Tizen Native API
6.5
|
Dali::BaseHandle is a handle to an internal Dali resource. More...
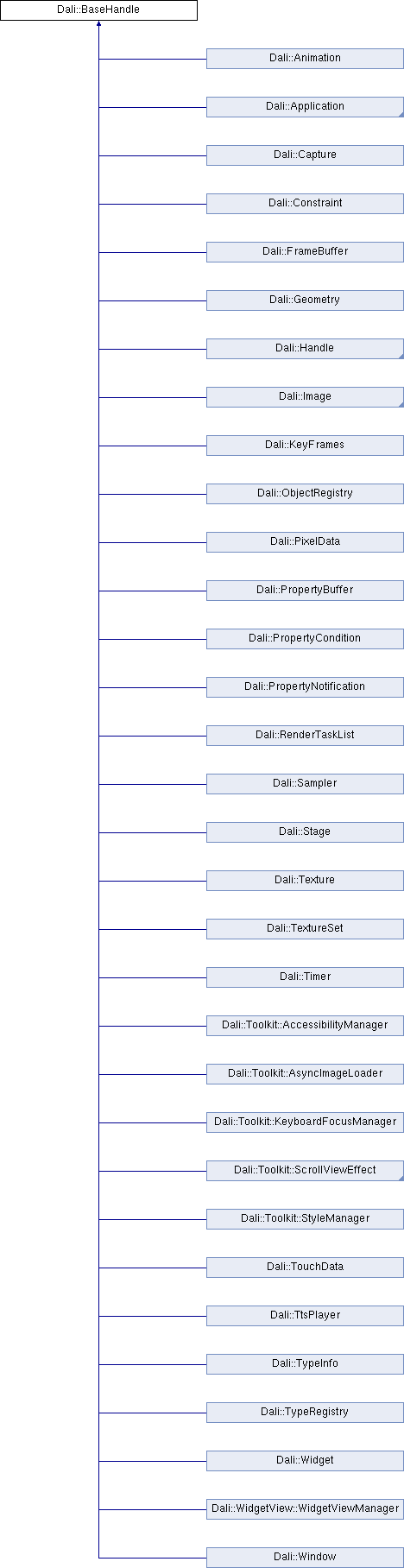
Public Types | |
typedef void(BaseHandle::* | BooleanType )() const |
Pointer-to-member type. Objects can be implicitly converted to this for validity checks. | |
Public Member Functions | |
BaseHandle (Dali::BaseObject *handle) | |
This constructor is used by Dali New() methods. | |
BaseHandle () | |
This constructor provides an uninitialized Dali::BaseHandle. | |
~BaseHandle () | |
Dali::BaseHandle is intended as a base class. | |
BaseHandle (const BaseHandle &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
BaseHandle & | operator= (const BaseHandle &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
template<class T > | |
bool | ConnectSignal (ConnectionTrackerInterface *connectionTracker, const std::string &signalName, const T &functor) |
Connects a void() functor to a specified signal. | |
bool | DoAction (const std::string &actionName, const Property::Map &attributes) |
Performs an action on this object with the given action name and attributes. | |
const std::string & | GetTypeName () const |
Returns the type name for the Handle. | |
bool | GetTypeInfo (Dali::TypeInfo &info) const |
Returns the type info for the Handle. | |
BaseObject & | GetBaseObject () |
Retrieves the internal Dali resource. | |
const BaseObject & | GetBaseObject () const |
Retrieves the internal Dali resource. | |
void | Reset () |
Resets the handle. | |
operator BooleanType () const | |
Converts an handle to a BooleanType. | |
bool | operator== (const BaseHandle &rhs) const |
Equality operator overload. | |
bool | operator!= (const BaseHandle &rhs) const |
Inequality operator overload. | |
Dali::RefObject * | GetObjectPtr () const |
Gets the reference counted object pointer. | |
Protected Member Functions | |
void | ThisIsSaferThanReturningVoidStar () const |
Used by the safe bool idiom. |
Detailed Description
Dali::BaseHandle is a handle to an internal Dali resource.
Each Dali handle consists of a single private pointer, and a set of non-virtual forwarding functions. This hides the internal implementation, so it may be modified without affecting the public interface.
Dali handles have implicit smart-pointer semantics. This avoids the need to match resource allocation methods like new/delete (the RAII idiom).
Dali handles can be copied by value. When a Dali handle is copied, both the copy and original will point to the same Dali resource.
The internal Dali resources are reference counted. copying a Dali handle will increase the reference count. A resource will not be deleted until all its Dali::BaseHandle handles are destroyed, or reset.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Dali::BaseHandle::BaseHandle | ( | Dali::BaseObject * | handle | ) |
This constructor provides an uninitialized Dali::BaseHandle.
This should be initialized with a Dali New() method before use. Methods called on an uninitialized Dali::BaseHandle will assert.
BaseHandle handle; // uninitialized handle.SomeMethod(); // unsafe! This will assert handle = SomeClass::New(); // now initialized handle.SomeMethod(); // safe
- Since:
- 2.4, DALi version 1.0.0
Dali::BaseHandle is intended as a base class.
This is non-virtual since derived BaseHandle types must not contain data.
- Since:
- 2.4, DALi version 1.0.0
Dali::BaseHandle::BaseHandle | ( | const BaseHandle & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
bool Dali::BaseHandle::ConnectSignal | ( | ConnectionTrackerInterface * | connectionTracker, |
const std::string & | signalName, | ||
const T & | functor | ||
) |
Connects a void() functor to a specified signal.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] connectionTracker A connection tracker which can be used to disconnect [in] signalName Name of the signal to connect to [in] functor The functor to copy
- Returns:
- True if the signal was available
- Precondition:
- The signal must be available in this object.
bool Dali::BaseHandle::DoAction | ( | const std::string & | actionName, |
const Property::Map & | attributes | ||
) |
Performs an action on this object with the given action name and attributes.
Usage example: -
BaseHandle handle = SomeClass::New(); // Initialized with New() method Property::Map attributes; // Type is Property Map handle.DoAction("show", attributes);
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actionName The command for the action [in] attributes The list of attributes for the action
- Returns:
- The action is performed by the object or not
Retrieves the internal Dali resource.
This is useful for checking the reference count of the internal resource. This method will not check the validity of the handle so the caller is expected to check it by using if (handle).
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The BaseObject which is referenced by the BaseHandle
const BaseObject& Dali::BaseHandle::GetBaseObject | ( | ) | const |
Retrieves the internal Dali resource.
This is useful for checking the reference count of the internal resource. This method will not check the validity of the handle so the caller is expected to check it by using if (handle).
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The BaseObject which is referenced by the BaseHandle
Dali::RefObject* Dali::BaseHandle::GetObjectPtr | ( | ) | const |
Gets the reference counted object pointer.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A pointer to the reference counted object
bool Dali::BaseHandle::GetTypeInfo | ( | Dali::TypeInfo & | info | ) | const |
Returns the type info for the Handle.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[out] info The type information
- Returns:
- true if the type info exists
const std::string& Dali::BaseHandle::GetTypeName | ( | ) | const |
Returns the type name for the Handle.
Will return an empty string if the typename does not exist. This will happen for types that have not registered with type-registry.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The type name. Empty string if the typename does not exist
Dali::BaseHandle::operator BooleanType | ( | ) | const |
Converts an handle to a BooleanType.
This is useful for checking whether the handle is empty.
- Since:
- 2.4, DALi version 1.0.0
bool Dali::BaseHandle::operator!= | ( | const BaseHandle & | rhs | ) | const |
Inequality operator overload.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the compared handle
- Returns:
- True if the handle handles point to the different Dali resources
BaseHandle& Dali::BaseHandle::operator= | ( | const BaseHandle & | rhs | ) |
This assignment operator is required for (smart) pointer semantics.
It makes this handle use the same BaseObject as the copied handle
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this handle
bool Dali::BaseHandle::operator== | ( | const BaseHandle & | rhs | ) | const |
Equality operator overload.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the compared handle
- Returns:
- True if the handle handles point to the same Dali resource, or if both are NULL
void Dali::BaseHandle::Reset | ( | ) |
Resets the handle.
If no other handle copies exist, the internal Dali resource will be deleted. Calling this is not required i.e. it will happen automatically when a Dali::BaseHandle is destroyed.
- Since:
- 2.4, DALi version 1.0.0
Reimplemented in Dali::Toolkit::AccessibilityManager.
void Dali::BaseHandle::ThisIsSaferThanReturningVoidStar | ( | ) | const [protected] |
Used by the safe bool idiom.
The safe bool idiom basically provides a Boolean test for classes. It validates objects in a boolean context without the usual harmful side effects.
- Since:
- 2.4, DALi version 1.0.0