Tizen Native API
6.5
|
Provides the functionality of handling keyboard navigation and maintaining the two dimensional keyboard focus chain. More...
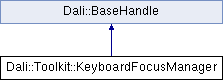
Public Types | |
typedef Signal< Actor(Actor, Actor, Control::KeyboardFocus::Direction) > | PreFocusChangeSignalType |
Pre focus change signal. | |
typedef Signal< void(Actor, Actor) > | FocusChangedSignalType |
Focus changed signal. | |
typedef Signal< void(Actor, bool) > | FocusGroupChangedSignalType |
Focus group changed signal. | |
typedef Signal< void(Actor) > | FocusedActorEnterKeySignalType |
Focused actor has the enter key pressed signal. | |
Public Member Functions | |
KeyboardFocusManager () | |
Creates a KeyboardFocusManager handle; this can be initialized with KeyboardFocusManager::New(). | |
~KeyboardFocusManager () | |
Destructor. | |
bool | SetCurrentFocusActor (Actor actor) |
Moves the keyboard focus to the given actor. | |
Actor | GetCurrentFocusActor () |
Gets the current focused actor. | |
bool | MoveFocus (Control::KeyboardFocus::Direction direction) |
Moves the focus to the next focusable actor in the focus chain in the given direction (according to the focus traversal order). | |
void | ClearFocus () |
Clears the focus from the current focused actor if any, so that no actor is focused in the focus chain. | |
void | SetFocusGroupLoop (bool enabled) |
Sets whether the focus movement should be looped within the same focus group. | |
bool | GetFocusGroupLoop () const |
Gets whether the focus movement should be looped within the same focus group. | |
void | SetAsFocusGroup (Actor actor, bool isFocusGroup) |
Sets whether an actor is a focus group that can limit the scope of focus movement to its child actors in the focus chain. | |
bool | IsFocusGroup (Actor actor) const |
Checks whether the actor is set as a focus group or not. | |
Actor | GetFocusGroup (Actor actor) |
Returns the closest ancestor of the given actor that is a focus group. | |
void | SetFocusIndicatorActor (Actor indicator) |
Sets the focus indicator actor. | |
Actor | GetFocusIndicatorActor () |
Gets the focus indicator actor. | |
void | MoveFocusBackward () |
Move the focus to prev focused actor. | |
PreFocusChangeSignalType & | PreFocusChangeSignal () |
This signal is emitted before the focus is going to be changed. | |
FocusChangedSignalType & | FocusChangedSignal () |
This signal is emitted after the current focused actor has been changed. | |
FocusGroupChangedSignalType & | FocusGroupChangedSignal () |
This signal is emitted when the focus group has been changed. | |
FocusedActorEnterKeySignalType & | FocusedActorEnterKeySignal () |
This signal is emitted when the current focused actor has the enter key pressed on it. | |
Static Public Member Functions | |
static KeyboardFocusManager | Get () |
Gets the singleton of KeyboardFocusManager object. |
Detailed Description
Provides the functionality of handling keyboard navigation and maintaining the two dimensional keyboard focus chain.
It provides functionality of setting the focus and moving the focus in four directions (i.e. Left, Right, Up and Down). It also draws a highlight for the focused actor and emits a signal when the focus is changed.
Signals | Signal Name | Method | |------------------------------|------------------------------------| | keyboardPreFocusChange | PreFocusChangeSignal() | | keyboardFocusChanged | FocusChangedSignal() | | keyboardFocusGroupChanged | FocusGroupChangedSignal() | | keyboardFocusedActorEnterKey | FocusedActorEnterKeySignal() |
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Creates a KeyboardFocusManager handle; this can be initialized with KeyboardFocusManager::New().
Calling member functions with an uninitialized handle is not allowed.
- Since:
- 2.4, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Member Function Documentation
Clears the focus from the current focused actor if any, so that no actor is focused in the focus chain.
It will emit focus changed signal without current focused actor.
- Since:
- 2.4, DALi version 1.0.0
- Precondition:
- The KeyboardFocusManager has been initialized.
This signal is emitted after the current focused actor has been changed.
A callback of the following type may be connected:
void YourCallbackName(Actor originalFocusedActor, Actor currentFocusedActor);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Object has been initialized.
This signal is emitted when the current focused actor has the enter key pressed on it.
A callback of the following type may be connected:
void YourCallbackName(Actor enterPressedActor);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Object has been initialized.
This signal is emitted when the focus group has been changed.
If the current focus group has a parent layout control, KeyboardFocusManager will make the best guess for the next focus group to move the focus to in the given direction (forward or backward). If not, the application has to set the new focus.
A callback of the following type may be connected:
void YourCallbackName(Actor currentFocusedActor, bool forward);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Object has been initialized.
static KeyboardFocusManager Dali::Toolkit::KeyboardFocusManager::Get | ( | ) | [static] |
Gets the singleton of KeyboardFocusManager object.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to the KeyboardFocusManager control
Gets the current focused actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to the current focused actor or an empty handle if no actor is focused
- Precondition:
- The KeyboardFocusManager has been initialized.
Returns the closest ancestor of the given actor that is a focus group.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
actor The actor to be checked for its focus group
- Returns:
- The focus group the given actor belongs to or an empty handle if the given actor doesn't belong to any focus group
bool Dali::Toolkit::KeyboardFocusManager::GetFocusGroupLoop | ( | ) | const |
Gets whether the focus movement should be looped within the same focus group.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Whether the focus movement should be looped
- Precondition:
- The KeyboardFocusManager has been initialized.
Gets the focus indicator actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to the focus indicator actor
- Precondition:
- The KeyboardFocusManager has been initialized.
bool Dali::Toolkit::KeyboardFocusManager::IsFocusGroup | ( | Actor | actor | ) | const |
Checks whether the actor is set as a focus group or not.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
actor The actor to be checked
- Returns:
- Whether the actor is set as a focus group
- Precondition:
- The KeyboardFocusManager has been initialized.
- The Actor has been initialized.
Moves the focus to the next focusable actor in the focus chain in the given direction (according to the focus traversal order).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
direction The direction of focus movement
- Returns:
- true if the movement was successful
- Precondition:
- The KeyboardFocusManager has been initialized.
Move the focus to prev focused actor.
- Since:
- 4.0, DALi version 1.2.19
This signal is emitted before the focus is going to be changed.
KeyboardFocusManager makes the best guess for which actor to focus towards the given direction, but applications might want to change that. By connecting with this signal, they can check the proposed actor to focus and return a different actor if they wish. This signal is only emitted when the navigation key is pressed and KeyboardFocusManager tries to move the focus automatically. It won't be emitted for focus movement by calling SetCurrentFocusActor directly.
A callback of the following type may be connected:
Actor YourCallbackName(Actor currentFocusedActor, Actor proposedActorToFocus, Control::KeyboardFocus::Direction direction);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Object has been initialized.
void Dali::Toolkit::KeyboardFocusManager::SetAsFocusGroup | ( | Actor | actor, |
bool | isFocusGroup | ||
) |
Sets whether an actor is a focus group that can limit the scope of focus movement to its child actors in the focus chain.
Layout controls set themselves as focus groups by default.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
actor The actor to be set as a focus group isFocusGroup Whether to set the actor as a focus group or not
- Precondition:
- The KeyboardFocusManager has been initialized.
- The Actor has been initialized.
Moves the keyboard focus to the given actor.
Only one actor can be focused at the same time. The actor must be in the stage already and keyboard focusable.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
actor The actor to be focused
- Returns:
- Whether the focus is successful or not
- Precondition:
- The KeyboardFocusManager has been initialized.
- The Actor has been initialized.
void Dali::Toolkit::KeyboardFocusManager::SetFocusGroupLoop | ( | bool | enabled | ) |
Sets whether the focus movement should be looped within the same focus group.
The focus movement is not looped by default.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
enabled Whether the focus movement should be looped
- Precondition:
- The KeyboardFocusManager has been initialized.
void Dali::Toolkit::KeyboardFocusManager::SetFocusIndicatorActor | ( | Actor | indicator | ) |
Sets the focus indicator actor.
This will replace the default focus indicator actor in KeyboardFocusManager and will be added to the focused actor as a highlight.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
indicator The indicator actor to be added
- Precondition:
- The KeyboardFocusManager has been initialized.
- The indicator actor has been initialized.