Tizen Native API
6.5
|
CameraActor controls a camera. More...
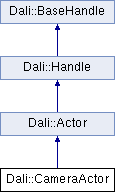
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the CameraActor class. More... | |
Public Member Functions | |
CameraActor () | |
Creates an uninitialized CameraActor handle. | |
~CameraActor () | |
Destructor. | |
CameraActor (const CameraActor ©) | |
Copy constructor. | |
CameraActor & | operator= (const CameraActor &rhs) |
Assignment operator. | |
void | SetType (Dali::Camera::Type type) |
Sets the camera type. The default type is Dali::Camera::FREE_LOOK. | |
Dali::Camera::Type | GetType () const |
Gets the type of the camera. | |
void | SetProjectionMode (Dali::Camera::ProjectionMode mode) |
Sets the projection mode. | |
Dali::Camera::ProjectionMode | GetProjectionMode () const |
Gets the projection mode. | |
void | SetFieldOfView (float fieldOfView) |
Sets the field of view. | |
float | GetFieldOfView () |
Gets the field of view in Radians. | |
void | SetAspectRatio (float aspectRatio) |
Sets the aspect ratio. | |
float | GetAspectRatio () |
Gets the aspect ratio of the camera. | |
void | SetNearClippingPlane (float nearClippingPlane) |
Sets the near clipping plane distance. | |
float | GetNearClippingPlane () |
Gets the near clipping plane distance. | |
void | SetFarClippingPlane (float farClippingPlane) |
Sets the far clipping plane distance. | |
float | GetFarClippingPlane () |
Gets the far clipping plane distance. | |
void | SetTargetPosition (const Vector3 &targetPosition) |
Sets the target position of the camera. | |
Vector3 | GetTargetPosition () const |
Gets the Camera Target position. | |
void | SetInvertYAxis (bool invertYAxis) |
Requests for an inversion on the Y axis on the projection calculation. | |
bool | GetInvertYAxis () |
Gets whether the Y axis is inverted. | |
void | SetPerspectiveProjection (const Size &size) |
Sets the default camera perspective projection for the given canvas size. | |
void | SetOrthographicProjection (const Size &size) |
Sets the camera projection to use orthographic projection. | |
void | SetOrthographicProjection (float left, float right, float top, float bottom, float near, float far) |
Sets the camera projection to use orthographic projection with the given clip planes. | |
Static Public Member Functions | |
static CameraActor | New () |
Creates a CameraActor object. | |
static CameraActor | New (const Size &size) |
Creates a CameraActor object. | |
static CameraActor | DownCast (BaseHandle handle) |
Downcasts a handle to CameraActor handle. |
Detailed Description
CameraActor controls a camera.
Allows the developer to use actor semantics to control a camera.
DALi has a concept of a camera to display its virtual 3D world to a 2D screen. There are 2 ways of using the camera in DALi:
- For 2D applications, you do not need to care about the camera at all. The default camera is already best suited for 2D applications (configured to have the origin of the coordinate system at the top-left corner of the screen, and unit 1 as 1 pixel of the screen). This is a typical way.
- For 3D applications, you can change the view by manipulating the camera. You can translate or rotate the camera in this case. Note that the top-left corner of the screen and unit 1 no longer are (0,0,0) and 1 pixel after manipulating the camera.
There are two types of camera actor, FREE_LOOK and LOOK_AT_TARGET. By default, the camera actor will be FREE_LOOK.
- A FREE_LOOK camera uses actor's orientation to control where the camera is looking. If no additional rotations are specified, the camera looks in the negative Z direction.
- For LOOK_AT_TARGET, the actor's orientation is ignored, instead the camera looks at TARGET_POSITION in world coordinates.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Creates an uninitialized CameraActor handle.
Initialize it using CameraActor::New(). Calling member functions with an uninitialized CameraActor handle is not allowed.
- Since:
- 2.4, DALi version 1.0.0
Destructor.
This is non-virtual, since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::CameraActor::CameraActor | ( | const CameraActor & | copy | ) |
Copy constructor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] copy The actor to copy
Member Function Documentation
static CameraActor Dali::CameraActor::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to CameraActor handle.
If handle points to a CameraActor, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle to An object
- Returns:
- Handle to a CameraActor or an uninitialized handle
Reimplemented from Dali::Actor.
float Dali::CameraActor::GetAspectRatio | ( | ) |
Gets the aspect ratio of the camera.
The default aspect ratio is 4.0f/3.0f.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The aspect ratio
float Dali::CameraActor::GetFarClippingPlane | ( | ) |
Gets the far clipping plane distance.
The default value is the default near clipping plane + (0xFFFF>>4).
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The far clipping plane value
float Dali::CameraActor::GetFieldOfView | ( | ) |
Gets the field of view in Radians.
The default field of view is 45 degrees.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The field of view in radians
bool Dali::CameraActor::GetInvertYAxis | ( | ) |
Gets whether the Y axis is inverted.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
True
if the Y axis is inverted,false
otherwise
float Dali::CameraActor::GetNearClippingPlane | ( | ) |
Gets the near clipping plane distance.
The default near clipping plane is 800.0f, to match the default screen height. Reduce this value to see objects closer to the camera.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The near clipping plane value
Gets the projection mode.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- One of PERSPECTIVE_PROJECTION or ORTHOGRAPHIC_PROJECTION
Vector3 Dali::CameraActor::GetTargetPosition | ( | ) | const |
Gets the Camera Target position.
The default target position is Vector3::ZERO.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The target position of the camera
- Precondition:
- Camera type is LOOK_AT_TARGET.
Dali::Camera::Type Dali::CameraActor::GetType | ( | ) | const |
Gets the type of the camera.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The type of camera
static CameraActor Dali::CameraActor::New | ( | ) | [static] |
Creates a CameraActor object.
- Note:
- Sets the default camera perspective projection for the size of the scene this is added to.
- See also:
- SetPerspectiveProjection().
- Note:
- When this actor gets added to a scene, then it's Z position will be modified according to the required perspective projection.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The newly created camera actor
Reimplemented from Dali::Actor.
static CameraActor Dali::CameraActor::New | ( | const Size & | size | ) | [static] |
Creates a CameraActor object.
Sets the default camera perspective projection for the given canvas size.
- See also:
- SetPerspectiveProjection().
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The canvas size
- Returns:
- The newly created camera actor
CameraActor& Dali::CameraActor::operator= | ( | const CameraActor & | rhs | ) |
Assignment operator.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs The actor to copy
- Returns:
- A reference to this
void Dali::CameraActor::SetAspectRatio | ( | float | aspectRatio | ) |
Sets the aspect ratio.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] aspectRatio The aspect ratio
void Dali::CameraActor::SetFarClippingPlane | ( | float | farClippingPlane | ) |
Sets the far clipping plane distance.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] farClippingPlane Distance of the far clipping plane
void Dali::CameraActor::SetFieldOfView | ( | float | fieldOfView | ) |
Sets the field of view.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] fieldOfView The field of view in radians
void Dali::CameraActor::SetInvertYAxis | ( | bool | invertYAxis | ) |
Requests for an inversion on the Y axis on the projection calculation.
The default value is not inverted.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] invertYAxis True if the Y axis should be inverted
void Dali::CameraActor::SetNearClippingPlane | ( | float | nearClippingPlane | ) |
Sets the near clipping plane distance.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] nearClippingPlane Distance of the near clipping plane
void Dali::CameraActor::SetOrthographicProjection | ( | const Size & | size | ) |
Sets the camera projection to use orthographic projection.
The XY plane is centered on the camera axis. The units in the X/Y plane directly equate to pixels on an equivalently sized framebuffer.
The Z position of the actor, and the near and far clip planes of the bounding box match those that would be created by using SetPerspectiveProjection with the same size.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size Size of XY plane (normal to camera axis)
void Dali::CameraActor::SetOrthographicProjection | ( | float | left, |
float | right, | ||
float | top, | ||
float | bottom, | ||
float | near, | ||
float | far | ||
) |
Sets the camera projection to use orthographic projection with the given clip planes.
This does not change the Z value of the camera actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] left Distance to left clip plane (normal to camera axis) [in] right Distance to right clip plane (normal to camera axis) [in] top Distance to top clip plane (normal to camera axis) [in] bottom Distance to bottom clip plane (normal to camera axis) [in] near Distance to the near clip plane (along camera axis) [in] far Distance to the far clip plane (along camera axis)
void Dali::CameraActor::SetPerspectiveProjection | ( | const Size & | size | ) |
Sets the default camera perspective projection for the given canvas size.
Sets the near and far clipping planes, the field of view, the aspect ratio, and the Z position of the actor based on the canvas size so that 1 unit in XY (z=0) plane is 1 pixel on screen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The canvas size
- Precondition:
- The canvas size must be greater than zero.
- Note:
- If either of the values of size is 0.0f, then we use the default perspective projection for the size of the scene this actor is added to.
- This modifies the Z position property of this actor as well.
Sets the projection mode.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] mode One of PERSPECTIVE_PROJECTION or ORTHOGRAPHIC_PROJECTION
void Dali::CameraActor::SetTargetPosition | ( | const Vector3 & | targetPosition | ) |
Sets the target position of the camera.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] targetPosition The position of the target to look at
- Precondition:
- Camera type is LOOK_AT_TARGET.
void Dali::CameraActor::SetType | ( | Dali::Camera::Type | type | ) |
Sets the camera type. The default type is Dali::Camera::FREE_LOOK.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] type The camera type