |
Gets the layer of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The layer of the window
- See also:
- elm_win_layer_set()
void elm_win_layer_set | ( | Evas_Object * | obj, |
int | layer | ||
) |
Sets the layer of the window.
- Since :
- 2.3.1
- Remarks:
- What this means exactly depends on the underlying engine used.
-
In case of X11 backed engines, the value in layer has one of the following meanings:
- < 3: The window is placed below all others.
- > 5: The window is placed above all others.
- other: The window is placed in the default layer.
- Parameters:
-
[in] obj The window object [in] layer The layer of the window
void elm_win_lower | ( | Evas_Object * | obj | ) |
Lowers the window object.
- Since :
- 2.3.1
- Remarks:
- Places the window pointed by obj at the bottom of the stack, so that no other window is covered by it.
- If elm_win_override_set() is not set, the Windows Manager may ignore this request.
- Parameters:
-
[in] obj The window object
Eina_Bool elm_win_maximized_get | ( | const Evas_Object * | obj | ) |
Gets the maximized state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is maximized, otherwisefalse
void elm_win_maximized_set | ( | Evas_Object * | obj, |
Eina_Bool | maximized | ||
) |
Sets the maximized state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] maximized If true
the window is maximized, otherwisefalse
Eina_Bool elm_win_modal_get | ( | const Evas_Object * | obj | ) |
Gets the modal state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- If
true
the window is modal, otherwisefalse
void elm_win_modal_set | ( | Evas_Object * | obj, |
Eina_Bool | modal | ||
) |
Sets the modal state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] modal If true
the window is modal, otherwisefalse
int elm_win_norender_get | ( | Evas_Object * | obj | ) |
Returns the number of times norender has been pushed on the window.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The number of times norender has been pushed
void elm_win_norender_pop | ( | Evas_Object * | obj | ) |
Pops (decrements) the norender counter on the window.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- Once norender has gone back to
0
, automatic rendering continues in the given window. If it is already at0
, this has no effect.
- Parameters:
-
[in] obj The window object
void elm_win_norender_push | ( | Evas_Object * | obj | ) |
Pushes (incriments) the norender counter on the window.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- There are some occasions where you wish to suspend rendering on a window. You may be "sleeping" and may have nothing to update and do not want animations or other theme side-effects causing rendering to the window while "asleep". You can push (and pop) the norender mode to make this work.
- If combined with evas_render_dump(), you can minimize memory footprint significantly while "asleep", and the pausing of rendering ensures that evas does not re-load data into memory until needed. When rendering is resumed, data is re-loaded as needed, which may result in some lag, but does save memory.
- Parameters:
-
[in] obj The window object
Eina_Bool elm_win_override_get | ( | const Evas_Object * | obj | ) |
Gets the override state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is overridden, otherwisefalse
- See also:
- elm_win_override_set()
void elm_win_override_set | ( | Evas_Object * | obj, |
Eina_Bool | override | ||
) |
Sets the override state of a window.
- Since :
- 2.3.1
- Remarks:
- A window with override set to
EINA_TRUE
is not managed by the Windows Manager. This means that no decorations of any kind are shown for it, moving and resizing must be handled by the application as well as the window visibility. - This should not be used for normal windows, and even for not so normal ones, it should only be used with a lot of care and when there's a good reason. Mishandling override windows may result in situations that disrupt the normal workflow of the end user.
- Parameters:
-
[in] obj The window object [in] override If true
the window is overridden, otherwisefalse
const char* elm_win_profile_get | ( | const Evas_Object * | obj | ) |
Gets the profile of a window.
- Since :
- 2.3.1
- Remarks:
- The returned string is an internal one and should not be freed or modified. It is also rendered invalid if a new role is set or if the window is destroyed.
- Parameters:
-
[in] obj The window object
- Returns:
- The profile name
void elm_win_profiles_set | ( | Evas_Object * | obj, |
const char ** | profiles, | ||
unsigned int | num_profiles | ||
) |
Sets the profile list of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] profiles The list of profile names [in] num_profiles The number of profile names
void elm_win_prop_focus_skip_set | ( | Evas_Object * | obj, |
Eina_Bool | skip | ||
) |
Sets the window to be skipped by keyboard focus.
This sets the window to be skipped by normal keyboard input. This means the Windows Manager is asked to not focus this window as well as omit it from things like the taskbar, pager, "alt-tab" list, etc.
- Since :
- 2.3.1
- Remarks:
- Call this and enable it on a window BEFORE you show it for the first time, otherwise it may have no effect.
- Use this for windows that have only output information or might only be interacted with by a mouse or fingers, and never for typing input. Be careful that this may have side-effects like making the window non-accessible in some cases unless the window is specially handled. Use this with care.
- Parameters:
-
[in] obj The window object [in] skip The skip flag state ( EINA_TRUE
if it is to be skipped)
Eina_Bool elm_win_quickpanel_get | ( | const Evas_Object * | obj | ) |
Gets whether this window is a quickpanel.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- A boolean value that indicates whether this window is a quickpanel
int elm_win_quickpanel_priority_major_get | ( | const Evas_Object * | obj | ) |
Gets the major priority of a quickpanel window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The major priority of this quickpanel
void elm_win_quickpanel_priority_major_set | ( | Evas_Object * | obj, |
int | priority | ||
) |
Sets the major priority of a quickpanel window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] priority The major priority for this quickpanel
int elm_win_quickpanel_priority_minor_get | ( | const Evas_Object * | obj | ) |
Gets the minor priority of a quickpanel window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The minor priority of this quickpanel
void elm_win_quickpanel_priority_minor_set | ( | Evas_Object * | obj, |
int | priority | ||
) |
Sets the minor priority of a quickpanel window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] priority The minor priority for this quickpanel
void elm_win_quickpanel_set | ( | Evas_Object * | obj, |
Eina_Bool | quickpanel | ||
) |
Sets a window to be an illume quickpanel window.
- Since :
- 2.3.1
- Remarks:
- By default, window objects are not quickpanel windows.
- Parameters:
-
[in] obj The window object [in] quickpanel The quickpanel flag ( 1
= quickpanel,0
= normal window)
int elm_win_quickpanel_zone_get | ( | const Evas_Object * | obj | ) |
Gets the zone in which this should appear.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The requested zone for this quickpanel
void elm_win_quickpanel_zone_set | ( | Evas_Object * | obj, |
int | zone | ||
) |
Sets the zone in which this quickpanel should appear.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] zone The requested zone for this quickpanel
void elm_win_raise | ( | Evas_Object * | obj | ) |
Raises the window object.
- Since :
- 2.3.1
- Remarks:
- Places the window pointed by obj at the top of the stack, so that it's not covered by any other window.
- If elm_win_override_set() is not set, the Windows Manager may ignore this request.
- Parameters:
-
[in] obj The window object
void elm_win_render | ( | Evas_Object * | obj | ) |
Manually asks evas to render the window immediately.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- You should NEVER call this unless you really know what you are doing and why. Never call this unless you are asking for performance degredation and possibly weird behavior. Windows gets automatically rendered when the application goes idle so there is never a need to call this UNLESS you have enabled the "norender" mode.
- Parameters:
-
[in] obj The window object
void elm_win_resize_object_add | ( | Evas_Object * | obj, |
Evas_Object * | subobj | ||
) |
Adds subobj as a resize object of the window obj.
- Since :
- 2.3.1
- Remarks:
- Setting an object as a resize object of the window means that the subobj child's size and position is controlled by the window directly. That is, the object is resized to match the window size and should never be moved or resized manually by the developer.
- In addition, resize objects of the window control the minimum size of it as well as whether it can or cannot be resized by the user.
- For the end user to be able to resize a window by dragging the handles or borders provided by the Windows Manager, or using any other similar mechanism, all of the resize objects in the window should have their evas_object_size_hint_weight_set() set to EVAS_HINT_EXPAND.
- Also notice that the window can get resized to the current size of the object if EVAS_HINT_EXPAND is set after the call to elm_win_resize_object_add(). So if the object should get resized to the size of the window, set this hint before adding it as a resize object (this happens because the size of the window and the object are evaluated as soon as the object is added to the window).
- Parameters:
-
[in] obj The window object [in] subobj The resize object to add
void elm_win_resize_object_del | ( | Evas_Object * | obj, |
Evas_Object * | subobj | ||
) |
Deletes subobj as a resize object of the window obj.
This function removes the object subobj from the resize objects of the window obj. It does not delete the object itself, which is left unmanaged and should be deleted by the developer, manually handled or set as the child of some other container.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] subobj The resize object to add
const char* elm_win_role_get | ( | const Evas_Object * | obj | ) |
Gets the role of the window.
- Since :
- 2.3.1
- Remarks:
- The returned string is an internal one and should not be freed or modified. It should also be rendered invalid if a new role is set or if the window is destroyed.
- Parameters:
-
[in] obj The window object
- Returns:
- The role
void elm_win_role_set | ( | Evas_Object * | obj, |
const char * | role | ||
) |
Sets the role of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] role The role to set
int elm_win_rotation_get | ( | const Evas_Object * | obj | ) |
Gets the rotation of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The rotation of the window in degrees (0-360)
void elm_win_rotation_set | ( | Evas_Object * | obj, |
int | rotation | ||
) |
Sets the rotation of the window.
- Since :
- 2.3.1
- Remarks:
- Most engines only work with multiples of
90
. - This function is used to set the orientation of the window obj to match that of the screen. The window itself is resized to adjust to the new geometry of its contents. If you want to keep the window size,
- Parameters:
-
[in] obj The window object [in] rotation The rotation of the window, in degrees (0-360), counter-clockwise
- See also:
- elm_win_rotation_with_resize_set()
void elm_win_rotation_with_resize_set | ( | Evas_Object * | obj, |
int | rotation | ||
) |
Rotates the window and resizes it.
- Since :
- 2.3.1
- Remarks:
- Like elm_win_rotation_set(), but it also resizes the window's contents so that they fit inside the current window geometry.
- Parameters:
-
[in] obj The window object [in] rotation The rotation of the window, in degrees (0-360), counter-clockwise
Retrieves the constraints on the maximum width and height of a window relative to the width and height of its screen.
- Since :
- 2.3.1
- Remarks:
- When this function returns
true
, obj never resizes to a size larger than the screen.
- Parameters:
-
[in] obj The window object
- Returns:
EINA_TRUE
to restrict the window's maximum size, otherwiseEINA_FALSE
to disable restriction
void elm_win_screen_constrain_set | ( | Evas_Object * | obj, |
Eina_Bool | constrain | ||
) |
Limits the maximum width and height of a window to the width and height of its screen.
- Since :
- 2.3.1
- Remarks:
- When constrain is
true
, obj never resizes to a size larger than the screen.
- Parameters:
-
[in] obj The window object [in] constrain EINA_TRUE
to restrict the window's maximum size, otherwiseEINA_FALSE
to disable restriction
void elm_win_screen_dpi_get | ( | const Evas_Object * | obj, |
int * | xdpi, | ||
int * | ydpi | ||
) |
Gets the screen dpi for the screen that a window is on.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window to query [out] xdpi The pointer to the value that stores the returned horizontal dpi
This may beNULL
.[out] ydpi The pointer to the value that stores the returned vertical dpi
This may beNULL
.
void elm_win_screen_position_get | ( | const Evas_Object * | obj, |
int * | x, | ||
int * | y | ||
) |
Get the screen position of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [out] x The integer that stores the x coordinate [out] y The integer that stores the y coordinate
void elm_win_screen_size_get | ( | const Evas_Object * | obj, |
int * | x, | ||
int * | y, | ||
int * | w, | ||
int * | h | ||
) |
Gets the screen geometry details for the screen that a window is on.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window to query [out] x The coordinate at which to return the horizontal offset value
This may beNULL
.[out] y The coordinate at which to return the vertical offset value
This may beNULL
.[out] w The coordinate at which to return the width value
This may beNULL
.[out] h The coordinate at which to return the height value
This may beNULL
.
Eina_Bool elm_win_shaped_get | ( | const Evas_Object * | obj | ) |
Gets the shaped state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is shaped, otherwisefalse
- See also:
- elm_win_shaped_set()
void elm_win_shaped_set | ( | Evas_Object * | obj, |
Eina_Bool | shaped | ||
) |
Sets the shaped state of a window.
- Since :
- 2.3.1
- Remarks:
- Shaped windows, when supported, render the parts of the window that has no content and is transparent.
-
If shaped is
EINA_FALSE
, then it is strongly advised to have some background object or cover the entire window in any other way, otherwise the parts of the canvas that have no data show framebuffer artifacts.
- Parameters:
-
[in] obj The window object [in] shaped If true
the window is shaped, otherwisefalse
- See also:
- elm_win_alpha_set()
void elm_win_size_base_get | ( | Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Gets the base size of a window.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [out] w The pointer that stores the returned base width [out] h The pointer that stores the returned base height
void elm_win_size_base_set | ( | Evas_Object * | obj, |
int | w, | ||
int | h | ||
) |
Sets the base window size used with stepping calculation.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- Base size + stepping is what is calculated for window sizing restrictions.
- Parameters:
-
[in] obj The window object [in] w The base width [in] h The base height
void elm_win_size_step_get | ( | Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Gets the stepping of a window.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [out] w The pointer that stores the returned stepping width [out] h The pointer that stores the returned stepping height
void elm_win_size_step_set | ( | Evas_Object * | obj, |
int | w, | ||
int | h | ||
) |
Sets the window stepping used with sizing calculation.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- Base size + stepping is what is calculated for window sizing restrictions.
- Parameters:
-
[in] obj The window object [in] w The stepping width ( 0
disables)[in] h The stepping height ( 0
disables)
Eina_Bool elm_win_socket_listen | ( | Evas_Object * | obj, |
const char * | svcname, | ||
int | svcnum, | ||
Eina_Bool | svcsys | ||
) |
Creates a socket to provide the service for a Plug widget.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] svcname The name of the service to be advertised
Ensure that it is unique (when combined with svcnum), otherwise the creation may fail.[in] svcnum A number (any value, 0
being the common default) to differentiate multiple instances of services with the same name[in] svcsys If true
it specifies to create a system-wide service that all users can connect to, otherwisefalse
if the service is private to the user ID that created the service
- Returns:
- The boolean value that indicates whether the socket creation is successful
Eina_Bool elm_win_sticky_get | ( | const Evas_Object * | obj | ) |
Gets the sticky state of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- If
true
the window's sticky state is enabled, otherwisefalse
- See also:
- elm_win_sticky_set()
void elm_win_sticky_set | ( | Evas_Object * | obj, |
Eina_Bool | sticky | ||
) |
Sets the sticky state of the window.
- Since :
- 2.3.1
- Remarks:
- Hints the Windows Manager that the window in obj should be left fixed at its position even when the virtual desktop that it's on moves or changes.
- Parameters:
-
[in] obj The window object [in] sticky If true
the window's sticky state is enabled, otherwisefalse
const char* elm_win_title_get | ( | const Evas_Object * | obj | ) |
Gets the title of the window.
- Since :
- 2.3.1
- Remarks:
- The returned string is an internal one and should not be freed or modified. It should also be rendered invalid if a new title is set or if the window is destroyed.
- Parameters:
-
[in] obj The window object
- Returns:
- The title
void elm_win_title_set | ( | Evas_Object * | obj, |
const char * | title | ||
) |
Sets the title of the window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] title The title to set
Eina_Bool elm_win_trap_set | ( | const Elm_Win_Trap * | trap | ) |
Sets the trap to be used for internal Ecore_Evas
management.
- Since (EFL) :
- 1.7
- Since :
- 2.3.1
- Remarks:
- This is an advanced feature that you should avoid using.
- Parameters:
-
[in] trap The trap to be used, otherwise NULL
to remove traps
The pointer is not modified or copied, it is kept alive.
- Returns:
EINA_TRUE
on success, otherwiseEINA_FALSE
if there is a problem, such as an invalid version number
Elm_Win_Type elm_win_type_get | ( | Evas_Object * | obj | ) |
Gets the type of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object for which to get the type
- Returns:
- The type of a window object
If the object is not a window object, return-1
.
Eina_Bool elm_win_urgent_get | ( | const Evas_Object * | obj | ) |
Gets the urgent state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is urgent, otherwisefalse
void elm_win_urgent_set | ( | Evas_Object * | obj, |
Eina_Bool | urgent | ||
) |
Sets the urgent state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] urgent If true
the window is urgent, otherwisefalse
Evas_Object* elm_win_util_standard_add | ( | const char * | name, |
const char * | title | ||
) |
Adds a window object with a standard setup.
- Since :
- 2.3.1
- Remarks:
- This creates a window like elm_win_add(), but also puts in a standard background using elm_bg_add() as well as setting the window title to title. The window type created is of type
ELM_WIN_BASIC
withNULL
as the parent widget.
- Parameters:
-
[in] name The name of the window [in] title The title of the window
- Returns:
- The created object, otherwise
NULL
on failure
- See also:
- elm_win_add()
Eina_Bool elm_win_withdrawn_get | ( | const Evas_Object * | obj | ) |
Gets the withdrawn state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the window is withdrawn, otherwisefalse
void elm_win_withdrawn_set | ( | Evas_Object * | obj, |
Eina_Bool | withdrawn | ||
) |
Sets the withdrawn state of a window.
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] withdrawn If true
the window is withdrawn, otherwisefalse
Eina_Bool elm_win_wm_rotation_available_rotations_get | ( | const Evas_Object * | obj, |
int ** | rotations, | ||
unsigned int * | count | ||
) |
Gets the array of available window rotations.
This function is used to get the available rotations.
- Since (EFL) :
- 1.9
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [out] rotations The array of rotation values [out] count The number of arrays of rotations
- Returns:
- EINA_TRUE if succeed, otherwise EINA_FALSE
void elm_win_wm_rotation_available_rotations_set | ( | Evas_Object * | obj, |
const int * | rotations, | ||
unsigned int | count | ||
) |
Sets the array of available window rotations.
This function is used to set the available rotations to give hints to WM. WM refers these hints and sets the orientation of the window properly.
- Since (EFL) :
- 1.9
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] rotations The array of rotation values [in] count The number of arrays of rotations
void elm_win_wm_rotation_manual_rotation_done | ( | Evas_Object * | obj | ) |
Sets the rotation finish manually.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
Eina_Bool elm_win_wm_rotation_manual_rotation_done_get | ( | const Evas_Object * | obj | ) |
Gets the manual rotation done mode of the window.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
true
if the manual rotation done mode is enabled, otherwisefalse
void elm_win_wm_rotation_manual_rotation_done_set | ( | Evas_Object * | obj, |
Eina_Bool | set | ||
) |
Sets the manual rotation done mode of the window.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] set If true
, the Windows Manager does not rotate the window until the rotation done event is received by elm_win_wm_rotation_manual_rotation_done, otherwisefalse
if the manual rotation mode is disabled
int elm_win_wm_rotation_preferred_rotation_get | ( | const Evas_Object * | obj | ) |
Gets the preferred rotation value.
This function is used to get the preferred rotation value.
- Since (EFL) :
- 1.9
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object
- Returns:
- The preferred rotation of the window, in degrees (0-360), counter-clockwise
void elm_win_wm_rotation_preferred_rotation_set | ( | Evas_Object * | obj, |
const int | rotation | ||
) |
Set the preferred rotation value.
This function is used to set the orientation of window obj
to spicific angle fixed.
- Since (EFL) :
- 1.9
- Since :
- 2.3.1
- Parameters:
-
[in] obj The window object [in] rotation The preferred rotation of the window in degrees (0-360), counter-clockwise.
Eina_Bool elm_win_wm_rotation_supported_get | ( | const Evas_Object * | obj | ) |
Queries whether Windows Manager supports window rotation.
- Since (EFL) :
- 1.9
- Since :
- 2.3.1
- Remarks:
- The Windows Manager rotation allows the WM to control the rotation of application windows. It is designed to support synchronized rotation for multiple application windows at the same time.
- Parameters:
-
[in] obj The window object
- Returns:
EINA_TRUE
if the Windows Manager rotation is supported, otherwiseEINA_FALSE
- See also:
- elm_win_wm_rotation_supported_get()
- elm_win_wm_rotation_preferred_rotation_set()
- elm_win_wm_rotation_preferred_rotation_get()
- elm_win_wm_rotation_available_rotations_set()
- elm_win_wm_rotation_available_rotations_get()
- elm_win_wm_rotation_manual_rotation_done_set()
- elm_win_wm_rotation_manual_rotation_done_get()
- elm_win_wm_rotation_manual_rotation_done()