Tizen Native API
6.5
|
The Stage is a top-level object used for displaying a tree of Actors. More...
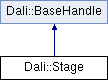
Public Types | |
typedef Signal< void(const KeyEvent &) > | KeyEventSignalType |
Key event signal type. | |
typedef Signal< void() > | EventProcessingFinishedSignalType |
Event Processing finished signal type. | |
typedef Signal< void(const TouchEvent &) > | TouchedSignalType |
Touched signal type. | |
typedef Signal< void(const TouchData &) > | TouchSignalType |
Touch signal type. | |
typedef Signal< void(const WheelEvent &) > | WheelEventSignalType |
Touched signal type. | |
typedef Signal< void() > | ContextStatusSignal |
Context status signal type. | |
typedef Signal< void() > | SceneCreatedSignalType |
Scene created signal type. | |
Public Member Functions | |
Stage () | |
Allows the creation of an empty stage handle. | |
~Stage () | |
Destructor. | |
Stage (const Stage &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
Stage & | operator= (const Stage &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | Add (Actor &actor) |
Adds a child Actor to the Stage. | |
void | Remove (Actor &actor) |
Removes a child Actor from the Stage. | |
Vector2 | GetSize () const |
Returns the size of the Stage in pixels as a Vector. | |
RenderTaskList | GetRenderTaskList () const |
Retrieves the list of render-tasks. | |
uint32_t | GetLayerCount () const |
Queries the number of on-stage layers. | |
Layer | GetLayer (uint32_t depth) const |
Retrieves the layer at a specified depth. | |
Layer | GetRootLayer () const |
Returns the Stage's Root Layer. | |
void | SetBackgroundColor (Vector4 color) |
Sets the background color of the stage. | |
Vector4 | GetBackgroundColor () const |
Retrieves the background color of the stage. | |
Vector2 | GetDpi () const |
Retrieves the DPI of the display device to which the stage is connected. | |
ObjectRegistry | GetObjectRegistry () const |
Gets the Object registry. | |
void | KeepRendering (float durationSeconds) |
Keep rendering for at least the given amount of time. | |
KeyEventSignalType & | KeyEventSignal () |
This signal is emitted when key event is received. | |
EventProcessingFinishedSignalType & | EventProcessingFinishedSignal () |
This signal is emitted just after the event processing is finished. | |
TouchedSignalType & | TouchedSignal () DALI_DEPRECATED_API |
This signal is emitted when the screen is touched and when the touch ends (i.e. the down & up touch events only). | |
TouchSignalType & | TouchSignal () |
This signal is emitted when the screen is touched and when the touch ends (i.e. the down & up touch events only). | |
WheelEventSignalType & | WheelEventSignal () |
This signal is emitted when wheel event is received. | |
ContextStatusSignal & | ContextLostSignal () |
This signal is emitted when the GL context is lost (Platform specific behaviour). | |
ContextStatusSignal & | ContextRegainedSignal () |
This signal is emitted when the GL context is regained (Platform specific behavior). | |
SceneCreatedSignalType & | SceneCreatedSignal () |
This signal is emitted after the initial scene is created. | |
Static Public Member Functions | |
static Stage | GetCurrent () |
Gets the current Stage. | |
static bool | IsInstalled () |
Queries whether the Stage exists; this should only return false during or after destruction of Dali core. | |
Static Public Attributes | |
static const Vector4 | DEFAULT_BACKGROUND_COLOR |
Default black background. | |
static const Vector4 | DEBUG_BACKGROUND_COLOR |
Green background, useful when debugging. |
Detailed Description
The Stage is a top-level object used for displaying a tree of Actors.
Stage is a top-level object that represents the entire screen. It is used for displaying a hierarchy of actors managed by the scene graph structure, which means an actor inherits a position relative to its parent, and can be moved in relation to this point.
The stage instance is a singleton object (the only instance of its class during the lifetime of the program). You can get it using a static function.
To display the contents of an actor, it must be added to a stage. The following example shows how to connect a new actor to the stage:
Actor actor = Actor::New(); Stage::GetCurrent().Add( actor );
The stage has a 2D size that matches the size of the application window. The default unit 1 is 1 pixel with the default camera.
Multiple stage/window support is not currently provided.
Signals | Signal Name | Method | |-------------------------|--------------------------------------| | keyEvent | KeyEventSignal() | | eventProcessingFinished | EventProcessingFinishedSignal() | | touched | TouchedSignal() | | wheelEvent | WheelEventSignal() | | contextLost | ContextLostSignal() | | contextRegained | ContextRegainedSignal() | | sceneCreated | SceneCreatedSignal() |
- Since:
- 2.4, DALi version 1.0.0
Member Typedef Documentation
typedef Signal< void () > Dali::Stage::ContextStatusSignal |
Context status signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void () > Dali::Stage::EventProcessingFinishedSignalType |
Event Processing finished signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void (const KeyEvent&) > Dali::Stage::KeyEventSignalType |
Key event signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void () > Dali::Stage::SceneCreatedSignalType |
Scene created signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void (const TouchEvent&) > Dali::Stage::TouchedSignalType |
Touched signal type.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.37
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void (const TouchData&) > Dali::Stage::TouchSignalType |
Touch signal type.
- Since:
- 3.0, DALi version 1.1.37
typedef Signal< void (const WheelEvent&) > Dali::Stage::WheelEventSignalType |
Touched signal type.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Allows the creation of an empty stage handle.
To retrieve the current stage, this handle can be set using Stage::GetCurrent().
- Since:
- 2.4, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::Stage::Stage | ( | const Stage & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
void Dali::Stage::Add | ( | Actor & | actor | ) |
This signal is emitted when the GL context is lost (Platform specific behaviour).
If the application is responsible for handling context loss, it should listen to this signal and tear down UI components when received.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The context lost signal to connect to
This signal is emitted when the GL context is regained (Platform specific behavior).
If the application is responsible for handling context loss, it should listen to this signal and rebuild UI components on receipt.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The context regained signal to connect to
This signal is emitted just after the event processing is finished.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
Vector4 Dali::Stage::GetBackgroundColor | ( | ) | const |
Retrieves the background color of the stage.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The background color
static Stage Dali::Stage::GetCurrent | ( | ) | [static] |
Gets the current Stage.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current stage or an empty handle if the internal core has not been created or has been already destroyed
Vector2 Dali::Stage::GetDpi | ( | ) | const |
Retrieves the DPI of the display device to which the stage is connected.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The horizontal and vertical DPI
Layer Dali::Stage::GetLayer | ( | uint32_t | depth | ) | const |
Retrieves the layer at a specified depth.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] depth The depth
- Returns:
- The layer found at the given depth
- Precondition:
- Depth is less than layer count; see GetLayerCount().
uint32_t Dali::Stage::GetLayerCount | ( | ) | const |
Queries the number of on-stage layers.
Note that a default layer is always provided (count >= 1).
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The number of layers
ObjectRegistry Dali::Stage::GetObjectRegistry | ( | ) | const |
Gets the Object registry.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The object registry
RenderTaskList Dali::Stage::GetRenderTaskList | ( | ) | const |
Retrieves the list of render-tasks.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A valid handle to a RenderTaskList
Layer Dali::Stage::GetRootLayer | ( | ) | const |
Vector2 Dali::Stage::GetSize | ( | ) | const |
static bool Dali::Stage::IsInstalled | ( | ) | [static] |
Queries whether the Stage exists; this should only return false during or after destruction of Dali core.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True when it's safe to call Stage::GetCurrent()
void Dali::Stage::KeepRendering | ( | float | durationSeconds | ) |
Keep rendering for at least the given amount of time.
By default, Dali will stop rendering when no Actor positions are being set, and when no animations are running etc. This method is useful to force screen refreshes e.g. when updating a NativeImage.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] durationSeconds Time to keep rendering, 0 means render at least one more frame
This signal is emitted when key event is received.
A callback of the following type may be connected:
void YourCallbackName(const KeyEvent& event);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
This assignment operator is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::Stage::Remove | ( | Actor & | actor | ) |
This signal is emitted after the initial scene is created.
It will be triggered after the application init signal.
A callback of the following type may be connected:
void YourCallbackName();
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
void Dali::Stage::SetBackgroundColor | ( | Vector4 | color | ) |
Sets the background color of the stage.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] color The new background color
This signal is emitted when the screen is touched and when the touch ends (i.e. the down & up touch events only).
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.37 Use TouchSignal() instead.
If there are multiple touch points, then this will be emitted when the first touch occurs and then when the last finger is lifted. An interrupted event will also be emitted (if it occurs). A callback of the following type may be connected:
void YourCallbackName(const TouchEvent& event);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The touch signal to connect to
- Note:
- Motion events are not emitted.
This signal is emitted when the screen is touched and when the touch ends (i.e. the down & up touch events only).
If there are multiple touch points, then this will be emitted when the first touch occurs and then when the last finger is lifted. An interrupted event will also be emitted (if it occurs). A callback of the following type may be connected:
void YourCallbackName( TouchData event );
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- The touch signal to connect to
- Note:
- Motion events are not emitted.
This signal is emitted when wheel event is received.
A callback of the following type may be connected:
void YourCallbackName(const WheelEvent& event);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to