Tizen Native API
6.5
|
The AsyncImageLoader is used to load pixel data from a URL asynchronously. More...
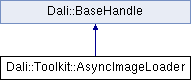
Public Types | |
typedef Signal< void(uint32_t, PixelData) > | ImageLoadedSignalType |
Image loaded signal type. | |
Public Member Functions | |
AsyncImageLoader () | |
Constructor which creates an empty AsyncImageLoader handle. | |
~AsyncImageLoader () | |
Destructor. | |
AsyncImageLoader (const AsyncImageLoader &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
AsyncImageLoader & | operator= (const AsyncImageLoader &handle) |
This assignment operator is required for (smart) pointer semantics. | |
uint32_t | Load (const std::string &url) |
Starts an image loading task. Note: When using this method, the following defaults will be used: fittingMode = FittingMode::DEFAULT samplingMode = SamplingMode::BOX_THEN_LINEAR orientationCorrection = true. | |
uint32_t | Load (const std::string &url, ImageDimensions dimensions) |
Starts an image loading task. Note: When using this method, the following defaults will be used: fittingMode = FittingMode::DEFAULT samplingMode = SamplingMode::BOX_THEN_LINEAR orientationCorrection = true. | |
uint32_t | Load (const std::string &url, ImageDimensions dimensions, FittingMode::Type fittingMode, SamplingMode::Type samplingMode, bool orientationCorrection) |
Starts an image loading task. | |
bool | Cancel (uint32_t loadingTaskId) |
Cancels an image loading task if it is still queueing in the work thread. | |
void | CancelAll () |
Cancels all the loading tasks in the queue. | |
ImageLoadedSignalType & | ImageLoadedSignal () |
Signal emitted for connected callback functions to get access to the loaded pixel data. | |
Static Public Member Functions | |
static AsyncImageLoader | New () |
Creates a new loader to load the image asynchronously in a worker thread. | |
static AsyncImageLoader | DownCast (BaseHandle handle) |
Downcasts a handle to AsyncImageLoader handle. |
Detailed Description
The AsyncImageLoader is used to load pixel data from a URL asynchronously.
The images are loaded in a worker thread to avoid blocking the main event thread.
To keep track of the loading images, each load call is assigned an ID (which is returned by the Load() call). To know when the Load has completed, connect to the ImageLoadedSignal. This signal should be connected before Load is called (in case the signal is emitted immediately).
Load errors can be detected by checking the PixelData object is valid from within the signal handler.
Note: The PixelData object will automatically be destroyed when it leaves its scope.
Example:
class MyClass : public ConnectionTracker { public: MyCallback( uint32_t loadedTaskId, PixelData pixelData ) { // First check if the image loaded correctly. if( pixelData ) { if( loadedTaskId == mId1 ) { // use the loaded pixel data from the first image } else if( loadedTaskId == mId2 ) { // use the loaded pixel data from the second image } } } uint32_t mId1; uint32_t mId2; }; MyClass myObject; AsyncImageLoader imageLoader = AsyncImageLoader::New(); // Connect the signal here. imageLoader.ImageLoadedSignal().Connect( &myObject, &MyClass::MyCallback ); // Invoke the load calls (must do this after connecting the signal to guarantee callbacks occur). myObject.mId1 = imageLoader.Load( "first_image_url.jpg" ); myObject.mId2 = imageLoader.Load( "second_image_url.jpg" );
Member Typedef Documentation
typedef Signal< void( uint32_t, PixelData ) > Dali::Toolkit::AsyncImageLoader::ImageLoadedSignalType |
Image loaded signal type.
- Since:
- 3.0, DALi version 1.2.14
Constructor & Destructor Documentation
Constructor which creates an empty AsyncImageLoader handle.
- Since:
- 3.0, DALi version 1.2.14
Use AsyncImageLoader::New() to create an initialised object.
Destructor.
- Since:
- 3.0, DALi version 1.2.14
This is non-virtual since derived Handle types must not contain data or virtual methods.
Dali::Toolkit::AsyncImageLoader::AsyncImageLoader | ( | const AsyncImageLoader & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.2.14
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
bool Dali::Toolkit::AsyncImageLoader::Cancel | ( | uint32_t | loadingTaskId | ) |
Cancels an image loading task if it is still queueing in the work thread.
- Since:
- 3.0, DALi version 1.2.14
- Parameters:
-
[in] loadingTaskId The task id returned when invoking the load call.
- Returns:
- If true, the loading task is removed from the queue, otherwise the loading is already implemented and unable to cancel anymore
Cancels all the loading tasks in the queue.
- Since:
- 3.0, DALi version 1.2.14
static AsyncImageLoader Dali::Toolkit::AsyncImageLoader::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to AsyncImageLoader handle.
If the handle points to an AsyncImageLoader object, the downcast produces a valid handle. If not, the returned handle is left uninitialized.
- Since:
- 3.0, DALi version 1.2.14
- Parameters:
-
[in] handle A handle to an object
- Returns:
- A handle to a AsyncImageLoader object or an uninitialized handle
Signal emitted for connected callback functions to get access to the loaded pixel data.
A callback of the following type may be connected:
void YourCallbackName( uint32_t id, PixelData pixelData );
- Since:
- 3.0, DALi version 1.2.14
- Returns:
- A reference to a signal object to Connect() with
uint32_t Dali::Toolkit::AsyncImageLoader::Load | ( | const std::string & | url | ) |
Starts an image loading task. Note: When using this method, the following defaults will be used: fittingMode = FittingMode::DEFAULT samplingMode = SamplingMode::BOX_THEN_LINEAR orientationCorrection = true.
- Since:
- 3.0, DALi version 1.2.14
- Remarks:
- http://tizen.org/privilege/internet is needed if url is a http or https address.
- http://tizen.org/privilege/mediastorage is needed if url is relevant to media storage.
- http://tizen.org/privilege/externalstorage is needed if url is relevant to external storage.
- Parameters:
-
[in] url The URL of the image file to load
- Returns:
- The loading task id
uint32_t Dali::Toolkit::AsyncImageLoader::Load | ( | const std::string & | url, |
ImageDimensions | dimensions | ||
) |
Starts an image loading task. Note: When using this method, the following defaults will be used: fittingMode = FittingMode::DEFAULT samplingMode = SamplingMode::BOX_THEN_LINEAR orientationCorrection = true.
- Since:
- 3.0, DALi version 1.2.14
- Remarks:
- http://tizen.org/privilege/internet is needed if url is a http or https address.
- http://tizen.org/privilege/mediastorage is needed if url is relevant to media storage.
- http://tizen.org/privilege/externalstorage is needed if url is relevant to external storage.
- Parameters:
-
[in] url The URL of the image file to load [in] dimensions The width and height to fit the loaded image to
- Returns:
- The loading task id
uint32_t Dali::Toolkit::AsyncImageLoader::Load | ( | const std::string & | url, |
ImageDimensions | dimensions, | ||
FittingMode::Type | fittingMode, | ||
SamplingMode::Type | samplingMode, | ||
bool | orientationCorrection | ||
) |
Starts an image loading task.
- Since:
- 3.0, DALi version 1.2.14
- Remarks:
- http://tizen.org/privilege/internet is needed if url is a http or https address.
- http://tizen.org/privilege/mediastorage is needed if url is relevant to media storage.
- http://tizen.org/privilege/externalstorage is needed if url is relevant to external storage.
- Parameters:
-
[in] url The URL of the image file to load [in] dimensions The width and height to fit the loaded image to [in] fittingMode The method used to fit the shape of the image before loading to the shape defined by the size parameter [in] samplingMode The filtering method used when sampling pixels from the input image while fitting it to desired size [in] orientationCorrection Reorient the image to respect any orientation metadata in its header
- Returns:
- The loading task id
static AsyncImageLoader Dali::Toolkit::AsyncImageLoader::New | ( | ) | [static] |
Creates a new loader to load the image asynchronously in a worker thread.
- Since:
- 3.0, DALi version 1.2.14
- Returns:
- The image loader
AsyncImageLoader& Dali::Toolkit::AsyncImageLoader::operator= | ( | const AsyncImageLoader & | handle | ) |
This assignment operator is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.2.14
- Parameters:
-
[in] handle A reference to the copied handle
- Returns:
- A reference to this