Tizen Native API
6.5
|
Dali::Handle is a handle to an internal property owning Dali object that can have constraints applied to it. More...
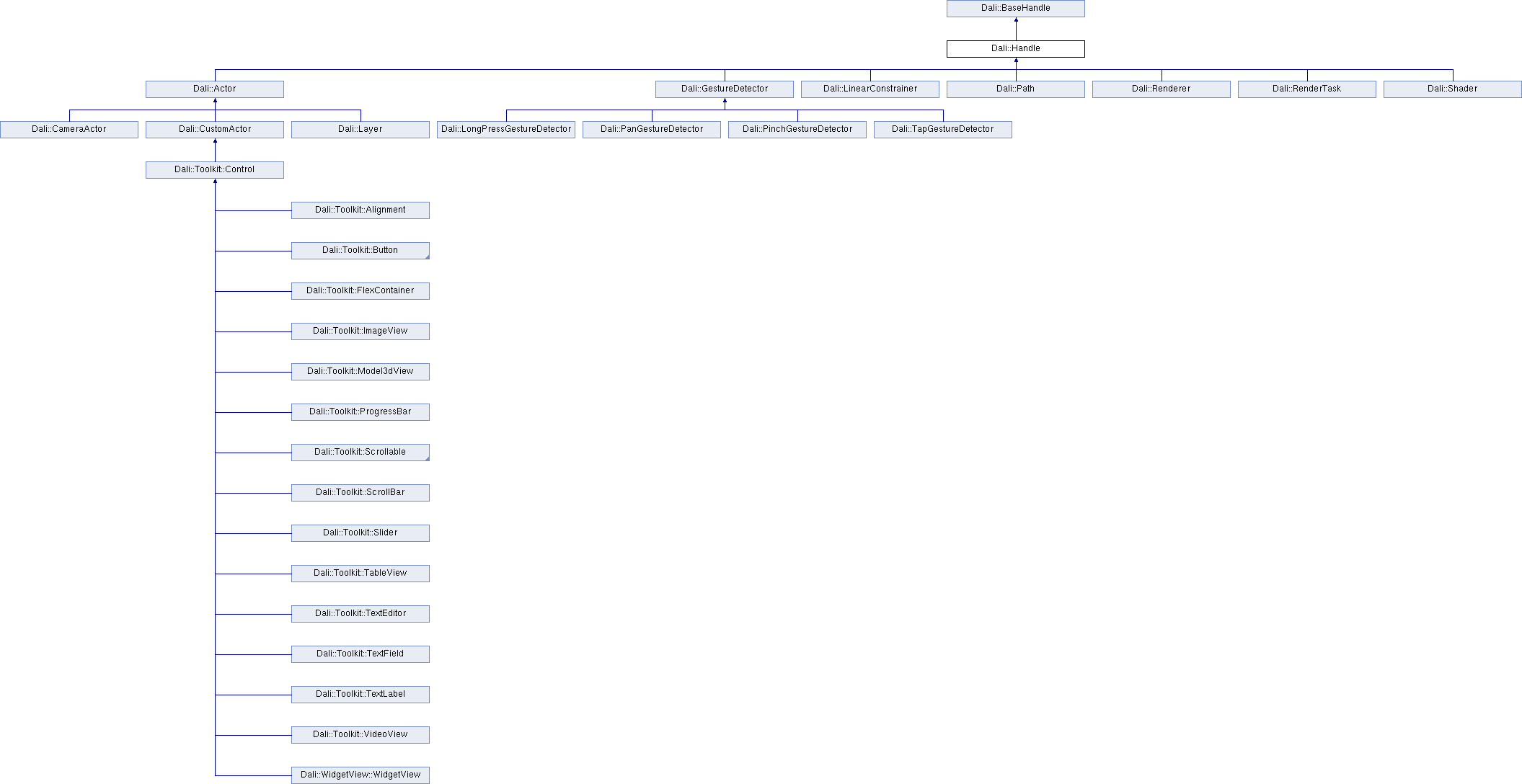
Public Types | |
enum | Capability |
Enumeration for Handle's capabilities that can be queried using Handle::Supports(). More... | |
Public Member Functions | |
Handle (Dali::Internal::Object *handle) | |
This constructor is used by Dali New() methods. | |
Handle () | |
This constructor provides an uninitialized Dali::Handle. | |
~Handle () | |
Dali::Handle is intended as a base class. | |
Handle (const Handle &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
Handle & | operator= (const Handle &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
bool | Supports (Capability capability) const |
Queries whether an handle supports a given capability. | |
uint32_t | GetPropertyCount () const |
Queries how many properties are provided by an handle. | |
std::string | GetPropertyName (Property::Index index) const |
Queries the name of a property. | |
Property::Index | GetPropertyIndex (const std::string &name) const |
Queries the index of a property. | |
bool | IsPropertyWritable (Property::Index index) const |
Queries whether a property can be set using SetProperty(). | |
bool | IsPropertyAnimatable (Property::Index index) const |
Queries whether a writable property can be the target of an animation or constraint. | |
bool | IsPropertyAConstraintInput (Property::Index index) const |
Queries whether a property can be used as in input to a constraint. | |
Property::Type | GetPropertyType (Property::Index index) const |
Queries the type of a property. | |
void | SetProperty (Property::Index index, const Property::Value &propertyValue) |
Sets the value of an existing property. | |
Property::Index | RegisterProperty (const std::string &name, const Property::Value &propertyValue) |
Registers a new animatable property. | |
Property::Index | RegisterProperty (const std::string &name, const Property::Value &propertyValue, Property::AccessMode accessMode) |
Registers a new property. | |
Property::Value | GetProperty (Property::Index index) const |
Retrieves a property value. | |
template<typename T > | |
T | GetProperty (Property::Index index) const |
Convenience function for obtaining a property of a known type. | |
Property::Value | GetCurrentProperty (Property::Index index) const |
Retrieves the latest value of the property from the scene-graph. | |
template<typename T > | |
T | GetCurrentProperty (Property::Index index) const |
Convenience function for obtaining the current value of a property of a known type. | |
void | GetPropertyIndices (Property::IndexContainer &indices) const |
Retrieves all the property indices for this object (including custom properties). | |
PropertyNotification | AddPropertyNotification (Property::Index index, const PropertyCondition &condition) |
Adds a property notification to this object. | |
PropertyNotification | AddPropertyNotification (Property::Index index, int componentIndex, const PropertyCondition &condition) |
Adds a property notification to this object. | |
void | RemovePropertyNotification (Dali::PropertyNotification propertyNotification) |
Removes a property notification from this object. | |
void | RemovePropertyNotifications () |
Removes all property notifications from this object. | |
void | RemoveConstraints () |
Removes all constraints from an Object. | |
void | RemoveConstraints (uint32_t tag) |
Removes all the constraint from the Object with a matching tag. | |
Static Public Member Functions | |
static Handle | New () |
Creates a new object. | |
static Handle | DownCast (BaseHandle handle) |
Downcasts to a handle. |
Detailed Description
Dali::Handle is a handle to an internal property owning Dali object that can have constraints applied to it.
- Since:
- 2.4, DALi version 1.0.0
Member Enumeration Documentation
Enumeration for Handle's capabilities that can be queried using Handle::Supports().
- Since:
- 2.4, DALi version 1.0.0
- Enumerator:
DYNAMIC_PROPERTIES Some objects support dynamic property creation at run-time.
New properties are registered by calling RegisterProperty() with an unused property name.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Dali::Handle::Handle | ( | Dali::Internal::Object * | handle | ) |
This constructor provides an uninitialized Dali::Handle.
This should be initialized with a Dali New() method before use. Methods called on an uninitialized Dali::Handle will assert.
Handle handle; // uninitialized handle.SomeMethod(); // unsafe! This will assert handle = SomeClass::New(); // now initialized handle.SomeMethod(); // safe
- Since:
- 2.4, DALi version 1.0.0
Dali::Handle is intended as a base class.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::Handle::Handle | ( | const Handle & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
PropertyNotification Dali::Handle::AddPropertyNotification | ( | Property::Index | index, |
const PropertyCondition & | condition | ||
) |
Adds a property notification to this object.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property [in] condition The notification will be triggered when this condition is satisfied
- Returns:
- A handle to the newly created PropertyNotification
PropertyNotification Dali::Handle::AddPropertyNotification | ( | Property::Index | index, |
int | componentIndex, | ||
const PropertyCondition & | condition | ||
) |
Adds a property notification to this object.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property [in] componentIndex Index to the component of a complex property such as a Vector [in] condition The notification will be triggered when this condition is satisfied
- Returns:
- A handle to the newly created PropertyNotification
static Handle Dali::Handle::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts to a handle.
If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle to An object
- Returns:
- handle or an uninitialized handle
Reimplemented in Dali::Toolkit::ScrollView, Dali::Actor, Dali::Toolkit::TextField, Dali::Toolkit::TextEditor, Dali::Toolkit::Control, Dali::Toolkit::TextLabel, Dali::Renderer, Dali::Toolkit::FlexContainer, Dali::Toolkit::VideoView, Dali::Toolkit::Button, Dali::Toolkit::ItemView, Dali::Toolkit::TableView, Dali::Toolkit::Control, Dali::Toolkit::ScrollBar, Dali::Toolkit::ImageView, Dali::Layer, Dali::Toolkit::Slider, Dali::Toolkit::ProgressBar, Dali::WidgetView::WidgetView, Dali::RenderTask, Dali::Toolkit::PushButton, Dali::CameraActor, Dali::Toolkit::Alignment, Dali::Shader, Dali::Toolkit::Model3dView, Dali::Toolkit::Scrollable, Dali::Toolkit::RadioButton, Dali::PanGestureDetector, Dali::Toolkit::CheckBoxButton, Dali::LongPressGestureDetector, Dali::TapGestureDetector, Dali::PinchGestureDetector, Dali::LinearConstrainer, Dali::Path, Dali::GestureDetector, and Dali::CustomActor.
Property::Value Dali::Handle::GetCurrentProperty | ( | Property::Index | index | ) | const |
Retrieves the latest value of the property from the scene-graph.
- Since:
- 4.0, DALi version 1.2.41
- Parameters:
-
[in] index The index of the property
- Returns:
- The property value
T Dali::Handle::GetCurrentProperty | ( | Property::Index | index | ) | const |
Convenience function for obtaining the current value of a property of a known type.
- Since:
- 4.0, DALi version 1.2.41
- Parameters:
-
[in] index The index of the property
- Returns:
- The property value
- Precondition:
- The property types match i.e. PropertyTypes::Get<T>() is equal to GetPropertyType(index).
Property::Value Dali::Handle::GetProperty | ( | Property::Index | index | ) | const |
Retrieves a property value.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- The property value
T Dali::Handle::GetProperty | ( | Property::Index | index | ) | const |
Convenience function for obtaining a property of a known type.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- The property value
- Precondition:
- The property types match i.e. PropertyTypes::Get<T>() is equal to GetPropertyType(index).
uint32_t Dali::Handle::GetPropertyCount | ( | ) | const |
Queries how many properties are provided by an handle.
This may vary between instances of a class, if dynamic properties are supported.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The number of properties
Property::Index Dali::Handle::GetPropertyIndex | ( | const std::string & | name | ) | const |
Queries the index of a property.
Returns the first property index that matches the given name exactly.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] name The name of the property
- Returns:
- The index of the property, or Property::INVALID_INDEX if no property exists with the given name
void Dali::Handle::GetPropertyIndices | ( | Property::IndexContainer & | indices | ) | const |
Retrieves all the property indices for this object (including custom properties).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[out] indices A container of property indices for this object
- Note:
- The added container is cleared.
std::string Dali::Handle::GetPropertyName | ( | Property::Index | index | ) | const |
Queries the name of a property.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- The name of the property
Property::Type Dali::Handle::GetPropertyType | ( | Property::Index | index | ) | const |
Queries the type of a property.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- The type of the property
bool Dali::Handle::IsPropertyAConstraintInput | ( | Property::Index | index | ) | const |
Queries whether a property can be used as in input to a constraint.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- True if the property can be used as a constraint input
bool Dali::Handle::IsPropertyAnimatable | ( | Property::Index | index | ) | const |
Queries whether a writable property can be the target of an animation or constraint.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- True if the property is animatable
bool Dali::Handle::IsPropertyWritable | ( | Property::Index | index | ) | const |
Queries whether a property can be set using SetProperty().
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property
- Returns:
- True if the property is writable
- Precondition:
- Property::INVALID_INDEX < index.
static Handle Dali::Handle::New | ( | ) | [static] |
Creates a new object.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to a newly allocated object
Reimplemented in Dali::Toolkit::ScrollView, Dali::Actor, Dali::Toolkit::TextField, Dali::Toolkit::TextEditor, Dali::Toolkit::TextLabel, Dali::Toolkit::FlexContainer, Dali::Layer, Dali::Toolkit::VideoView, Dali::Toolkit::Control, Dali::Toolkit::Slider, Dali::Toolkit::ProgressBar, Dali::Toolkit::PushButton, Dali::Toolkit::ImageView, Dali::CameraActor, Dali::Toolkit::RadioButton, Dali::PanGestureDetector, Dali::Toolkit::CheckBoxButton, Dali::Toolkit::Model3dView, Dali::TapGestureDetector, Dali::LongPressGestureDetector, Dali::PinchGestureDetector, Dali::LinearConstrainer, and Dali::Path.
This assignment operator is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
Property::Index Dali::Handle::RegisterProperty | ( | const std::string & | name, |
const Property::Value & | propertyValue | ||
) |
Registers a new animatable property.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] name The name of the property [in] propertyValue The new value of the property
- Returns:
- The index of the property or Property::INVALID_INDEX if registration failed
- Precondition:
- The object supports dynamic properties i.e. Supports(Handle::DYNAMIC_PROPERTIES) returns true. Property names are expected to be unique, but this is not enforced. Property indices are unique to each registered custom property in a given object. returns Property::INVALID_INDEX if registration failed. This can happen if you try to register animatable property on an object that does not have scene graph object.
- Note:
- Only the following types can be animated:
- If a property with the desired name already exists, then the value given is just set.
Property::Index Dali::Handle::RegisterProperty | ( | const std::string & | name, |
const Property::Value & | propertyValue, | ||
Property::AccessMode | accessMode | ||
) |
Registers a new property.
Properties can be set as non animatable using property attributes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] name The name of the property [in] propertyValue The new value of the property [in] accessMode The property access mode (writable, animatable etc)
- Returns:
- The index of the property
- Precondition:
- The handle supports dynamic properties i.e. Supports(Handle::DYNAMIC_PROPERTIES) returns true.
- name is unused i.e. GetPropertyIndex(name) returns PropertyIndex::INVALID.
- Note:
- Only the following types can be animated:
- If a property with the desired name already exists, then the value given is just set.
void Dali::Handle::RemoveConstraints | ( | ) |
Removes all constraints from an Object.
- Since:
- 2.4, DALi version 1.0.0
- Precondition:
- The object has been initialized.
void Dali::Handle::RemoveConstraints | ( | uint32_t | tag | ) |
Removes all the constraint from the Object with a matching tag.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] tag The tag of the constraints which will be removed
- Precondition:
- The Object has been initialized.
void Dali::Handle::RemovePropertyNotification | ( | Dali::PropertyNotification | propertyNotification | ) |
Removes a property notification from this object.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] propertyNotification The propertyNotification to be removed
Removes all property notifications from this object.
- Since:
- 2.4, DALi version 1.0.0
void Dali::Handle::SetProperty | ( | Property::Index | index, |
const Property::Value & | propertyValue | ||
) |
Sets the value of an existing property.
Property should be write-able. Setting a read-only property is a no-op.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the property [in] propertyValue The new value of the property
- Precondition:
- The property types match i.e. propertyValue.GetType() is equal to GetPropertyType(index).
bool Dali::Handle::Supports | ( | Capability | capability | ) | const |
Queries whether an handle supports a given capability.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] capability The queried capability
- Returns:
- True if the capability is supported