Tizen Native API
6.5
|
ScrollView contains actors that can be scrolled manually (via touch) or automatically. More...
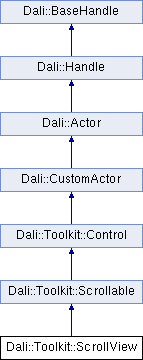
Classes | |
struct | ClampEvent |
Clamps signal event's data. More... | |
struct | Property |
Enumeration for the instance of properties belonging to the ScrollView class. More... | |
struct | SnapEvent |
Snaps signal event's data. More... | |
Public Types | |
enum | PropertyRange |
Enumeration for the start and end property ranges for this control. More... | |
typedef Signal< void(const SnapEvent &) > | SnapStartedSignalType |
SnapStarted signal type. | |
Public Member Functions | |
ScrollView () | |
Creates an empty ScrollView handle. | |
ScrollView (const ScrollView &handle) | |
Copy constructor. | |
ScrollView & | operator= (const ScrollView &handle) |
Assignment operator. | |
~ScrollView () | |
Destructor. | |
AlphaFunction | GetScrollSnapAlphaFunction () const |
Gets snap-animation's AlphaFunction. | |
void | SetScrollSnapAlphaFunction (AlphaFunction alpha) |
Sets snap-animation's AlphaFunction. | |
AlphaFunction | GetScrollFlickAlphaFunction () const |
Gets flick-animation's AlphaFunction. | |
void | SetScrollFlickAlphaFunction (AlphaFunction alpha) |
Sets flick-animation's AlphaFunction. | |
float | GetScrollSnapDuration () const |
Gets the time for the scroll snap-animation. | |
void | SetScrollSnapDuration (float time) |
Sets the time for the scroll snap-animation. | |
float | GetScrollFlickDuration () const |
Gets the time for the scroll flick-animation. | |
void | SetScrollFlickDuration (float time) |
Sets the time for the scroll flick-animation. | |
void | SetRulerX (RulerPtr ruler) |
Sets X axis ruler. | |
void | SetRulerY (RulerPtr ruler) |
Sets Y axis ruler. | |
void | SetScrollSensitive (bool sensitive) |
Sets scroll sensitivity of pan gesture. | |
void | SetMaxOvershoot (float overshootX, float overshootY) |
Sets maximum overshoot amount. | |
void | SetSnapOvershootAlphaFunction (AlphaFunction alpha) |
Sets Snap Overshoot animation's AlphaFunction. | |
void | SetSnapOvershootDuration (float duration) |
Sets Snap Overshoot animation's Duration. | |
void | SetActorAutoSnap (bool enable) |
Enables or Disables Actor Auto-Snap mode. | |
void | SetWrapMode (bool enable) |
Enables or Disables Wrap mode for ScrollView contents. | |
int | GetScrollUpdateDistance () const |
Gets the current distance needed to scroll for ScrollUpdatedSignal to be emitted. | |
void | SetScrollUpdateDistance (int distance) |
Sets the distance needed to scroll for ScrollUpdatedSignal to be emitted. | |
bool | GetAxisAutoLock () const |
Returns state of Axis Auto Lock mode. | |
void | SetAxisAutoLock (bool enable) |
Enables or Disables Axis Auto Lock mode for panning within the ScrollView. | |
float | GetAxisAutoLockGradient () const |
Gets the gradient threshold at which a panning gesture should be locked to the Horizontal or Vertical axis. | |
void | SetAxisAutoLockGradient (float gradient) |
Sets the gradient threshold at which a panning gesture should be locked to the Horizontal or Vertical axis. | |
float | GetFrictionCoefficient () const |
Gets the friction coefficient setting for ScrollView when flicking in free panning mode. | |
void | SetFrictionCoefficient (float friction) |
Sets the friction coefficient for ScrollView when flicking in free panning mode. | |
float | GetFlickSpeedCoefficient () const |
Gets the flick speed coefficient for ScrollView when flicking in free panning mode. | |
void | SetFlickSpeedCoefficient (float speed) |
Sets the flick speed coefficient for ScrollView when flicking in free panning mode. | |
Vector2 | GetMinimumDistanceForFlick () const |
Returns the minimum pan distance required for a flick gesture in pixels. | |
void | SetMinimumDistanceForFlick (const Vector2 &distance) |
Sets the minimum pan distance required for a flick in pixels. | |
float | GetMinimumSpeedForFlick () const |
Returns the minimum pan speed required for a flick gesture in pixels per second. | |
void | SetMinimumSpeedForFlick (float speed) |
Sets the minimum pan speed required for a flick in pixels per second. | |
float | GetMaxFlickSpeed () const |
Gets the maximum flick speed setting for ScrollView when flicking in free panning mode. | |
void | SetMaxFlickSpeed (float speed) |
Sets the maximum flick speed for the ScrollView when flicking in free panning mode. | |
Vector2 | GetWheelScrollDistanceStep () const |
Gets the step of scroll distance in actor coordinates for each wheel event received in free panning mode. | |
void | SetWheelScrollDistanceStep (Vector2 step) |
Sets the step of scroll distance in actor coordinates for each wheel event received in free panning mode. | |
Vector2 | GetCurrentScrollPosition () const |
Retrieves current scroll position. | |
unsigned int | GetCurrentPage () const |
Retrieves current scroll page based on ScrollView dimensions being the size of one page, and all pages laid out in a grid fashion, increasing from left to right until the end of the X-domain. | |
void | ScrollTo (const Vector2 &position) |
Scrolls View to position specified (contents will scroll to this position). | |
void | ScrollTo (const Vector2 &position, float duration) |
Scrolls View to position specified (contents will scroll to this position). | |
void | ScrollTo (const Vector2 &position, float duration, AlphaFunction alpha) |
Scrolls View to position specified (contents will scroll to this position). | |
void | ScrollTo (const Vector2 &position, float duration, DirectionBias horizontalBias, DirectionBias verticalBias) |
Scrolls View to position specified (contents will scroll to this position). | |
void | ScrollTo (const Vector2 &position, float duration, AlphaFunction alpha, DirectionBias horizontalBias, DirectionBias verticalBias) |
Scrolls View to position specified (contents will scroll to this position). | |
void | ScrollTo (unsigned int page) |
Scrolls View to page currently based on assumption that each page is "(page) * ScrollViewSize.width, 0". | |
void | ScrollTo (unsigned int page, float duration) |
Scrolls View to page currently based on assumption that each page is "(page) * ScrollViewSize.width, 0". | |
void | ScrollTo (unsigned int page, float duration, DirectionBias bias) |
Scrolls View to page currently based on assumption that each page is "(page) * ScrollViewSize.width, 0". | |
void | ScrollTo (Actor &actor) |
Scrolls View such that actor appears in the center of the ScrollView. | |
void | ScrollTo (Actor &actor, float duration) |
Scrolls View such that actor appears in the center of the ScrollView. | |
bool | ScrollToSnapPoint () |
Scrolls View to the nearest snap points as specified by the Rulers. | |
void | ApplyConstraintToChildren (Constraint constraint) |
Applies a constraint that will affect the children of ScrollView. | |
void | RemoveConstraintsFromChildren () |
Removes all constraints that will affect the children of ScrollView. | |
void | ApplyEffect (ScrollViewEffect effect) |
Applies Effect to ScrollView. | |
void | RemoveEffect (ScrollViewEffect effect) |
Removes Effect from ScrollView. | |
void | RemoveAllEffects () |
Remove All Effects from ScrollView. | |
void | BindActor (Actor child) |
Binds actor to this ScrollView. | |
void | UnbindActor (Actor child) |
Unbinds Actor from this ScrollView. | |
void | SetScrollingDirection (Radian direction, Radian threshold=PanGestureDetector::DEFAULT_THRESHOLD) |
Allows the user to constrain the scroll view in a particular direction. | |
void | RemoveScrollingDirection (Radian direction) |
Removes a direction constraint from the scroll view. | |
SnapStartedSignalType & | SnapStartedSignal () |
Signal emitted when the ScrollView has started to snap or flick (it tells the target position, scale, rotation for the snap or flick). | |
Static Public Member Functions | |
static ScrollView | New () |
Creates an initialized ScrollView. | |
static ScrollView | DownCast (BaseHandle handle) |
Downcasts a handle to ScrollView handle. |
Detailed Description
ScrollView contains actors that can be scrolled manually (via touch) or automatically.
Signals | Signal Name | Method | |-------------------|----------------------------| | snap-started | SnapStartedSignal() |
- Since:
- 2.4, DALi version 1.0.0
Member Typedef Documentation
typedef Signal< void ( const SnapEvent& ) > Dali::Toolkit::ScrollView::SnapStartedSignalType |
SnapStarted signal type.
- Since:
- 2.4, DALi version 1.0.0
Member Enumeration Documentation
Enumeration for the start and end property ranges for this control.
- Since:
- 2.4, DALi version 1.0.0
- Enumerator:
Reimplemented from Dali::Toolkit::Scrollable.
Constructor & Destructor Documentation
Creates an empty ScrollView handle.
- Since:
- 2.4, DALi version 1.0.0
Dali::Toolkit::ScrollView::ScrollView | ( | const ScrollView & | handle | ) |
Copy constructor.
Creates another handle that points to the same real object.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to copy from
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Member Function Documentation
void Dali::Toolkit::ScrollView::ApplyConstraintToChildren | ( | Constraint | constraint | ) |
Applies a constraint that will affect the children of ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] constraint The constraint to apply
- Note:
- This affects all existing and future Actors that are added to scrollview.
void Dali::Toolkit::ScrollView::ApplyEffect | ( | ScrollViewEffect | effect | ) |
Applies Effect to ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] effect The effect to apply to scroll view
void Dali::Toolkit::ScrollView::BindActor | ( | Actor | child | ) |
Binds actor to this ScrollView.
Once an actor is bound to a ScrollView, it will be subject to that ScrollView's properties.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The actor to add to this ScrollView
static ScrollView Dali::Toolkit::ScrollView::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to ScrollView handle.
If handle points to a ScrollView, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to an object
- Returns:
- A handle to a ScrollView or an uninitialized handle
Reimplemented from Dali::Toolkit::Scrollable.
bool Dali::Toolkit::ScrollView::GetAxisAutoLock | ( | ) | const |
Returns state of Axis Auto Lock mode.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Whether Axis Auto Lock mode has been enabled or not
float Dali::Toolkit::ScrollView::GetAxisAutoLockGradient | ( | ) | const |
Gets the gradient threshold at which a panning gesture should be locked to the Horizontal or Vertical axis.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The gradient, a value between 0.0 and 1.0f
unsigned int Dali::Toolkit::ScrollView::GetCurrentPage | ( | ) | const |
Retrieves current scroll page based on ScrollView dimensions being the size of one page, and all pages laid out in a grid fashion, increasing from left to right until the end of the X-domain.
- Since:
- 2.4, DALi version 1.0.0
- Note:
- Pages start from 0 as the first page, not 1.
- Returns:
- The Current page
Retrieves current scroll position.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current scroll position
float Dali::Toolkit::ScrollView::GetFlickSpeedCoefficient | ( | ) | const |
Gets the flick speed coefficient for ScrollView when flicking in free panning mode.
This is a constant which multiplies the input touch flick velocity to determine the actual velocity at which to move the scrolling area.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The flick speed coefficient is returned
float Dali::Toolkit::ScrollView::GetFrictionCoefficient | ( | ) | const |
Gets the friction coefficient setting for ScrollView when flicking in free panning mode.
This is a value in stage-diagonals per second^2. stage-diagonal = Length( stage.width, stage.height )
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Friction coefficient is returned
float Dali::Toolkit::ScrollView::GetMaxFlickSpeed | ( | ) | const |
Gets the maximum flick speed setting for ScrollView when flicking in free panning mode.
This is a value in stage-diagonals per second. stage-diagonal = Length( stage.width, stage.height )
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Maximum flick speed is returned
Returns the minimum pan distance required for a flick gesture in pixels.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Minimum pan distance vector with separate x and y distance
float Dali::Toolkit::ScrollView::GetMinimumSpeedForFlick | ( | ) | const |
Returns the minimum pan speed required for a flick gesture in pixels per second.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Minimum pan speed
Gets flick-animation's AlphaFunction.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Current easing alpha function of the flick animation
float Dali::Toolkit::ScrollView::GetScrollFlickDuration | ( | ) | const |
Gets the time for the scroll flick-animation.
This animation occurs when the user flicks scroll view.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The time in seconds for the animation to take
Gets snap-animation's AlphaFunction.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Current easing alpha function of the snap animation
float Dali::Toolkit::ScrollView::GetScrollSnapDuration | ( | ) | const |
Gets the time for the scroll snap-animation.
This animation occurs when the user drags, and releases.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The time in seconds for the animation to take
int Dali::Toolkit::ScrollView::GetScrollUpdateDistance | ( | ) | const |
Gets the current distance needed to scroll for ScrollUpdatedSignal to be emitted.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Current scroll update distance
Gets the step of scroll distance in actor coordinates for each wheel event received in free panning mode.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The step of scroll distance(pixel) in X and Y axes
static ScrollView Dali::Toolkit::ScrollView::New | ( | ) | [static] |
Creates an initialized ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to a newly allocated Dali resource
Reimplemented from Dali::Toolkit::Control.
ScrollView& Dali::Toolkit::ScrollView::operator= | ( | const ScrollView & | handle | ) |
Assignment operator.
Changes this handle to point to another real object.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle The handle to copy from
- Returns:
- A reference to this
Remove All Effects from ScrollView.
- Since:
- 2.4, DALi version 1.0.0
Removes all constraints that will affect the children of ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Note:
- This removes all constraints from actors that have been added to scrollview.
void Dali::Toolkit::ScrollView::RemoveEffect | ( | ScrollViewEffect | effect | ) |
Removes Effect from ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] effect The effect to remove
void Dali::Toolkit::ScrollView::RemoveScrollingDirection | ( | Radian | direction | ) |
Removes a direction constraint from the scroll view.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] direction The axis to stop constraining to. Usually will be PanGestureDetector::DIRECTION_VERTICAL or PanGestureDetector::DIRECTION_HORIZONTAL (but can be any other angle if desired).
void Dali::Toolkit::ScrollView::ScrollTo | ( | const Vector2 & | position | ) |
Scrolls View to position specified (contents will scroll to this position).
Position 0,0 is the origin. Increasing X scrolls contents left, while increasing Y scrolls contents up.
- If Rulers have been applied to the axes, then the contents will scroll until reaching the domain boundary.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The position to scroll to
- Note:
- Contents will not snap to ruler snap points.
void Dali::Toolkit::ScrollView::ScrollTo | ( | const Vector2 & | position, |
float | duration | ||
) |
Scrolls View to position specified (contents will scroll to this position).
Position 0,0 is the origin. Increasing X scrolls contents left, while increasing Y scrolls contents up.
- If Rulers have been applied to the axes, then the contents will scroll until reaching the domain boundary.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The position to scroll to [in] duration The duration of the animation in seconds
- Note:
- Contents will not snap to ruler snap points.
void Dali::Toolkit::ScrollView::ScrollTo | ( | const Vector2 & | position, |
float | duration, | ||
AlphaFunction | alpha | ||
) |
Scrolls View to position specified (contents will scroll to this position).
Position 0,0 is the origin. Increasing X scrolls contents left, while increasing Y scrolls contents up.
- If Rulers have been applied to the axes, then the contents will scroll until reaching the domain boundary.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The position to scroll to [in] duration The duration of the animation in seconds [in] alpha The alpha function to use
- Note:
- Contents will not snap to ruler snap points.
void Dali::Toolkit::ScrollView::ScrollTo | ( | const Vector2 & | position, |
float | duration, | ||
DirectionBias | horizontalBias, | ||
DirectionBias | verticalBias | ||
) |
Scrolls View to position specified (contents will scroll to this position).
Position 0,0 is the origin. Increasing X scrolls contents left, while increasing Y scrolls contents up.
- If Rulers have been applied to the axes, then the contents will scroll until reaching the domain boundary.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The position to scroll to [in] duration The duration of the animation in seconds [in] horizontalBias Whether to bias scrolling to left or right [in] verticalBias Whether to bias scrolling to top or bottom
- Note:
- Contents will not snap to ruler snap points. Biasing parameters are provided such that in scenarios with 2 or 2x2 pages in wrap mode, the application developer can decide whether to scroll left or right to get to the target page.
void Dali::Toolkit::ScrollView::ScrollTo | ( | const Vector2 & | position, |
float | duration, | ||
AlphaFunction | alpha, | ||
DirectionBias | horizontalBias, | ||
DirectionBias | verticalBias | ||
) |
Scrolls View to position specified (contents will scroll to this position).
Position 0,0 is the origin. Increasing X scrolls contents left, while increasing Y scrolls contents up.
- If Rulers have been applied to the axes, then the contents will scroll until reaching the domain boundary.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The position to scroll to [in] duration The duration of the animation in seconds [in] horizontalBias Whether to bias scrolling to left or right [in] verticalBias Whether to bias scrolling to top or bottom [in] alpha Alpha function to use
- Note:
- Contents will not snap to ruler snap points. Biasing parameters are provided such that in scenarios with 2 or 2x2 pages in wrap mode, the application developer can decide whether to scroll left or right to get to the target page.
void Dali::Toolkit::ScrollView::ScrollTo | ( | unsigned int | page | ) |
Scrolls View to page currently based on assumption that each page is "(page) * ScrollViewSize.width, 0".
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] page The page to scroll to
- Note:
- Should probably be upgraded so that page is an abstract class, that can be a function of ScrollViewSize, ruler domain, ruler snap points etc. as pages may be orchestrated in a 2D grid fashion, or variable width.
void Dali::Toolkit::ScrollView::ScrollTo | ( | unsigned int | page, |
float | duration | ||
) |
Scrolls View to page currently based on assumption that each page is "(page) * ScrollViewSize.width, 0".
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] page The page to scroll to [in] duration The duration of the animation in seconds
- Note:
- Should probably be upgraded so that page is an abstract class, that can be a function of ScrollViewSize, ruler domain, ruler snap points etc. as pages may be orchestrated in a 2D grid fashion, or variable width.
void Dali::Toolkit::ScrollView::ScrollTo | ( | unsigned int | page, |
float | duration, | ||
DirectionBias | bias | ||
) |
Scrolls View to page currently based on assumption that each page is "(page) * ScrollViewSize.width, 0".
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] page The page to scroll to [in] duration The duration of the animation in seconds [in] bias Whether to bias scrolling to left or right
- Note:
- Should probably be upgraded so that page is an abstract class, that can be a function of ScrollViewSize, ruler domain, ruler snap points etc. as pages may be orchestrated in a 2D grid fashion, or variable width. A biasing parameter is provided such that in scenarios with 2 pages in wrap mode, the application developer can decide whether to scroll left or right to get to the target page.
void Dali::Toolkit::ScrollView::ScrollTo | ( | Actor & | actor | ) |
Scrolls View such that actor appears in the center of the ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to center in on (via Scrolling)
- Note:
- Actor must be a direct child of ScrollView, otherwise will cause an assertion failure.
void Dali::Toolkit::ScrollView::ScrollTo | ( | Actor & | actor, |
float | duration | ||
) |
Scrolls View such that actor appears in the center of the ScrollView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor to center in on (via Scrolling) [in] duration The duration of the animation in seconds
- Note:
- Actor must be a direct child of ScrollView, otherwise will cause an assertion failure.
Scrolls View to the nearest snap points as specified by the Rulers.
If already at snap points, then will return false, and not scroll.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if Snapping necessary
void Dali::Toolkit::ScrollView::SetActorAutoSnap | ( | bool | enable | ) |
Enables or Disables Actor Auto-Snap mode.
When Actor Auto-Snap mode has been enabled, ScrollView will automatically snap to the closest actor (The closest actor will appear in the center of the ScrollView).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] enable Enables (true), or disables (false) Actor AutoSnap
void Dali::Toolkit::ScrollView::SetAxisAutoLock | ( | bool | enable | ) |
Enables or Disables Axis Auto Lock mode for panning within the ScrollView.
When enabled, any pan gesture that appears mostly horizontal or mostly vertical, will be automatically restricted to horizontal only or vertical only panning, until the pan gesture has completed.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] enable Enables (true), or disables (false) AxisAutoLock mode
void Dali::Toolkit::ScrollView::SetAxisAutoLockGradient | ( | float | gradient | ) |
Sets the gradient threshold at which a panning gesture should be locked to the Horizontal or Vertical axis.
By default, this is 0.36 (0.36:1) which means angles less than 20 degrees to an axis will lock to that axis.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] gradient A value between 0.0 and 1.0 (auto-lock for all angles)
- Note:
- Specifying a value of 1.0 (the maximum value accepted) indicates that all panning gestures will auto-lock either to the horizontal or vertical axis.
void Dali::Toolkit::ScrollView::SetFlickSpeedCoefficient | ( | float | speed | ) |
Sets the flick speed coefficient for ScrollView when flicking in free panning mode.
This is a constant which multiplies the input touch flick velocity to determine the actual velocity at which to move the scrolling area.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] speed The flick speed coefficient (default = 1.0)
void Dali::Toolkit::ScrollView::SetFrictionCoefficient | ( | float | friction | ) |
Sets the friction coefficient for ScrollView when flicking in free panning mode.
This is a value in stage-diagonals per second^2. stage-diagonal = Length( stage.width, stage.height ). example: A stage 480x800 in size has a diagonal length of 933. Friction coefficient of 1.0 means the swipe velocity will reduce by 1.0 * 933 pixels/sec^2.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] friction Friction coefficient must be greater than 0.0 (default = 1.0)
void Dali::Toolkit::ScrollView::SetMaxFlickSpeed | ( | float | speed | ) |
Sets the maximum flick speed for the ScrollView when flicking in free panning mode.
This is a value in stage-diagonals per second. stage-diagonal = Length( stage.width, stage.height ) example: A stage 480x800 in size has a diagonal length of 933. Max Flick speed of 1.0 means the maximum velocity of a swipe can be 1.0 * 933 pixels/sec.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] speed Maximum flick speed (default = 3.0)
void Dali::Toolkit::ScrollView::SetMaxOvershoot | ( | float | overshootX, |
float | overshootY | ||
) |
Sets maximum overshoot amount.
The final overshoot value is within 0.0f to 1.0f, but the maximum overshoot is in pixels (e.g. if you scroll 75 pixels beyond the edge of a scrollable area and the maximum overshoot is 100 then the final overshoot value will be 0.75f).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] overshootX The maximum number of horizontally scrolled pixels before overshoot X reaches 1.0f [in] overshootY The maximum number of vertically scrolled pixels before overshoot Y reaches 1.0f
void Dali::Toolkit::ScrollView::SetMinimumDistanceForFlick | ( | const Vector2 & | distance | ) |
Sets the minimum pan distance required for a flick in pixels.
Takes a Vector2 containing separate x and y values. As long as the pan distance exceeds one of these axes, a flick will be allowed.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] distance The minimum pan distance for a flick
void Dali::Toolkit::ScrollView::SetMinimumSpeedForFlick | ( | float | speed | ) |
Sets the minimum pan speed required for a flick in pixels per second.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] speed The minimum pan speed for a flick
void Dali::Toolkit::ScrollView::SetRulerX | ( | RulerPtr | ruler | ) |
Sets X axis ruler.
Defines how scrolling horizontally is snapped, and the boundary (domain) in which the ScrollView can pan.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] ruler The ruler to be used for the X axis
void Dali::Toolkit::ScrollView::SetRulerY | ( | RulerPtr | ruler | ) |
Sets Y axis ruler.
Defines how scrolling vertically is snapped, and the boundary (domain) in which the ScrollView can pan.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] ruler The ruler to be used for the Y axis
Sets flick-animation's AlphaFunction.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] alpha Easing alpha function of the flick animation
void Dali::Toolkit::ScrollView::SetScrollFlickDuration | ( | float | time | ) |
Sets the time for the scroll flick-animation.
This animation occurs when the user flicks scroll view.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] time The time in seconds for the animation to take
void Dali::Toolkit::ScrollView::SetScrollingDirection | ( | Radian | direction, |
Radian | threshold = PanGestureDetector::DEFAULT_THRESHOLD |
||
) |
Allows the user to constrain the scroll view in a particular direction.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] direction The axis to constrain the scroll-view to. Usually set to PanGestureDetector::DIRECTION_VERTICAL or PanGestureDetector::DIRECTION_HORIZONTAL (but can be any other angle if desired). [in] threshold The threshold to apply around the axis
- Note:
- If no threshold is specified, then the default threshold of PI * 0.25 radians (or 45 degrees) is used.
void Dali::Toolkit::ScrollView::SetScrollSensitive | ( | bool | sensitive | ) |
Sets scroll sensitivity of pan gesture.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] sensitive true
to enable scroll,false
to disable scrolling
- Note:
- Unlike Actor::Property::SENSITIVE, this determines whether this ScrollView should react (e.g. pan), without disrupting the sensitivity of its children.
Sets snap-animation's AlphaFunction.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] alpha Easing alpha function of the snap animation
void Dali::Toolkit::ScrollView::SetScrollSnapDuration | ( | float | time | ) |
Sets the time for the scroll snap-animation.
This animation occurs when the user drags, and releases.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] time The time in seconds for the animation to take
void Dali::Toolkit::ScrollView::SetScrollUpdateDistance | ( | int | distance | ) |
Sets the distance needed to scroll for ScrollUpdatedSignal to be emitted.
The scroll update distance tells ScrollView how far to move before ScrollUpdatedSignal the informs application. Each time the ScrollView crosses this distance the signal will be emitted.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] distance The distance for ScrollView to move before emitting update signal
Sets Snap Overshoot animation's AlphaFunction.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] alpha Easing alpha function of the overshoot snap animation
void Dali::Toolkit::ScrollView::SetSnapOvershootDuration | ( | float | duration | ) |
Sets Snap Overshoot animation's Duration.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] duration The duration of the overshoot snap animation
- Note:
- Set duration to 0 seconds to disable Animation.
Sets the step of scroll distance in actor coordinates for each wheel event received in free panning mode.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] step The step of scroll distance(pixel) in X and Y axes
- Note:
- If snap points are defined in the rulers, it will always scroll to the next snap point towards the scroll direction while receiving the wheel events.
void Dali::Toolkit::ScrollView::SetWrapMode | ( | bool | enable | ) |
Enables or Disables Wrap mode for ScrollView contents.
When enabled, the ScrollView contents are wrapped over the X/Y Domain.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] enable Enables (true), or disables (false) Wrap Mode
- Note:
- You must apply a position constraint that causes Wrapping to all children.
Signal emitted when the ScrollView has started to snap or flick (it tells the target position, scale, rotation for the snap or flick).
A callback of the following type may be connected:
void YourCallbackName(const SnapEvent& event);
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Object has been initialized.
void Dali::Toolkit::ScrollView::UnbindActor | ( | Actor | child | ) |
Unbinds Actor from this ScrollView.
Once Unbound, this ScrollView will not affect the actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The actor to be unbound
- Note:
- This does not remove the child from the ScrollView container