Tizen Native API
6.5
|
Dali::Animation can be used to animate the properties of any number of objects, typically Actors. More...
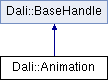
Public Types | |
enum | EndAction |
Enumeration for what to do when the animation ends, is stopped, or is destroyed. More... | |
enum | Interpolation |
Enumeration for what interpolation method to use on key-frame animations. More... | |
enum | State |
Enumeration for what state the animation is in. More... | |
enum | LoopingMode |
Enumeration for what looping mode is in. More... | |
typedef Signal< void(Animation &) > | AnimationSignalType |
Animation finished signal type. | |
typedef Any | AnyFunction |
Interpolation function. | |
Public Member Functions | |
Animation () | |
Creates an uninitialized Animation; this can be initialized with Animation::New(). | |
~Animation () | |
Destructor. | |
Animation (const Animation &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
Animation & | operator= (const Animation &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | SetDuration (float seconds) |
Sets the duration of an animation. | |
float | GetDuration () const |
Retrieves the duration of an animation. | |
void | SetLooping (bool looping) |
Sets whether the animation will loop. | |
void | SetLoopCount (int32_t count) |
Enables looping for 'count' repeats. | |
int32_t | GetLoopCount () |
Gets the loop count. | |
int32_t | GetCurrentLoop () |
Gets the current loop count. | |
bool | IsLooping () const |
Queries whether the animation will loop. | |
void | SetEndAction (EndAction action) |
Sets the end action of the animation. | |
EndAction | GetEndAction () const |
Returns the end action of the animation. | |
void | SetDisconnectAction (EndAction disconnectAction) |
Sets the disconnect action. | |
EndAction | GetDisconnectAction () const |
Returns the disconnect action. | |
void | SetDefaultAlphaFunction (AlphaFunction alpha) |
Sets the default alpha function for an animation. | |
AlphaFunction | GetDefaultAlphaFunction () const |
Retrieves the default alpha function for an animation. | |
void | SetCurrentProgress (float progress) |
Sets the progress of the animation. | |
float | GetCurrentProgress () |
Retrieves the current progress of the animation. | |
void | SetSpeedFactor (float factor) |
Specifies a speed factor for the animation. | |
float | GetSpeedFactor () const |
Retrieves the speed factor of the animation. | |
void | SetPlayRange (const Vector2 &range) |
Sets the playing range. | |
Vector2 | GetPlayRange () const |
Gets the playing range. | |
void | Play () |
Play the animation. | |
void | PlayFrom (float progress) |
Plays the animation from a given point. | |
void | PlayAfter (float delaySeconds) |
Play the animation after a given delay time. | |
void | Pause () |
Pauses the animation. | |
State | GetState () const |
Queries the state of the animation. | |
void | Stop () |
Stops the animation. | |
void | Clear () |
Clears the animation. | |
void | SetLoopingMode (LoopingMode loopingMode) |
Sets the looping mode. | |
LoopingMode | GetLoopingMode () const |
Gets one of the current looping mode. | |
AnimationSignalType & | FinishedSignal () |
Connects to this signal to be notified when an Animation's animations have finished. | |
void | AnimateBy (Property target, Property::Value relativeValue) |
Animates a property value by a relative amount. | |
void | AnimateBy (Property target, Property::Value relativeValue, AlphaFunction alpha) |
Animates a property value by a relative amount. | |
void | AnimateBy (Property target, Property::Value relativeValue, TimePeriod period) |
Animates a property value by a relative amount. | |
void | AnimateBy (Property target, Property::Value relativeValue, AlphaFunction alpha, TimePeriod period) |
Animates a property value by a relative amount. | |
void | AnimateTo (Property target, Property::Value destinationValue) |
Animates a property to a destination value. | |
void | AnimateTo (Property target, Property::Value destinationValue, AlphaFunction alpha) |
Animates a property to a destination value. | |
void | AnimateTo (Property target, Property::Value destinationValue, TimePeriod period) |
Animates a property to a destination value. | |
void | AnimateTo (Property target, Property::Value destinationValue, AlphaFunction alpha, TimePeriod period) |
Animates a property to a destination value. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, Interpolation interpolation) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, AlphaFunction alpha) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, AlphaFunction alpha, Interpolation interpolation) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, TimePeriod period) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, TimePeriod period, Interpolation interpolation) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, AlphaFunction alpha, TimePeriod period) |
Animates a property between keyframes. | |
void | AnimateBetween (Property target, KeyFrames &keyFrames, AlphaFunction alpha, TimePeriod period, Interpolation interpolation) |
Animates a property between keyframes. | |
void | Animate (Actor actor, Path path, const Vector3 &forward) |
Animates an actor's position and orientation through a predefined path. | |
void | Animate (Actor actor, Path path, const Vector3 &forward, AlphaFunction alpha) |
Animates an actor's position and orientation through a predefined path. | |
void | Animate (Actor actor, Path path, const Vector3 &forward, TimePeriod period) |
Animates an actor's position and orientation through a predefined path. | |
void | Animate (Actor actor, Path path, const Vector3 &forward, AlphaFunction alpha, TimePeriod period) |
Animates an actor's position and orientation through a predefined path. | |
void | Show (Actor actor, float delaySeconds) |
Shows an actor during the animation. | |
void | Hide (Actor actor, float delaySeconds) |
Hides an actor during the animation. | |
Static Public Member Functions | |
static Animation | New (float durationSeconds) |
Creates an initialized Animation. | |
static Animation | DownCast (BaseHandle handle) |
Downcasts a handle to Animation handle. |
Detailed Description
Dali::Animation can be used to animate the properties of any number of objects, typically Actors.
An example animation setup is shown below:
struct MyProgram { Actor mActor; // The object we wish to animate Animation mAnimation; // Keep this to control the animation } // ...To play the animation mAnimation = Animation::New(3.0f); // duration 3 seconds mAnimation.AnimateTo(Property(mActor, Actor::Property::POSITION), Vector3(10.0f, 50.0f, 0.0f)); mAnimation.Play();
Dali::Animation supports "fire and forget" behaviour i.e. an animation continues to play if the handle is discarded. Note that in the following example, the "Finish" signal will be emitted:
void ExampleCallback( Animation& source ) { std::cout << "Animation has finished" << std::endl; } void ExampleAnimation( Actor actor ) { Animation animation = Animation::New(2.0f); // duration 2 seconds animation.AnimateTo(Property(actor, Actor::Property::POSITION), 10.0f, 50.0f, 0.0f); animation.FinishedSignal().Connect( ExampleCallback ); animation.Play(); } // At this point the animation handle has gone out of scope Actor actor = Actor::New(); Stage::GetCurrent().Add( actor ); // Fire animation and forget about it ExampleAnimation( actor ); // However the animation will continue, and "Animation has finished" will be printed after 2 seconds.
If the "Finish" signal is connected to a member function of an object, it must be disconnected before the object is destroyed. This is typically done in the object destructor, and requires either the Dali::Connection object or Dali::Animation handle to be stored.
The overall animation time is superseded by the values given in the TimePeriod structure used when calling the AnimateTo(), AnimateBy(), AnimateBetween() and Animate() methods. If any of the individual calls to those functions exceeds the overall animation time, then the overall animation time is automatically extended.
Using AnimateTo and AnimateBy for the same property of the same Actor will yield undefined behaviour especially if the TimePeriod overlaps.
After calling Animation::Play(), Handle::GetProperty will return the target value of the animated property.
Signals | Signal Name | Method | |--------------|--------------------------| | finished | FinishedSignal() |
Actions | Action Name | Animation method called | |--------------|--------------------------| | play | Play() | | stop | Stop() | | pause | Pause() |
- Since:
- 2.4, DALi version 1.0.0
Member Typedef Documentation
typedef Signal< void (Animation&) > Dali::Animation::AnimationSignalType |
Animation finished signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Any Dali::Animation::AnyFunction |
Interpolation function.
- Since:
- 2.4, DALi version 1.0.0
Member Enumeration Documentation
Enumeration for what to do when the animation ends, is stopped, or is destroyed.
- Since:
- 2.4, DALi version 1.0.0
- Enumerator:
Enumeration for what interpolation method to use on key-frame animations.
- Since:
- 2.4, DALi version 1.0.0
Enumeration for what looping mode is in.
- Since:
- 4.0, DALi version 1.2.60
- Enumerator:
Enumeration for what state the animation is in.
Note: Calling Reset() on this class will NOT reset the animation. It will call BaseHandle::Reset() which drops the object handle.
- Since:
- 3.0, DALi version 1.1.21
- Enumerator:
STOPPED Animation has stopped.
- Since:
- 3.0, DALi version 1.1.21
PLAYING The animation is playing.
- Since:
- 3.0, DALi version 1.1.21
PAUSED The animation is paused.
- Since:
- 3.0, DALi version 1.1.21
Constructor & Destructor Documentation
Creates an uninitialized Animation; this can be initialized with Animation::New().
Calling member functions with an uninitialized Animation handle is not allowed.
- Since:
- 2.4, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::Animation::Animation | ( | const Animation & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
void Dali::Animation::Animate | ( | Actor | actor, |
Path | path, | ||
const Vector3 & | forward | ||
) |
Animates an actor's position and orientation through a predefined path.
The actor will rotate to orient the supplied forward vector with the path's tangent. If forward is the zero vector then no rotation will happen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to animate [in] path The path. It defines position and orientation [in] forward The vector (in local space coordinate system) that will be oriented with the path's tangent direction
void Dali::Animation::Animate | ( | Actor | actor, |
Path | path, | ||
const Vector3 & | forward, | ||
AlphaFunction | alpha | ||
) |
Animates an actor's position and orientation through a predefined path.
The actor will rotate to orient the supplied forward vector with the path's tangent. If forward is the zero vector then no rotation will happen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to animate [in] path The path. It defines position and orientation [in] forward The vector (in local space coordinate system) that will be oriented with the path's tangent direction [in] alpha The alpha function to apply
void Dali::Animation::Animate | ( | Actor | actor, |
Path | path, | ||
const Vector3 & | forward, | ||
TimePeriod | period | ||
) |
Animates an actor's position and orientation through a predefined path.
The actor will rotate to orient the supplied forward vector with the path's tangent. If forward is the zero vector then no rotation will happen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to animate [in] path The path. It defines position and orientation [in] forward The vector (in local space coordinate system) that will be oriented with the path's tangent direction [in] period The effect will occur during this time period
void Dali::Animation::Animate | ( | Actor | actor, |
Path | path, | ||
const Vector3 & | forward, | ||
AlphaFunction | alpha, | ||
TimePeriod | period | ||
) |
Animates an actor's position and orientation through a predefined path.
The actor will rotate to orient the supplied forward vector with the path's tangent. If forward is the zero vector then no rotation will happen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to animate [in] path The path. It defines position and orientation [in] forward The vector (in local space coordinate system) that will be oriented with the path's tangent direction [in] alpha The alpha function to apply [in] period The effect will occur during this time period
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
Interpolation | interpolation | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] interpolation The method used to interpolate between values
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
AlphaFunction | alpha | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] alpha The alpha function to apply
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
AlphaFunction | alpha, | ||
Interpolation | interpolation | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] alpha The alpha function to apply [in] interpolation The method used to interpolate between values
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
TimePeriod | period | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] period The effect will occur during this time period
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
TimePeriod | period, | ||
Interpolation | interpolation | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] period The effect will occur during this time period [in] interpolation The method used to interpolate between values
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
AlphaFunction | alpha, | ||
TimePeriod | period | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] alpha The alpha function to apply [in] period The effect will occur during this time period
void Dali::Animation::AnimateBetween | ( | Property | target, |
KeyFrames & | keyFrames, | ||
AlphaFunction | alpha, | ||
TimePeriod | period, | ||
Interpolation | interpolation | ||
) |
Animates a property between keyframes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object property to animate [in] keyFrames The set of time/value pairs between which to animate [in] alpha The alpha function to apply to the overall progress [in] period The effect will occur during this time period [in] interpolation The method used to interpolate between values
void Dali::Animation::AnimateBy | ( | Property | target, |
Property::Value | relativeValue | ||
) |
Animates a property value by a relative amount.
The default alpha function will be used. The effect will start & end when the animation begins & ends.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] relativeValue The property value will change by this amount
void Dali::Animation::AnimateBy | ( | Property | target, |
Property::Value | relativeValue, | ||
AlphaFunction | alpha | ||
) |
Animates a property value by a relative amount.
The effect will start & end when the animation begins & ends.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] relativeValue The property value will change by this amount [in] alpha The alpha function to apply
void Dali::Animation::AnimateBy | ( | Property | target, |
Property::Value | relativeValue, | ||
TimePeriod | period | ||
) |
Animates a property value by a relative amount.
The default alpha function will be used.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] relativeValue The property value will increase/decrease by this amount [in] period The effect will occur during this time period
void Dali::Animation::AnimateBy | ( | Property | target, |
Property::Value | relativeValue, | ||
AlphaFunction | alpha, | ||
TimePeriod | period | ||
) |
Animates a property value by a relative amount.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] relativeValue The property value will increase/decrease by this amount [in] alpha The alpha function to apply [in] period The effect will occur during this time period
void Dali::Animation::AnimateTo | ( | Property | target, |
Property::Value | destinationValue | ||
) |
Animates a property to a destination value.
The default alpha function will be used. The effect will start & end when the animation begins & ends.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] destinationValue The destination value
void Dali::Animation::AnimateTo | ( | Property | target, |
Property::Value | destinationValue, | ||
AlphaFunction | alpha | ||
) |
Animates a property to a destination value.
The effect will start & end when the animation begins & ends.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] destinationValue The destination value [in] alpha The alpha function to apply
void Dali::Animation::AnimateTo | ( | Property | target, |
Property::Value | destinationValue, | ||
TimePeriod | period | ||
) |
Animates a property to a destination value.
The default alpha function will be used.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] destinationValue The destination value [in] period The effect will occur during this time period
void Dali::Animation::AnimateTo | ( | Property | target, |
Property::Value | destinationValue, | ||
AlphaFunction | alpha, | ||
TimePeriod | period | ||
) |
Animates a property to a destination value.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] target The target object/property to animate [in] destinationValue The destination value [in] alpha The alpha function to apply [in] period The effect will occur during this time period
void Dali::Animation::Clear | ( | ) |
Clears the animation.
This disconnects any objects that were being animated, effectively stopping the animation.
- Since:
- 2.4, DALi version 1.0.0
static Animation Dali::Animation::DownCast | ( | BaseHandle | handle | ) | [static] |
Connects to this signal to be notified when an Animation's animations have finished.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A signal object to connect with
int32_t Dali::Animation::GetCurrentLoop | ( | ) |
Gets the current loop count.
A value 0 to GetLoopCount() indicating the current loop count when looping.
- Since:
- 3.0, DALi version 1.1.20
- Returns:
- The current number of loops that have occured
float Dali::Animation::GetCurrentProgress | ( | ) |
Retrieves the current progress of the animation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current progress as a normalized value between [0,1]
Retrieves the default alpha function for an animation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The default alpha function
Returns the disconnect action.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The disconnect action
float Dali::Animation::GetDuration | ( | ) | const |
Retrieves the duration of an animation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The duration in seconds
EndAction Dali::Animation::GetEndAction | ( | ) | const |
Returns the end action of the animation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The end action
int32_t Dali::Animation::GetLoopCount | ( | ) |
Gets the loop count.
A zero is the same as SetLooping(true) ie repeat forever. The loop count is initially 1 for play once.
- Since:
- 3.0, DALi version 1.1.20
- Returns:
- The number of times to loop
LoopingMode Dali::Animation::GetLoopingMode | ( | ) | const |
Gets one of the current looping mode.
- Since:
- 4.0, DALi version 1.2.60
- Returns:
- The current looping mode
Vector2 Dali::Animation::GetPlayRange | ( | ) | const |
Gets the playing range.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The play range defined for the animation
float Dali::Animation::GetSpeedFactor | ( | ) | const |
Retrieves the speed factor of the animation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Speed factor
State Dali::Animation::GetState | ( | ) | const |
void Dali::Animation::Hide | ( | Actor | actor, |
float | delaySeconds | ||
) |
Hides an actor during the animation.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to animate [in] delaySeconds The initial delay from the start of the animation
bool Dali::Animation::IsLooping | ( | ) | const |
Queries whether the animation will loop.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the animation will loop
static Animation Dali::Animation::New | ( | float | durationSeconds | ) | [static] |
Creates an initialized Animation.
The animation will not loop. The default end action is "Bake". The default alpha function is linear.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] durationSeconds The duration in seconds
- Returns:
- A handle to a newly allocated Dali resource
- Note:
- durationSeconds can not be negative.
This assignment operator is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::Animation::Pause | ( | ) |
Pauses the animation.
- Since:
- 2.4, DALi version 1.0.0
void Dali::Animation::Play | ( | ) |
Play the animation.
- Since:
- 2.4, DALi version 1.0.0
void Dali::Animation::PlayAfter | ( | float | delaySeconds | ) |
Play the animation after a given delay time.
The delay time is not included in the looping time. When the delay time is negative value, it would treat as play immediately.
- Since:
- 4.0, DALi version 1.2.60
- Parameters:
-
[in] delaySeconds The delay time
void Dali::Animation::PlayFrom | ( | float | progress | ) |
Plays the animation from a given point.
The progress must be in the 0-1 interval or in the play range interval if defined ( See Animation::SetPlayRange ), otherwise, it will be ignored.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] progress A value between [0,1], or between the play range if specified, from where the animation should start playing
void Dali::Animation::SetCurrentProgress | ( | float | progress | ) |
Sets the progress of the animation.
The animation will play (or continue playing) from this point. The progress must be in the 0-1 interval or in the play range interval if defined ( See Animation::SetPlayRange ), otherwise, it will be ignored.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] progress The new progress as a normalized value between [0,1] or between the play range if specified
void Dali::Animation::SetDefaultAlphaFunction | ( | AlphaFunction | alpha | ) |
Sets the default alpha function for an animation.
This is applied to individual property animations, if no further alpha functions are supplied.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] alpha The default alpha function
void Dali::Animation::SetDisconnectAction | ( | EndAction | disconnectAction | ) |
Sets the disconnect action.
If any of the animated property owners are disconnected from the stage while the animation is being played, then this action is performed. Default action is to BakeFinal.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] disconnectAction The disconnect action
void Dali::Animation::SetDuration | ( | float | seconds | ) |
Sets the duration of an animation.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] seconds The duration in seconds
- Precondition:
- DurationSeconds must be greater than zero.
void Dali::Animation::SetEndAction | ( | EndAction | action | ) |
Sets the end action of the animation.
This action is performed when the animation ends or if it is stopped. Default end action is bake.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] action The end action
void Dali::Animation::SetLoopCount | ( | int32_t | count | ) |
Enables looping for 'count' repeats.
A zero is the same as SetLooping(true) i.e. repeat forever. This function resets the looping value and should not be used with SetLooping(bool). Setting this parameter does not cause the animation to Play().
- Since:
- 3.0, DALi version 1.1.20
- Parameters:
-
[in] count The number of times to loop
void Dali::Animation::SetLooping | ( | bool | looping | ) |
Sets whether the animation will loop.
This function resets the loop count and should not be used with SetLoopCount(int). Setting this parameter does not cause the animation to Play().
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] looping True if the animation will loop
void Dali::Animation::SetLoopingMode | ( | LoopingMode | loopingMode | ) |
Sets the looping mode.
Animation plays forwards and then restarts from the beginning or runs backwards again.
- Since:
- 4.0, DALi version 1.2.60
- Parameters:
-
[in] loopingMode The looping mode is one of RESTART and AUTO_REVERSE
void Dali::Animation::SetPlayRange | ( | const Vector2 & | range | ) |
Sets the playing range.
Animation will play between the values specified. Both values ( range.x and range.y ) should be between 0-1, otherwise they will be ignored. If the range provided is not in proper order ( minimum,maximum ), it will be reordered.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] range Two values between [0,1] to specify minimum and maximum progress. The animation will play between those values
void Dali::Animation::SetSpeedFactor | ( | float | factor | ) |
Specifies a speed factor for the animation.
The speed factor is a multiplier of the normal velocity of the animation. Values between [0,1] will slow down the animation and values above one will speed up the animation. It is also possible to specify a negative multiplier to play the animation in reverse.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] factor A value which will multiply the velocity
void Dali::Animation::Show | ( | Actor | actor, |
float | delaySeconds | ||
) |
Shows an actor during the animation.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to animate [in] delaySeconds The initial delay from the start of the animation
void Dali::Animation::Stop | ( | ) |
Stops the animation.
- Since:
- 2.4, DALi version 1.0.0